Please help me on this assignment! It's Java!
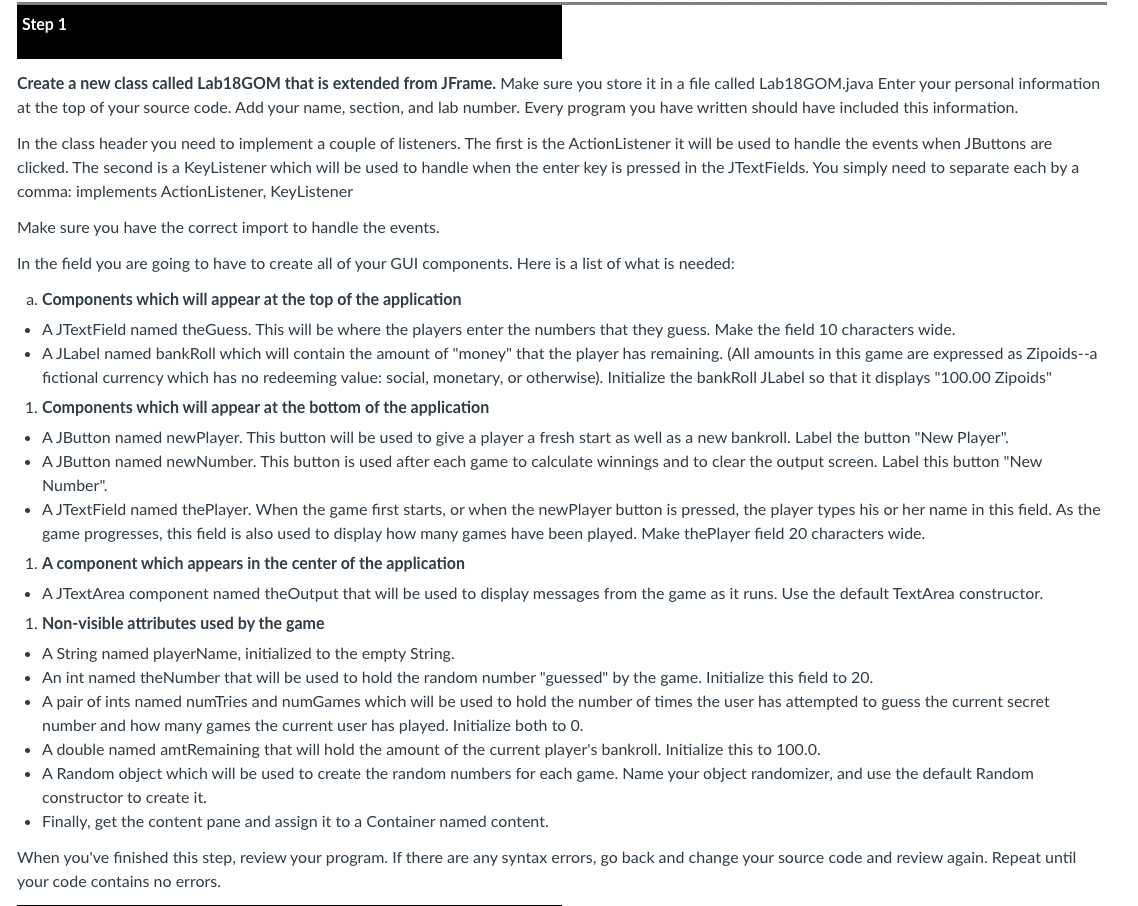
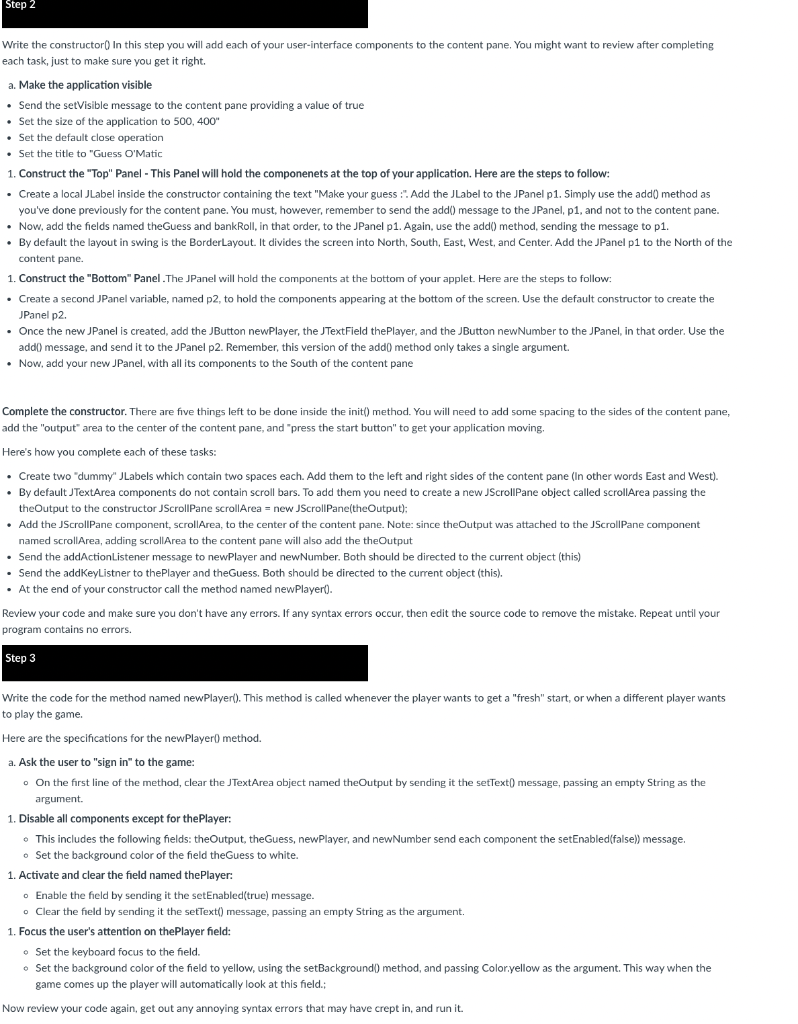
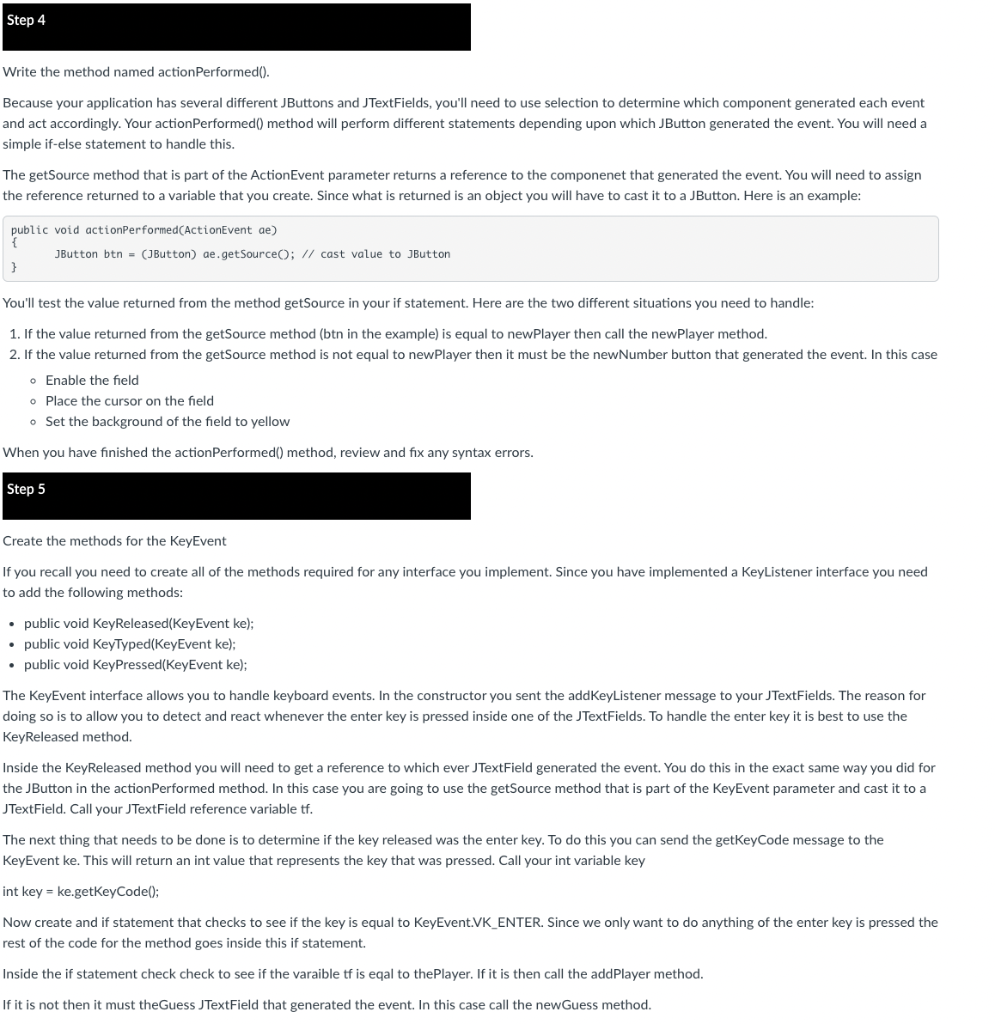
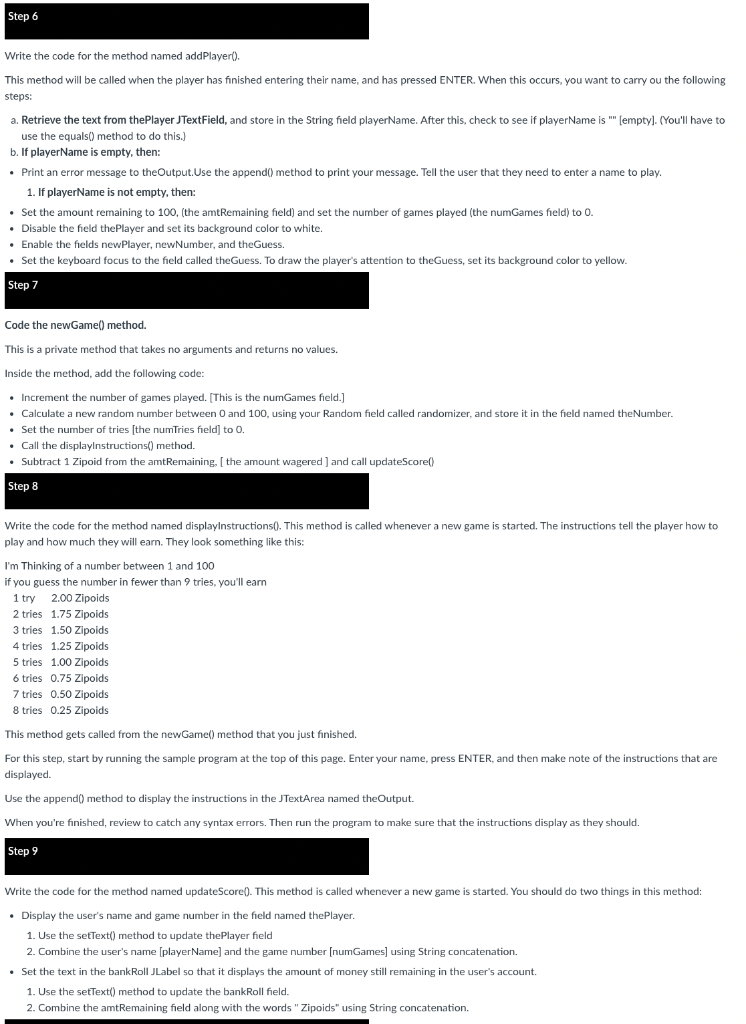
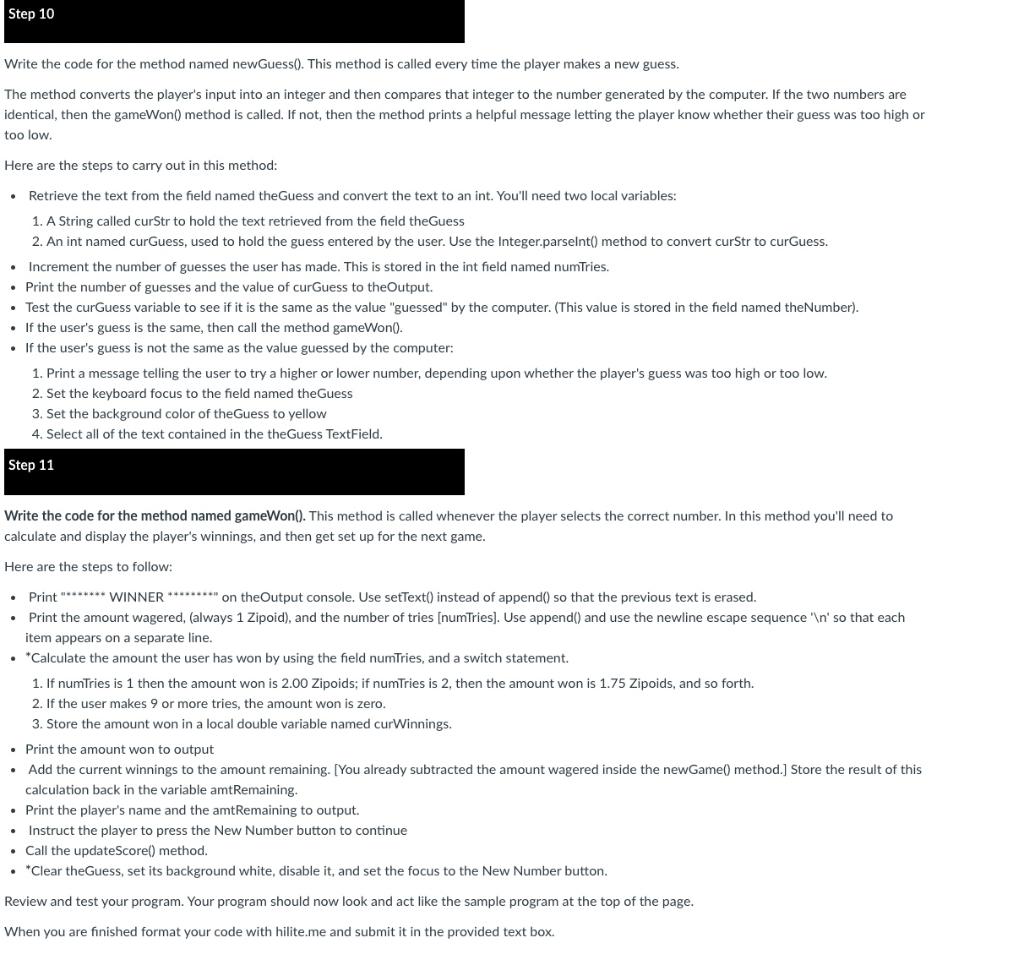
Create a new class called Lab18GOM that is extended from JFrame. Make sure you store it in a file called Lab18GOM.java Enter your personal information at the top of your source code. Add your name, section, and lab number. Every program you have written should have included this information. In the class header you need to implement a couple of listeners. The first is the ActionListener it will be used to handle the events when JButtons are clicked. The second is a KeyListener which will be used to handle when the enter key is pressed in the JTextFields. You simply need to separate each by a comma: implements ActionListener, KeyListener Make sure you have the correct import to handle the events. In the field you are going to have to create all of your GUI components. Here is a list of what is needed: a. Components which will appear at the top of the application - A JTextField named theGuess. This will be where the players enter the numbers that they guess. Make the field 10 characters wide. - A JLabel named bankRoll which will contain the amount of "money" that the player has remaining. (All amounts in this game are expressed as Zipoids--a fictional currency which has no redeeming value: social, monetary, or otherwise). Initialize the bankRoll JLabel so that it displays "100.00 Zipoids" 1. Components which will appear at the bottom of the application - A JButton named newPlayer. This button will be used to give a player a fresh start as well as a new bankroll. Label the button "New Player". - A JButton named newNumber. This button is used after each game to calculate winnings and to clear the output screen. Label this button "New Number". - A JTextField named thePlayer. When the game first starts, or when the newPlayer button is pressed, the player types his or her name in this field. As the game progresses, this field is also used to display how many games have been played. Make thePlayer field 20 characters wide. 1. A component which appears in the center of the application - A JTextArea component named theOutput that will be used to display messages from the game as it runs. Use the default TextArea constructor. 1. Non-visible attributes used by the game - A String named playerName, initialized to the empty String. - An int named theNumber that will be used to hold the random number "guessed" by the game. Initialize this field to 20. - A pair of ints named numTries and numGames which will be used to hold the number of times the user has attempted to guess the current secret number and how many games the current user has played. Initialize both to 0. - A double named amtRemaining that will hold the amount of the current player's bankroll. Initialize this to 100.0. - A Random object which will be used to create the random numbers for each game. Name your object randomizer, and use the default Random constructor to create it. - Finally, get the content pane and assign it to a Container