Question
please help me write this program. It's all in C, NOT C++ Program Description Your first program is to implement the game of Nim. There
please help me write this program. It's all in C, NOT C++
Program Description
Your first program is to implement the game of Nim. There are many variations to Nim, we will be using a simple version that has an easy to understand winning strategy. In this game, there is a pile of objects (well call them stones.) Each player, in turn, removes at least one to at most half of the remaining stones. The player who takes the last stone loses.
This program will allow a person to play against the computer. First present a menu allowing the person to choose to Play or Quit. If any other option is entered, display an error message, then redisplay the menu. Upon choosing to play, the program will generate a random number of stones between 10 and 100, inclusive, as the beginning pile of stones. The program will also randomly determine if the person or computer will play first, and also randomly determine if the computer will play in smart or dumb mode.
Computer Play Modes
In dumb mode, the computer will make plays by simply choosing a random number of stones to remove in the range of 1 to n/2, inclusive, where n is the number of stones currently on the pile. The range 1 to n/2, inclusive, is the limit of legal moves that one can make at any time.
Smart mode is a bit more complex. If possible, the computer will remove enough stones so the remaining pile is of size 2m-1, that is, a power of two minus 1 (3, 7, 15, 31, 63). This will always be possible, except in the case where the number of stones is already a value in that set. In that event, the computer will make any random, legal move, as describe in the dumb mode.
You will find that if the computer has the first turn and is playing in smart mode, it cannot be beat, unless the beginning number of stones is one of the 2m-1 values. Similarly, a human player going first who plays the winning strategy can win against the computer.
Random number generation
You will need to have the following additional libraries included in your program:
#include
#include
In main( ), you will have the following statement (it should only execute once!)
srand((int)time(NULL)); //initialize random number generator
Then, when you want a random value, use the statement such as:
num = rand() % 51; //get random value, range 0-50
Note that the rand() function returns values in the range 0 to 32767, inclusive. You must modify that value to suit your needs.
In your code, at the point where you generate random values for number of stones, person or computer first, smart or dumb, make the selections in that order. Use the value 1 for true, 0 for false.
For logical decisions, let 1 indicate the human plays first, and let 1 indicate the computer plays smartly.
Study the sample run of program below. Comments in square brackets are not part of the program output, but are explanations of the state of the sample game.
*** Play the Game of Nim ***
P - Play a game
Q - Quit
Your choice( P, Q): t
"t" is not a valid option. Try again
*** Play the Game of Nim ***
P - Play a game
Q - Quit
Your choice( P, Q): p
There are 70 in the pile. Begin! [ computer playing in dumb mode!]
70 stones left in pile.
The computer takes 15 stones.
55 stones left in pile. Number to remove? 24
31 stones left in pile.
The computer takes 8 stones.
23 stones left in pile. Number to remove? 8
15 stones left in pile.
The computer takes 6 stones.
9 stones left in pile. Number to remove? 2
7 stones left in pile.
The computer takes 3 stones.
4 stones left in pile. Number to remove? 1
3 stones left in pile.
The computer takes 1 stones.
2 stones left in pile. Number to remove? 1
You won. Better buy a smarter computer.
*** Play the Game of Nim ***
P - Play a game
Q - Quit
Your choice( P, Q): p
There are 57 in the pile. Begin!
57 stones left in pile. Number to remove? 7
50 stones left in pile.
The computer takes 18 stones. [ computer playing in dumb mode again!]
32 stones left in pile. Number to remove? 1
31 stones left in pile.
The computer takes 15 stones.
16 stones left in pile. Number to remove? 1
15 stones left in pile.
The computer takes 3 stones.
12 stones left in pile. Number to remove? 5
7 stones left in pile.
The computer takes 2 stones.
5 stones left in pile. Number to remove? 1 [ I gave it a chance to win, it goofed!]
4 stones left in pile.
The computer takes 2 stones.
2 stones left in pile. Number to remove? 1
You won. Better buy a smarter computer.
*** Play the Game of Nim ***
P - Play a game
Q - Quit
Your choice( P, Q): p
There are 79 in the pile. Begin! [ computer playing in smart mode!]
79 stones left in pile.
The computer takes 16 stones.
63 stones left in pile. Number to remove? 5
58 stones left in pile.
The computer takes 27 stones.
31 stones left in pile. Number to remove? 15
16 stones left in pile.
The computer takes 1 stones.
15 stones left in pile. Number to remove? 2
13 stones left in pile.
The computer takes 6 stones.
7 stones left in pile. Number to remove? 3
4 stones left in pile.
The computer takes 1 stones.
3 stones left in pile. Number to remove? 1
2 stones left in pile.
The computer takes 1 stones.
You lost. Better luck next time.
*** Play the Game of Nim ***
P - Play a game
Q - Quit
Your choice( P, Q): q
Bye!
int main( t nun stones: int stones pick int stones_left; char play int turn, srand((int tine NULL)); //initalize rand numb generator printf( Play and Quit (P, Q):) scanf(, Splay) whose turn rand() % 2 nun stones rand() % 98 10; if play P & whose_turn-1) printf("There are %d stones in the pile. Begin! rintf sd stones left in scanf("%d. &stones-pick); stones Left-num_stones stones pick ", num-stones); pile- Number to renove? ", num stones); while (stonesleft 1) - printf("%d stores left in pile. ", stones left); stones-pick rand() % (stones left/2) + printf("The computer takes %d stones. ", stones-pick); stones _left num stones stones_pick; printf("%d stones left in the pile. Number to remove? scanf("%d", &stones pick: stones leftnu stones-stones _pick; printfT sd stones Left in pile.n, stones left); stones left ); hile(stones pick 1(stones pickstones left /2) l stones_leftl) printf The marbles you're trying to pick is out of range.n) printf( Enter the number of stones to be picked in the scanf ("%d", &stones-pick); (1 .%dNn", (stones left /2))Step by Step Solution
There are 3 Steps involved in it
Step: 1
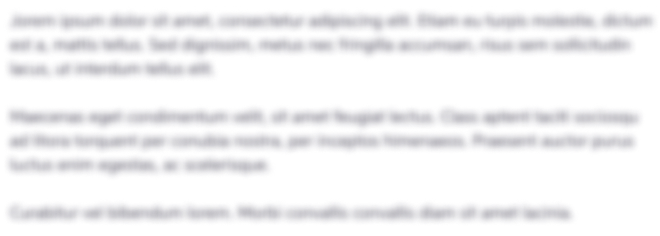
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started