Question
PLEASE MAKE THE 3 DIAGRAMS BELOW(take as much time needed): 1. 2. 3. BUILDING.JAVA package itec3030.assignments.a1; import itec3030.smarthome.standards.*; import java.util.ArrayList; public class Building {
PLEASE MAKE THE 3 DIAGRAMS BELOW(take as much time needed):
1.

2.
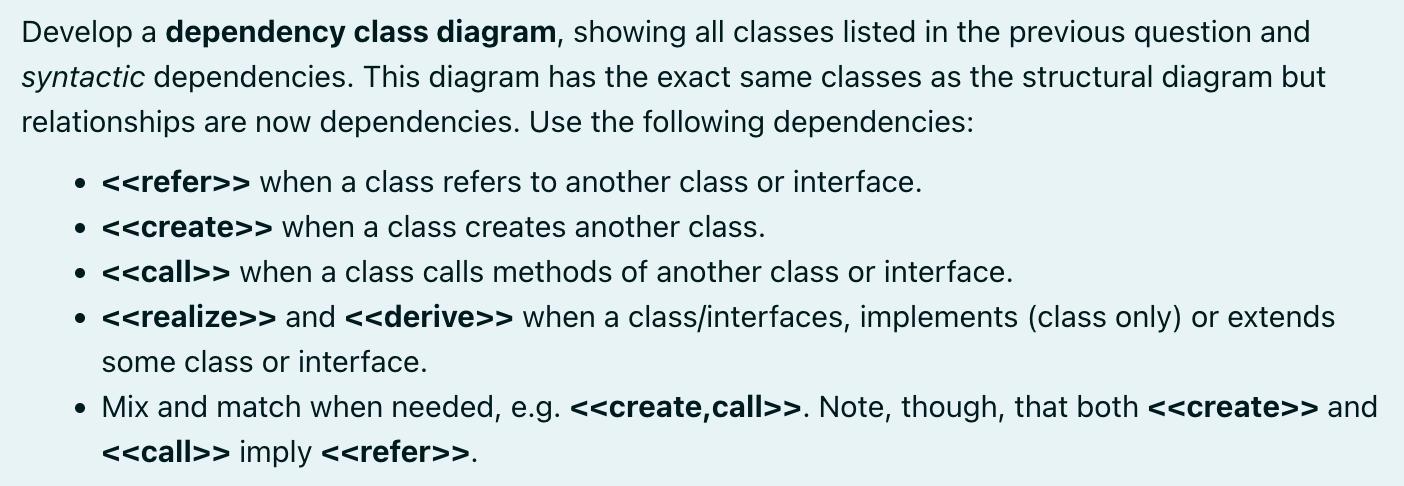
3.
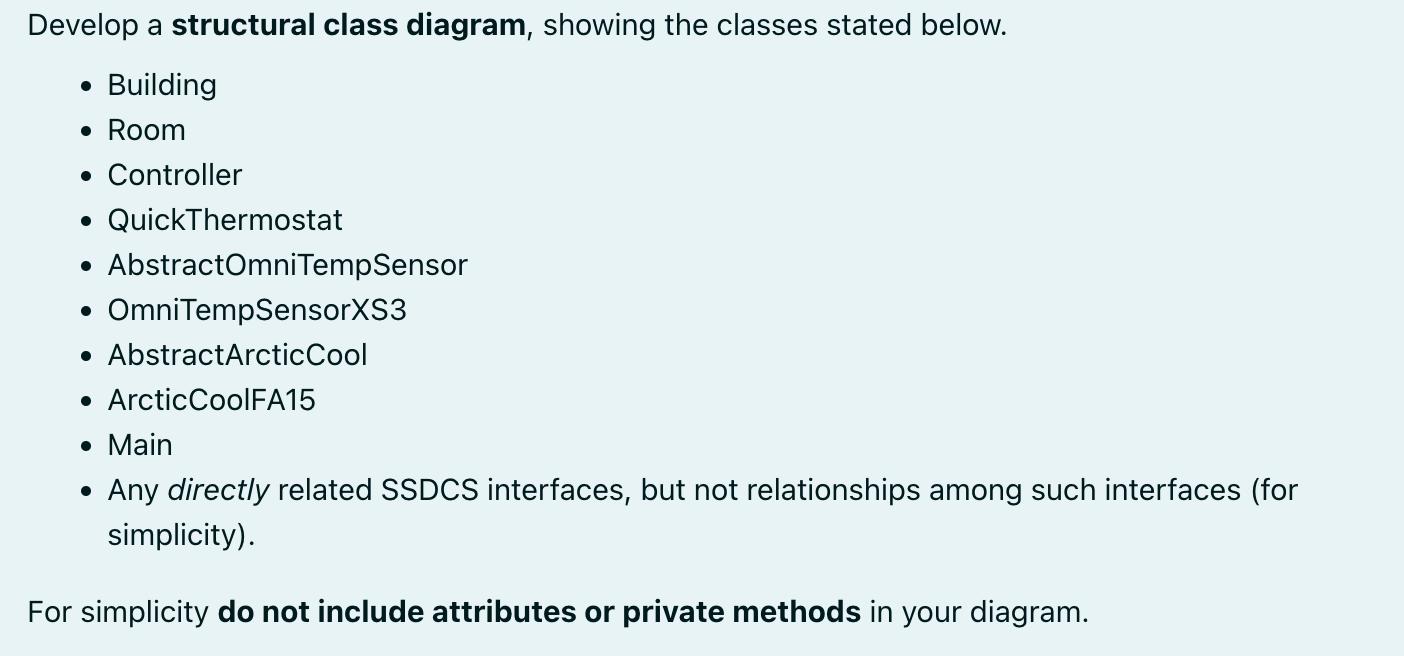
BUILDING.JAVA
package itec3030.assignments.a1;
import itec3030.smarthome.standards.*;
import java.util.ArrayList;
public class Building {
private ArrayList
private ICooler ac = null;
private ITemperaturePreferenceSensor thermostat = null;
public int getDesiredTemperature() {
return thermostat.getReading();
}
public ICooler getCoolingDevice() {
return ac;
}
public void setCoolingDevice(ICooler clr){
this.ac = clr;
}
public ITemperaturePreferenceSensor getThermostat() {
return thermostat;
}
public void setThermostat(ITemperaturePreferenceSensor sth){
this.thermostat = sth;
}
public float getAvergeTemperature(){
float count = 0, sum = 0;
for (Room r : rooms){
sum += r.getAvergeTemperature();
count++;
}
return(float) (sum/count);
}
public Room findRoomOf(ISmartObject s){
Room found = null;
for (Room r : rooms){
if (r.hasThing((ISmartObject) s))
found = r;
}
return(found);
}
//
// Install Constituent Rooms
//
public void add(Room room){
rooms.add(room);
}
}
ROOM.JAVA
package itec3030.assignments.a1;
import java.util.ArrayList;
import itec3030.smarthome.standards.*;
public class Room {
private String name = "default";
private ArrayList
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
//
// ROOM SETUP
//
public void install(ITemperatureSensor t){
tempSensors.add(t);
}
//
// OFFER ROOM INFORMATION
//
public float getAvergeTemperature(){
float count = 0;
float sum = 0;
for (ITemperatureSensor s : tempSensors){
sum += s.getReading();
count++;
}
return(float) (sum/count);
}
public boolean hasThing(ISmartObject s) {
boolean found = false;
for (ITemperatureSensor t : tempSensors) {
if (t.equals(s)) found = true;
}
// Collection of other types can be added here.
return (found);
}
}
CONTROLLER.JAVA
package itec3030.assignments.a1;
import itec3030.smarthome.standards.*;
/**
*
* @author Sotirios Liaskos
*/
public class Controller implements IController {
/**
* Reference to a building model.
*/
protected Building house = new Building();
/**
* Set the building model which the Controller controls
* @param bld
*/
public void setBuildingModel(Building bld){
house = bld;
}
/**
* (See SSDCS documentation)
*/
@Override
public void changeDetected(ISmartObject s) {
this.update();
}
private void update() {
// See if you need to turn on heating
if (house.getAvergeTemperature() > house.getDesiredTemperature() && !house.getCoolingDevice().isOn()) {