named content. When you've finished this step, review your program. If there are any syntax errors, go back and change your source code and review again. Repeat until your code contains no errors. Step 4 Write the method named actionPerformed(). Because your application has several different JButtons and JTextFields, you'll need to use selection to determine which component generated each event and act accordingly. Your actionPerformed() method will perform different statements depending upon which JButton generated the event. You will need a simple if-else statement to handle this. The getSource method that is part of the ActionEvent parameter returns a reference to the componenet that generated the event. You will need to assign the reference returned to a variable that you create. Since what is returned is an object you will have to cast it to a JButton. Here is an example: public void actionPerformed(ActionEvent ae) {JButtonbtn=(JButton)ae.getSourceO;//castvaluetoJButton} You'll test the value returned from the method getSource in your if statement. Here are the two different situations you need to handle: 1. If the value returned from the getSource method (btn in the example) is equal to newPlayer then call the newPlayer method. 2. If the value returned from the getSource method is not equal to newPlayer then it must be the newNumber button that generated the event. In this case - Enable the field - Place the cursor on the field - Set the background of the field to yellow When you have finished the actionPerformed() method, review and fix any syntax errors. Step 5 Create the methods for the KeyEvent If you recall you need to create all of the methods required for any interface you implement. Since you have implemented a KeyListener interface you need to add the following methods: - public void KeyReleased(KeyEvent ke); - public void KeyTyped(KeyEvent ke); - public void KeyPressed(KeyEvent ke); The KeyEvent interface allows you to handle keyboard events. In the constructor you sent the addKeyListener message to your JTextFields. The reason for doing so is to allow you to detect and react whenever the enter key is pressed inside one of the JTextFields. To handle the enter key it is best to use the KeyReleased method. Inside the KeyReleased method you will need to get a reference to which ever JTextField generated the event. You do this in the exact same way you did for the JButton in the actionPerformed method. In this case you are going to use the getSource method that is part of the KeyEvent parameter and cast it to a JTextField. Call your JTextField reference variable tff The next thing that needs to be done is to determine if the key released was the enter key. To do this you can send the getKeyCode message to the KeyEvent ke. This will return an int value that represents the key that was pressed. Call your int variable key int key = ke.getKeyCode() Now create and if statement that checks to see if the key is equal to KeyEvent.VK_ENTER. Since we only want to do anything of the enter key is pressed the rest of the code for the method goes inside this if statement. Inside the if statement check check to see if the varaible tf