Question
Please help me to make the Java program follow the below steps, ------------------------------------------------------- QuestionMain.Java ------------------------------------------------------ // 20 Questions // // To use the jGRASP debugger
Please help me to make the Java program follow the below steps,
-------------------------------------------------------
QuestionMain.Java
------------------------------------------------------
// 20 Questions // // To use the jGRASP debugger with this program, set a breakpoint // and once the execution breaks, open 'this' or 'tq' on the left, // then look at its variable 'tree'. That's your QuestionTree. // Drag your 'tree' over to the right to see a visualization of it. // // (Your QuestionTree is constructed by this file on line 30. // The overall loop to play games is around line 68.)
import java.io.*; import java.util.Scanner;
/** A basic text user interface for the 20 questions game. */ public class QuestionMain implements UserInterface { public static void main(String[] args) { QuestionMain tq = new QuestionMain(); tq.run(); } // fields private Scanner console; private QuestionTree tree; /** Constructs a text user interface and its question tree. */ public QuestionMain() { console = new Scanner(System.in); tree = new QuestionTree(this); } /** * Returns the user's response as a String. */ public String nextLine() { return console.nextLine(); }
/** Prints the given string to the console. */ public void print(String message) { System.out.print(message); System.out.print(" "); }
/** Prints the given string to the console. */ public void println(String message) { System.out.println(message); }
/** Prints a blank line to the console. */ public void println() { System.out.println(); }
/** * Waits for the user to answer a yeso question on the console and returns the * user's response as a boolean (true for anything that starts with "y" or "Y"). */ public boolean nextBoolean() { String answer = console.nextLine(); return answer.trim().toLowerCase().startsWith("y"); } // private helper for overall game(s) loop private void run() { println("Welcome to the game of 20 Questions!"); load(); // "Think of an item, and I will guess it in N tries." println(" " + BANNER_MESSAGE); do { // play one complete game println(); // blank line between games tree.play(); print(PLAY_AGAIN_MESSAGE); } while (nextBoolean()); // prompt to play again // print overall stats // Games played: N ... I have won: M println(" " + String.format(STATUS_MESSAGE, tree.totalGames(), tree.gamesWon()));
save(); } // common code for asking the user whether they want to save or load private void load() { print(LOAD_MESSAGE); if (nextBoolean()) { print(SAVE_LOAD_FILENAME_MESSAGE); String filename = nextLine(); try { Scanner in = new Scanner(new File(filename)); tree.load(in); } catch (FileNotFoundException e) { System.out.println("Error: " + e.getMessage()); } } } // common code for asking the user whether they want to save or load private void save() { print(SAVE_MESSAGE); if (nextBoolean()) { print(SAVE_LOAD_FILENAME_MESSAGE); String filename = nextLine(); try { PrintStream out = new PrintStream(new File(filename)); tree.save(out); out.close(); } catch (FileNotFoundException e) { System.out.println("Error: " + e.getMessage()); } } } }
-----------------------------------------------------
QuestionTree.java
-----------------------------------------------------
import java.util.*; import java.io.*;
public class QuestionTree {
UserInterface my;
public QuestionTree (UserInterface ui) { my = ui; } public void play() { my.println("Play Game here"); } public void save(PrintStream output) { my.println("Save the current tree here"); } public void load(Scanner input) { my.println("Save the current file here"); } public int totalGames() { return 0; } public int gamesWon() { return 0; } }
----------------------------------------------------------
UserInterface.java
--------------------------------------------------------
// 20 Questions
/** * Interface describing abstract user interaction operations. * This interface is implemented by the graphical and text UIs for the game. * Your QuestionTree interacts with the UI through this interface.