System.out.println("Average house temperature is now: " + house.getAvergeTemperature() + " while desired is " + house.getDesiredTemperature() + ". Turning A/C on.");
turnCoolingOn();
}
// See if you need to turn heating off
if (house.getAvergeTemperature() <= house.getDesiredTemperature() && house.getCoolingDevice().isOn()) {
System.out.println("Average house temperature is now: " + house.getAvergeTemperature() + " while desired is " + house.getDesiredTemperature() + ". Turning A/C off.");
turnCoolingOff();
}
}
private void turnCoolingOff(){
house.getCoolingDevice().turnOff();
}
private void turnCoolingOn(){
house.getCoolingDevice().turnOn();
}
}
QUICKTHERMOSTAT.JAVA
package itec3030.assignments.a1;
import itec3030.smarthome.standards.*;
public class QuickThermostat implements INumericInstrument,ITemperaturePreferenceSensor {
private int reading;
@Override
public int getReading() {
return reading;
}
@Override
public IController getController() {
// No controller needed
return null;
}
@Override
public void setController(IController arg0) {
// No need to set a controller
}
@Override
public void newPreferredTemperature(int reading) {
this.reading = reading;
}
}
MAIN.JAVA
package itec3030.assignments.a1;
import itec3030.smarthome.standards.*;
import itec3030.assignments.a1.actuators.arcticcool.ArcticCoolFA15;
import itec3030.assignments.a1.sensors.omnitemp.OmniTempSensorXS3;
/**
*
* @author Sotirios Liaskos
*/
public class Main {
Main p = new Main();
public static void main(String[] args) {
Controller c = new Controller();
//Create Building
Building h = new Building();
Room livingRoom = new Room();
Room bedRoom = new Room();
livingRoom.setName("Living Room");
bedRoom.setName("Bedoom");
h.add(livingRoom);
h.add(bedRoom);
//Create the sensors
ITemperatureSensor o1 = new OmniTempSensorXS3(c,"o1");
ITemperatureSensor o2 = new OmniTempSensorXS3(c,"o2");
ITemperatureSensor o3 = new OmniTempSensorXS3(c,"o3");
ICooler ac = new ArcticCoolFA15("ac");
ITemperaturePreferenceSensor th = new QuickThermostat();
//"Install the sensors in the rooms
livingRoom.install(o1);
livingRoom.install(o2);
bedRoom.install(o3);
//Thermostat and AC go in the building not individual rooms
h.setCoolingDevice(ac);
h.setThermostat(th);
//The controller needs access to the building model (to acquire temperature info etc.)
c.setBuildingModel(h);
//Scenario starts with the A/C running.
ac.powerOn();
//The Scenario object simulates the sensing hardware and sends a number of values to the sensor drivers o1 - o3
// and the thermostat (how? - look at the SSDCS spec!) according to a random pattern (which you
// are not supposed to reverse engineer!).