is eqal to thePlayer. If it is then call the addPlayer method. If it is not then it must theGuess JTextField that generated the event. In this case call the newGuess method. Step 6 Write the code for the method named addPlayer(). This method will be called when the player has finished entering their name, and has pressed ENTER. When this occurs, you want to carry ou the following steps: a. Retrieve the text from thePlayer JTextField, and store in the String field playerName. After this, check to see if playerName is "" [empty]. (You'll have to use the equals() method to do this.] b. If playerName is empty, then: - Print an error message to theOutput. Use the append() method to print your message. Tell the user that they need to enter a name to play. 1. If playerName is not empty, then: - Set the amount remaining to 100 , (the amtRemaining field) and set the number of games played (the numGames field) to 0. - Disable the field thePlayer and set its background color to white. - Enable the fields newPlayer, newNumber, and theGuess. - Set the keyboard focus to the field called theGuess. To draw the player's attention to theGuess, set its background color to yellow. Step 7 Code the newGame() method. This is a private method that takes no arguments and returns no values. Inside the method, add the following code: - Increment the number of games played. [This is the numGames field.] - Calculate a new random number between 0 and 100, using your Random field called randomizer, and store it in the field named theNumber. - Set the number of tries [the numTries field] to 0. - Call the displaylnstructions() method. - Subtract 1 Zipoid from the amtRemaining, [ the amount wagered ] and call updateScore() Step 8 Write the code for the method named displaylnstructions 0 . This method is called whenever a new game is started. The instructions tell the player how to play and how much they will earn. They look something like this: I'm Thinking of a number between 1 and 100 if you guess the number in fewer than 9 tries, you'll earn 1 try 2.00 Zipoids 2 tries 1.75 Zipoids 3 tries 1.50 Zipoids 4 tries 1.25 Zipoids 5 tries 1.00 Zipoids 6 tries 0.75 Zipoids 7 tries 0.50 Zipoids 8 tries 0.25 Zipoids This method gets called from the newGame() method that you just finished. For this step, start by running the sample program at the top of this page. Enter your name, press ENTER, and then make note of the instructions that are displayed. Use the append() method to display the instructions in the JTextArea named theOutput. When you're finished, review to catch any syntax errors. Then run the program to make sure that the instructions display as they should. Step 9 Write the code for the method named updateScore). This method is called whenever a new game is started. You should do two things in this method: - Display the user's name and game number in the field named thePlayer. 1. Use the setText() method to update thePlayer field 2. Combine the user's name [playerName] and the game number [numGames] using String concatenation. - Set the text in the bankRoll JLabel so that it displays the amount of money still remaining in the user's account. 