Question
Please help me with these programs in order. There is a zip file named assignment09_part2. zip I cannot upload a zip file but here are
Please help me with these programs in order. There is a zip file named assignment09_part2. zip I cannot upload a zip file but here are the contents. class_0.txt class_1.txt class_2.txt class_3.txt class_4.txt class_5.txt class_6.txt class_7.txt class_8.txt class_9.txt class_10.txt class_11.txt class_12.txt class_13.txt class_14.txt class_15.txt class_16.txt class_17.txt class_18.txt class_19.txt class_20.txt class_21.txt class_22.txt class_23.txt class_24.txt class_25.txt class_26.txt class_27.txt class_28.txt class_29.txt class_30.txt class_31.txt class_32.txt class_33.txt class_34.txt class_35.txt class_36.txt class_37.txt class_38.txt class_39.txt class_40.txt class_41.txt class_42.txt class_43.txt class_44.txt class_45.txt class_46.txt class_47.txt class_48.txt class_49.txt quizzes.txt
I understand this is limited information but I'll provide a request/output of a program to provide an idea of how it is supposed to work.
There are 50 "class" files and one "quizzes.txt" file. The "class" files contain quiz answers provided by students in a particular class. The "quizzes.txt" file contains a listing of all of the quizzes that were delivered, along with their solutions.
quizzes.txt looks like this
Quiz1,ABCDABCDDD
Quiz2,ABABAABAAC
Quiz3,BBBABCCDEABAABC
Quiz4,CDDABCDABCDDABCDADCA
Quiz5,ABDDABDEADDABDA
class.6txt looks like this
Quiz1
N000000111,ABC_ABCD_D
N000000379,ABADABCDDD
N000000153,AB_DABB_DC
N000000344,ABCDABCDDD
N000000091,ABCDADCD_D
N000000316,_BC_ABCDD_
N000000297,ABCD_BCDDD
N000000284,A_CD__CDD_
N000000035,B_CDD__DDD
N000000440,AB__A_CDDD
N000000001,AB_DA_CBDC
N000000158,A_C_ABC__D
etc
etc
Part 2a
In this part you will program menu system that allows the user to explore "quizzes.txt" file. This file is organized as follows:
Quiz1,ABCDABCDDD Quiz2,ABABAABAAC Quiz3,BBBABCCDEABAABC Quiz4,CDDABCDABCDDABCDADCA Quiz5,ABDDABDEADDABDA
You'll notice that each line of the file begins with the title of a Quiz, followed by a comma, followed by a series of letters. These letters indicate the correct answers to that multiple choice quiz.
Your task is to program that does the following:
- A menu system that allows the user to view information about the quizzes described in "quizzes.txt" or quit the program
- If the user elects to quit the program should end
- If the user wants information about each quiz the system should tell the user how many quizzes there are in total, along with how many questions each quiz contains. Note that you cannot "hard-code" your program! You must open and read the "quizzes.txt" file to obtain this information. When you submit your homework we will be testing your program using a completely different "quizzes.txt" file that is organized in a similar manner.
- All inputs should be case-insensitive
Here is a sample running of this part of the program:
NYU Quizzing System - Main Menu (q)uiz info or (e)xit: foobar Unknown command, please try again NYU Quizzing System - Main Menu (q)uiz info or (e)xit: Q There are 5 quizzes available "Quiz1" has 10 questions "Quiz2" has 10 questions "Quiz3" has 15 questions "Quiz4" has 20 questions "Quiz5" has 15 questions NYU Quizzing System - Main Menu (q)uiz info or (e)xit: e Goodbye!
Part 2b
Next, update your program to allow the user to begin to score one of the 50 "class" files. This will involve you adding in a new option to your main menu - once the user indicates that they wish to score a file, ask them for a filename. If the filename does not exist you should let them know and return them to the main menu. Otherwise, you should open the file in question and identify which quiz this file uses, as well as how many students are represented in the file.