Scenario e = new Scenario(o1,o2,o3,th);
e.play();
ac.powerOff();
}
}
ABSTRACTOMNIREMPSENSOR
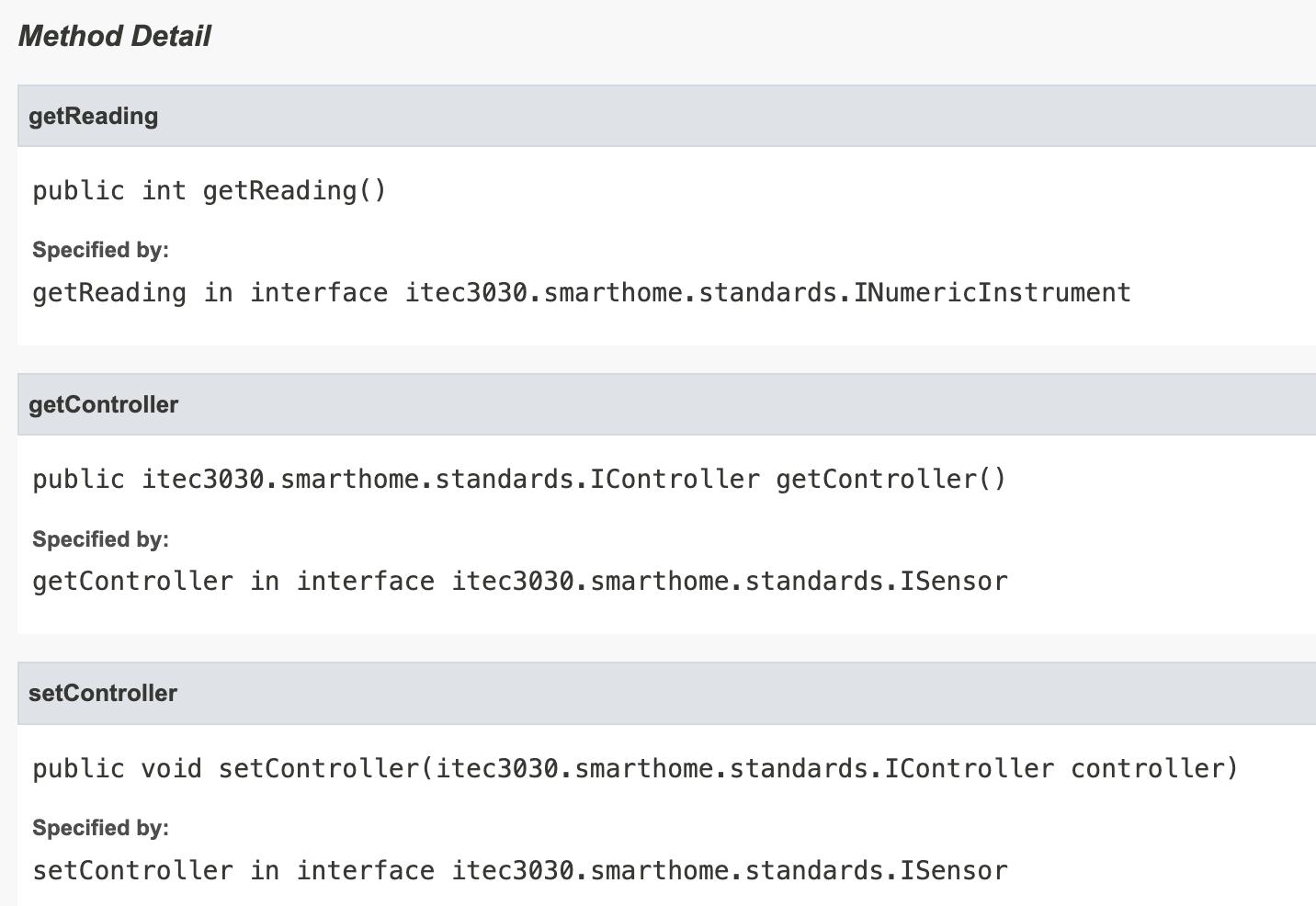
OMNITEMPSENSORXS3
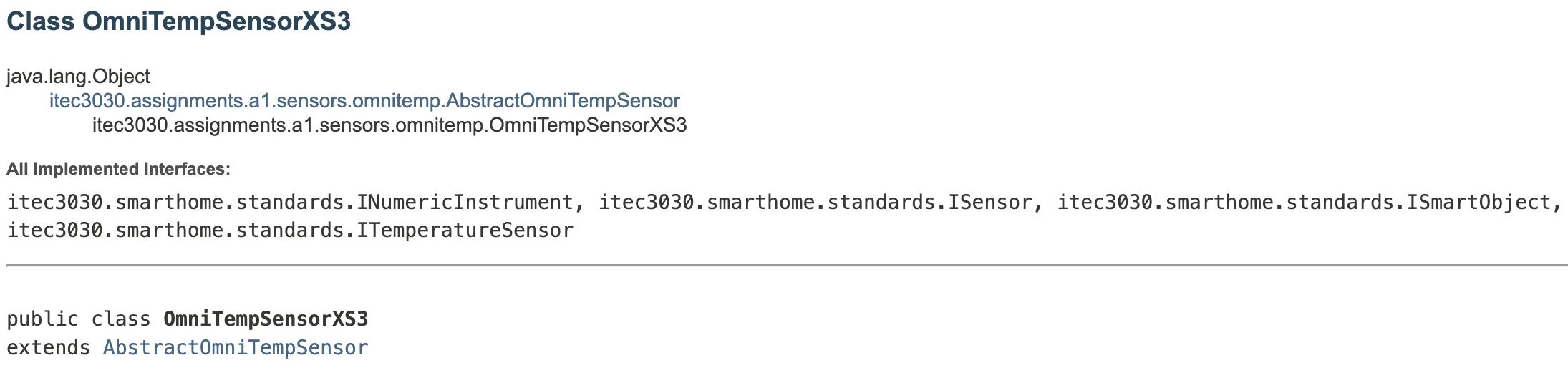