*/ public interface UserInterface { /** * Waits for the user to input a yeso answer (by typing, clicking, etc.), * and returns that answer as a boolean value. * @return the answer typed by the user as a boolean (yes is true, no is false) */ boolean nextBoolean();
/** * Waits for the user to input a text value, and returns that answer as a String. * @return the answer typed by the user as a String (empty string if no answer typed) */ String nextLine(); /** * Displays the given output message to the user. * @param message The message to display. Assumes not null. */ void print(String message); /** * Displays the given output message to the user. * If the UI is a text UI, also inserts a line break ( ). * @param message The message to display. Assumes not null. */ void println(String message);
// various messages that are output by the user interface // (your QuestionTree does not need to refer to these messages) final String PLAY_AGAIN_MESSAGE = "Challenge me again?"; final String SAVE_MESSAGE = "Shall I remember these games?"; final String LOAD_MESSAGE = "Shall I recall our previous games?"; final String SAVE_LOAD_FILENAME_MESSAGE = "What is the file name?"; final String STATUS_MESSAGE = "Games played: %d I won: %d"; final String BANNER_MESSAGE = "Think of an item, and I will guess it."; }
-------------------------------------------------
VaderMain.java
------------------------------------------------
// 20 Questions
import java.applet.*; import java.awt.*; import java.awt.event.*; import java.io.*; import java.net.*; import java.util.Scanner; import java.util.concurrent.*; import javax.swing.*;
/** * The graphical user interface (GUI) that talks to your QuestionTree * to play a graphical 20 questions game ("The Sith Sense"). */ public class VaderMain implements ActionListener, KeyListener, Runnable, UserInterface { private static final boolean CONSOLE_OUTPUT = true; // set to true to print I/O messages
// sound options private static final boolean MUSIC = true; private static final boolean SOUNDFX = true; private static final double SOUND_PERCENTAGE = 0.6; // how often Vader talks private static int NUM_SOUNDS = 17; // # of wav files (up to vader17.wav) private static int NUM_MUSICS = 9; // # of wav files (up to empire9.mid) // text messages that appear on the window // (these don't need to be constants, but it is bad practice to // scatter various strings and messages throughout a GUI program's code.) private static final String MUSIC_MESSAGE = "Music"; private static final String SFX_MESSAGE = "Sound FX"; private static final String YES_MESSAGE = "Yes"; private static final String NO_MESSAGE = "No"; private static final String ERROR_MESSAGE = "Error"; private static String TITLE = "The Sith Sense"; // file names, paths, URLs private static final String RESOURCE_URL = "http://www.cs.washington.edu/education/courses/cse143/12wi/homework/7/"; private static final String SAVE_DEFAULT_FILE_NAME = "memory.txt"; private static final String MUSIC_FILE_NAME = "empire%d.mid"; private static String BACKGROUND_IMAGE_FILE_NAME = "background.png"; private static String SOUND_FILE_NAME = "vader%d.wav";
// visual elements private static final Font FONT = new Font("SansSerif", Font.BOLD, 18); private static final Color COLOR = new Color(6, 226, 240); // light teal
// set to true if it is a trap; false if it is not a trap private static final boolean ITS_A_TRAP = false;
// runs the program public static void main(String[] args) { new VaderMain(); } // data fields private JFrame frame; private JLabel vader, bannerLabel; private JTextArea statsArea, messageLabel; private JTextField inputField; private JButton yesButton, noButton; private JCheckBox musicBox, soundBox; private AudioClip musicClip; private QuestionTree game; // these queues hold boolean or String user input waiting to be read private BlockingQueue
// constructs and sets up GUI and components public VaderMain() { if (ITS_A_TRAP) { // set up GUI settings to show the man himself, Admiral Ackbar TITLE = "It's a Trap!!!"; BACKGROUND_IMAGE_FILE_NAME = "ackbar.png"; SOUND_FILE_NAME = "ackbar%d.wav"; NUM_SOUNDS = 4; } game = new QuestionTree(this); // construct everybody frame = new JFrame(TITLE); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setResizable(false); frame.addKeyListener(this);
vader = new JLabel(); vader.setLayout(null); if (ensureFile(BACKGROUND_IMAGE_FILE_NAME)) { // vader.setIcon(new ImageIcon(ClassLoader.getSystemResource(BACKGROUND_IMAGE_FILE_NAME))); vader.setIcon(new ImageIcon(BACKGROUND_IMAGE_FILE_NAME)); }
