Question
please Help this code is in C++ You are working at XYZ Engineering Associates and your job is to carry out a number of software
please Help this code is in C++
You are working at XYZ Engineering Associates and your job is to carry out
a number of software
engineering tasks related their new software project.
The following marketing requirements for a particular software component
are as follows:
Software should be designed to maintain a list of students (hereafter
referred to as the Student List).
The Student List is required to contain Student Records.
An interface should be designed to add a Student Record to the Student
List.
An interface should be designed to reset the list such that no Student
Records are contained in the list.
An interface should be designed to provide the Student Record with the
highest grade in the Student List.
A Student Record should contain an alphanumeric representation of the
student's name and the student's final grade.
The student's name can be any length. The student's final grade is
required to be between 0 and 100.
If a final grade is entered that is greater than 100, then the grade
should be truncated and set to 100.
If a final grade is entered that is less than 0, then the grade should be
truncated and set to 0.
The initial condition of the Student List is that it contains no Student
Records.
If there are no Student Records in the Student List, then the interface to
return the Student Record with
the highest grade should result in "No Entry" for the name and 0 for the
grade.
It is required that there be a maximum number of Student Records allowed
in the Student List. This maximum
number is set to a constant at the beginning of the software. If a Student
Record is added that exceeds
the maximum allowed, then ignore and discard that Student Record.
The above marketing requirements were received by the software team and
software was designed to meet the
marketing requirements with the following files and interfaces.
A class called Student that implements the Student Record of the
requirements. There are corresponding
Student.h and Student.cpp files for this class.
A class called StudentGrades that implements the Student List of the above
requirements. There are corresponding
StudentGrades.h and StudentGrades.cpp files for this class.
Here is the interface to the StudentGrades class:
StudentGrades::StudentGrades()
Student StudentGrades::getStudentWithHighestGrade() const
void StudentGrades::setStudentGrade(std::string name, int grade)
void StudentGrades::resetList()
The software engineering team is requesting that an additional technical
requirement be added that ensures
an assignment of a StudentGrades object to another StudentGrades object
does not casue any object
interdependencies (e.g. shallow copy issues). It is required that two test
cases for this technical requirement
be developed and performed by creating two StudentGrades objects, one
called "a" and the other called "b",
and then a command of b = a; is implemented. After b = a; is executed, the
objects must be equal
(use the getStudentWithHighestGrade() function to determine this). Then
another test must be created
to check for any object interdependencies.
Conditions for the following tasks:
For all the Tasks below, assume that the maximum number of Student Records
allowed in a Student List is set to 5.
Only use a maximum of 3 Student Records for any test case that is created
or implemented.
Therefore, you should not test for a condition that exceeds the maximum
number of Student Records.
You can only use the member functions of the StudentGrades class for your
tests.
Task 1 - You are taking the role of a software test engineer. Your task is
to write software black box
test cases for the above marketing requirements and software engineering
technical requirements.
You are to write test cases that cover nominal conditions and boundary
conditions for this software component.
See the class slides on software development starting on slide 14 for
examples and help on writing test cases.
No system level tests (e.g. testing for Memory Leaks) are required. Number
your Test Cases starting from 1.
The first Test Case is given to you here:
Test Case 1: When a StudentGrades object is first created, test that the
getStudentWithHighestGrade() function
returns "No Entry" for the name and 0 for the grade.
Submit your numbered test cases in a text file called task1.txt.
Task 2 - You are to write a main() program in C++ implementing the test
cases that were created
for Task 1. Your main() program will use the files StudentGrades.h,
StudentGrades.cpp,
Student.h, and Student.cpp that are provided in the zip file. Every place
in the main() function that implements
a test case should be preceded by a comment identifying that test case. A
main.cpp file is provided to you
that you must add your test case implementation to. Test Case 1 is already
implemented, so use this as
an example of how to write your next test cases. Hint: Before you start
writing new test cases, compile and execute
Test Case 1 to make sure everything is working well. The first test case
should fail. When all the test results
are completed, you will save the test case results.
Submit your main.cpp file and submit the test case results in a text file
called task2.txt.
Task 3 - You are now in the role of performing maintenance on the software
component that was just tested.
Using the test results that were performed in Task 2 and by performing
code inspections on the software,
you are to modify only the StudentGrades.h and StudentGrades.cpp files to
correct all defects.
Defects include any part of the software that does not meet the stated
requirements above, any part of the
software that is designed incorrectly and might cause software issues
either internal or external to this
component, and any coding standard violations of the XYZ Engineering
Associates. Fortunately, the coding
standards of XYZ Engineering Associates are the same as the ones we are
using in this class.
