Question
Please help to solve main method. Only need help on TestMyHashMap The TestMyHashMap class main method should follow the following algorithm: Create a MyHashMap object
Please help to solve main method. Only need help on TestMyHashMap
The TestMyHashMap class main method should follow the following algorithm:
Create a MyHashMap object that has FileChar keys and HuffElement values.
Read the FDREconomics.txt file into a String object using the provided readFile method.
Walk through the String object, character-by-character, to record the character frequency in the String object:
For each new different character encountered you must instantiate a new FileChar object and add the character to it. Then you must instantiate a HuffElement object and add the character to it and increment the character count in it. This map entry pair is then added to the HashMap object you created.
For each character previously encountered you must use the FileChar key object for it to retrieve the HuffElement value object and increment the character count in it.
Once you have completely read the whole file, you must retrieve the key set from the hash map and iterate through the key set using a for each loop and for each key, retrieve the character from the FileChar key and the count from the HuffElement value and print these, as follows:
Sample Output:
Char: D Count: 1
Char: E Count: 1
Char: F Count: 2
Char: A Count: 4
Char: d Count: 49
Char: Count: 271
Char: e Count: 149
Char: b Count: 12
Char: c Count: 42
Char: ' Count: 2
Here is the other java File
1. FileChar
public class FileChar { private char ch;
FileChar() { ch = 0; } FileChar(char c) { ch = c; } public char getChar() { return ch; } public void setChar(char c) { ch = c; } public int hashCode() { return (int)ch; } public int compareTo(FileChar ch) { if(this.compareTo(ch)>0) { return 1; } else if(this.compareTo(ch)<0) { return -1; } else { return 0; } } public boolean equals(Object chr) { return this.ch== ((FileChar)chr).ch; } public String toString() { return ch+""; } }
2. HuffElement
public class HuffElement { private char ch; private int chCount; private String code; public HuffElement() { ch = 0; chCount = 0; code = ""; } public HuffElement (char c) { this.ch = c; chCount = 0; code = ""; } public char getChar() { return ch; } public void setChar(char c) { ch = c; } public int getCount() { return chCount; } public void setCount(int c) { chCount = c; } public void incCount() { chCount++; } public String getCode() { return code; } public void setCode (String c) { code = c; } public int compareTo (HuffElement he) { if(this.getCount() > he.getCount()) { return 1; } else if(this.getCount() < he.getCount()) { return -1; } else { return 0; } } public String toString() { return "Char: " +ch+ " Code: " +code+ " Count: "+chCount; } }
3. MyHashMap
import java.util.LinkedList;
public class MyHashMap
// Define the maximum hash table size. 1 << 30 is same as 2^30 private static int MAXIMUM_CAPACITY = 1 << 30;
// Current hash table capacity. Capacity is a power of 2 private int capacity;
// Define default load factor private static float DEFAULT_MAX_LOAD_FACTOR = 0.75f;
// Specify a load factor used in the hash table private float loadFactorThreshold;
// The number of entries in the map private int size = 0;
// Hash table is an array with each cell that is a linked list LinkedList
/** Construct a map with the default capacity and load factor */ public MyHashMap() { this (DEFAULT_INITIAL_CAPACITY, DEFAULT_MAX_LOAD_FACTOR); }
/** Construct a map with the specified initial capacity and * default load factor */ public MyHashMap (int initialCapacity) { this (initialCapacity, DEFAULT_MAX_LOAD_FACTOR); }
/** Construct a map with the specified initial capacity * and load factor */ public MyHashMap (int initialCapacity, float loadFactorThreshold) { if (initialCapacity > MAXIMUM_CAPACITY) { this.capacity = MAXIMUM_CAPACITY; } else { this.capacity = trimToPowerOf2 (initialCapacity); }
this.loadFactorThreshold = loadFactorThreshold;
table = new LinkedList[capacity]; }
/** Remove all of the entries from this map */ @Override public void clear() { size = 0; removeEntries(); }
/** Return true if the specified key is in the map */ @Override public boolean containsKey(K key) { if (get(key) != null) { return true; } else { return false; } }
/** Return true if this map contains the value */ @Override public boolean containsValue(V value) { for (int i = 0; i < capacity; i++) { if (table[i] != null) { LinkedList
/** Return a set of entries in the map */ @Override public java.util.Set
for (int i = 0; i < capacity; i++) { if (table[i] != null) { LinkedList
/** Return the value that matches the specified key */ @Override public V get (K key) { int bucketIndex = hash(key.hashCode()); if (table[bucketIndex] != null) { LinkedList
/** Return true if this map contains no entries */ @Override public boolean isEmpty() { return size == 0; }
/** Return a set consisting of the keys in this map */ @Override public java.util.Set
for (int i = 0; i < capacity; i++) { if (table[i] != null) { LinkedList
/** Add an entry (key, value) into the map */ @Override public V put(K key, V value) { if (get(key) != null) // key already in map { int bucketIndex = hash (key.hashCode());
LinkedList
// Replace old value with new value entry.value = value;
// Return the old value for the key return oldValue; } } }
// Check load factor if (size >= capacity * loadFactorThreshold) { if (capacity == MAXIMUM_CAPACITY) { throw new RuntimeException ("Exceeding maximum capacity"); }
rehash(); }
int bucketIndex = hash(key.hashCode());