// layout frame.add(vader); frame.pack(); center(frame);
// construct other components inputField = new JTextField(30); setupComponent(inputField, new Point(20, 180), new Dimension(300, 25)); inputField.setCaretColor(Color.GREEN); inputField.addActionListener(this); messageLabel = new JTextArea(); setupComponent(messageLabel, new Point(20, 120), new Dimension(365, 125)); messageLabel.setLineWrap(true); messageLabel.setWrapStyleWord(true); messageLabel.setEditable(false); messageLabel.setFocusable(false); bannerLabel = new JLabel(); setupComponent(bannerLabel, new Point(0, 0), new Dimension(vader.getWidth(), 30)); bannerLabel.setHorizontalAlignment(SwingConstants.CENTER);
statsArea = new JTextArea(); setupComponent(statsArea, new Point(vader.getWidth() - 200, vader.getHeight() - 50), new Dimension(200, 50)); statsArea.setEditable(false); statsArea.setFocusable(false); yesButton = makeButton(YES_MESSAGE, new Point(340, 50), new Dimension(80, 30)); noButton = makeButton(NO_MESSAGE, new Point(440, 50), new Dimension(80, 30)); yesButton.addKeyListener(this); noButton.addKeyListener(this);
musicBox = makeCheckBox(MUSIC_MESSAGE, MUSIC, new Point(vader.getWidth() - 200, vader.getHeight() - 120), new Dimension(120, 20)); soundBox = makeCheckBox(SFX_MESSAGE, SOUNDFX, new Point(vader.getWidth() - 200, vader.getHeight() - 95), new Dimension(120, 20)); musicBox.addKeyListener(this); soundBox.addKeyListener(this); doEnabling(); frame.setVisible(true);
// start background thread to loop and play the actual games // (it has to be in a thread so that the game loop can wait without // the GUI locking up) new Thread(this).start(); }
/** Handles user interactions with the graphical components. */ public void actionPerformed(ActionEvent event) { Object src = event.getSource(); if (src == yesButton) { yes(); } else if (src == noButton) { no(); } else if (src == inputField) { input(); } else if (src == musicBox) { // play or stop music if (musicClip != null) { if (musicBox.isSelected()) { musicClip.loop(); } else { musicClip.stop(); } } }
playSound(); } /** Part of the KeyListener interface. Responds to key presses. */ public void keyPressed(KeyEvent event) { if (!yesButton.isVisible() || event.isAltDown() || event.isControlDown()) { return; } char key = Character.toLowerCase(event.getKeyChar()); if (key == 'y') { yes(); } else if (key == 'n') { no(); } } /** Part of the KeyListener interface. Responds to key releases. */ public void keyReleased(KeyEvent event) {} /** Part of the KeyListener interface. Responds to key typing. */ public void keyTyped(KeyEvent event) {} /** Waits for the user to type a line of text and returns that line. */ public String nextLine() { return nextLine(null); }
/** Outputs the given text onto the GUI. */ public void print(String text) { messageLabel.setText(text); if (CONSOLE_OUTPUT) { System.out.print(text + " "); } } /** Outputs the given text onto the GUI. */ public void println(String text) { messageLabel.setText(text); if (CONSOLE_OUTPUT) { System.out.println(text); } } /** Outputs the given text onto the GUI. */ public void println() { if (CONSOLE_OUTPUT) { System.out.println(); } } /* The basic game loop, which will be run in a separate thread. */ public void run() { // load audio resources playMusic(); // load data saveLoad(false); // play many games do { if (CONSOLE_OUTPUT) System.out.println(); game.play(); print(PLAY_AGAIN_MESSAGE); } while (nextBoolean());
// save data saveLoad(true); bannerLabel.setVisible(false); // shut down // frame.setVisible(false); // frame.dispose(); // System.exit(0); }
/** Waits for the user to press Yes or No and returns the boolean. */ public boolean nextBoolean() { setWaitingForBoolean(true); try { boolean result = booleanQueue.take(); messageLabel.setText(null); if (CONSOLE_OUTPUT) { System.out.println(result ? "yes" : "no"); } return result; } catch (InterruptedException e) { return false; } finally { setWaitingForBoolean(false); } }