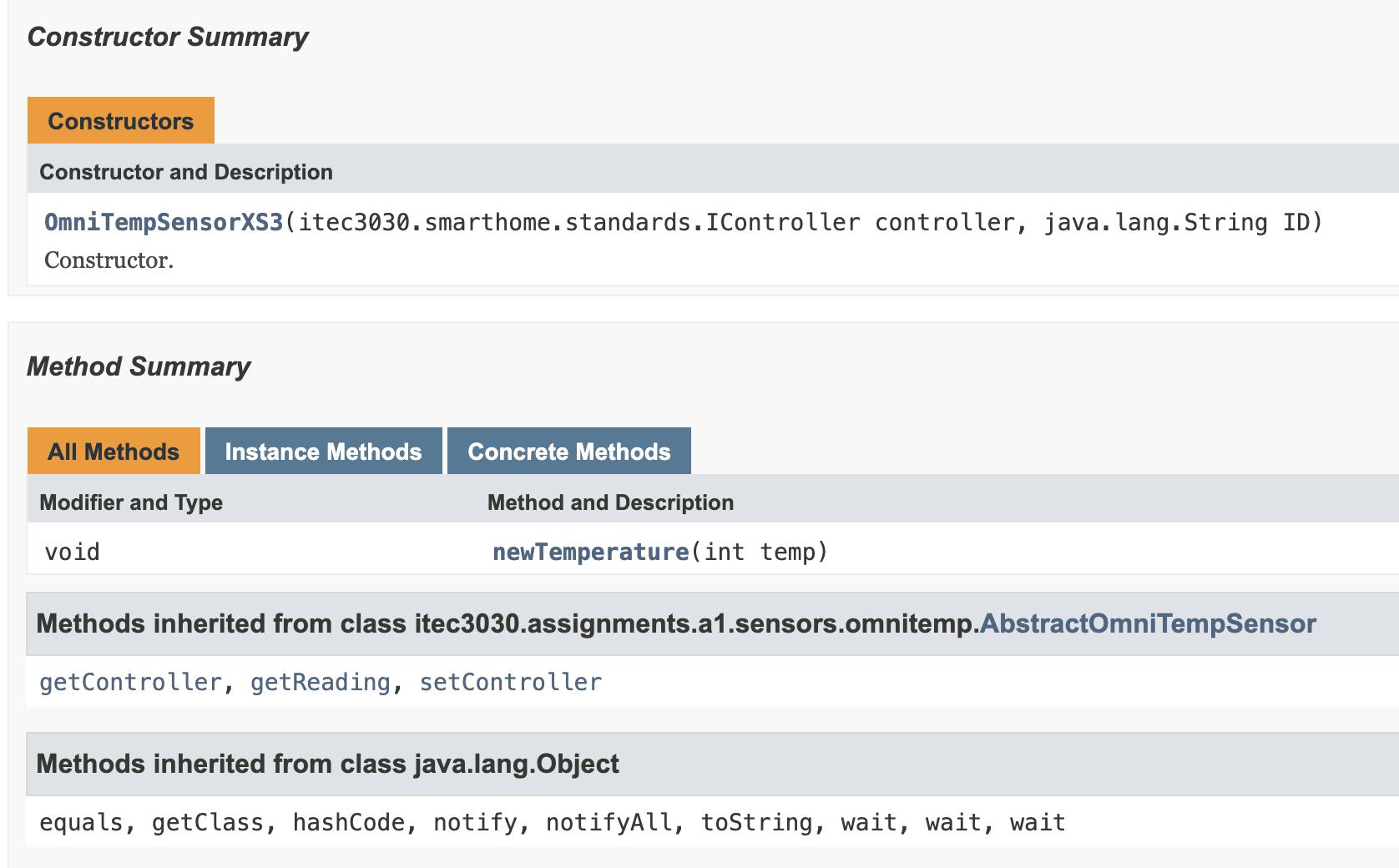
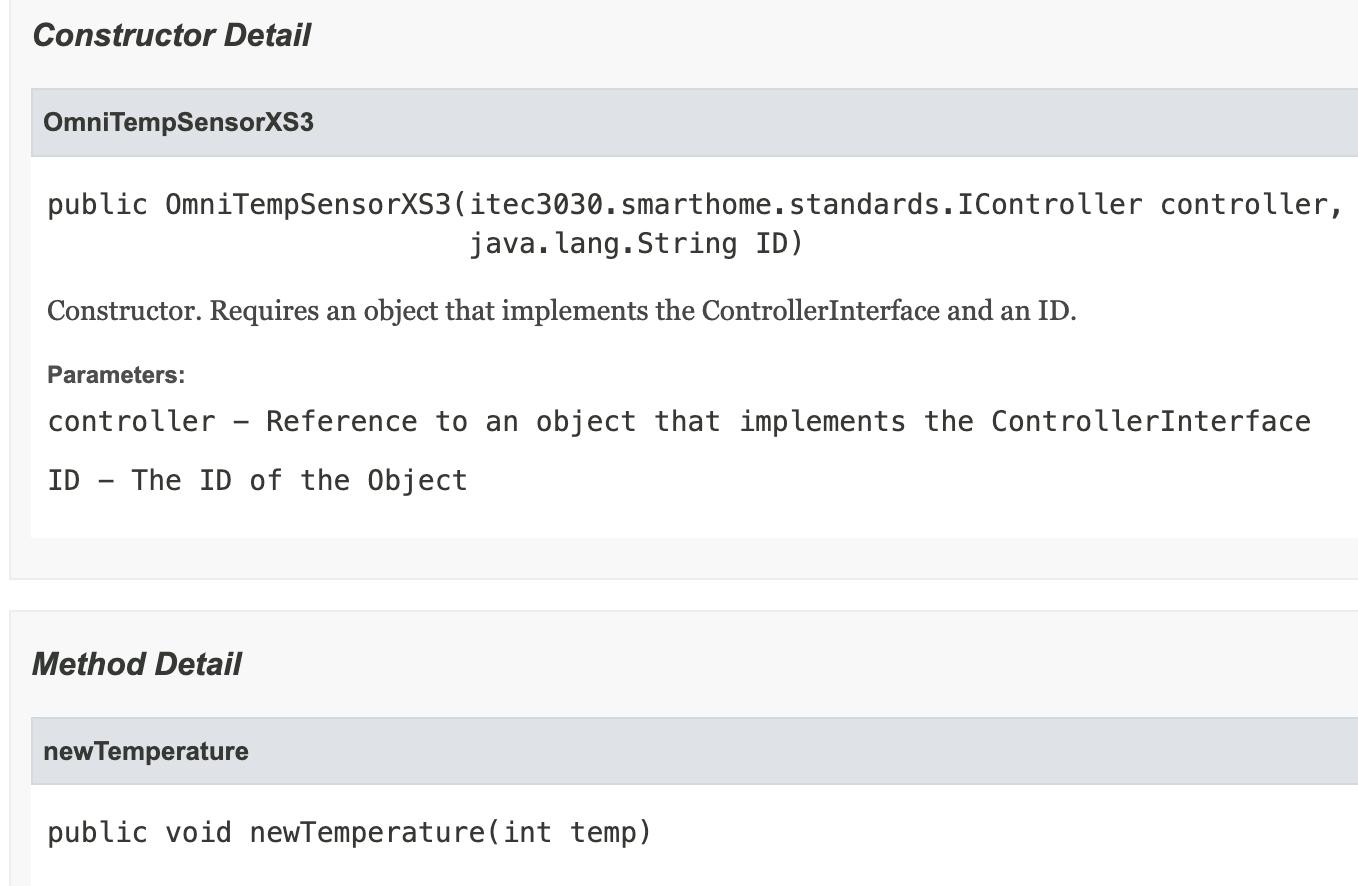
