Question
Please, I need this to be done in Java (eclypse), I have provided some classes, but I need to implement the JUnitTest8 class, bellow is
Please, I need this to be done in Java (eclypse), I have provided some classes, but I need to implement the JUnitTest8 class, bellow is the requirement this class. Thank you
It is a game that generates three numbers from 0 to 9 inclusive, the random numbers must be unique. For example, 2 5 7 is valid but 2 5 5 is not valid,
The user guesses three numbers, The application will respond with the words
Nano, Pico, and Fermi in a String array (String[]) .
Fermi = when the number is correct and in the right position,
Pico = when the number is correct but not in the right position,
and Nano = when the number is not a correct number
so if the randon generated number is 1, 2, 3 and the user guesses 1, 3, 8 it would show Fermi, Pico, Nano.
The application response when the method guess is called will be a String array response with all "Fermi" strings in that case. Fermi, Fermi, Fermi
The Fermi string must be the one provided by the user in the constructor or the default value if no override is provided. In the reference implementation these are stored in the instance variables m_fermi, m_nano and m_pico.
If the first number the provided matches the first random number, assign the word representing fermi (m_fermi, default is "Fermi") first in the response.
- If the first number the user entered matches the second or third random numbers, assign the word representing pico (m_pico, default is "Pico") first in the response.
Assign the word representing nano (m_nano, default is "Nano") first in the response if the first number the user entered doesn't match any of the three random numbers.
Use the same logic for guesses at the other two positions. 4. Keep track of whether the user won. The reference implementation uses m_wonGame. This is used by the method isWinner.
The output should be something like this****
Guesses 6, 7, 3
Results: Pico, Nano, Nano
Guesses 1, 6, 2
Results: Nano, Pico, Nano
Guesses 6, 4, 5
Results: Pico, Nano, Nano
Guesses 8, 6, 9
Results: Fermi, Pico, Pico
Guesses 8, 9, 6
Won game*******
*****
class To implement
import java.util.Random;
import java.util.ArrayList;
public class JUnitTest8 {
}
*****************
Provided classes
import java.util.Random;
public class FermiGame
{
public FermiGame()
{
this("Fermi", "Pico", "Nano");
}
public FermiGame(String fermi, String pico, String nano)
{
validateParameters(fermi, pico, nano);
}
public void newGame()
{
m_wonGame = false;
generateRandomIntegers();
}
public String[] guess(int one, int two, int three)
throws InvalidArgumentException
{
String result[] = null;
if(validateGuess(one, two, three))
{
String response1 = testMatch(one, 0);
String response2 = testMatch(two, 1);
String response3 = testMatch(three, 2);
if(response1.endsWith(m_fermi) && response2.endsWith(m_fermi)
&& response3.endsWith(m_fermi))
{
m_wonGame = true;
}
result = new String[] { response1, response2, response3 };
}
return result;
}
public boolean isWinner()
{
return m_wonGame;
}
private boolean validateGuess(int one, int two, int three)
throws InvalidArgumentException
{
boolean result1 = true;
boolean result2 = true;
boolean result3 = true;
String msg = "";
if(!(MIN < one && one < MAX))
{
result1 = false;
msg = String.format("Pos %d, Value: %d", 1, one);
}
if(!(MIN < two && two < MAX))
{
result2 = false;
msg += String.format(" Pos %d, Value: %d", 2, two);
}
if(!(MIN < three && three < MAX))
{
result3 = false;
msg += String.format(" Pos %d, Value: %d", 3, three);
}
if(!(result1 && result2 && result3))
{
throw new InvalidArgumentException("Invalid Guess: " + msg);
}
return true;
}
private void validateParameters(String fermi, String pico, String nano)
{
m_fermi = fermi != null ? fermi : FERMI;
m_pico = pico != null ? pico : PICO;
m_nano = nano != null ? nano : NANO;
if(m_fermi == m_pico)
{
m_pico = PICO;
}
if(m_fermi == m_nano || m_pico == m_nano)
{
m_nano = NANO;
}
}
private String testMatch(int guess, int pos)
{
String response = m_nano;
if(guess == m_random[pos])
{
response = m_fermi;
}
else
{
for(int i = 0; i < m_random.length; i++)
{
if(guess == m_random[i] && i != pos)
{
response = m_pico;
break;
}
}
}
return response;
}
private void generateRandomIntegers()
{
int rand1;
int rand2;
int rand3;
do
{
Random m_Random = new Random();
rand1 = m_Random.nextInt(9) + 1;
rand2 = m_Random.nextInt(9) + 1;
rand3 = m_Random.nextInt(9) + 1;
}
while(rand1 == rand2 || rand1 == rand3 || rand2 == rand3);
m_random = new int[] { rand1, rand2, rand3 };
}
private String m_fermi = FERMI;
private String m_pico = PICO;
private String m_nano = NANO;
private boolean m_wonGame = false;
private int[] m_random;
private static String FERMI = "Fermi";
private static String PICO = "Pico";
private static String NANO = "Nano";
private static int MIN = 0;
private static int MAX = 10;
}
public class InvalidArgumentException extends Exception
{
private static final long serialVersionUID = -4242998573088432171L;
public InvalidArgumentException()
{
}
public InvalidArgumentException(String arg0)
{
super(arg0);
}
public InvalidArgumentException(Throwable arg0)
{
super(arg0);
}
public InvalidArgumentException(String arg0, Throwable arg1)
{
super(arg0, arg1);
}
public InvalidArgumentException(int invalidValue)
{
badValue = invalidValue;
}
@Override
public String getMessage()
{
return String.format("Bad value: %d", badValue);
}
int badValue;
}
*************************************
import java.util.List;
import org.junit.runner.Result;
import org.junit.runner.notification.Failure;
public class TestHarness
{
public static void main(String[] args)
{
trace("TestHarness");
try
{
Result result = org.junit.runner.JUnitCore
.runClasses(JUnitTest8.class);
int runs = result.getRunCount();
int ignores = result.getIgnoreCount();
trace(String.format("Runs: %d", runs));
trace(String.format("Ingores: %d", ignores));
int failCount = result.getFailureCount();
if(failCount > 0)
{
List
for(Failure fail : failures)
{
trace("FAILED: " + fail.getMessage());
}
}
else
{
trace("SUCCESS");
}
}
catch(Exception ex)
{
trace("Unhandled exception: " + ex.getMessage());
}
}
private static void trace(String msg)
{
System.out.println(msg);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
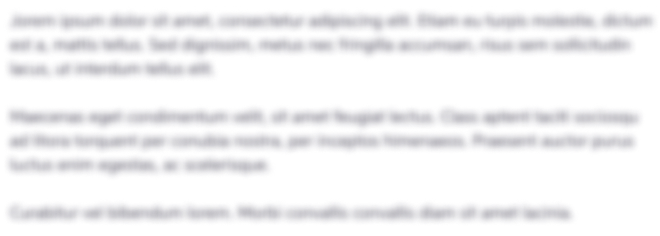
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started