Question
Please help with agent-based modeling! Coding language- Python. Any help is greatly appreciated! Code to copy: # create the environment (the ground) in an array
Please help with agent-based modeling! Coding language- Python. Any help is greatly appreciated!
Code to copy:
# create the environment (the ground) in an array
# rows size_1 = 20 # columns size_2 = 20 # fill the2D array with random numbers between 0 and 1 yard = np.random.random((size_1, size_2))
# add a wall to the yard
yard[5:14,5] = 4 yard[5:15,14] = 4 yard[5,5:14] = 4 yard[14,5:15] = 4
# add a skunk inside the yard
skunk_row = np.random.randint(6,14) skunk_col = .... ###
yard[skunk_row, skunk_col] = 2 new_skunk_row = skunk_row new_skunk_col = .... ###
# loop over time
num_steps = .... ### for time_step in range(num_steps):
# pick a random direction using four possible integers direction = np.random.randint(0,4)
# compute possible new position given current position and direction if direction == 0: new_skunk_row = skunk_row - 1 elif direction == 1: new_skunk_row = skunk_row + 1 elif direction == 2: new_skunk_col = skunk_col - .... ### elif direction == 3: .... ###
# check to see if the possible new position is the wall if yard[new_skunk_row, new_skunk_col] == 4: print("Skunk bumped into the wall - ouch!") else: # not the wall, skunk moves skunk_row = new_skunk_row skunk_col = .... ### yard[skunk_row,skunk_col] = 3
# let's see where the skunk is right now fig = plt.figure(figsize=(8,8)) plt.imshow(yard) # play with different colors! # plt.imshow(data_new, cmap='plasma') # plt.imshow(data_new, cmap='magma') # plt.imshow(data_2, cmap = 'jet') plt.show() sleep(0.5) clear_output(wait=True)
THANK YOU
Part 3: Simple Agent-Based Model with 2D NumPy Arrays In this portion of the course, we are examining three modeling approaches that use: 1. data, 2. equations (ODE/compartmental), 3. rules In this part of the HW, you will build a simple rule-based model. You live in a house with a backyard that is enclosed by a high brick wall. A skunk climbs onto the wall from tree branch and falls into your yard; there is no way for it to escape now that it is on the ground surrounded by a high wall. You want to model the skunk's movements around the yard, hopefully to guide you were to put a trap so that you can safely relocate it to its home. This is a type of agent-based model (ABM) in which the agent is the skunk, which interacts only with its environment Question #7 (15 points) Write a code that implements these rules: 1. The ground will be represented by a 2D array, initialize the ground to a 20 x 20 array with values between 0 and 1. Copy any code from above that might be useful! 2. Add a wall to the environment by changing the values of the environment to a value of 4 in the form of a square centered in the environment with side length 10. Copy any code from above that might be helpful! 3. Add the skunk at a random place inside the yard that is enclosed by the wall. Again, use previous code! 4. Loop for 100 steps. At each step, choose a random number that selects a direction up, down, left or right. (Do this however you want, but consider using randint from NumPy.) 5. Change the location of the skunk according the movement choice just made and update the environment array to put the skunk at the new, updated location. 6. But, if the skunk moves into the wall, do not update that movement: do nothing. 7. Make a movie using imshow of this random path of the skunk. Please copy any code from above that might be useful. Pick a good color scheme for your ABM. Consider making the ground brown, the skunk black, etc. As above, some of the code is given here to help you get started and .... ### is used where code has been removed for you to fill in. But, feel free to change any of it, including starting over with your own code from scratch. [@]: # create the environment (the ground) in an array # rows size_1 = 20 # columns size_2 = 20 # fill the2D array with random numbers between @ and 1 yard = np.random.random( (size_1, size_2)) # add a wall to the yard yard[5:14,5) = 4 yard[5:15,14] = 4 yard[5,5:14] = 4 yard(14,5:15] = 4 # add a skunk inside the yard skunk_row = np.random.randint(6,14) skunk_col = .... *** yard[skunk_row, skunk_col] = 2 new_skunk_row = skunk_row new_skunk_col = .... *** # Loop over time num_steps = .... *** for time_step in range(num_steps): # pick a random direction using four possible integers direction = np.random.randint(0,4) # compute possible new position given current position and direction if direction == : new_skunk_row = skunk_row - 1 elif direction == 1: new_skunk_row = skunk_row + 1 elif direction == 2: new_skunk_col = skunk_col - .... ### elif direction == 3: # check to see if the possible new position is the wall if yard[new_skunk_row, new_skunk_col] == 4: print("Skunk bumped into the wall - ouch!") else: # not the wall, skunk moves skunk_row = new_skunk_row skunk_col = .... *** yard[Skunk_row, skunk_col] = 3 # Let's see where the skunk is right now fig = plt.figure(figsize=(8,8)) plt.imshow(yard) # play with different colors! # plt. imshow(data_new, cmap='plasma') # plt. imshow(data_new, Cmap='magma') # plt. imshow(data_2, map = 'jet') plt.show() sleep(0.5) clear_output(wait=True) Next, modify your code to allow the skunk a bit more flexibility in how it can move around. In ABM models of this type the use of "up","down", "left" and "right" is called using the_von Neumann neighborhood, and it looks like this: The blue square is where the skunk is now, and the red cells are where it can go. We won't use it here, but the lighter red is called the extended von Neumann neighborhood. Instead, modify your code to have a smarter skunk that can move in more directions, like this: This is called the Moore neighborhood. Use the code you wrote above by simply modifying it to allow for both the von Neumann and Moore neighborhoods. Create a Boolean variable that is True for von Neumann and False for Moore at the beginning of your code. Then, write the code to use that variable to pick the neighborhood choice. In this case, take the part of your code that implements the skunk's