// Create a linked list for the bucket if it is not created if (table[bucketIndex] == null) { table[bucketIndex] = new LinkedList
// Add a new entry (key, value) to hashTable[index] table[bucketIndex].add (new MyMap.Entry
size++; // Increase size
return value; }
/** Remove the entries for the specified key */ @Override public void remove(K key) { int bucketIndex = hash (key.hashCode());
// Remove the first entry that matches the key from a bucket if (table[bucketIndex] != null) { LinkedList
/** Return the number of entries in this map */ @Override public int size() { return size; }
/** Return a set consisting of the values in this map */ @Override public java.util.Set
for (int i = 0; i < capacity; i++) { if (table[i] != null) { LinkedList
/** Hash function */ private int hash (int hashCode) { return supplementalHash (hashCode) & (capacity - 1); }
/** Ensure the hashing is evenly distributed */ private static int supplementalHash(int h) { h = h ^ (h >>> 20) ^ (h >>> 12); return h ^ (h >>> 7) ^ (h >>> 4); }
/** Return a power of 2 for initialCapacity */ private int trimToPowerOf2(int initialCapacity) { int capacity = 1; while (capacity < initialCapacity) { capacity <<= 1; }
return capacity; }
/** Remove all entries from each bucket */ private void removeEntries() { for (int i = 0; i < capacity; i++) { if (table[i] != null) { table[i].clear(); } } }
/** Rehash the map */ private void rehash() { java.util.Set
capacity = capacity << 1; // Double capacity
table = new LinkedList[capacity]; // Create a new hash table
size = 0; // Reset size to 0
for (Entry
@Override public String toString() { StringBuilder builder = new StringBuilder("[");
for (int i = 0; i < capacity; i++) { if (table[i] != null && table[i].size() > 0) { for (Entry
builder.append("]");
return builder.toString(); } }
4. MyMap
public interface MyMap
/** Return true if the specified key is in the map */ public boolean containsKey(K key);
/** Return true if this map contains the specified value */ public boolean containsValue(V value);
/** Return a set of entries in the map */ public java.util.Set
/** Return the first value that matches the specified key */ public V get(K key);
/** Return true if this map contains no entries */ public boolean isEmpty();
/** Return a set consisting of the keys in this map */ public java.util.Set
/** Add an entry (key, value) into the map */ public V put(K key, V value);
/** Remove the entries for the specified key */ public void remove(K key);
/** Return the number of mappings in this map */ public int size();
/** Return a set consisting of the values in this map */ public java.util.Set
/** Define inner class for Entry */ public static class Entry
public Entry (K key, V value) { this.key = key; this.value = value; }
public K getKey() { return key; }
public V getValue() { return value; }
@Override public String toString() { return "[" + key + ", " + value + "]"; } } }
5. TestMyHashMap
import java.util.*; import java.io.*; public class TestMyHashMap { // File to read and characters counted
private static final String INPUT_FILE = ".\\src\\FDREconomics.txt"; private static final int NUM_ASCII_CHARS = 127; public static void main (String[] args) throws Exception { // Read in the whole plaintext file to a string String fileStr = readFile (INPUT_FILE);
// Create a map // Populate the HuffMap with FileChar, HuffElement entries // containing characters and character frequency counts
/* Code todo */ // Retrieve the set of keys // Loop through the keys: // Print each character from the FileChar object // Print the count from the HuffElement object
/* Code todo */ map.containsKey(fileStr); }
/** * Reads the input file into a String * * @param filepath Path to the file to be read. * @throws Exception */ private static String readFile (String filepath) throws Exception { String text = ""; Scanner input;
File file = new File (filepath); input = new Scanner (file);
while (input.hasNext()) { text += input.nextLine(); }
input.close();
return text; } }
6. FDREconomics.txt
FDR's Economic Bill of Rights
In our day these economic truths have become accepted as self-evident. We have accepted, so to speak, a second Bill of Rights under which a new basis of security and prosperity can be established for all regardless of station, race, or creed.
Among these are: 1. The right to a useful and remunerative job in the industries or shops or farms or mines of the Nation; 2. The right to earn enough to provide adequate food and clothing and recreation; 3. The right of every farmer to raise and sell his products at a return which will give him and his family a decent living; 4. The right of every businessman, large and small, to trade in an atmosphere of freedom from unfair competition and domination by monopolies at home or abroad; 5. The right of every family to a decent home; 6. The right to adequate medical care and the opportunity to achieve and enjoy good health; 7. The right to adequate protection from the economic fears of old age, sickness, accident, and unemployment; 8. The right to a good education.
All of these rights spell security. And after this war is won wee must be prepared to move forward, in the implementation of these rights, to new goals of human happiness and well-being.
America's own rightful place in the world depends in large part upon how fully these and similar rights have been carried into practice for our citizens. For unless there is security here at home there cannot be lasting peace in the world.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
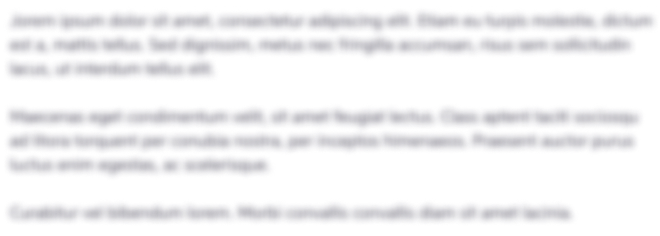
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started