Question
Please help me, my code won't pass all the tests, need to know what it should look like. The following is an example given: //
Please help me, my code won't pass all the tests, need to know what it should look like. The following is an example given: // Unit tests for the rectangle class class RectangleTest { Rectangle r1; Rectangle r2; Rectangle r3; @BeforeEach void runBefore() { r1 = new Rectangle(10, 15); r2 = new Rectangle(5, 2); r3 = new Rectangle(10, 10); } @Test void testArea() { assertEquals(10 * 15, r1.area()); assertEquals(5 * 2, r2.area()); } @Test void testIsTall() { assertTrue(r1.isTall()); assertFalse(r2.isTall()); assertFalse(r3.isTall()); } }
This is the problem:
Note that we have provided stubs for a constructor and for the following methods:
- isGreyScale - this method produces true if the colour is on the grey scale and false otherwise. Note that a colour is on the greyscale if its red, green and blue components are equal.
- toHex - this methods produces a hexadecimal representation of the colour. Don't worry if you're not familiar with this terminology - we provide details below.
Your task is to complete the design of the Colour class by doing the following (please do so in the order specified). If you get stuck at any point, please refer to the Rectangle example that we worked through earlier in the lab before asking for help from a TA.
- Open the ColourTest class and add examples of at least three colours to the runBefore method (you will also need to add corresponding fields to the class that can be used to reference these examples).
- Add examples for how the isGreyScale and toHex methods will be called in the corresponding test methods. If necessary, add further examples of Colour objects. In the process, you must make appropriate use of jUnit's assertEquals, assertTrue and assertFalse methods. Before you do this, please read the note on the hexadecimal representation of colours presented below.
Note: in order to have these methods available in your IDE, you might need to add one of these import statements to the top of your file: import static org.junit.jupiter.api.Assertions.*;
Run the tests. Note that you should be able to run them but they will not necessarily pass, given that we have only stubs in place for the isGreyScale andtoHexmethods.
- Add fields to the Colour class that are necessary to represent a colour.
- Complete the implementation of the constructor and methods specified in the Colour class.
Again, refer to section below on hexadecimal representation of colours for hints on how to implement the toHex method. The isGreyScale method will require you to construct a Boolean expression. Please see the Oracle Java Tutorial on Equality, Relational and Conditional Operators for related information.
- Run the tests and debug until all tests pass.
Hexadecimal representation of colours
If you have worked with drawing applications or with web design, you may have seen colours represented as strings of length 6 that look like "f8452a" or "20a30e". Each pair of digits represents one of the colours red, green and blue. So, for example, the colour "f8452a" represents the colour whose red, green and blue components are "f8", "45" and "2a".
In this lab, we will not go into the details of how to interpret these hexadecimal values but, if you're interested, you can find an overview on Wikipedia. All you need to know is how to convert a colour represented as RGB values in the range [0, 255] to a single hexadecimal string. First we combine the three RGB values into a single integer:
(r * 256 + g) * 256 + b
and then convert that integer to a hexadecimal string by passing it as an argument to the built-in method Integer.toHexString
There are some handy conversion applications on the web (use your favourite search engine to find one!) that will allow you to enter a decimal number and obtain the corresponding hexadecimal representation. Such an application will be handy when constructing your examples/tests for the toHex method.
Note: when given the value 0 as input, the Integer.toHexString method produces "0" rather than "000000" - keep this in mind when desigining your tests! Also note that the toHex method in the Colour class is also expected to produce "0", not "000000" to represent the colour whose red, green and blue components are all zero.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
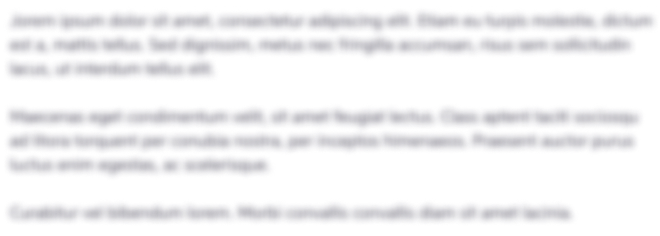
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started