Question
Please give me all necessary screenshot and comments . (I use Eclipse) Thanks /** * Implementation of {@link LongFifo} which uses a circular array internally.
Please give me all necessary screenshot and comments. (I use Eclipse) Thanks
/** * Implementation of {@link LongFifo} which uses a circular array internally. * * Look at the documentation in LongFifo to see how the methods are supposed to * work. * * The data is stored in the slots array. count is the number of items currently * in the FIFO. When the FIFO is not empty, head is the index of the next item * to remove. When the FIFO is not full, tail is the index of the next available * slot to use for an added item. Both head and tail need to jump to index 0 * when they "increment" past the last valid index of slots (this is what makes * it circular). * * See Circular Buffer * on Wikipedia for more information. */ public class CircularArrayLongFifo implements LongFifo { // do not change any of these fields: private final long[] slots; private int head; private int tail; private int count; private final Object lockObject;
// this constructor is correct as written - do not change public CircularArrayLongFifo(int fixedCapacity, Object proposedLockObject) {
lockObject = proposedLockObject != null ? proposedLockObject : new Object();
slots = new long[fixedCapacity]; head = 0; tail = 0; count = 0; }
// this constructor is correct as written - do not change public CircularArrayLongFifo(int fixedCapacity) { this(fixedCapacity, null); }
// this method is correct as written - do not change @Override public int getCount() { synchronized ( lockObject ) { return count; } }
@Override public boolean isEmpty() { // FIXME return false; }
@Override public boolean isFull() { // FIXME return false; }
@Override public void clear() { // FIXME }
@Override public int getCapacity() { // FIXME return 0; }
@Override public boolean add(long value) { // FIXME return false; }
@Override public LongFifo.RemoveResult remove() { // FIXME return null; }
// this method is correct as written - do not change @Override public Object getLockObject() { return lockObject; } } ==============================================================
import com.abc.sync.LongFifo.RemoveResult;
public class Demo { private static void simpleCheck() { LongFifo fifo = new CircularArrayLongFifo(5);
// expect: empty->true, full->false, count->0 System.out.println("fifo.isEmpty()=" + fifo.isEmpty()); System.out.println("fifo.isFull()=" + fifo.isFull()); System.out.println("fifo.getCount()=" + fifo.getCount());
fifo.add(5); fifo.add(7); fifo.add(3);
// expect: empty->false, full->false, count->3 System.out.println("fifo.isEmpty()=" + fifo.isEmpty()); System.out.println("fifo.isFull()=" + fifo.isFull()); System.out.println("fifo.getCount()=" + fifo.getCount());
RemoveResult rrA = fifo.remove(); if (rrA.isValid()) { long a = rrA.getValue(); System.out.println("a=" + a); } RemoveResult rrB = fifo.remove(); if (rrB.isValid()) { long b = rrB.getValue(); System.out.println("b=" + b); } RemoveResult rrC = fifo.remove(); if (rrC.isValid()) { long c = rrC.getValue(); System.out.println("c=" + c); } }
public static void main(String[] args) { simpleCheck(); } }
======================================================================
public interface LongFifo { /** Returns the number if items currently in the FIFO. */ int getCount();
/** Returns true if {@link #getCount()} == 0. */ boolean isEmpty();
/** Returns true if {@link #getCount()} == {@link #getCapacity()}. */ boolean isFull();
/** Removes any and all items in the FIFO leaving it in an empty state. */ void clear();
/** * Returns the maximum number of items which can be stored in this FIFO. * This value never changes. */ int getCapacity();
/** * Add if possible. If false is returned, the add was not possible (full). * If true is returned, the add was successful. */ boolean add(long value);
/** * Removes and returns the next item if there is one. * This method never returns null. * Check the isValid() method of RemoveResult: if true, then the remove * was successful. If false, the remove was not possible (empty). */ RemoveResult remove();
/** * Returns a reference to use for synchronized blocks which need to * call multiple methods without other threads being able to get in. * Never returns null. */ Object getLockObject();
/** * Used to allow callers of {@link #remove()} to see if a value was actually * removed or not. * Check {@link #isValid()} and only call {@link #getValue()} if true. * Instances are immutable. */ public static final class RemoveResult { /** * A shared, immutable instance which is never valid. */ public static final RemoveResult INVALID = new RemoveResult(false, 0);
private final boolean valid; private final long value;
private RemoveResult(boolean valid, long value) { this.valid = valid; this.value = value; }
public static RemoveResult createValid(long value) { return new RemoveResult(true, value); }
public static RemoveResult createInvalid() { return INVALID; }
/** * Returns true if {@link #getValue()} holds the value of the removed * item. If false, then getValue() cannot be called - nothing was * removed. */ public boolean isValid() { return valid; }
/** * Returns the value which was removed. * Only call this method if {@link #isValid()} returns true. * @throws IllegalStateException if there is no valid value. */ public long getValue() throws IllegalStateException { if (!valid) { throw new IllegalStateException( "invalid - cannot call getValue()"); } return value; } } // type RemoveResult }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
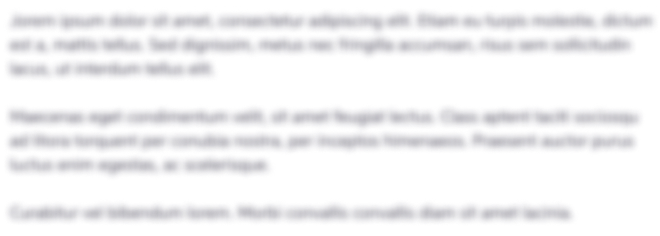
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started