Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please follow the steps to write a small project This is the code that was done before: Step 1 : Transform General class into an
Please follow the steps to write a small project
This is the code that was done before:
Step: Transform General class into an abstract class
abstract class Generalval name: String
val maxHP: Int
var currentHP: Int
init
printlnGeneral $name created."
Step : Implement Simple Factory Pattern
object GeneralFactory
fun createGeneralname: String: General
return when name
"LiuBei" LiuBeiname
"CaoCao" CaoCaoname
"SunQuan" SunQuanname
else throw IllegalArgumentExceptionUnknown general name"
apply
currentHP maxHP
println$name has $maxHP health point."
Step : Implement Factory Method Pattern
abstract class GeneralFactory
abstract fun createRandomGeneral: General
class LordFactory : GeneralFactory
private val generatedGenerals mutableSetOf
override fun createRandomGeneral: General
val possibleGenerals listOfLiuBei "CaoCao", "SunQuan"
val availableGenerals possibleGenerals.filter it in generatedGenerals
val randomGeneralName availableGenerals.random
generatedGenerals.addrandomGeneralName
return GeneralFactory.createGeneralrandomGeneralName
NonLordFactory can be similarly implemented if needed
Final Step: Modify main function to use factories and add generals to the manager
fun main
val manager GeneralManager
val liuBei GeneralFactory.createGeneralLiuBei
val caoCao GeneralFactory.createGeneralCaoCao
val sunQuan GeneralFactory.createGeneralSunQuan
manager.addGeneralliuBei
manager.addGeneralcaoCao
manager.addGeneralsunQuan
val lordFactory LordFactory
for i in
val newLord lordFactory.createRandomGeneral
manager.addGeneralnewLord
val nonLordFactory NonLordFactory
for i in
val newNonLord nonLordFactory.createRandomGeneral
manager.addGeneralnewNonLord
val totalGenerals manager.getGeneralCount
printlnTotal number of generals: $totalGenerals"
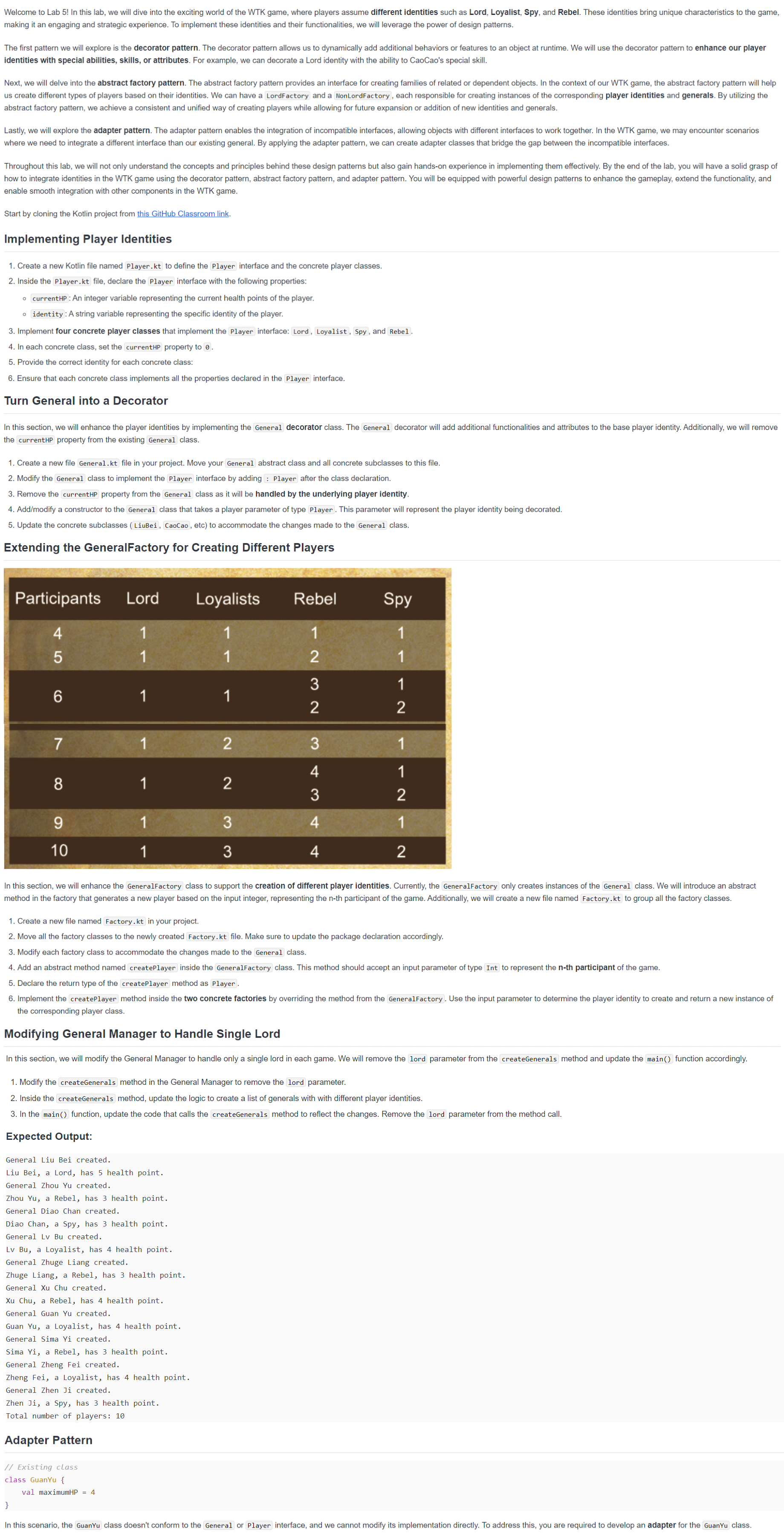
Step by Step Solution
There are 3 Steps involved in it
Step: 1
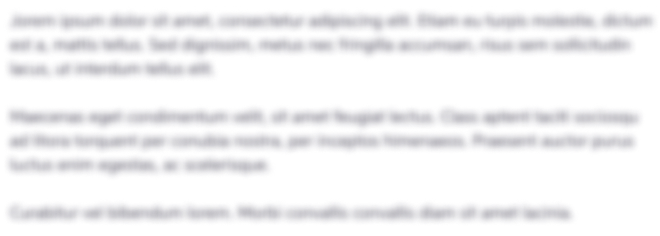
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started