Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please help me with the following Java programming project task, I hope to get a correct answer as a reference, thank you very much. The
Please help me with the following Java programming project task, I hope to get a correct answer as a reference, thank you very much.
The system shows an ordering menu for people to order dim sum dishes. When they have finished, they can ask for the bill and see the order summary.
To complete the system, you will need to finish two tasks:
Complete the DimSum class for storing the information of the dim sum dishesfinished
Create the ordering menuunfinished
Show the bill with the order summaryunfinished
You can type and then press Enter to stop the program.
Let's first work on the first stage of the program, showing the ordering menu. To do that you need to do the following:
Printing the menu
Ordering the dish based on the input
Printing the Menu
At the moment the menu only shows the choice Bill and payment'. In this part you need to complete the menu and show the first four choices, ie the dim sum dishes.
If you look at the DimSumOrdering class you will find that there is an attribute declared at the top like this:
This stores the dim sum dishes in the system
DimSum dishes;
The attribute is an array of dim sum dishes. You will store the dim sum dishes of the system in this array. You can do that in the constructor of the class. There are four dim sum dishes that you need to assign to the array. Before assigning the dishes to the array, you need to create the array using this line of code:
dishes new DimSum;
Then, for example, you can put 'Siu Mai' as the first dish in the array like this:
dishes new DimSumSiu Mai", f;
The above code puts a new instance of DimSum as the first item of the array. Since we have four dim sum dishes you will put the other three dishes with appropriate names in the rest of the dishes array. For the exercise, let's set the price of the dim sum dishes to be: Barbecued Pork Bun $ Shrimp Dumpling $ Siu Mai $ and Spring Roll $
Now, you have the array of the dim sum dishes you can print the first four choices of the menu easily using a loop. Inside the do while loop in the start method you can see the location where the menu is printed. Before printing the fifth choice, ie Bill and payment' you need to print the dim sum dishes as the first four choices in the menu. To do that you can use a for loop like this:
for i ; i dishes.length; i
print the text using dishesigetName and dishesigetPrice
After showing the correct menu, you can enter or to start to order the dim sum dishes. In the program, the input is read using this line of code:
Read the input
choice scanner.next;
Whatever entered by you has been stored in the choice variable. Depending on the value of the variable you can choose to increase the quantity for one of the dishes. There are many ways to do that. For example, you can use if statements to do that.
In this exercise, let's use the switch statement on the choice variable.
Here is what you need to set up after reading the input:
switch choice
case :
order the first dish
break;
case :
order the second dish
break;
case :
order the third dish
break;
case :
order the fourth dish
break;
The above switch statement uses the value of choice to order the corresponding dish. To do that, simply use the order method of that dish with a quantity of However, be careful that the choice input of corresponds to the first dish with an index of ie dishes
After selecting in the menu you need to show the bill and the order summary.
You will do two things in this part. The first thing is to show the order summary.
Showing the Order Summary
You first display the summary of all orders. This can be done using a for loop to go through the dishes and print one at a time, like this:
for i ; i dishes.length; i
print the information of dishesi
You need to show the ordered quantity, the subtotal of the dim sum dish and the name of the dish.
Finally, you will show the total amount of the bill by adding up all the orders of the dim sum dishes. Again, you can make use of a for loop to do that, like this:
total ;
for i ; i dishes.length; i
add to the total using dishesigetQuantity and dishesigetPrice
The following is the unfinished part of the code, please complete this part of the code according to the above requirements on the basis of it
public class DimSumOrdering
This stores the dim sum dishes in the system
DimSum dishes;
The constructor of DimSumOrdering
public DimSumOrdering
Task Initialize the dim sum dishes in the system
Start the ordering system
public void start
Step by Step Solution
There are 3 Steps involved in it
Step: 1
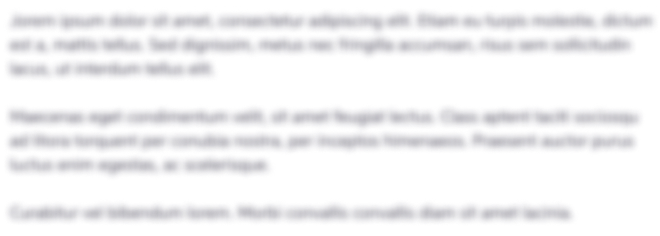
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started