possible next move and put that into a function using def called move_skunk, which takes as its inputs the current location of the skunk and the Boolean variable. The function returns a possible new position for the skunk using the Boolean variable to decide which neighborhood to use. As this class progresses and your codes get longer think about how you can use more and more functions to take care of smaller tasks like this one. (For example, you could also write a function hit_wall that tests to see if the skunk hit the wall, returning a Boolean variable.) In many ABM models we need to make choices like this one. Which choice we make depends on the particular problem we are trying to solve. Which of these neighborhoods do you think better describes a skunk? Part 3: Simple Agent-Based Model with 2D NumPy Arrays In this portion of the course, we are examining three modeling approaches that use: 1. data, 2. equations (ODE/compartmental), 3. rules In this part of the HW, you will build a simple rule-based model. You live in a house with a backyard that is enclosed by a high brick wall. A skunk climbs onto the wall from tree branch and falls into your yard; there is no way for it to escape now that it is on the ground surrounded by a high wall. You want to model the skunk's movements around the yard, hopefully to guide you were to put a trap so that you can safely relocate it to its home. This is a type of agent-based model (ABM) in which the agent is the skunk, which interacts only with its environment Question #7 (15 points) Write a code that implements these rules: 1. The ground will be represented by a 2D array, initialize the ground to a 20 x 20 array with values between 0 and 1. Copy any code from above that might be useful! 2. Add a wall to the environment by changing the values of the environment to a value of 4 in the form of a square centered in the environment with side length 10. Copy any code from above that might be helpful! 3. Add the skunk at a random place inside the yard that is enclosed by the wall. Again, use previous code! 4. Loop for 100 steps. At each step, choose a random number that selects a direction up, down, left or right. (Do this however you want, but consider using randint from NumPy.) 5. Change the location of the skunk according the movement choice just made and update the environment array to put the skunk at the new, updated location. 6. But, if the skunk moves into the wall, do not update that movement: do nothing. 7. Make a movie using imshow of this random path of the skunk. Please copy any code from above that might be useful. Pick a good color scheme for your ABM. Consider making the ground brown, the skunk black, etc. As above, some of the code is given here to help you get started and .... ### is used where code has been removed for you to fill in. But, feel free to change any of it, including starting over with your own code from scratch. [@]: # create the environment (the ground) in an array # rows size_1 = 20 # columns size_2 = 20 # fill the2D array with random numbers between @ and 1 yard = np.random.random( (size_1, size_2)) # add a wall to the yard yard[5:14,5) = 4 yard[5:15,14] = 4 yard[5,5:14] = 4 yard(14,5:15] = 4 # add a skunk inside the yard skunk_row = np.random.randint(6,14) skunk_col = .... *** yard[skunk_row, skunk_col] = 2 new_skunk_row = skunk_row new_skunk_col = .... *** # Loop over time num_steps = .... *** for time_step in range(num_steps): # pick a random direction using four possible integers direction = np.random.randint(0,4) # compute possible new position given current position and direction if direction == : new_skunk_row = skunk_row - 1 elif direction == 1: new_skunk_row = skunk_row + 1 elif direction == 2: new_skunk_col = skunk_col - .... ### elif direction == 3: # check to see if the possible new position is the wall if yard[new_skunk_row, new_skunk_col] == 4: print("Skunk bumped into the wall - ouch!") else: # not the wall, skunk moves skunk_row = new_skunk_row skunk_col = .... *** yard[Skunk_row, skunk_col] = 3 # Let's see where the skunk is right now fig = plt.figure(figsize=(8,8)) plt.imshow(yard) # play with different colors! # plt. imshow(data_new, cmap='plasma') # plt. imshow(data_new, Cmap='magma') # plt. imshow(data_2, map = 'jet') plt.show() sleep(0.5) clear_output(wait=True) Next, modify your code to allow the skunk a bit more flexibility in how it can move around. In ABM models of this type the use of "up","down", "left" and "right" is called using the_von Neumann neighborhood, and it looks like this: The blue square is where the skunk is now, and the red cells are where it can go. We won't use it here, but the lighter red is called the extended von Neumann neighborhood. Instead, modify your code to have a smarter skunk that can move in more directions, like this: This is called the Moore neighborhood. Use the code you wrote above by simply modifying it to allow for both the von Neumann and Moore neighborhoods. Create a Boolean variable that is True for von Neumann and False for Moore at the beginning of your code. Then, write the code to use that variable to pick the neighborhood choice. In this case, take the part of your code that implements the skunk's possible next move and put that into a function using def called move_skunk, which takes as its inputs the current location of the skunk and the Boolean variable. The function returns a possible new position for the skunk using the Boolean variable to decide which neighborhood to use. As this class progresses and your codes get longer think about how you can use more and more functions to take care of smaller tasks like this one. (For example, you could also write a function hit_wall that tests to see if the skunk hit the wall, returning a Boolean variable.) In many ABM models we need to make choices like this one. Which choice we make depends on the particular problem we are trying to solve. Which of these neighborhoods do you think better describes a skunkStep by Step Solution
There are 3 Steps involved in it
Step: 1
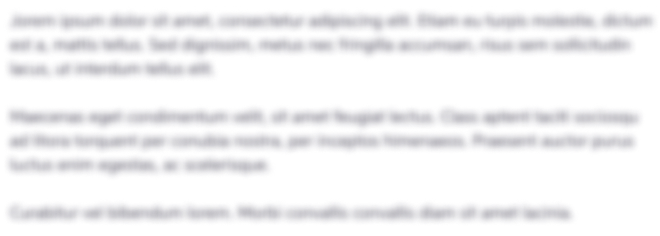
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started