ABSTRACTARCTICCOOL
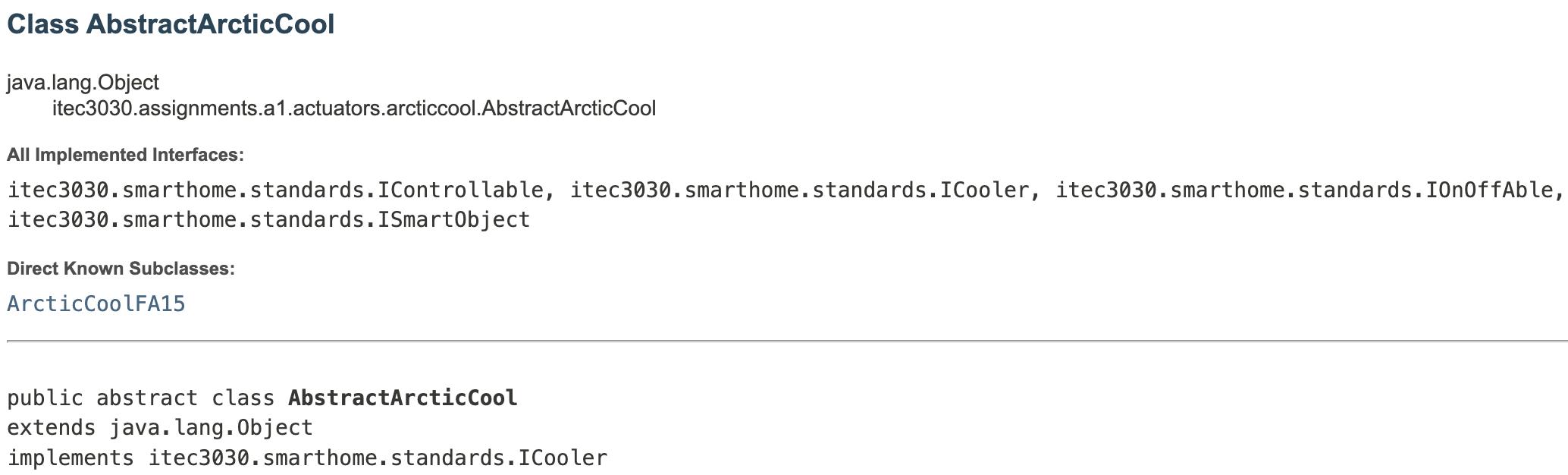
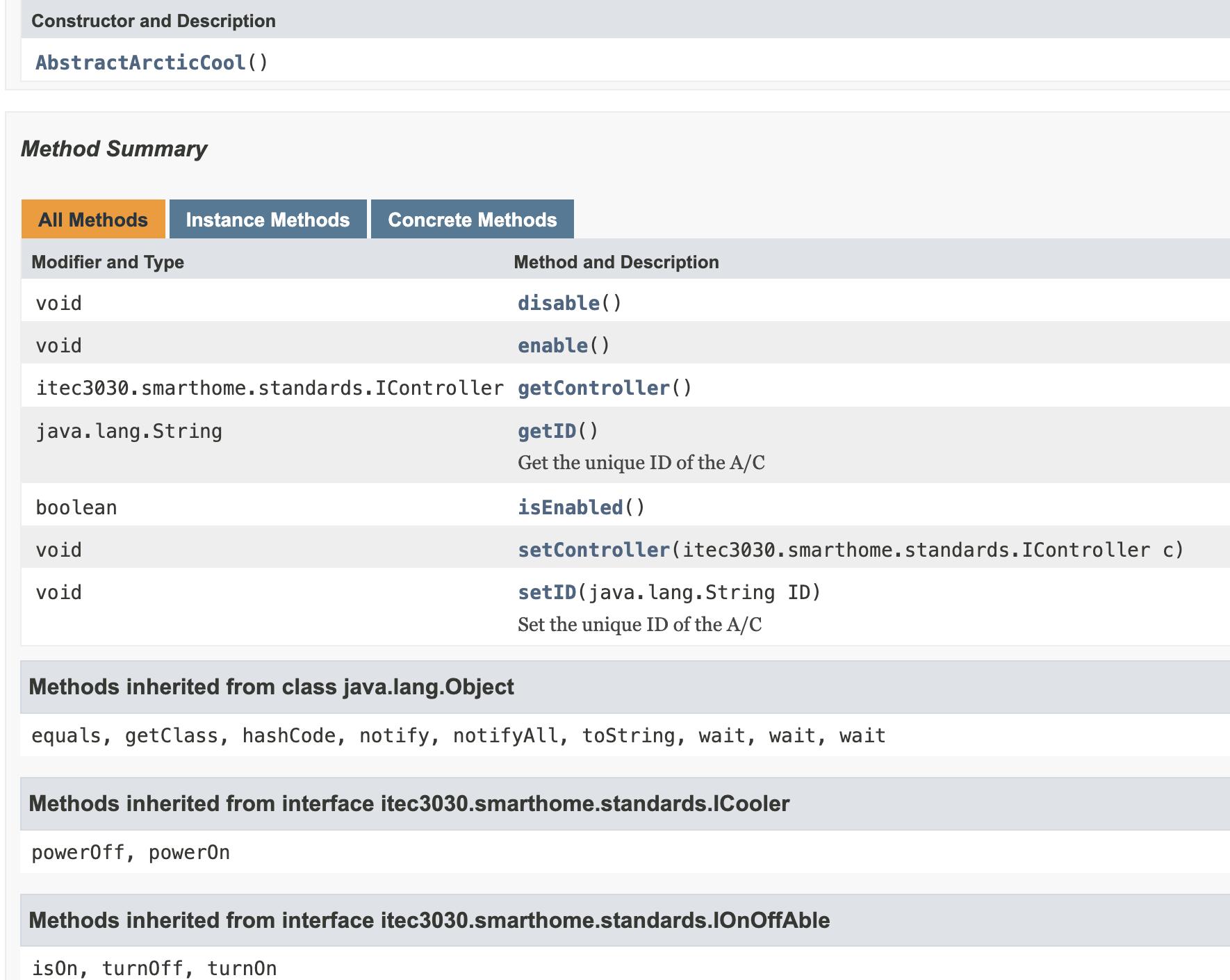