Note that all class files are organized as follows:
QuizID NYU_N_NUMBER,STUDENT_ANSWERS NYU_N_NUMBER,STUDENT_ANSWERS NYU_N_NUMBER,STUDENT_ANSWERS NYU_N_NUMBER,STUDENT_ANSWERS NYU_N_NUMBER,STUDENT_ANSWERS
For example, here is the full text for the "class_41.txt" file:
Quiz1 N000000024,A_CDACCDDD N000000149,DBCD_B_D_D N000000394,A_CDA__DDD N000000168,ABC_A__D_D N000000016,ABC_ABCDDD N000000058,_BC_DBCD_A N000000232,A_C_AB___B N000000226,ABCDABC_DD N000000070,D_C_ABCDDD N000000248,_BCDA_CDD_ N000000470,A_C_AB__DD N000000085,ABCB_BCD__ N000000371,_BCDABC_D_ N000000204,ABCDAB_C_D N000000051,_B_D_AADCD N000000114,_BCDA__D_D
The first line always contains the ID of the quiz being used, and the remaining lines each contain a student record (one student per line). Here's a sample running of this part of the program:
NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: test.txt File not found. NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_41.txt This file contains 16 student entries for the test "Quiz1" NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_1.txt This file contains 40 student entries for the test "Quiz2" NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_2.txt This file contains 153 student entries for the test "Quiz4" NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: e Goodbye!
Some hints:
- tryandexceptmay be useful here in identifying files that don't exist.
- How can you isolate each line of a file from the other lines? Remember that when you read the file you are given the entire file as a string.
- How can you isolate the first line? How about isolating all lines after the first line? Slicing might be helpful here.
Part 2c
Next you are going to score the file using the answer key provided in the "quizzes.txt" file. Here's a sample running of this part of the program:
NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_41.txt This file contains 16 student entries for the test "Quiz1" The answer key for this test is: ABCDABCDDD N000000024 earned 8 out of 10 (80.00%) N000000149 earned 6 out of 10 (60.00%) N000000394 earned 7 out of 10 (70.00%) N000000168 earned 6 out of 10 (60.00%) N000000016 earned 9 out of 10 (90.00%) N000000058 earned 5 out of 10 (50.00%) N000000232 earned 4 out of 10 (40.00%) N000000226 earned 9 out of 10 (90.00%) N000000070 earned 7 out of 10 (70.00%) N000000248 earned 7 out of 10 (70.00%) N000000470 earned 6 out of 10 (60.00%) N000000085 earned 6 out of 10 (60.00%) N000000371 earned 7 out of 10 (70.00%) N000000204 earned 7 out of 10 (70.00%) N000000051 earned 4 out of 10 (40.00%) N000000114 earned 6 out of 10 (60.00%) NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_1.txt This file contains 40 student entries for the test "Quiz2" The answer key for this test is: ABABAABAAC N000000429 earned 10 out of 10 (100.00%) N000000041 earned 6 out of 10 (60.00%) N000000019 earned 9 out of 10 (90.00%) N000000062 earned 8 out of 10 (80.00%) N000000186 earned 7 out of 10 (70.00%) N000000480 earned 5 out of 10 (50.00%) N000000222 earned 9 out of 10 (90.00%) N000000126 earned 7 out of 10 (70.00%) N000000236 earned 5 out of 10 (50.00%) N000000425 earned 7 out of 10 (70.00%) N000000389 earned 5 out of 10 (50.00%) N000000387 earned 8 out of 10 (80.00%) N000000123 earned 4 out of 10 (40.00%) N000000348 earned 8 out of 10 (80.00%) N000000340 earned 6 out of 10 (60.00%) N000000332 earned 9 out of 10 (90.00%) N000000091 earned 8 out of 10 (80.00%) N000000007 earned 6 out of 10 (60.00%) N000000018 earned 8 out of 10 (80.00%) N000000248 earned 5 out of 10 (50.00%) N000000307 earned 6 out of 10 (60.00%) N000000063 earned 8 out of 10 (80.00%) N000000401 earned 6 out of 10 (60.00%) N000000366 earned 9 out of 10 (90.00%) N000000382 earned 8 out of 10 (80.00%) N000000315 earned 6 out of 10 (60.00%) N000000006 earned 7 out of 10 (70.00%) N000000319 earned 5 out of 10 (50.00%) N000000162 earned 8 out of 10 (80.00%) N000000074 earned 10 out of 10 (100.00%) N000000353 earned 7 out of 10 (70.00%) N000000042 earned 8 out of 10 (80.00%) N000000196 earned 8 out of 10 (80.00%) N000000178 earned 7 out of 10 (70.00%) N000000108 earned 8 out of 10 (80.00%) N000000473 earned 9 out of 10 (90.00%) N000000459 earned 6 out of 10 (60.00%) N000000349 earned 7 out of 10 (70.00%) N000000181 earned 7 out of 10 (70.00%) N000000061 earned 2 out of 10 (20.00%) NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: e Goodbye!
Some hints
- You can see the expected output for all 50 files here
- Begin by matching the quiz ID on the 1st line of the "class" file with the quiz in the "quizzes.txt" file. It might be easiest to at the beginning of the program since you know you'll be using this info throughout the program. Or you can open the "quizzes.txt" file every time you score a test, but this is not very efficient (you won't lose points for doing it, though)
- Once you have isolated the correct answer key print it out for the user to see.
