Question
Please help me to solve the problem with java language! An implementation of the Merge Sort algorithm. Modify the algorithm so that it splits the
Please help me to solve the problem with java language!
An implementation of the Merge Sort algorithm. Modify the algorithm so that it splits the list into 3 sublists (instead of two). Each sublist should contain about n/3 items. The algorithm should sort each sublist recursively and merge the three sorted sublists. The traditional merge sort algorithm has an average and worst-case performance of O(n log2 n). What is the performance of the 3-way Merge Sort algorithm?
Merge Sort algorithm
import java.util.Arrays;
public class MergeSort
{
public static void main(String[] args)
{
int[] values = new int[15];
for(int i=0; i values[i] = (int)(90*Math.random())+10; System.out.println("Unsorted: " + Arrays.toString(values)); sort(values); System.out.println(" Sorted: " + Arrays.toString(values)); } public static void sort(int[] data) { sort(data, 0, data.length-1); } private static void sort(int[] data, int min, int max) { if(max>min) { int pivot = (min+max)/2; sort(data, min, pivot); sort(data, pivot+1, max); int left = min; //the beginning of the left sublist int right = pivot+1; //the beginning of the right sublist int[] merged = new int[max-min+1]; for(int i=0; i { if(left<=pivot && (right>max || data[left] merged[i] = data[left++]; else merged[i] = data[right++]; } for(int i=0, j=min; i data[j] = merged[i]; // System.out.println(" Sorting: " + Arrays.toString(data) + " " + min + "-" + max); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
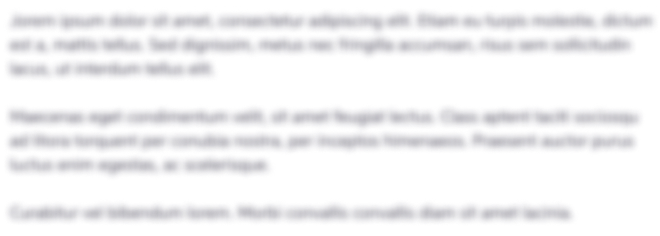
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started