Question
Please help write the last method- the instructions are listed. Thanks! import java.io.File; import java.io.PrintWriter; /** * Simulates playing the sort card game: * 1.
Please help write the last method- the instructions are listed. Thanks!
import java.io.File; import java.io.PrintWriter; /** * Simulates playing the sort card game: * 1. Places each Card from a shuffled Deck on a Stack on the Table * using the rules of the game. * 2. At the same time a copy of the Card is placed on the top of a * sequence LinkedList such that it is pointing to the top * Card of the stack previous to the stack on which it is placed * 3. Prints a longest decreasing subsequence for each Card in last stack * 4. Uses an additional stack to reverse the subsequence and print the * corresponding longest increasing subsequence * 5. Uses the Card pile stacks on the Table to return a sorted Deck * 6. Passes in the PrintWriter object to the playGame method * to make it easier to print the output to File. */
public class SortCardGame { private static final String OUTPUT_FILE = "./src/SortGameOut.txt";
public static void main(String[] args) throws Exception { int runs = 5; // number of games to play,
// Create an output File writer
File outFile = new File (OUTPUT_FILE); PrintWriter writer = new PrintWriter (outFile);
// Display number of runs
System.out.println ("Playing " + runs + " games.");
// Loop to play the game for the number of specified runs // Display the Begin Game and End Game messages
for (int run = 1; run <= runs; run++) { writer.println(" =========== Begin Game " + run + " ==========");
playGame (writer); // Play the Game
writer.println("=========== End Game " + run + " =========="); }
writer.close();
System.out.println ("Simulation complete.");
return; }
// Simulates playing the sort card game and finding longest subsequences: // 1. As each Card is placed on the correct stack on the Table, it is // also placed correctly in a linked list of Cards forming an // ordered longest subsequence. // 2. Prints a longest decreasing and increasing subsequence for // each Card in last stack // 3. After the Cards have all been placed on the Table in their // correct stack, they are picked back up in sorted ordrer. // // Algorithm: // 1.Create the Table and Deck objects // 2.Print the Deck before shuffling // 3.Shuffle the deck 7 times, calling the Deck shuffle method // 4.Print the Deck after shuffling // 5.Construct the card piles on the Table, calling the // Table constructCardPiles method // 6.Print the card piles before doing the sort // calling the Table printCardPiles method // 7.Print the longest subsequences, calling // the Table printLongestSubSeqs method // 8.Use the piles on the Table to make a sorted Deck, // calling the Table makeSortedDeck method // 9.Display the sorted Deck
public static void playGame (PrintWriter writer) { // code todo } }
............................................................................................
public class Table { // Holds the stacks of cards in an ArrayList of Card Stacks private MyArrayList
// Holds a list of sequences in an ArrayList of Card Linked Lists
private MyArrayList
// The number of Stacks of Cards in the ArrayList
private int numPiles;
// Constructor: initialize instance variables
public Table() { // code todo piles = null; subSeqs = null; numPiles = 0; }
// This method creates the piles (stacks) from the Deck of Cards, // according to the following rules: // A. Initially, there are no piles. The first Card dealt // forms a new pile consisting of the first Card. // B. Each new Card picked from the Deck must be placed on top // of the leftmost pile (lowest MyArrayList index), whose // top Card has a value higher than the new Card's value. // C. If there are only piles with top Cards that are lower // in value than the new Card's value, then use the new // Card to start a new pile to the right of all the // existing piles (at end of MyArrayList of MyStack piles) // D. Save card in ArrayList of LinkedList subsequences (See below) // E. The game ends when all the cards have been dealt. // // Dealing the cards in this way provides us a way of retrieving // a subset of the longest increasing and decreasing subsequences. // A. The number of piles is the length of a longest subsequence. // B. For each new Card, add a copy of that card to the list of // subsequences and link it to the top Card in the previous // pile to the left of this pile - the one with the lower // ArrayList index - By design, the pile's top Card has a // lower value than the value of the new Card. // // The Algorithm: // Loop retrieving each card in the Deck // 1. Retrieve the card from the Deck: cardFromDeck // 2. Set a flag to indicate if we placed the // cardFromDeck in an existing pile // 3. Loop through each pile starting from the // leftmost pile - ArrayList index 0 - to find // the correct one on which to place the cardFromDeck // a. Retrieve top Card on the Stack using peek // b. If there exists a pile whose top Card is // is higher than the cardFromDeck, then // i. Set flag to say we have found a pile on // which to place the cardFromDeck // ii. Retrieve a reference to the top Card // on the previous pile - the one to the // left of where you just placed the // cardFromDeck: (one less index value // in ArrayList) // iii. Add the cardFromDeck to the list of // subsequences using the addCardToSubSeq method // iv. Push the cardFromDeck onto the pile // 4. Check the flag: // If we haven't found a place for the cardFromDeck // in an existing pile, then // a. Create a new pile (in ArrayList of Stacks) // b. Retrieve a reference to the top Card on the // previous pile, - the one to the left of where // you just placed the cardFromDeck: (one less // index value in ArrayList), unless this first // card from the Deck: numPiles equal 0 // c. Add the cardFromDeck to the list of // subsequences using the addCardToSubSeq method // d. Add the cardFromDeck onto the pile // e. Increment the pile count
