Question
PLEASE HURRY. Below is the prompt for this problem. Use the code for bag1.cxx, bag1.h and my code for bag.cpp. Also I have provided errors
PLEASE HURRY. Below is the prompt for this problem. Use the code for bag1.cxx, bag1.h and my code for bag.cpp. Also I have provided errors from linux for bag.cpp. Please use that code and fix my errors please. Thank you
The goal of assignment 3 is to reinforce implementation of container class concepts in C++. Specifically, the assignment is to do problem 3.5 on page 149 of the text. You need to implement the set operations union, intersection, and relative complement. Your code must implement the style guidelines of the author (preconditions, postconditions, class invariant, and function efficiencies). Your test program should use a unit test style test each implemented function and clearly label your output.
You will be provided with bag1.h and bag1.cpp files as reference. You will need to adapt this class to implement a set.
Here are some typical features of a set:
- A set can have unique elements only no duplicates allowed
- Union of 2 sets s1 s2 = a new set with all elements from s1 and s2 without any duplicates
If s1{1,2,3}, s2{3,4,5}
then s1s2 = {1,2,3,4,5}
- Intersection of 2 sets s1s2 = a new set with elements common to both s1 and s2 => {3}
- Relative complement of s1 to s2 = a new set with all elements from s1 that are not present in s2 => {1,2}
Here are the changes you should ideally be making to the .h and implementation files:
- Change the name of the class to set and rename the files to set.h and set.cpp
- You will need to add new functions Union, Intersection and Relative complement, these will need to be non- member friend functions.
- You should also add a function called contains that checks to see if an entry is already present in the set. It should return a boolean value. This function should be a private function that can be called anytime, you need to insert an entry into a set (to make sure you dont add duplicate entries).
- You do not need the following functions from the bag class:
- Erase
- Count
- += overloaded function
- + overloaded function
- Do not add any more functions besides the ones listed under 2. & 3.
- Test the 3 set operations and clearly label your input and outputs.
bag1.cxx
// FILE: bag1.cxx // CLASS IMPLEMENTED: bag (see bag1.h for documentation) // INVARIANT for the bag class: // 1. The number of items in the bag is in the member variable used; // 2. For an empty bag, we do not care what is stored in any of data; for a // non-empty bag the items in the bag are stored in data[0] through // data[used-1], and we don't care what's in the rest of data.
#include
namespace main_savitch_3 { const bag::size_type bag::CAPACITY; bag::size_type bag::erase(const value_type& target) { size_type index = 0; size_type many_removed = 0;
while (index < used) { if (data[index] == target) { --used; data[index] = data[used]; ++many_removed; } else ++index; }
return many_removed; }
bool bag::erase_one(const value_type& target) { size_type index; // The location of target in the data array
// First, set index to the location of target in the data array, // which could be as small as 0 or as large as used-1. If target is not // in the array, then index will be set equal to used. index = 0; while ((index < used) && (data[index] != target)) ++index;
if (index == used) return false; // target is in the bag, so no work to do.
// When execution reaches here, target is in the bag at data[index]. // So, reduce used by 1 and copy the last item onto data[index]. --used; data[index] = data[used]; return true; }
void bag::insert(const value_type& entry) // Library facilities used: cassert { assert(size( ) < CAPACITY);
data[used] = entry; ++used; }
void bag::operator +=(const bag& addend) // Library facilities used: algorithm, cassert { assert(size( ) + addend.size( ) <= CAPACITY); copy(addend.data, addend.data + addend.used, data + used); used += addend.used; }
bag::size_type bag::count(const value_type& target) const { size_type answer; size_type i;
answer = 0; for (i = 0; i < used; ++i) if (target == data[i]) ++answer; return answer; }
bag operator +(const bag& b1, const bag& b2) // Library facilities used: cassert { bag answer;
assert(b1.size( ) + b2.size( ) <= bag::CAPACITY);
answer += b1; answer += b2; return answer; } }
bag1.h
// FILE: bag1.h // CLASS PROVIDED: bag (part of the namespace main_savitch_3) // // TYPEDEF and MEMBER CONSTANTS for the bag class: // typedef ____ value_type // bag::value_type is the data type of the items in the bag. It may be any of // the C++ built-in types (int, char, etc.), or a class with a default // constructor, an assignment operator, and operators to // test for equality (x == y) and non-equality (x != y). // // typedef ____ size_type // bag::size_type is the data type of any variable that keeps track of how many items // are in a bag. // // static const size_type CAPACITY = _____ // bag::CAPACITY is the maximum number of items that a bag can hold. // // CONSTRUCTOR for the bag class: // bag( ) // Postcondition: The bag has been initialized as an empty bag. // // MODIFICATION MEMBER FUNCTIONS for the bag class: // size_type erase(const value_type& target); // Postcondition: All copies of target have been removed from the bag. // The return value is the number of copies removed (which could be zero). // // void erase_one(const value_type& target) // Postcondition: If target was in the bag, then one copy has been removed; // otherwise the bag is unchanged. A true return value indicates that one // copy was removed; false indicates that nothing was removed. // // void insert(const value_type& entry) // Precondition: size( ) < CAPACITY. // Postcondition: A new copy of entry has been added to the bag. // // void operator +=(const bag& addend) // Precondition: size( ) + addend.size( ) <= CAPACITY. // Postcondition: Each item in addend has been added to this bag. // // CONSTANT MEMBER FUNCTIONS for the bag class: // size_type size( ) const // Postcondition: The return value is the total number of items in the bag. // // size_type count(const value_type& target) const // Postcondition: The return value is number of times target is in the bag. // // NONMEMBER FUNCTIONS for the bag class: // bag operator +(const bag& b1, const bag& b2) // Precondition: b1.size( ) + b2.size( ) <= bag::CAPACITY. // Postcondition: The bag returned is the union of b1 and b2. // // VALUE SEMANTICS for the bag class: // Assignments and the copy constructor may be used with bag objects.
#ifndef MAIN_SAVITCH_BAG1_H #define MAIN_SAVITCH_BAG1_H #include
namespace main_savitch_3 { class bag { public: // TYPEDEFS and MEMBER CONSTANTS typedef int value_type; typedef std::size_t size_type; static const size_type CAPACITY = 30; // CONSTRUCTOR bag( ) { used = 0; } // MODIFICATION MEMBER FUNCTIONS size_type erase(const value_type& target); bool erase_one(const value_type& target); void insert(const value_type& entry); void operator +=(const bag& addend); // CONSTANT MEMBER FUNCTIONS size_type size( ) const { return used; } size_type count(const value_type& target) const; private: value_type data[CAPACITY]; // The array to store items size_type used; // How much of array is used };
// NONMEMBER FUNCTIONS for the bag class bag operator +(const bag& b1, const bag& b2); }
#endif
bag.cpp
#include
#include "bag1.h"
using namespace std;
int set_operation::search(int a[],int sz,int n)
{
for(int i=0;i { if(a[i]==n) return(1); } return(0); } void set_operation::set_union(int a[],int b[],int acount,int bcount) { int i;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
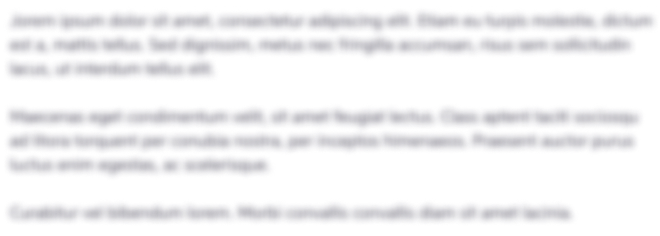
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started