Question
Please help fix my code. I'd like to write a java program that prompts the user for a list of words (quit to end the
Please help fix my code. I'd like to write a java program that prompts the user for a list of words ("quit" to end the list), then prints the list in sorted order.
My Code So far
import java.util.Scanner;
public class Lab15 {
public static void main(String[] args) {
System.out.println("Enter a list of words (enter 'quit' to quit) : ");
Scanner in = new Scanner(System.in);
String word = in.nextLine();
String[] list = new String[word];
do {
word = in.nextLine();
}while (!word.equals("quit"));
sortedList(list);
for (int i = 0; i < list.length; i++) {
System.out.println(list[i]);
}
in.close();
}
//insertion sort
public static void sortedList(String[] list ) {
String key;
for (int i = 0; i < list.length; ++i) {
key = list[i];
int j = i - 1;
if (j>i)
{
swap(list, i,j);
}
}
}
public static void swap(String[] list, int i, int j ) {
String pos=list[i];
list[i]=list[j];
list[j]=pos;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
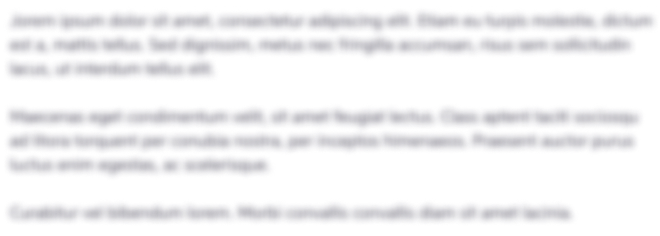
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started