1. Use the setText() method to update the bankRoll field. 2. Combine the amtRemaining field along with the words " Zipoids" using String concatenation. Write the code for the method named newGuess(). This method is called every time the player makes a new guess. The method converts the player's input into an integer and then compares that integer to the number generated by the computer. If the two numbers are identical, then the gameWon() method is called. If not, then the method prints a helpful message letting the player know whether their guess was too high or too low. Here are the steps to carry out in this method: - Retrieve the text from the field named theGuess and convert the text to an int. You'll need two local variables: 1. A String called curStr to hold the text retrieved from the field theGuess 2. An int named curGuess, used to hold the guess entered by the user. Use the Integer.parselnt() method to convert curStr to curGuess. - Increment the number of guesses the user has made. This is stored in the int field named numTries. - Print the number of guesses and the value of curGuess to theOutput. - Test the curGuess variable to see if it is the same as the value "guessed" by the computer. (This value is stored in the field named theNumber). - If the user's guess is the same, then call the method gameWon(). - If the user's guess is not the same as the value guessed by the computer: 1. Print a message telling the user to try a higher or lower number, depending upon whether the player's guess was too high or too low. 2. Set the keyboard focus to the field named theGuess 3. Set the background color of theGuess to yellow 4. Select all of the text contained in the theGuess TextField. Step 11 Write the code for the method named gameWon(). This method is called whenever the player selects the correct number. In this method you'll need to calculate and display the player's winnings, and then get set up for the next game. Here are the steps to follow: - Print " WINNER ***....*" on theOutput console. Use setText() instead of append() so that the previous text is erased. - Print the amount wagered, (always 1 Zipoid), and the number of tries [numTries]. Use append() and use the newline escape sequence ' n ' so that each item appears on a separate line. - Calculate the amount the user has won by using the field numTries, and a switch statement. 1. If numTries is 1 then the amount won is 2.00 Zipoids; if numTries is 2, then the amount won is 1.75 Zipoids, and so forth. 2. If the user makes 9 or more tries, the amount won is zero. 3. Store the amount won in a local double variable named curWinnings. - Print the amount won to output - Add the current winnings to the amount remaining. [You already subtracted the amount wagered inside the newGame() method.] Store the result of this calculation back in the variable amtRemaining. - Print the player's name and the amtRemaining to output. - Instruct the player to press the New Number button to continue - Call the updatescore() method. - Clear theGuess, set its background white, disable it, and set the focus to the New Number button. Review and test your program. Your program should now look and act like the sample