- Next, you will need to examine all students in the "class" file and isolate the NYU N# from the student's actual answers.
- If a student answer matches the answer in the answer key for a given question then they should be given one point. Student answers that differ, or those that are "skipped" (i.e. the_character) should not be worth any points.
- For example, consider the first student in "class_41.txt":
N000000024,A_CDACCDDD
- Their N# isN000000024and their answer isA_CDACCDDD. This file uses "Quiz1" as its answer key, which is "ABCDABCDDD". Comparing these two files would look like the following:
A_CDACCDDD # student answer ABCDABCDDD # answer key 1st answer: A == A, 1 point 2nd answer: _ != B, 0 points 3rd answer: C == C, 1 point 4th answer: D == D, 1 point 5th answer: A == A, 1 point 6th answer: C != B, 0 points 7th answer: C == C, 1 point 8th answer: D == D, 1 point 9th answer: D == D, 1 point 10th answer: D == D, 1 point Final score: 8 out of 10 (80%)
Part 2d
Next, compute some summary statistics for the class you just scored. These statistics should include the average score, the highest score, the lowest score and the range (the difference between the highest and the lowest). Here's a sample running of this part of the program:
NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_41.txt This file contains 16 student entries for the test "Quiz1" The answer key for this test is: ABCDABCDDD N000000024 earned 8 out of 10 (80.00%) N000000149 earned 6 out of 10 (60.00%) N000000394 earned 7 out of 10 (70.00%) N000000168 earned 6 out of 10 (60.00%) N000000016 earned 9 out of 10 (90.00%) N000000058 earned 5 out of 10 (50.00%) N000000232 earned 4 out of 10 (40.00%) N000000226 earned 9 out of 10 (90.00%) N000000070 earned 7 out of 10 (70.00%) N000000248 earned 7 out of 10 (70.00%) N000000470 earned 6 out of 10 (60.00%) N000000085 earned 6 out of 10 (60.00%) N000000371 earned 7 out of 10 (70.00%) N000000204 earned 7 out of 10 (70.00%) N000000051 earned 4 out of 10 (40.00%) N000000114 earned 6 out of 10 (60.00%) *** Class Report *** Average score: 6.50 Highest score: 9 Lowest score: 4 Range of scores: 5 NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_1.txt This file contains 40 student entries for the test "Quiz2" The answer key for this test is: ABABAABAAC N000000429 earned 10 out of 10 (100.00%) N000000041 earned 6 out of 10 (60.00%) N000000019 earned 9 out of 10 (90.00%) N000000062 earned 8 out of 10 (80.00%) N000000186 earned 7 out of 10 (70.00%) N000000480 earned 5 out of 10 (50.00%) N000000222 earned 9 out of 10 (90.00%) N000000126 earned 7 out of 10 (70.00%) N000000236 earned 5 out of 10 (50.00%) N000000425 earned 7 out of 10 (70.00%) N000000389 earned 5 out of 10 (50.00%) N000000387 earned 8 out of 10 (80.00%) N000000123 earned 4 out of 10 (40.00%) N000000348 earned 8 out of 10 (80.00%) N000000340 earned 6 out of 10 (60.00%) N000000332 earned 9 out of 10 (90.00%) N000000091 earned 8 out of 10 (80.00%) N000000007 earned 6 out of 10 (60.00%) N000000018 earned 8 out of 10 (80.00%) N000000248 earned 5 out of 10 (50.00%) N000000307 earned 6 out of 10 (60.00%) N000000063 earned 8 out of 10 (80.00%) N000000401 earned 6 out of 10 (60.00%) N000000366 earned 9 out of 10 (90.00%) N000000382 earned 8 out of 10 (80.00%) N000000315 earned 6 out of 10 (60.00%) N000000006 earned 7 out of 10 (70.00%) N000000319 earned 5 out of 10 (50.00%) N000000162 earned 8 out of 10 (80.00%) N000000074 earned 10 out of 10 (100.00%) N000000353 earned 7 out of 10 (70.00%) N000000042 earned 8 out of 10 (80.00%) N000000196 earned 8 out of 10 (80.00%) N000000178 earned 7 out of 10 (70.00%) N000000108 earned 8 out of 10 (80.00%) N000000473 earned 9 out of 10 (90.00%) N000000459 earned 6 out of 10 (60.00%) N000000349 earned 7 out of 10 (70.00%) N000000181 earned 7 out of 10 (70.00%) N000000061 earned 2 out of 10 (20.00%) *** Class Report *** Average score: 7.05 Highest score: 10 Lowest score: 2 Range of scores: 8 NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: e Goodbye!