// sets JFrame's position to be in the center of the screen private void center(JFrame frame) { Dimension screen = Toolkit.getDefaultToolkit().getScreenSize(); frame.setLocation((screen.width - frame.getWidth()) / 2, (screen.height - frame.getHeight()) / 2); } // turns on/off various graphical components when game events occur private void doEnabling() { inputField.setVisible(waitingForString); if (waitingForString) { inputField.requestFocus(); inputField.setCaretPosition(inputField.getText().length()); } yesButton.setVisible(waitingForBoolean); noButton.setVisible(waitingForBoolean); bannerLabel.setText(BANNER_MESSAGE); statsArea.setText(String.format(STATUS_MESSAGE, game.totalGames(), game.gamesWon())); } // helper to download from the given URL to the given local file private static void download(String urlString, String filename) throws IOException, MalformedURLException { File file = new File(filename); System.out.println("Downloading"); System.out.println("from: " + urlString); System.out.println(" to: " + file.getAbsolutePath()); System.out.println(); URL url = new URL(urlString); InputStream stream = url.openStream();
// read bytes from URL into a byte[] ByteArrayOutputStream bytes = new ByteArrayOutputStream(); while (true) { int b = stream.read(); if (b
if (CONSOLE_OUTPUT) { System.out.println(result); } return result; } catch (InterruptedException e) { return ""; } finally { setWaitingForString(false); } } // response to a 'no' button click or typing 'n' private void no() { try { booleanQueue.put(false); doEnabling(); } catch (InterruptedException e) {} } // loads and plays/loops the sound/music file with the given file name private AudioClip playAudioClip(String filename, boolean loop) { if (!ensureFile(filename)) { return null; } URL url = ClassLoader.getSystemResource(filename); if (url == null) { // on some students' systems the ClassLoader can't find URLs; // so fall back by trying to construct the URL myself try { url = new File(filename).toURI().toURL(); } catch (MalformedURLException e) {} } if (CONSOLE_OUTPUT) { System.out.println("Playing sound: " + url); }
AudioClip clip = null; try { clip = Applet.newAudioClip(url); if (loop) { clip.loop(); } else { clip.play(); } } catch (NullPointerException e) { if (CONSOLE_OUTPUT) { System.out.println("Error: Unable to load audio clip"); } } return clip; } // plays the background theme music private void playMusic() { if (musicBox.isSelected()) { try { if (musicClip == null) { int rand = 1 + (int) (Math.random() * NUM_MUSICS); if (Math.random()
---------------------------------
question1.txt
----------------------------------
Q:Does it use the Force? A:Jedi Q:Is it metal? A:droid A:hobbit
-------------------------------
question2.txt
-----------------------------
Q:Is it an animal? Q:Can it fly? A:bird Q:Does it have a tail? A:mouse A:spider Q:Does it have wheels? A:bicycle Q:Is it nice? A:TA A:teacher
------------------------------
animals.txt ( just some parts)
------------------------------
Q:Can it fly? Q:Does it have feathers? Q:Does it sometimes live in water? Q:Does it eat fish? Q:Does it have long legs? Q:Is it pink? Q:Does it look weird to humans? Q:Does it sometimes stand on one leg? Q:It's not pink or stands on one leg is it? Q:Is it some kind of jelly fish? Q:Does it have legs? Q:Is it not real? Q:Are you going to keep hitting yes? Q:Does it have stripes? Q:Is it white? Q:Is it a male? Q:Is it yellow? Q:Is it related to the cat family? Q:Can it be all animals? Q:Are you going to click yes for the last question? Q:Are you going to answer "no" for this question? Q:Can it do everything? A:God A:yes A:yes A:nothing A:Bengal tiger A:rabbit A:snow man A:snow woman A:my weird cat A:kangaroo Q:Does it have long legs? A:kangaroo A:I wasn't thinking of anything; I was just hitting all yes! Q:Is it a creature in the T.V series the Smurfs? Q:Is it blue? A:Papa Smurf A:Papa Smurf A:dog A:big pink fish Q:Does it quack? A:duck Q:Is it on Kim Possible? A:naked mole rat A:flamingo Q:It it a relative of the Husky family? Q:Is it a pet? A:Siberian husky Q:Does it eat fish? A:Bear A:wolf Q:An anteater doesn't stand on one leg? Q:Does it love the color pink and plays golf? A:craig davis A:flamingo Q:Is it pink and stands in one leg? A:flamingo A:anteater Q:Does is have long and pink legs? Q:Do people eat it? Q:Does it have a big beak to get fish with? Q:Can it shapeshift? A:shapeshifting alcolyte A:seagull A:hen A:flamingo Q:Does it swallow fish by flicking back its head? Q:Does it spend all day in the water? A:sea lion A:flamingo A:Davis diver bird Q:Does it have a curved bill? A:flamingo
--------------------------------------------
Then I have also files 20Q.gpj, ackbar.png, ackbar1.wav to ackbar4.wav, empire1.mid to empire9.mid, vader1.wav to vader17.wav.