program at the top of the page. When you are finished format your code with hilite.me and submit it in the provided text box. Create a new class called Lab18GOM that is extended from JFrame. Make sure you store it in a file called Lab18GOM.java Enter your personal information at the top of your source code. Add your name, section, and lab number. Every program you have written should have included this information. In the class header you need to implement a couple of listeners. The first is the ActionListener it will be used to handle the events when JButtons are clicked. The second is a KeyListener which will be used to handle when the enter key is pressed in the JTextFields. You simply need to separate each by a comma: implements ActionListener, KeyListener Make sure you have the correct import to handle the events. In the field you are going to have to create all of your GUI components. Here is a list of what is needed: a. Components which will appear at the top of the application - A JTextField named theGuess. This will be where the players enter the numbers that they guess. Make the field 10 characters wide. - A JLabel named bankRoll which will contain the amount of "money" that the player has remaining. (All amounts in this game are expressed as Zipoids--a fictional currency which has no redeeming value: social, monetary, or otherwise). Initialize the bankRoll JLabel so that it displays "100.00 Zipoids" 1. Components which will appear at the bottom of the application - A JButton named newPlayer. This button will be used to give a player a fresh start as well as a new bankroll. Label the button "New Player". - A JButton named newNumber. This button is used after each game to calculate winnings and to clear the output screen. Label this button "New Number". - A JTextField named thePlayer. When the game first starts, or when the newPlayer button is pressed, the player types his or her name in this field. As the game progresses, this field is also used to display how many games have been played. Make thePlayer field 20 characters wide. 1. A component which appears in the center of the application - A JTextArea component named theOutput that will be used to display messages from the game as it runs. Use the default TextArea constructor. 1. Non-visible attributes used by the game - A String named playerName, initialized to the empty String. - An int named theNumber that will be used to hold the random number "guessed" by the game. Initialize this field to 20. - A pair of ints named numTries and numGames which will be used to hold the number of times the user has attempted to guess the current secret number and how many games the current user has played. Initialize both to 0. - A double named amtRemaining that will hold the amount of the current player's bankroll. Initialize this to 100.0. - A Random object which will be used to create the random numbers for each game. Name your object randomizer, and use the default Random constructor to create it. - Finally, get the content pane and assign it to a Container named content. When you've finished this step, review your program. If there are any syntax errors, go back and change your