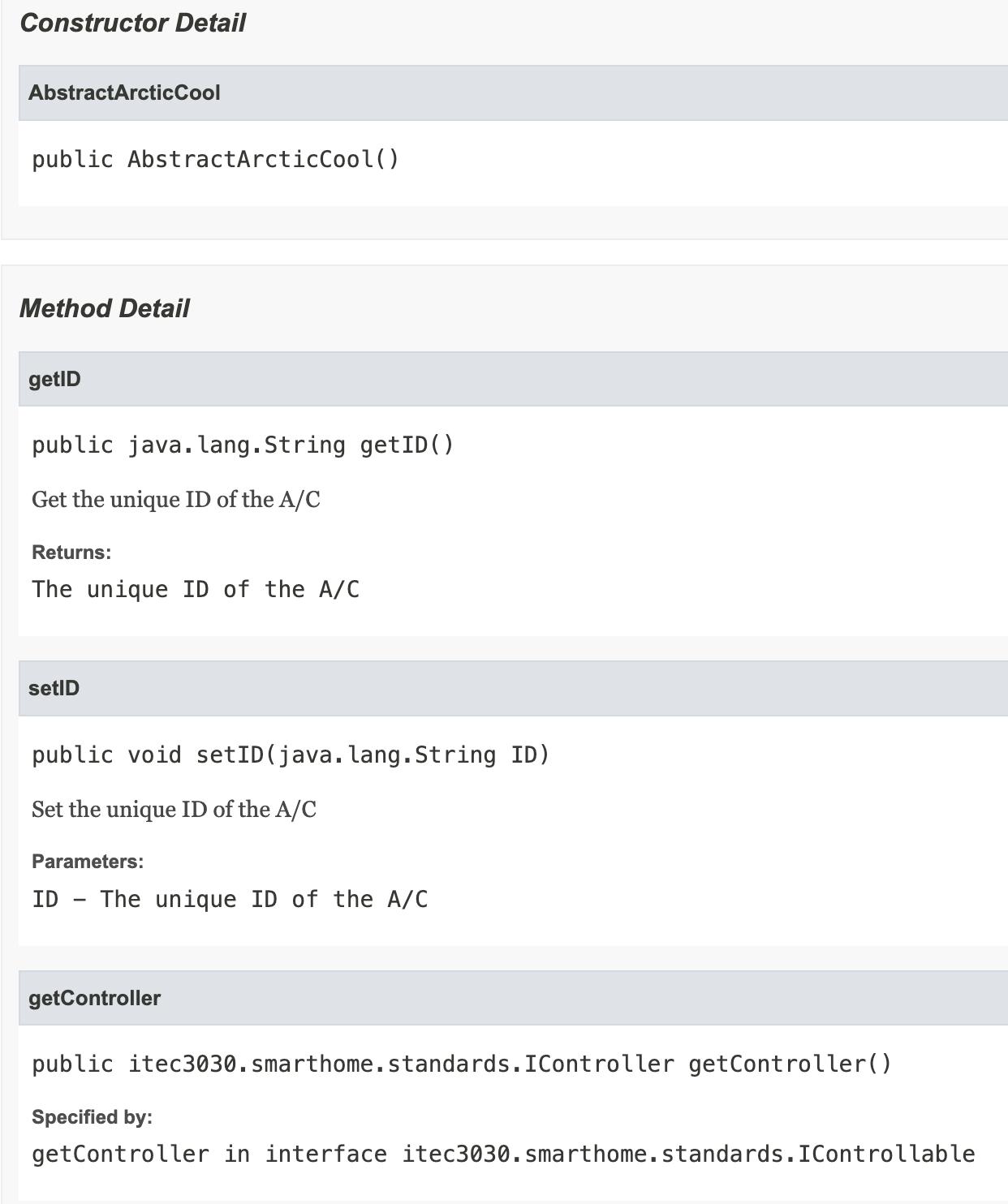
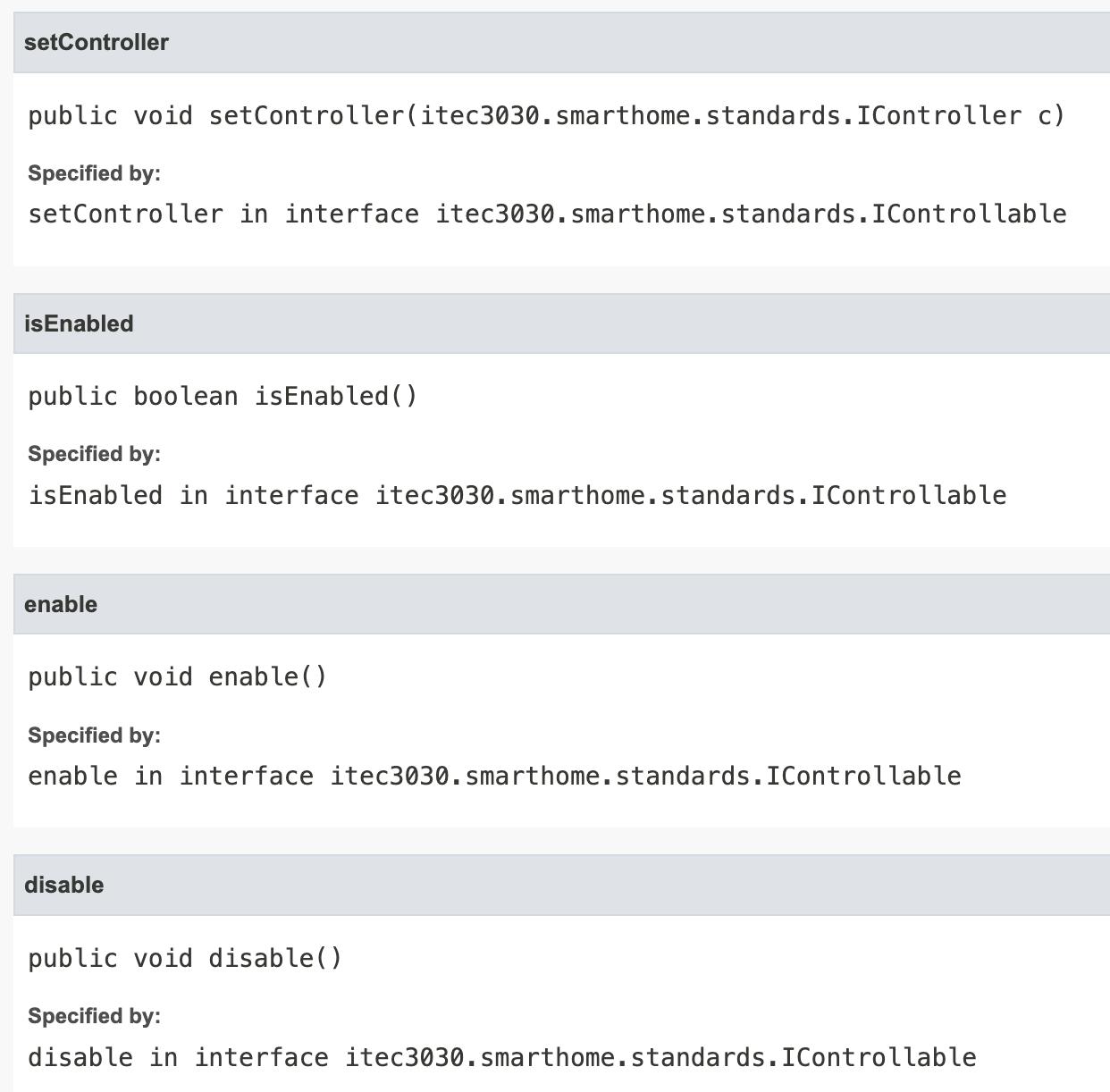