public void constructCardPiles (Deck deck) { // code todo Card cardFromDeck = null; Card cardFromTable = null;
// Loop through each card in the Deck
for (int count = 0; count < deck.size(); count++) { // Retrieve the card from the Deck: cardFromDeck
cardFromDeck = deck.get (count);
// Set a flag to indicate if we placed the // cardFromDeck in an existing pile
boolean cardPlaced = false;
// Loop through each pile starting from the leftmost // pile - ArrayList index 0 - to find the correct // one on which to place the cardFromDeck
for (int index = 0; index < numPiles; index++) { // Retrieve top Card on the Stack using peek
cardFromTable = piles.get (index).peek();
// If there exists a pile whose top Card is // is higher than the cardFromDeck, then // a. Set flag to say we have found a pile // on which to place the cardFromDeck // b. Retrieve a reference to the top Card // on the previous pile - the one to the // left of where you just placed the // cardFromDeck: (one less index value // in ArrayList) // c. Add the cardFromDeck to the list of // subsequences using the addCardToSeq // method // d. Push the cardFromDeck onto the Stack
if (cardFromTable.compareTo (cardFromDeck) > 0) { // Set flag to say we have found a pile // on which to place the cardFromDeck
cardPlaced = true;
// Retrieve a reference to the top Card // on the previous pile - the one to the // left of where you just placed the // cardFromDeck: (one less index value // in ArrayList)
Card cardFromPrevPile = null;
if (index > 0) { cardFromPrevPile = piles.get (index - 1).peek(); }
// Add the cardFromDeck to the list of // subsequences using the addCardToSeq method
addCardToSubSeq (cardFromDeck, cardFromPrevPile);
// Push the cardFromDeck onto the Stack
piles.get (index).push (cardFromDeck);
break; } }
// Check flag: // If we haven't found a place for the cardFromDeck // in an existing pile, then // a. Create a new pile (in ArrayList of Stacks) // b. Retrieve a reference to the top Card on the // previous pile, - the one to the left of where // you just placed the cardFromDeck: (one less // index value in ArrayList), unless this first // card from the Deck: numPiles equal 0 // c. Add the cardFromDeck to the list of // subsequences using the addCardToSeq method // d. Add the cardFromDeck onto the pile // e. Increment the pile count
if (!cardPlaced) { // Create a new pile (in ArrayList of Stacks)
piles.add (new MyStack
// Retrieve a reference to the top Card on the // previous pile, - the one to the left of where // you just placed the cardFromDeck: (one less // index value in ArrayList), unless this first // card from the Deck: numPiles equal 0
Card cardFromPrevPile = null; if (numPiles > 0) { cardFromPrevPile = piles.get (numPiles - 1).peek(); }
// Add the cardFromDeck to the list of // subsequences using the addCardToSeq method
addCardToSubSeq (cardFromDeck, cardFromPrevPile);
// Add the cardFromDeck onto the pile
piles.get (numPiles).push (cardFromDeck);
// Increment the pile count
numPiles++; } } }
// Use the passed in PrintWriter to print each pile // on the ArrayList of Card Stacks
public void printCardPiles (PrintWriter writer) { // code todo for (int i = 0; i < piles.size; i++) { for (int j = 0; j < piles.get(i).getSize(); j++) { writer.print(piles.get(i).getList().get(j).toString()); }//for j }//for i }
// Use the passed in PrintWriter to print each of the decreasing // and increasing subsequences // // The Algorithm: // 1. Loop through the subsequence list and obtain each // subsequence whose size equals the number of piles - // the longest subsequences - Use the PrintWriter to // print each of these subsequence // 2. Loop through the subsequence list again and obtain // each subsequence whose size equals the number of piles // - this time reverse the subsequence and use the // PrintWriter to print it
public void printLongestSubSeqs (PrintWriter writer) { // code todo //descending order for (int i = (piles.size - 1); i >= 0; i--) { if (piles.get(i).getSize() == i) { for (int j = 0; j < piles.get(i).getSize(); j++) { writer.print(piles.get(i).getList().get(j) + ", "); }//for j writer.println(); }//if }//for i //ascending order for (int i = 0; i > piles.size; i--) { if (piles.get(i).getSize() == i) { for (int j = 0; j < piles.get(i).getSize(); j++) { writer.print(piles.get(i).getList().get(j) + ", "); }//for j writer.println(); }//if }//for i }