source code and review again. Repeat until your code contains no errors. Step 4 Write the method named actionPerformed(). Because your application has several different JButtons and JTextFields, you'll need to use selection to determine which component generated each event and act accordingly. Your actionPerformed() method will perform different statements depending upon which JButton generated the event. You will need a simple if-else statement to handle this. The getSource method that is part of the ActionEvent parameter returns a reference to the componenet that generated the event. You will need to assign the reference returned to a variable that you create. Since what is returned is an object you will have to cast it to a JButton. Here is an example: public void actionPerformed(ActionEvent ae) {JButtonbtn=(JButton)ae.getSourceO;//castvaluetoJButton} You'll test the value returned from the method getSource in your if statement. Here are the two different situations you need to handle: 1. If the value returned from the getSource method (btn in the example) is equal to newPlayer then call the newPlayer method. 2. If the value returned from the getSource method is not equal to newPlayer then it must be the newNumber button that generated the event. In this case - Enable the field - Place the cursor on the field - Set the background of the field to yellow When you have finished the actionPerformed() method, review and fix any syntax errors. Step 5 Create the methods for the KeyEvent If you recall you need to create all of the methods required for any interface you implement. Since you have implemented a KeyListener interface you need to add the following methods: - public void KeyReleased(KeyEvent ke); - public void KeyTyped(KeyEvent ke); - public void KeyPressed(KeyEvent ke); The KeyEvent interface allows you to handle keyboard events. In the constructor you sent the addKeyListener message to your JTextFields. The reason for doing so is to allow you to detect and react whenever the enter key is pressed inside one of the JTextFields. To handle the enter key it is best to use the KeyReleased method. Inside the KeyReleased method you will need to get a reference to which ever JTextField generated the event. You do this in the exact same way you did for the JButton in the actionPerformed method. In this case you are going to use the getSource method that is part of the KeyEvent parameter and cast it to a JTextField. Call your JTextField reference variable tff The next thing that needs to be done is to determine if the key released was the enter key. To do this you can send the getKeyCode message to the KeyEvent ke. This will return an int value that represents the key that was pressed. Call your int variable key int key = ke.getKeyCode() Now create and if statement that checks to see if the key is equal to KeyEvent.VK_ENTER. Since we only want to do anything of the enter key is pressed the rest of the code for the method goes inside this if statement. Inside the if statement check check to see if the varaible tf is eqal to thePlayer. If it is then call the addPlayer method. If it is not then it must theGuess JTextField that generated