It is recommended that you create a new Project in Visual Studio to
perform this work. Note: You will need
to provide implementation for the copy constructor, assignment operator,
and destructor for the
StudentGrades class.
Submit your modified StudentGrades.h and StudentGrades.cpp files.
Task 4 - Make a numbered list of the defects that were corrected in Task
3. This numbered list should include
the reasons for any changes you made to the StudentGrades.h and
StudentGrades.cpp files. At the end of the
numbered defect list, give the reason why the destructor implementation is
needed and why the default copy
constructor and assignment operator implementation need to be overloaded
for the StudentGrades class.
Submit your defect list in a file called task4.txt.
Task 5 - With the modified code from Task 4, run the Test Cases
implemented in Task 2 and record the test case results.
Submit the test case results in a text file called task5.txt.
Task 6 (Extra Credit) - Comment on any missing or ambiguous marketing
requirements that could improve and clarify
the task of the software programmer and designer.
Submit your answers in a text file called task6.txt.
Here are the file codes:
(1)
main.cpp
include
#include "StudentGrades.h"
int main()
{
StudentGrades a;
StudentGrades b; // object b will be used for the tests that require
b = a;
Student s;
// Test Case 1
s = a.getStudentWithHighestGrade(); // get the student with highest
grade at initialization
std::cout << "Student " << s.getName() << " has highest grade of "
<< s.getGrade() << std::endl;
if ((s.getName().compare("No Entry") == 0) && (s.getGrade() == 0))
// if the test case is meet then Pass
std::cout << "Test Case 1 Passes" << std::endl;
else // else test case fails
std::cout << "Test Case 1 Fails" << std::endl;
// Test Case 2
}
(2)
Student.cpp
#include "Student.h"
#include
Student::Student()
{}
Student::Student(std::string n, int g)
: name(n), grade(g)
{}
Student::~Student()
{}
void Student::setName(std::string name)
{
this->name = name;
}
void Student::setGrade(int g)
{
grade = g;
}
std::string Student::getName() const
{
return name;
}
int Student::getGrade() const
{
return grade;
}
(3)
Student.h
#ifndef STUDENT_H
#define STUDENT_H
#include
class Student
{
public:
Student(); // default constructor
Student(std::string n, int g); // constructor
~Student(); // destructor
void setName(std::string name); // mutator function
void setGrade(int g); // mutator function
std::string getName() const; // accessor function
int getGrade() const; // accessor function
private:
std::string name; // holds the student name
int grade; // holds the student grage
};
#endif
(4)
StudentGrades.cpp
#include "StudentGrades.h"
#include
#include
StudentGrades::StudentGrades() : numberOfStudents(0)
{
students = new Student[MAX_STUDENTS];
}
StudentGrades::~StudentGrades()
{
}
Student StudentGrades::getStudentWithHighestGrade() const
{
Student returnValue = students[0];
for (int i = 0; i < (numberOfStudents-1); i++)
{
if (returnValue.getGrade() < students[i].getGrade())
{
returnValue = students[i];
}
}
return returnValue;
}
void StudentGrades::addStudentRecord(std::string name, int grade)
{
if (numberOfStudents < MAX_STUDENTS)
{
students[numberOfStudents].setName(name);
students[numberOfStudents].setGrade(grade);
numberOfStudents++;
}
}
void StudentGrades::resetList()
{
students[0].setName("No Entry");
students[0].setGrade(0);
for (int i = 1; i < numberOfStudents; i++)
{
students[i].setName("No Entry");
students[i].setGrade(0);
}
numberOfStudents = 0;
}
(5)
StudentGrades.h
#ifndef STUDENTGRADES_H
#define STUDENTGRADES_H
#include
#include "Student.h"
class StudentGrades
{
public:
StudentGrades(); // default constructor
~StudentGrades(); // destructor
Student getStudentWithHighestGrade() const; // accessor function
void addStudentRecord(std::string name, int grade);
void resetList();
private:
const static int MAX_STUDENTS = 5;
int numberOfStudents;
Student *students;
};
#endif
Thank you!!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
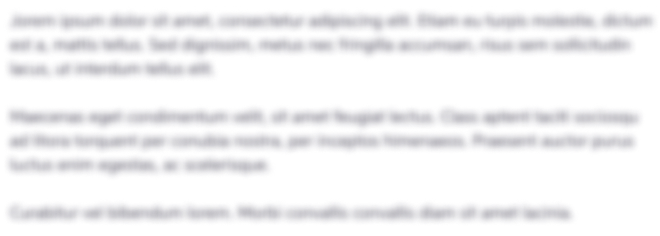
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started