Question
Please help me with this assignment. For this assignment you will implement divide and conquer algorithms: the maximum subarray. For merge sort, you will just
Please help me with this assignment. For this assignment you will implement divide and conquer algorithms: the maximum subarray. For merge sort, you will just implement the algorithm on an array of ints. I have provided the pseudo code for algorithms below. You will need to handle cases of all sizes, not just powers of 2.
Maximum Subarray using Divide and Conquer
Create a class called MaxSubFinder in the divideandconquer package. This class will implement the maximum subarray problem on both an array and a linked list using the pseudocode described above. Implement the following methods with the exact signatures below. You will need to create private helper methods to do most of the work. You can assume that the array and list are not empty.
public static Triple getMaxSubarray(int[] arr)
This method returns a triple that represents the maximum subarray. The first element of the triple is the index in arr where the maximum subarray starts, the middle element of the triple is where the index in the arr where maximum subarray ends, and the last element of the triple is the maximum subarray sum.
public static Triple getMaxSubList(LinkedList list)
This method returns a triple that represents the maximum sub list. The first element of the triple is the list node where the maximum sublist starts, the middle element of the triple is the list node where the maximum sublist ends, and the last element of the triple is the maximum sublist sum.
Triple.java
This file contains a generic Triple class. You will use this to return the starting point, ending point, and sum for the MaxSubFinder class. We use classes like this when we want to have a container that holds different types. Do not modify this class.
package divideandconquer;
public class Triple
public First getFirst() { return first; }
public void setFirst(First first) { this.first = first; }
public Middle getMiddle() { return middle; }
public void setMiddle(Middle middle) { this.middle = middle; }
public Last getLast() { return last; }
public void setLast(Last last) { this.last = last; }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
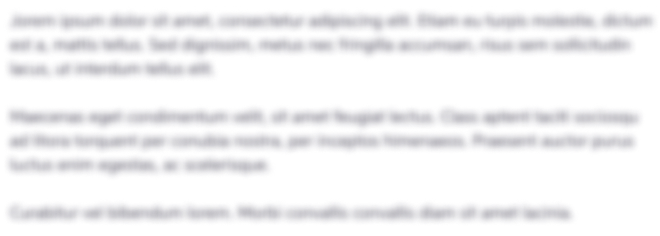
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started