the event. In this case call the newGuess method. Step 6 Write the code for the method named addPlayer(). This method will be called when the player has finished entering their name, and has pressed ENTER. When this occurs, you want to carry ou the following steps: a. Retrieve the text from thePlayer JTextField, and store in the String field playerName. After this, check to see if playerName is "" [empty]. (You'll have to use the equals() method to do this.] b. If playerName is empty, then: - Print an error message to theOutput. Use the append() method to print your message. Tell the user that they need to enter a name to play. 1. If playerName is not empty, then: - Set the amount remaining to 100 , (the amtRemaining field) and set the number of games played (the numGames field) to 0. - Disable the field thePlayer and set its background color to white. - Enable the fields newPlayer, newNumber, and theGuess. - Set the keyboard focus to the field called theGuess. To draw the player's attention to theGuess, set its background color to yellow. Step 7 Code the newGame() method. This is a private method that takes no arguments and returns no values. Inside the method, add the following code: - Increment the number of games played. [This is the numGames field.] - Calculate a new random number between 0 and 100, using your Random field called randomizer, and store it in the field named theNumber. - Set the number of tries [the numTries field] to 0. - Call the displaylnstructions() method. - Subtract 1 Zipoid from the amtRemaining, [ the amount wagered ] and call updateScore() Step 8 Write the code for the method named displaylnstructions 0 . This method is called whenever a new game is started. The instructions tell the player how to play and how much they will earn. They look something like this: I'm Thinking of a number between 1 and 100 if you guess the number in fewer than 9 tries, you'll earn 1 try 2.00 Zipoids 2 tries 1.75 Zipoids 3 tries 1.50 Zipoids 4 tries 1.25 Zipoids 5 tries 1.00 Zipoids 6 tries 0.75 Zipoids 7 tries 0.50 Zipoids 8 tries 0.25 Zipoids This method gets called from the newGame() method that you just finished. For this step, start by running the sample program at the top of this page. Enter your name, press ENTER, and then make note of the instructions that are displayed. Use the append() method to display the instructions in the JTextArea named theOutput. When you're finished, review to catch any syntax errors. Then run the program to make sure that the instructions display as they should. Step 9 Write the code for the method named updateScore). This method is called whenever a new game is started. You should do two things in this method: - Display the user's name and game number in the field named thePlayer. 1. Use the setText() method to update thePlayer field 2. Combine the user's name [playerName] and the game number [numGames] using String concatenation. - Set the text in the bankRoll JLabel so that it displays the amount of money still remaining in the user's account. 1. Use the setText() method to update the bankRoll field. 