Question
Please help me to implement the following Stacklinked.cpp and StackArray.cpp in C++. I need it done by tommow. test6.cpp //-------------------------------------------------------------------- // // Laboratory 6 test6.cpp
Please help me to implement the following Stacklinked.cpp and StackArray.cpp in C++. I need it done by tommow.
test6.cpp
//--------------------------------------------------------------------
//
// Laboratory 6 test6.cpp
//
// Test program for the operations in the Stack ADT
//
//--------------------------------------------------------------------
#include
using namespace std;
#include "config.h"
#if !LAB6_TEST1
# include "StackArray.cpp"
#else
# include "StackLinked.cpp"
#endif
void print_help()
{
cout << endl << "Commands:" << endl;
cout << " H : Help (displays this message)" << endl;
cout << " +x : Push x" << endl;
cout << " - : Pop" << endl;
cout << " C : Clear" << endl;
cout << " E : Empty stack?" << endl;
cout << " F : Full stack?" << endl;
cout << " Q : Quit the test program" << endl;
cout << endl;
}
template
void test_stack(Stack
{
DataType testDataItem; // Stack data item
char cmd; // Input command
print_help();
do
{
testStack.showStructure(); // Output stack
cout << endl << "Command: "; // Read command
cin >> cmd;
if ( cmd == '+' )
cin >> testDataItem;
try {
switch ( cmd )
{
case 'H' : case 'h':
print_help();
break;
case '+' : // push
cout << "Push " << testDataItem << endl;
testStack.push(testDataItem);
break;
case '-' : // pop
cout << "Popped " << testStack.pop() << endl;
break;
case 'C' : case 'c' : // clear
cout << "Clear the stack" << endl;
testStack.clear();
break;
case 'E' : case 'e' : // isEmpty
if ( testStack.isEmpty() )
cout << "Stack is empty" << endl;
else
cout << "Stack is NOT empty" << endl;
break;
case 'F' : case 'f' : // isFull
if ( testStack.isFull() )
cout << "Stack is full" << endl;
else
cout << "Stack is NOT full" << endl;
break;
case 'Q' : case 'q' : // Quit test program
break;
default : // Invalid command
cout << "Inactive or invalid command" << endl;
}
}
catch (logic_error e) {
cout << "Error: " << e.what() << endl;
}
} while ( cin && cmd != 'Q' && cmd != 'q' );
}
int main() {
#if !LAB6_TEST1
cout << "Testing array implementation" << endl;
StackArray
test_stack(s1);
#else
cout << "Testing linked implementation" << endl;
StackLinked
test_stack(s2);
#endif
}
Config.h
/**
* Stack class (Lab 6) configuration file.
* Activate test #N by defining the corresponding LAB6_TESTN to have the value 1.
*/
#define LAB6_TEST1 0 // 0 => use array implementation, 1 => use linked impl.
Stack.h
//--------------------------------------------------------------------
//
// Laboratory 6 Stack.h
//
// Class declaration of the abstract class interface to be used as
// the basis for implementations of the Stack ADT.
