Question
Please help inplement the RPNCalculator class (RPNCalculator.cpp) and ProducerConsumer class (producerconsumer.cpp). Instructions are below, and .hpp files are provided if they need to be referenced.
Please help inplement the RPNCalculator class (RPNCalculator.cpp) and ProducerConsumer class (producerconsumer.cpp). Instructions are below, and .hpp files are provided if they need to be referenced. Will rate!
.hpp files for reference:
rpncalculator:
/****************************************************************/
/* RPNCalculator Class */
/****************************************************************/
/* LEAVE THIS FILE AS IS! DO NOT MODIFY ANYTHING! =] */
/****************************************************************/
#pragma once
#include
struct Operand
{
float number;
Operand* next;
};
class RPNCalculator
{
public:
RPNCalculator();
~RPNCalculator();
bool isEmpty();
void push(float num);
void pop();
Operand* peek();
Operand* getStackHead() { return stackHead; } // no need to implement this
bool compute(std::string symbol);
private:
Operand* stackHead; // pointer to the top of the stack
};
producerconsumer.hpp:
/****************************************************************/
/* ProducerConsumer Class */
/****************************************************************/
/* LEAVE THIS FILE AS IS! DO NOT MODIFY ANYTHING! =] */
/****************************************************************/
#pragma once
#include
const int SIZE = 20; // max size of queue
class ProducerConsumer
{
public:
ProducerConsumer();
bool isEmpty();
bool isFull();
void enqueue(std::string player);
void dequeue();
int queueSize();
int getQueueFront() { return queueFront; } // no need to implement this
int getQueueEnd() { return queueEnd; } // no need to implement this
std::string* getQueue() { return queue; } // no need to implement this
std::string peek();
private:
int queueFront; // the index in queue[] that will be dequeued next
int queueEnd; // the index in queue[] that was most recently enqueued
std::string queue[SIZE]; // the queue in the form of an array
};
RPNCalculator C You will build an RPN calculator that can perform addition and multiplication on floating point numbers. This class utilizes the functionality of a linked list stack, which employs the following struct (included in RPNCalculator.hpp) struct Operand float number; Operand* next; l; Implement the RPNCalculator class according to the following specifications Operand* stackHead Points to the top of the stack (first node in the linked list). This is included in the class, no need to implement. RPNCalculator0) Constructor-set the stackHead pointer to NULL RPNCalculator) Destructor-pop everything off the stack and set stackhead to NULL. bool isEmpty0 Returns true if the stack is empty (i.e. stackHead is NULL), false otherwise void push(float number) Insert a new node with number onto the top of the stack (beginning of linked list) void pop(0 If the stack is empty, print "Stack empty, cannot pop an item. and return. Otherwise delete the top most item from the stack Operand* peek) If the stack is empty, print "Stack empty, cannot peek." and return NULL. Otherwise return a pointer to the top of the stack bool compute(std::string symbol) Perform the arithmetic operation symbol, which will be either+ or", on the top 2 numbers in the stack. The return value indicates whether the operation was carried out successfully If symbol is not "+or print "err: invalid operation" and return false #> 30 Invalid expression erConsumer Cla "Beware of edge cases that arise from the array being circular* In this class you will build a queue using the circular array implementation. Implement the methods of ProducerConsumer according to the following specifications. Note that in this queue implementation, the tail of the queue (queueEnd) is the index of the last item in the array and not the first available slot. By default, an empty queue will have head and tail indices (queueFront and queueEnd) set to -1 std::string queue[SIZE] A circular queue of strings in the form of an array. SIZE is initialized to a default value of 20 in ProducerConsumer.hpp int queueFront int queueEnd ProducerConsumer() Index in the array that keeps track of the front item Index in the array that keeps track of the last iterm Constructor-set queueFront and queueEnd to-1 bool isEmpty0 Return true if the queue is empty, false otherwise bool isFullO) Return true if the queue is full, false otherwise void enqueue(std::string item) If the queue is not full, then add the item to the end of the queue and modify queueFront and/or queueEnd if appropriate, else print "Queue full, cannot add new item" void dequeue() Remove the first item from the queue if the queue is not empty and modify queueFront and/or queueEnd if appropriate. Otherwise print "Queue empty, cannot dequeue an item" int queueSize() Return the number of items in the queue RPNCalculator C You will build an RPN calculator that can perform addition and multiplication on floating point numbers. This class utilizes the functionality of a linked list stack, which employs the following struct (included in RPNCalculator.hpp) struct Operand float number; Operand* next; l; Implement the RPNCalculator class according to the following specifications Operand* stackHead Points to the top of the stack (first node in the linked list). This is included in the class, no need to implement. RPNCalculator0) Constructor-set the stackHead pointer to NULL RPNCalculator) Destructor-pop everything off the stack and set stackhead to NULL. bool isEmpty0 Returns true if the stack is empty (i.e. stackHead is NULL), false otherwise void push(float number) Insert a new node with number onto the top of the stack (beginning of linked list) void pop(0 If the stack is empty, print "Stack empty, cannot pop an item. and return. Otherwise delete the top most item from the stack Operand* peek) If the stack is empty, print "Stack empty, cannot peek." and return NULL. Otherwise return a pointer to the top of the stack bool compute(std::string symbol) Perform the arithmetic operation symbol, which will be either+ or", on the top 2 numbers in the stack. The return value indicates whether the operation was carried out successfully If symbol is not "+or print "err: invalid operation" and return false #> 30 Invalid expression erConsumer Cla "Beware of edge cases that arise from the array being circular* In this class you will build a queue using the circular array implementation. Implement the methods of ProducerConsumer according to the following specifications. Note that in this queue implementation, the tail of the queue (queueEnd) is the index of the last item in the array and not the first available slot. By default, an empty queue will have head and tail indices (queueFront and queueEnd) set to -1 std::string queue[SIZE] A circular queue of strings in the form of an array. SIZE is initialized to a default value of 20 in ProducerConsumer.hpp int queueFront int queueEnd ProducerConsumer() Index in the array that keeps track of the front item Index in the array that keeps track of the last iterm Constructor-set queueFront and queueEnd to-1 bool isEmpty0 Return true if the queue is empty, false otherwise bool isFullO) Return true if the queue is full, false otherwise void enqueue(std::string item) If the queue is not full, then add the item to the end of the queue and modify queueFront and/or queueEnd if appropriate, else print "Queue full, cannot add new item" void dequeue() Remove the first item from the queue if the queue is not empty and modify queueFront and/or queueEnd if appropriate. Otherwise print "Queue empty, cannot dequeue an item" int queueSize() Return the number of items in the queueStep by Step Solution
There are 3 Steps involved in it
Step: 1
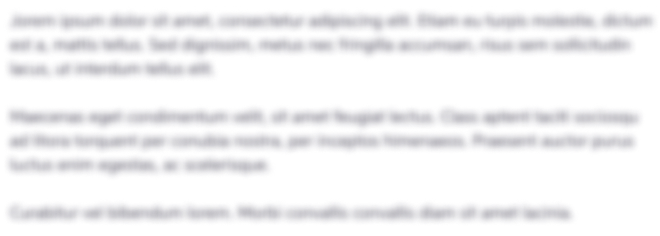
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started