2. Combine the amtRemaining field along with the words " Zipoids" using String concatenation. Write the code for the method named newGuess(). This method is called every time the player makes a new guess. The method converts the player's input into an integer and then compares that integer to the number generated by the computer. If the two numbers are identical, then the gameWon() method is called. If not, then the method prints a helpful message letting the player know whether their guess was too high or too low. Here are the steps to carry out in this method: - Retrieve the text from the field named theGuess and convert the text to an int. You'll need two local variables: 1. A String called curStr to hold the text retrieved from the field theGuess 2. An int named curGuess, used to hold the guess entered by the user. Use the Integer.parselnt() method to convert curStr to curGuess. - Increment the number of guesses the user has made. This is stored in the int field named numTries. - Print the number of guesses and the value of curGuess to theOutput. - Test the curGuess variable to see if it is the same as the value "guessed" by the computer. (This value is stored in the field named theNumber). - If the user's guess is the same, then call the method gameWon(). - If the user's guess is not the same as the value guessed by the computer: 1. Print a message telling the user to try a higher or lower number, depending upon whether the player's guess was too high or too low. 2. Set the keyboard focus to the field named theGuess 3. Set the background color of theGuess to yellow 4. Select all of the text contained in the theGuess TextField. Step 11 Write the code for the method named gameWon(). This method is called whenever the player selects the correct number. In this method you'll need to calculate and display the player's winnings, and then get set up for the next game. Here are the steps to follow: - Print " WINNER ***....*" on theOutput console. Use setText() instead of append() so that the previous text is erased. - Print the amount wagered, (always 1 Zipoid), and the number of tries [numTries]. Use append() and use the newline escape sequence ' n ' so that each item appears on a separate line. - Calculate the amount the user has won by using the field numTries, and a switch statement. 1. If numTries is 1 then the amount won is 2.00 Zipoids; if numTries is 2, then the amount won is 1.75 Zipoids, and so forth. 2. If the user makes 9 or more tries, the amount won is zero. 3. Store the amount won in a local double variable named curWinnings. - Print the amount won to output - Add the current winnings to the amount remaining. [You already subtracted the amount wagered inside the newGame() method.] Store the result of this calculation back in the variable amtRemaining. - Print the player's name and the amtRemaining to output. - Instruct the player to press the New Number button to continue - Call the updatescore() method. - Clear theGuess, set its background white, disable it, and set the focus to the New Number button. Review and test your program. Your program should now look and act like the sample program at the top of the page. When you are finished format your code with hilite.me and submit it in the provided text box