Question
Please just fix the errors and make it match with the expected output. The point of this program Create and test a class called Points2.
Please just fix the errors and make it match with the expected output.
The point of this program
Create and test a class called Points2. This class describes a sequence of 2D points. For example (1, 3), (4, 5) is a sequence of two points, where each coordinate is an integer. (1.2, 3.4), (5.6, 10.1), (11.1, 12.0) is a sequence of three points where each coordinate is a double. A sequence can have any size. An empty sequence has size 0
Below I have also included the expected output.
But for some reason, the output that my code produces is not the same as the expected code I have provided.
The copy assignment does not seem to work, the move assignment and the operator overloading + are only a few points that do not work.
Especially plz make the overloading operating + work and produce the expected output. Otherwise, my code fully works.
Please let me know what the mistakes of my code are! Thank you
#ifndef CSCI335_HOMEWORK1_POINTS2_H_
#define CSCI335_HOMEWORK1_POINTS2_H_
#include
#include
#include
#include
#include
namespace teaching_project {
// Place comments that provide a brief explanation of the class,
// and its sample usage.
template
class Points2 {
public:
// Default "big five" -- you have to alter them for your assignment.
// That means that you will remove the "= default" statement.
// and you will provide an implementation.
// Zero-parameter constructor.
// Set size to 0.
Points2():size_{0}, sequence_{nullptr} {
}
// Copy-constructor.
Points2(const Points2 &rhs){
size_ = rhs.size_; //copies over the size
//this->sequence_ = new std::array
sequence_= new std::array
for (size_t i=0; i this->sequence_[i] = rhs.sequence_[i]; } // sequence_= new std::array } // Copy-assignment. If you have already written // the copy-constructor and the move-constructor // you can just use: // { // Points2 copy = rhs; // std::swap(*this, copy); // return *this; // } /* Points2& operator=(const Points2 &rhs) { { Points2 copy = rhs; std::swap(*this, copy); return *this; } */ Points2& operator=(const Points2 &rhs) { if (this != &rhs){ *sequence_ = *rhs.sequence_; } return *this; } // Move-constructor. Points2(Points2 &&rhs){ sequence_=rhs.sequence_; size_=rhs.size_; *this = std::move(rhs); rhs.sequence_= nullptr; rhs.size_=0; } // Move-assignment. // Just use std::swap() for all variables. Points2& operator=(Points2 &&rhs) { /* *this = std::move(rhs); return *this;*/ std::swap(this->sequence_, rhs.sequence_); std::swap(this->size_, rhs.size_); return *this; } ~Points2() { delete []sequence_; } // End of big-five. // One parameter constructor. Points2(const std::array // Provide code. sequence_ = new std::array sequence_[0][0] = item[0]; sequence_[0][1] = item[1]; //std::copy(item.begin(), item.end(), sequence_); } // Read a chain from standard input. void ReadPoints2() { // Part of code included (without error checking). std::string input_line; std::getline(std::cin, input_line); std::stringstream input_stream(input_line); if (input_line.empty()) return; // Read size of sequence (an integer). int size_of_sequence; input_stream >> size_of_sequence; // std::cout << "Size: " << size_of_sequence; // Allocate space for sequence. // Add code here. sequence_ = new std::array size_ = size_of_sequence; // Object token; for (int i = 0 ; i < size_of_sequence; ++i) { // for (int i = 0 ;input_stream >> token; ++i) { Object x, y; input_stream >> x >> y; //std::cout << "Reading " << x << ", " << y << std::endl; //array //std::cout<<"("; sequence_[i][0] = x; //.fill(x); sequence_[i][1] = y; // fill(y); //std::cout<<")"; // } } /*for (int i = 0; i < size_; ++i){ std::cout << "("<< sequence_[i][0] << " " << sequence_[i][1] << ")" << std::endl; }*/ } size_t size() const { return size_; } // @location: an index to a location in the sequence. // @returns the point at @location. // const version. // abort() if out-of-range. const std::array if( location > size_ ) { abort(); } return sequence_[location]; } // Code missing. // @c1: A sequence. // @c2: A second sequence. // @return their sum. If the sequences are not of the same size, append the // result with the remaining part of the larger sequence. /* friend Points2 operator+(const Points2 &c1, const Points2 &c2) { Points2 //Points2 size_t c1_size = c1.size_; size_t c2_size = c2.size_; size_t min_size = c1_size < c2_size ? c1_size : c2_size; x.size_ = c1_size > c2_size ? c1_size : c2_size; std::size_t i; if (c1_size == c2_size) for (i = 0; i != min_size; ++i) x.sequence_[i][0] = c1.sequence_[i][0] + c2.sequence_[i][0]; //return x; if (c1_size > min_size) for (; i != c1_size; ++i) x.sequence_[i][0] = c1.sequence_[i][0]; if (c2_size > min_size) for (; i != c2_size; ++i) x.sequence_[i][0] = c2.sequence_[i][0]; return x; }*/ friend Points2 operator+(const Points2 &c1, const Points2 &c2) { Points2 //Points2 size_t c1_size = c1.size_; size_t c2_size = c2.size_; size_t min_size = c1_size < c2_size ? c1_size : c2_size; x.size_ = c1_size > c2_size ? c1_size : c2_size; std::size_t i; if (c1_size == c2_size) for (i = 0; i != min_size; ++i) x.sequence_[i][0] = c1.sequence_[i][0] + c2.sequence_[i][0]; //return x; if (c1_size > min_size) for (; i != c1_size; ++i) x.sequence_[i][0] = c1.sequence_[i][0]; if (c2_size > min_size) for (; i != c2_size; ++i) x.sequence_[i][0] = c2.sequence_[i][0]; return x.sequence_; } // Overloading the << operator friend std::ostream &operator<<(std::ostream &out, const Points2 &some_points2) { for(size_t i = 0; i < some_points2.size_; i++) //out << some_points2.sequence_[i][0] << ", " << some_points2.sequence_[i][1] << std::endl; out << "("< return out; } private: // Sequence of points. std::array // Size of sequence. size_t size_; }; } // namespace teaching_project #endif // CSCI_335_HOMEWORK1_POINTS2_H_ The following program is the main program to run the class on. // Do not change this file other than adding header files // if needed. using namespace std; using namespace teaching_project; // Place stand-alone function in unnamed namespace. namespace { void TestPart1() { Points2 a, b; // Two empty Points2 are created. cout << a.size() << " " << b.size() << endl; // yields 0 0. const array a_point2{{7, 10}}; Points2 d{a_point2}; // A Points2 containing (7, 10) should be created. cout << d; // Should just print (7, 10). cout << "Enter a sequence of points (integer)" << endl; a.ReadPoints2(); // User enters a set of points in the form: // 3 7 4 3 2 1 10 // 3 specifies number of points. Points are the pairs // (7, 4) (3, 2) and (1, 10). cout << "Output1: " << endl; cout << a; // Output should be what user entered. cout << "Enter a sequence of points (integer)" << endl; b.ReadPoints2(); // Enter another sequence. cout << "Output2: " << endl; cout << b; Points2 c{a}; // Calls copy constructor for c. cout << "After copy constructor1 c{a}: " << endl; cout << c; cout << a; a = b; // Should call the copy assignment operator for a. cout << "After assignment a = b" << endl; cout << a; Points2 e = move(c); // Move constructor for d. cout << "After e = move(c) " << endl; cout << e; cout << c; cout << "After a = move(e) " << endl; a = move(e); // Move assignment operator for a. cout << a; cout << e; } void TestPart2() { Points2 a, b; cout << "Enter a sequence of points (double)" << endl; a.ReadPoints2(); // User provides input for Points2 a. cout << a; cout << "Enter a sequence of points (double)" << endl; b.ReadPoints2(); // User provides input for Points2 b. cout << b << endl; cout << "Result of a + b" << endl; cout << a + b << endl; Points2 d = a + b; cout << "Result of d = a + b" << endl; cout << d; cout << "Second element in a: " << endl; cout << a[1][0] << ", " << a[1][1] << endl; // Should print the 2nd element. } } // namespace int main(int argc, char **argv) { TestPart1(); TestPart2(); return 0; } And the text file of points I read in from standard input 3 7 4 3 5 19 71 4 100 101 2 3 40 50 11 33 3 2.1 20.3 11.11 12.45 13.1 14.2 2 1.1 100.0 20.1 30.2 The expected output should be 0 0 (7, 10) Enter a sequence of points (integer) Output1: (7, 4) (3, 5) (19, 71) Enter a sequence of points (integer) Output2: (100, 101) (2, 3) (40, 50) (11, 33) After copy constructor1 c{a}: (7, 4) (3, 5) (19, 71) (7, 4) (3, 5) (19, 71) After assignment a = b (100, 101) (2, 3) (40, 50) (11, 33) After e = move(c) (7, 4) (3, 5) (19, 71) () After a = move(e) (7, 4) (3, 5) (19, 71) (100, 101) (2, 3) (40, 50) (11, 33) Enter a sequence of points (double) (2.1, 20.3) (11.11, 12.45) (13.1, 14.2) Enter a sequence of points (double) (1.1, 100) (20.1, 30.2) Result of a + b (3.2, 120.3) (31.21, 42.65) (13.1, 14.2) Result of d = a + b (3.2, 120.3) (31.21, 42.65) (13.1, 14.2) Second element in a: 11.11, 12.45
Step by Step Solution
There are 3 Steps involved in it
Step: 1
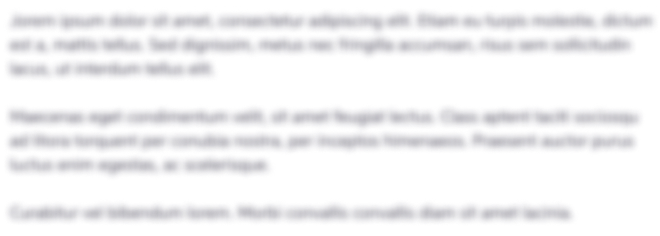
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started