Hint: use a list to store your scores as you compute them, and then process your list after your loop has completed.
Part 2e
Compute the mode (the score that occurs the most often). Hint: use the 'frequency' algorithm that we talked about when analyzing the "World Series Winners" data set in class. The mode may occur more than once and your program should be able to report this. Here's a sample running of this part of the program:
NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_41.txt This file contains 16 student entries for the test "Quiz1" The answer key for this test is: ABCDABCDDD N000000024 earned 8 out of 10 (80.00%) N000000149 earned 6 out of 10 (60.00%) N000000394 earned 7 out of 10 (70.00%) N000000168 earned 6 out of 10 (60.00%) N000000016 earned 9 out of 10 (90.00%) N000000058 earned 5 out of 10 (50.00%) N000000232 earned 4 out of 10 (40.00%) N000000226 earned 9 out of 10 (90.00%) N000000070 earned 7 out of 10 (70.00%) N000000248 earned 7 out of 10 (70.00%) N000000470 earned 6 out of 10 (60.00%) N000000085 earned 6 out of 10 (60.00%) N000000371 earned 7 out of 10 (70.00%) N000000204 earned 7 out of 10 (70.00%) N000000051 earned 4 out of 10 (40.00%) N000000114 earned 6 out of 10 (60.00%) *** Class Report *** Average score: 6.50 Highest score: 9 Lowest score: 4 Range of scores: 5 Mode(s): 6 7 NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_1.txt This file contains 40 student entries for the test "Quiz2" The answer key for this test is: ABABAABAAC N000000429 earned 10 out of 10 (100.00%) N000000041 earned 6 out of 10 (60.00%) N000000019 earned 9 out of 10 (90.00%) N000000062 earned 8 out of 10 (80.00%) N000000186 earned 7 out of 10 (70.00%) N000000480 earned 5 out of 10 (50.00%) N000000222 earned 9 out of 10 (90.00%) N000000126 earned 7 out of 10 (70.00%) N000000236 earned 5 out of 10 (50.00%) N000000425 earned 7 out of 10 (70.00%) N000000389 earned 5 out of 10 (50.00%) N000000387 earned 8 out of 10 (80.00%) N000000123 earned 4 out of 10 (40.00%) N000000348 earned 8 out of 10 (80.00%) N000000340 earned 6 out of 10 (60.00%) N000000332 earned 9 out of 10 (90.00%) N000000091 earned 8 out of 10 (80.00%) N000000007 earned 6 out of 10 (60.00%) N000000018 earned 8 out of 10 (80.00%) N000000248 earned 5 out of 10 (50.00%) N000000307 earned 6 out of 10 (60.00%) N000000063 earned 8 out of 10 (80.00%) N000000401 earned 6 out of 10 (60.00%) N000000366 earned 9 out of 10 (90.00%) N000000382 earned 8 out of 10 (80.00%) N000000315 earned 6 out of 10 (60.00%) N000000006 earned 7 out of 10 (70.00%) N000000319 earned 5 out of 10 (50.00%) N000000162 earned 8 out of 10 (80.00%) N000000074 earned 10 out of 10 (100.00%) N000000353 earned 7 out of 10 (70.00%) N000000042 earned 8 out of 10 (80.00%) N000000196 earned 8 out of 10 (80.00%) N000000178 earned 7 out of 10 (70.00%) N000000108 earned 8 out of 10 (80.00%) N000000473 earned 9 out of 10 (90.00%) N000000459 earned 6 out of 10 (60.00%) N000000349 earned 7 out of 10 (70.00%) N000000181 earned 7 out of 10 (70.00%) N000000061 earned 2 out of 10 (20.00%) *** Class Report *** Average score: 7.05 Highest score: 10 Lowest score: 2 Range of scores: 8 Mode(s): 8 NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: e Goodbye!
Some hints:
- You can see the expected output for all 50 files here
- Compute the mode using the frequency algorithm that we discussed in class. Don't use themathmodule or any other pre-written functions .
- To find multiple modes you may want to remove a mode from your lists once you've printed it out.
