Question
Please help. The code for the Ball and Button class is posted below the question Writing methods for the Ball class (Part 1) 1. Write
Please help. The code for the Ball and Button class is posted below the question
Writing methods for the Ball class (Part 1)
1. Write the body of the act() method of the Ball class.
The act method simply calls the following methods in the order specified below.
1. handleKeyPress
2. lookForButton
2. Write the body of the handleKeyPress() method of the Ball class.
The handleKeyPress method simply calls the following methods in the order specified below.
1. checkLeftArrow
2. checkRightArrow
3. checkUpArrow
4. checkDownArrow
3. Write the body of the checkLeftArrow() method of the Ball class.
This method checks to see if the left arrow key is being pressed. If it is, then the ball sets its rotation angle to zero degrees and moves backward 5 units. If it is not being pressed, this method does nothing. List of methods to use:
Greenfoot.isKeyDown
setRotation
move
4. Write the body of the checkRightArrow() method of the Ball class.
This method checks to see if the right arrow key is being pressed. If it is, then the ball sets its rotation angle to zero degrees and moves forward 5 units. If it is not being pressed, this method does nothing. List of methods to use:
Greenfoot.isKeyDown
setRotation
move
5. Write the body of the checkUpArrow() method of the Ball class.
This method checks to see if the up arrow key is being pressed. If it is, then the ball sets its rotation angle to 270 degrees and moves forward 5 units. If it is not being pressed, this method does nothing. List of methods to use:
Greenfoot.isKeyDown
setRotation
move
6. Write the body of the checkDownArrow() method of the Ball class.
This method checks to see if the down arrow key is being pressed. If it is, then the ball sets its rotation angle to 90 degrees and moves forward 5 units. If it is not being pressed, this method does nothing. List of methods to use:
Greenfoot.isKeyDown
setRotation
move
7. Write the body of the lookForButton() method of the Ball class.
This method checks to see if the ball is touching an object of the Button class. If it is, then the ball removes from the World a Button that it touches. If the ball isnt touching an object of the Button class, then this method does nothing. List of methods to use:
isTouching
removeTouching
Writing methods for the Button class (Part 2)
1. Write the body of the act() method of the Button class.
The act method changes the location of the Button object approximately 2% of the time; that is, on approximately 2 out of every 100 act cycles of the scenario, the Button object calls its changeLocationmethod. List of methods to use:
Greenfoot.getRandomNumber
changeLocation
2. Write the body of the changeLocation() method of the Button class.
The changeLocation method sets the location of the Button object to a new (x, y) coordinate selected at random. The x coordinate must be selected from the range 100 to 500 inclusive, and the ycoordinate must be selected from the range 100 to 300 inclusive. List of methods to use:
Greenfoot.getRandomNumber
setLocation
public class Ball extends Actor { /** * Responds to motion control from the arrow keys and removes * any buttons from the world when it intersects them. */ public void act() {
}
/** * Checks to see if the ball is "touching" an object of the Button class. * If it is, then the ball removes from the World a Button that it touches. * If the ball isn't touching an object of the Button class, then this * method does nothing. */ public void lookForButton() {
}
/** * Handles input from the arrow keys. */ public void handleKeyPress() {
}
/** * Checks to see if the left arrow key is being pressed. * If it is, then the ball sets its rotation angle to zero degrees * and moves backward 5 units. If it is not being pressed, * this method does nothing. */ public void checkLeftArrow() {
}
/** * Checks to see if the right arrow key is being pressed. * If it is, then the ball sets its rotation angle to zero degrees * and moves forward 5 units. If it is not being pressed, * this method does nothing. */ public void checkRightArrow() {
}
/** * Checks to see if the up arrow key is being pressed. * If it is, then the ball sets its rotation angle to 270 degrees * and moves forward 5 units. If it is not being pressed, * this method does nothing. */ public void checkUpArrow() {
}
/** * Checks to see if the down arrow key is being pressed. * If it is, then the ball sets its rotation angle to 90 degrees * and moves forward 5 units. If it is not being pressed, * this method does nothing. */ public void checkDownArrow() {
} }
public class Button extends Actor { /** * Changes the location of this button approximately * 2% of the time. */ public void act() { } /** * Sets the location of this Button object to a new (x, y) * coordinate selected at random. The x coordinate wil be in * the range 100 to 500 inclusive, and the y coordinate will * be the range 100 to 300 inclusive. */ public void changeLocation() { } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
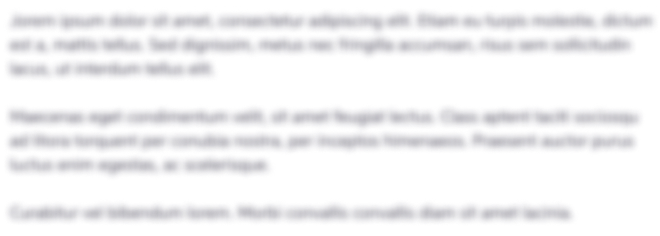
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started