Question
Please help me figure this out. Thanks!!!! Create a new java class and name it HealthProfile. Your HealthProfile class will be used to calculate a
Please help me figure this out. Thanks!!!!
Create a new java class and name it HealthProfile.
Your HealthProfile class will be used to calculate a person's BMI. It will require global variables for the patients first name, last name, gender, height, and weight. Use the comments and examples from zyBooks chapters 3 and 4 to guide you in completing this lab.
// Insert a program header comment describing the program; include your name and class in program header, for example:
/* Your Name
Name of Class
Module 1 Assignment - HealthProfile
Health Profile class definition
*/
public class HealthProfile{ --INSERT CODE HERE //Declare global variables for the patients first name, last name, gender, height, and weight, for example: private String firstName;
// Create a new health profile public HealthProfile(String fname, String lname, String myGender, double myHeight, double myWeight) { --INSERT CODE HERE // Assign constructor input parameter values to the global variables using the this keyword, for example: this.firstName = fname; }
// create get methods public String getFirstName() { --INSERT CODE HERE //return global variable first name, for example: return firstName; }
public String getLastName() { --INSERT CODE HERE //return last name }
public String getGender() { --INSERT CODE HERE //return gender }
public String getHeight() { --INSERT CODE HERE //return height }
public String getWeight() { --INSERT CODE HERE //return weight }
//create the following additional get methods:
--INSERT CODE HERE //Note: this method will return a double
//getBMI()
// return (getWeight() * 703) / (getHeight() * getHeight())
--INSERT CODE HERE //Note: this method will return void. Use the print statements provided in the comment block below.
//displayHealthProfile()
/* System.out.printf(" %nHEALTH PROFILE FOR:%s %s%n",getFirstName(), getLastName()) System.out.printf(" Gender: %s%n", getGender()) System.out.printf(" Height (in inches): %.1f%n", getHeight()) System.out.printf(" Weight (in pounds): %.1f%n", getWeight()) System.out.printf(" BMI: %f%n%n", getBMI()) System.out.println(" BMI VALUES") System.out.println(" Underweight: less than 18.5") System.out.println(" Normal: between 18.5 and 24.9") System.out.println(" Overweight: between 25 and 29.9") System.out.println(" Obese: 30 or greater") */
} // End of Health Profile
//main() method to invoke HealthProfile
public static void main(String[ ] args){
// Declare local variables for the patients first name, last name, gender, height, and weight. Assign a value to each variable (you can use the Scanner object to allow users to insert values instead- bonus points will be awarded), for example: String firstname = "Henry";
//Note height should be inches and weight should be pounds.
// create a new health profile object and include the variable names above as the input parameters, for example: HealthProfile myProfile = new HealthProfile(firstname, lastname, gender, height, weight);
//display the patients profile using the displayHealthProfile() method, for example: myProfile.displayHealthProfile();
}//End of main method }//End of Health Profile class
This is what I have put to together but keep getting errors when I try to compile it:
public class HealthProfile{ public String firstName; public String lastName; public String gender; public int height; public int weight; public int bMI; public HealthProfile(String fname, String lname, String myGender, int myHeight, int myWeight) { this.firstName = fname; this.lastName = lname; this.gender = myGender; this.height = myHeight; this.weight = myWeight; } public String getFirstName(){ return firstName; } public String getLastName(){ return lastName; } public String gender(){ return gender; } public int height(){ return height; } public int weight(){ return weight; } public int bmi() { return (weight()*703/(height()*height())); } void displayHealthProfile(){ System.out.printf(" %nHEALTH PROFILE FOR:%s %s%n",getFirstName(), getLastName()); System.out.printf(" Gender: %s%n", gender()); System.out.printf(" Height (in inches): %.1f%n", height()); System.out.printf(" Weight (in pounds): %.1f%n", weight()); System.out.printf(" BMI: %f%n%n", bmi()); System.out.println(" BMI VALUES"); System.out.println(" Underweight: less than 18.5"); System.out.println(" Normal: between 18.5 and 24.9"); System.out.println(" Overweight: between 25 and 29.9"); System.out.println(" Obese: 30 or greater") ; } HealthProfile test=new HealthProfile(); public static void main(String[] args) { String firstname = "John"; String lastname = "Doe"; String gender = "Male"; String height = "71"; String weight = "180"; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
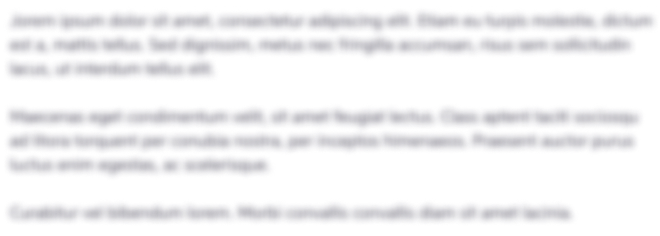
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started