Part 2f
Finally, program a "histogram" feature that will print out a horizontal bar chart graphing the scores for a given class. Here's a sample running of this part of the program:
NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_41.txt This file contains 16 student entries for the test "Quiz1" The answer key for this test is: ABCDABCDDD N000000024 earned 8 out of 10 (80.00%) N000000149 earned 6 out of 10 (60.00%) N000000394 earned 7 out of 10 (70.00%) N000000168 earned 6 out of 10 (60.00%) N000000016 earned 9 out of 10 (90.00%) N000000058 earned 5 out of 10 (50.00%) N000000232 earned 4 out of 10 (40.00%) N000000226 earned 9 out of 10 (90.00%) N000000070 earned 7 out of 10 (70.00%) N000000248 earned 7 out of 10 (70.00%) N000000470 earned 6 out of 10 (60.00%) N000000085 earned 6 out of 10 (60.00%) N000000371 earned 7 out of 10 (70.00%) N000000204 earned 7 out of 10 (70.00%) N000000051 earned 4 out of 10 (40.00%) N000000114 earned 6 out of 10 (60.00%) *** Class Report *** Average score: 6.50 Highest score: 9 Lowest score: 4 Range of scores: 5 Mode(s): 6 7 0 1 2 3 4 ** 5 * 6 ***** 7 ***** 8 * 9 ** 10 NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: s Enter a filename to score: class_1.txt This file contains 40 student entries for the test "Quiz2" The answer key for this test is: ABABAABAAC N000000429 earned 10 out of 10 (100.00%) N000000041 earned 6 out of 10 (60.00%) N000000019 earned 9 out of 10 (90.00%) N000000062 earned 8 out of 10 (80.00%) N000000186 earned 7 out of 10 (70.00%) N000000480 earned 5 out of 10 (50.00%) N000000222 earned 9 out of 10 (90.00%) N000000126 earned 7 out of 10 (70.00%) N000000236 earned 5 out of 10 (50.00%) N000000425 earned 7 out of 10 (70.00%) N000000389 earned 5 out of 10 (50.00%) N000000387 earned 8 out of 10 (80.00%) N000000123 earned 4 out of 10 (40.00%) N000000348 earned 8 out of 10 (80.00%) N000000340 earned 6 out of 10 (60.00%) N000000332 earned 9 out of 10 (90.00%) N000000091 earned 8 out of 10 (80.00%) N000000007 earned 6 out of 10 (60.00%) N000000018 earned 8 out of 10 (80.00%) N000000248 earned 5 out of 10 (50.00%) N000000307 earned 6 out of 10 (60.00%) N000000063 earned 8 out of 10 (80.00%) N000000401 earned 6 out of 10 (60.00%) N000000366 earned 9 out of 10 (90.00%) N000000382 earned 8 out of 10 (80.00%) N000000315 earned 6 out of 10 (60.00%) N000000006 earned 7 out of 10 (70.00%) N000000319 earned 5 out of 10 (50.00%) N000000162 earned 8 out of 10 (80.00%) N000000074 earned 10 out of 10 (100.00%) N000000353 earned 7 out of 10 (70.00%) N000000042 earned 8 out of 10 (80.00%) N000000196 earned 8 out of 10 (80.00%) N000000178 earned 7 out of 10 (70.00%) N000000108 earned 8 out of 10 (80.00%) N000000473 earned 9 out of 10 (90.00%) N000000459 earned 6 out of 10 (60.00%) N000000349 earned 7 out of 10 (70.00%) N000000181 earned 7 out of 10 (70.00%) N000000061 earned 2 out of 10 (20.00%) *** Class Report *** Average score: 7.05 Highest score: 10 Lowest score: 2 Range of scores: 8 Mode(s): 8 0 1 2 * 3 4 * 5 ***** 6 ******* 7 ******** 8 *********** 9 ***** 10 ** NYU Quizzing System - Main Menu (q)uiz info, (s)core a file or (e)xit: e Goodbye!
Some hints:
- You can see the expected output for all 50 files here
- You will be using your "frequency" data that you computed during for the "mode" portion of this assignment to generate the bar chart. program a copy of your "unique" and "count" lists before you delete the multiple modes from these lists.
- You can use string repetition to program your charts
- Format your numbers along the left to 2 spaces so they all line up correctly
- You may need a "for" loop to iterate over all possible scores for your test. Note that some scores won't have a bar next to them and that is OK - just don't print anything (but make sure the number itself prints along the left side of your chart)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
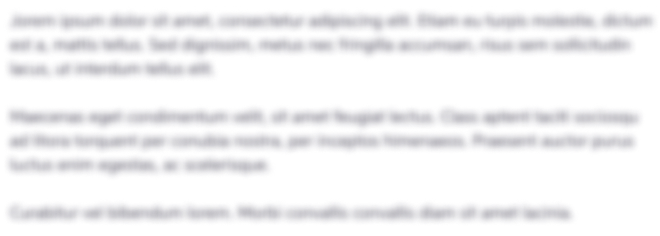
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started