Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please help me complete the java methods in the picture. Here is my code so far: public class DynamicNode { private Object info; private DynamicNode
Please help me complete the java methods in the picture.
Here is my code so far:
public class DynamicNode { private Object info; private DynamicNode next; public DynamicNode(Object x, DynamicNode n){ info = x; next = n; } public DynamicNode() { } public Object getInfo(){ return info; } public DynamicNode getNext(){ return next; } public void setInfo(Object x){ info = x; } public void setNext(DynamicNode n){ next = n; } } // end of DynamicNode class
public class DynamicList { private DynamicNode list; public DynamicList() { list = null; } public boolean isEmpty() { return list == null; } public void insertFirst(Object x) { DynamicNode q = new DynamicNode(x, null); if (isEmpty()) { q.setNext(list); } list = q; } // end of insertFirst public void insertAfter(DynamicNode p, Object x) { if (p == null) { System.out.println("void insertion"); System.exit(1); } DynamicNode q = new DynamicNode(x, p.getNext()); p.setNext(q); } // end of insertAfter public Object deleteFirst() { if (isEmpty()) { System.out.println("void deletion"); System.exit(1); } Object temp = list.getInfo(); if (list.getNext() = null) { list = null; } else { list = list.getNext(); } return temp; } // end of deleteFirst public Object deleteAfter(DynamicNode p) { if (p == null || p.getNext() == null) { System.out.println("void deletion"); System.exit(1); } DynamicNode q = p.getNext(); Object temp = q.getInfo(); p.setNext(q.setNext()); return temp; } // end of deleteAfter public void print() { // finish print method } public boolean appendList(DynamicList othrList) { // finish append list method } public void reverse() { // finish reverse method } public Object deleteMid(){ // finish delete mid } public static void main(String[] args) { } }
Here is the image of what needs to be done please:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
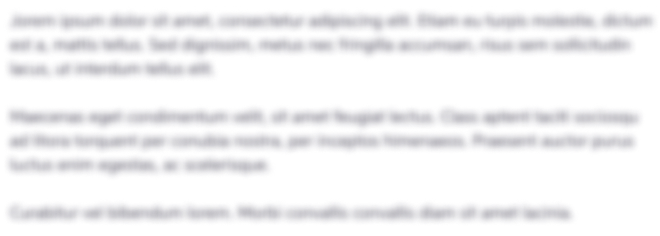
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started