// Builds a sorted Deck by looking at the top Card of each pile // (stack) and grabbing the card with the lowest value; thus, // Cards are picked up in sorted order and added to the new Deck // // Algorithm: // Loop for each card in the deck // 1. Keep an index to the pile with the lowest card, so far // 2. Keep the lowest Card found so far - initialize it to // the highest card for starters // 3. Walk across the piles - skipping empty ones - and find // the ArrayList index of the Stack with smallest card by // comparing the top card in the pile to the current // lowest card by using the Card compareTo method - // Dont' pop the card yet; it may not be the overall // lowest - Update the the lowest Card and its ArrayList // index along the way // 4. Now that we have exited the walk of the piles, we know // the index of the stack with the lowest Card - pop that // Card from the stack and put it on the new sorted Deck
public void makeSortedDeck (Deck deck) { // code todo // Loop for each card in the deck for (int i = 0; i < deck.size(); i++) { // Keep an index to the pile with the lowest card, so far
int lowStack = 0;
// Keep the lowest Card found so far // Initialize it to the highest card for starters
Card lowCard = new Card (13, 4);
// Walk across the piles (stacks) and get the // index of the pile (stack) with smallest card
for (int stk = 0; stk < numPiles; stk++) { // Walk across the piles - skipping empty ones -
if (!piles.get (stk).isEmpty()) { // Find the ArrayList index of the Stack with // smallest card by comparing the top card in the // pile to the current lowest card by using the // Card compareTo method - (Dont' pop the card // yet; it may not be the overall lowest) - // Update the the lowest Card and its ArrayList // index along the way
if (lowCard.compareTo (piles.get (stk).peek()) >= 0) { lowCard = piles.get (stk).peek(); lowStack = stk; } } }
// Now that we have exited the walk of the piles, we know // the index of the stack with the lowest Card - pop that // Card from the stack and put it on the new sorted Deck
lowCard = piles.get (lowStack).pop(); deck.set (i, lowCard); } }
// Adds a copy of the currentCard to one of the subsequences // in the subsequence list. The correct subsequence on which to // add the currrentCard is the one on which the previousCard // currently resides. // // The Algorithm: /// 1. Make a copy of the currentCard, using Card's copy // constructor. /// 2. Find the subsequence containing the previousCard // by calling the findSubSeq method /// 3. If there is no subsequence (null), create a new // subsequence LinkedList and add the copy of the // currentCard to the new subsequence and add the new // subsequence to the ArrayList of LinkedList of subsequences // 4. If there was a subsequence containing the previousCard, // then check to see if the previousCard is at the front // of the subsequence LinkedList: //// a. If the previousCard is at the head, then just add the // the copy of the currentCard to the front of the // subsequence LinkedList, thus linking the two Cards // b. If the previousCard is not at the head, // then we must //// i. Copy the subsequence containing the previousCard // by calling the copySubSeq method //// ii. Add the copied subsequence to the ArrayList of // subsequences //// iii. Traverse the copied subsequence from the head, // removing each Card that is not the previousCard. // Break out of the loop when finding the previousCard. // - Hint: You can use a FOR EACH loop //// iv. Now, the previousCard is at the front of the // subsequence, so we can add the copy of the // currentCard to the front of the subsequence, // thus linking the two Cards
private void addCardToSubSeq (Card currentCard, Card previousCard) { // code todo Card current = new Card(currentCard); Card previous = new Card(previousCard); Card currentCopy = new Card(currentCard); MyLinkedList
// Find the first subsequence in the ArrayList of // subsequences that contains the Card passed in // The Algorithm: /// 1. Make sure the card passed in is not null, // if so return null. // 2. Traverse the ArrayList of subsequence LinkedLists, // checking to see if the subsequence contains the // card passed in - Hint: You can use a FOR EACH loop // 3. Return the subsequence when found
private MyLinkedList
// Make a copy of a subsequence LinkedList by copying each Card // and adding the new copy to the new subsequence. // // The Algorithm: /// 1. Create a new LinkedList to hold the new subsequence /// 2. Traverse the passed in LinkedList, create a new Card from // each Card using the Card copy constructor and then add // each new Card to the end of the new subsequence LinkedList // Hint: You can use a FOR EACH loop /// 3. Return the new subsequence.
private MyLinkedList
// Reverse the given subsequence using a stack // // The Algorithm: /// 1. Create a new LinkedList to hold the new subsequence /// 2. Create a Stack to use to do the reversing /// 3. Traverse the passed in LinkedList and place each // Card on the Stack - Hint: You can use a FOR EACH loop /// 4. Loop through the Stack, popping off the Cards and // adding them to the end of the new subsequence LinkedList // 5. Return the new subsequence.
public MyLinkedList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
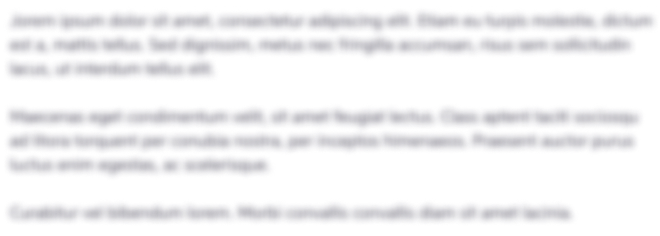
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started