CS145-PROGRAMMING ASSIGNMENT #7 CARD ARRAY LIST OVERVIEW This program focuses on binary trees and recursion. INSTRUCTIONS Turn in file QuestionTree.java and optionally Questionlode.java. (You may or may not have QuestionNode.java class if you choose to include inside Question Tree. java) You il need support files UserInterface.java, QuestionMain.java, VaderMain.java, and input text files from the Homework web page, place them in the same folder as your class. COLLECTABLE CARD In this assignment you will implement a yeso guessing game called "20 Questions." Each round of the game begins by you (the human player) thinking of an object. The computer will try to guess your object by asking you a series of yes or no questions Eventually the computer will have asked enough questions that it thinks it knows what object you are thinking of. It will make a guess about what your object is. If this guess is correct, the computer wins, if not, you win The computer keeps track of a binary tree whose nodes represent questions and answers. (Every node's data is a string representing the text of the question or answer.) A "question" node contains a left 'yes" subtree and a right "no" subtree. An "answer" node is a leaf. The idea is that this tree can be traversed to ask the human player a series of questions. (Though the game is called "20 Questions," our game will not limit the tree to a height of 20. Any height is allowed.) For example, in the tree below, the computer would begin the game by asking the player, "ls it an animal?" If the player says "yes," the computer goes left to the "yes subtree and then asks the user, "Can it fly?" If the user had instead said "no," the CS145-PROGRAMMING ASSIGNMENT #7 CARD ARRAY LIST OVERVIEW This program focuses on binary trees and recursion. INSTRUCTIONS Turn in file QuestionTree.java and optionally Questionlode.java. (You may or may not have QuestionNode.java class if you choose to include inside Question Tree. java) You il need support files UserInterface.java, QuestionMain.java, VaderMain.java, and input text files from the Homework web page, place them in the same folder as your class. COLLECTABLE CARD In this assignment you will implement a yeso guessing game called "20 Questions." Each round of the game begins by you (the human player) thinking of an object. The computer will try to guess your object by asking you a series of yes or no questions Eventually the computer will have asked enough questions that it thinks it knows what object you are thinking of. It will make a guess about what your object is. If this guess is correct, the computer wins, if not, you win The computer keeps track of a binary tree whose nodes represent questions and answers. (Every node's data is a string representing the text of the question or answer.) A "question" node contains a left 'yes" subtree and a right "no" subtree. An "answer" node is a leaf. The idea is that this tree can be traversed to ask the human player a series of questions. (Though the game is called "20 Questions," our game will not limit the tree to a height of 20. Any height is allowed.) For example, in the tree below, the computer would begin the game by asking the player, "ls it an animal?" If the player says "yes," the computer goes left to the "yes subtree and then asks the user, "Can it fly?" If the user had instead said "no," theStep by Step Solution
There are 3 Steps involved in it
Step: 1
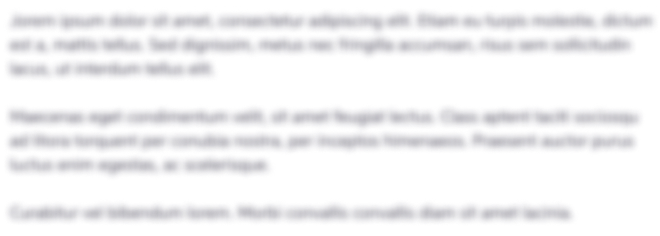
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started