//
//--------------------------------------------------------------------
// for the exception warnings
#pragma warning( disable : 4290 )
#ifndef STACK_H
#define STACK_H
#include
#include
using namespace std;
template
class Stack {
public:
static const int MAX_STACK_SIZE = 8;
virtual ~Stack();
virtual void push(const DataType& newDataItem) throw (logic_error) = 0;
virtual DataType pop() throw (logic_error) = 0;
virtual void clear() = 0;
virtual bool isEmpty() const = 0;
virtual bool isFull() const = 0;
virtual void showStructure() const = 0;
};
template
Stack
// Not worth having a separate class implementation file for the destuctor
{}
#endif // #ifndef STACK_H
StackArray.h
//--------------------------------------------------------------------
//
// Laboratory 6 StackLinked.h
//
// Class declaration for the linked implementation of the Stack ADT
//
//--------------------------------------------------------------------
#ifndef STACKARRAY_H
#define STACKARRAY_H
#include
#include
using namespace std;
#include "Stack.h"
template
class StackArray : public Stack
public:
StackArray(int maxNumber = Stack
StackArray(const StackArray& other);
StackArray& operator=(const StackArray& other);
~StackArray();
void push(const DataType& newDataItem) throw (logic_error);
DataType pop() throw (logic_error);
void clear();
bool isEmpty() const;
bool isFull() const;
void showStructure() const;
private:
int maxSize;
int top;
DataType* dataItems;
};
#endif //#ifndef STACKARRAY_H
StackLInked.h
//--------------------------------------------------------------------
//
// Laboratory 6 StackArray.h
//
// Class declaration for the array implementation of the Stack ADT
//
//--------------------------------------------------------------------
#ifndef STACKARRAY_H
#define STACKARRAY_H
#include
#include
using namespace std;
#include "Stack.h"
template
class StackLinked : public Stack
public:
StackLinked(int maxNumber = Stack
StackLinked(const StackLinked& other);
StackLinked& operator=(const StackLinked& other);
~StackLinked();
void push(const DataType& newDataItem) throw (logic_error);
DataType pop() throw (logic_error);
void clear();
bool isEmpty() const;
bool isFull() const;
void showStructure() const;
private:
class StackNode {
public:
StackNode(const DataType& nodeData, StackNode* nextPtr)
{
dataItem = nodeData;
next = nextPtr;
}
DataType dataItem;
StackNode* next;
};
StackNode* top;
};
#endif //#ifndef STACKARRAY_H
StackArray.cpp
#include "StackArray.h"
template
StackArray
{
}
template
StackArray
{
}
template
StackArray
{
}
template
StackArray
{
}
template
void StackArray
{
}
template
DataType StackArray
{
}
template
void StackArray
{
}
template
bool StackArray
{
return false;
}
template
bool StackArray
{
return false;
}
template
void StackArray
// Array implementation. Outputs the data items in a stack. If the
// stack is empty, outputs "Empty stack". This operation is intended
// for testing and debugging purposes only.
{
if( isEmpty() ) {
cout << "Empty stack." << endl;
}
else {
int j;
cout << "Top = " << top << endl;
for ( j = 0 ; j < maxSize ; j++ )
cout << j << "\t";
cout << endl;
for ( j = 0 ; j <= top ; j++ )
{
if( j == top )
{
cout << '[' << dataItems[j] << ']'<< "\t"; // Identify top
}
else
{
cout << dataItems[j] << "\t";
}
}
cout << endl;
}
cout << endl;
}
StackLinked.cpp
#include "StackLinked.h"
template
StackLinked
{
}
template
StackLinked
{
}
template
StackLinked
{
}
template
StackLinked
{
clear();
}
template
void StackLinked
{
}
template
DataType StackLinked
{
}
template
void StackLinked
{
}
template
bool StackLinked
{
return false;
}
template
bool StackLinked
{
return false;
}
template
void StackLinked
{
if( isEmpty() )
{
cout << "Empty stack" << endl;
}
else
{
cout << "Top\t";
for (StackNode* temp = top; temp != 0; temp = temp->next) {
if( temp == top ) {
cout << "[" << temp->dataItem << "]\t";
}
else {
cout << temp->dataItem << "\t";
}
}
cout << "Bottom" << endl;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
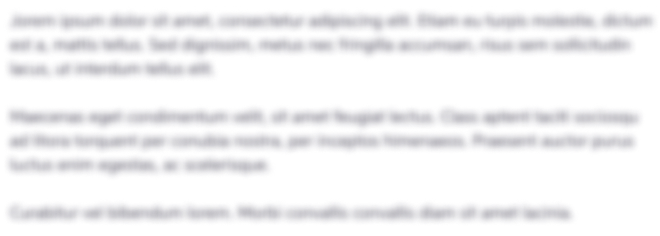
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started