ARCTICCOOLFA15
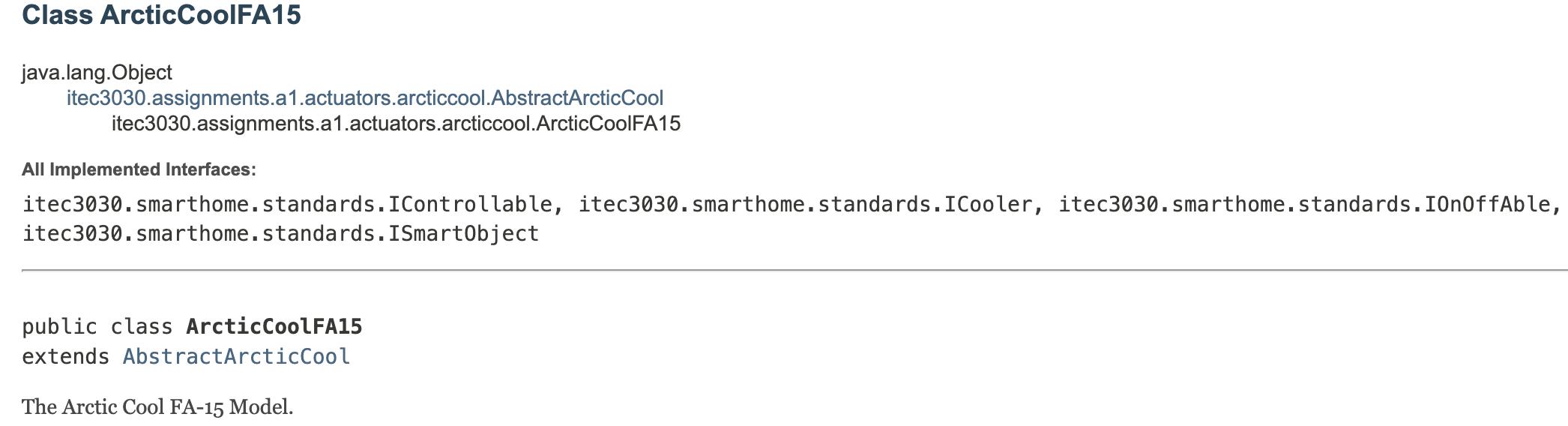
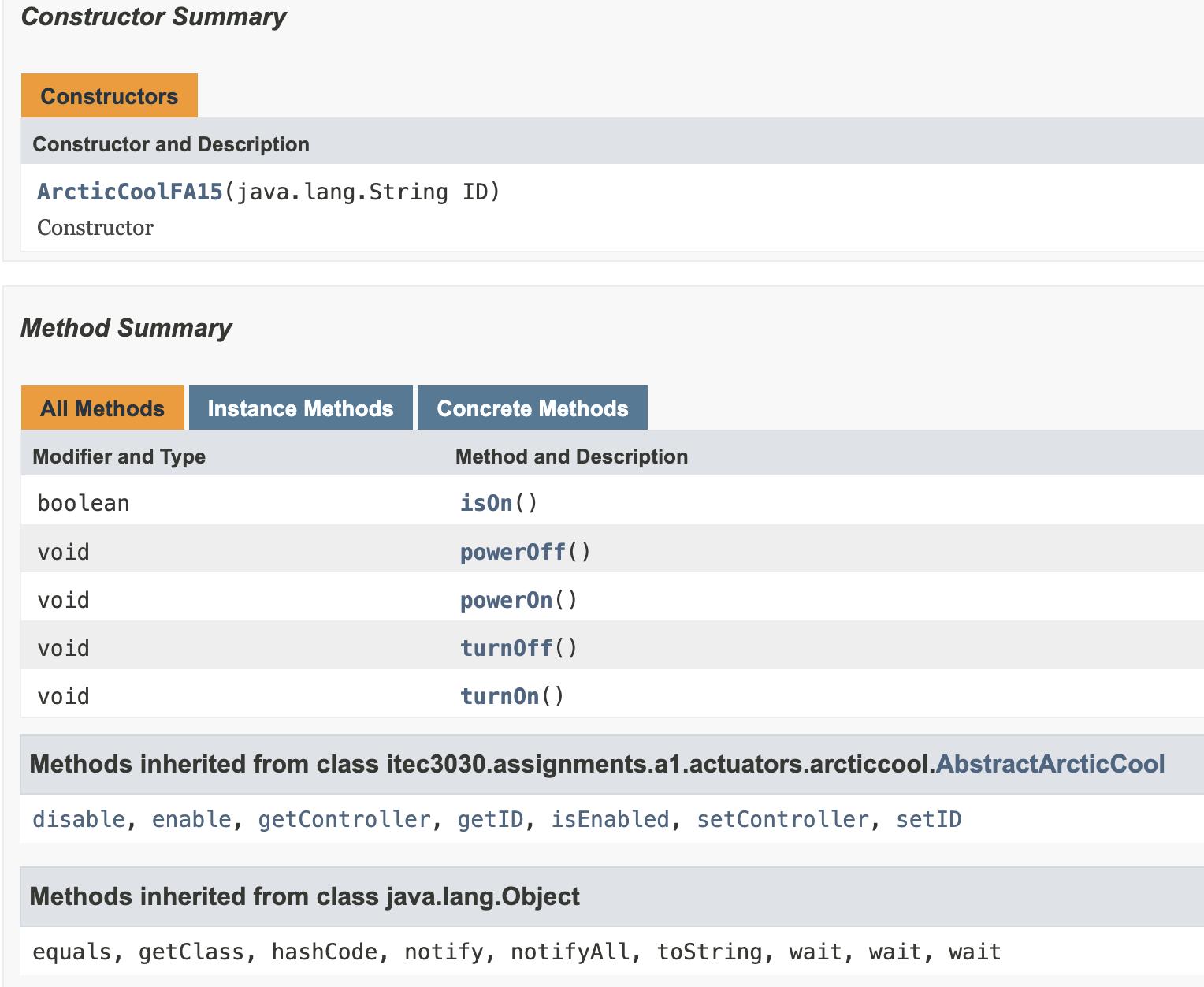
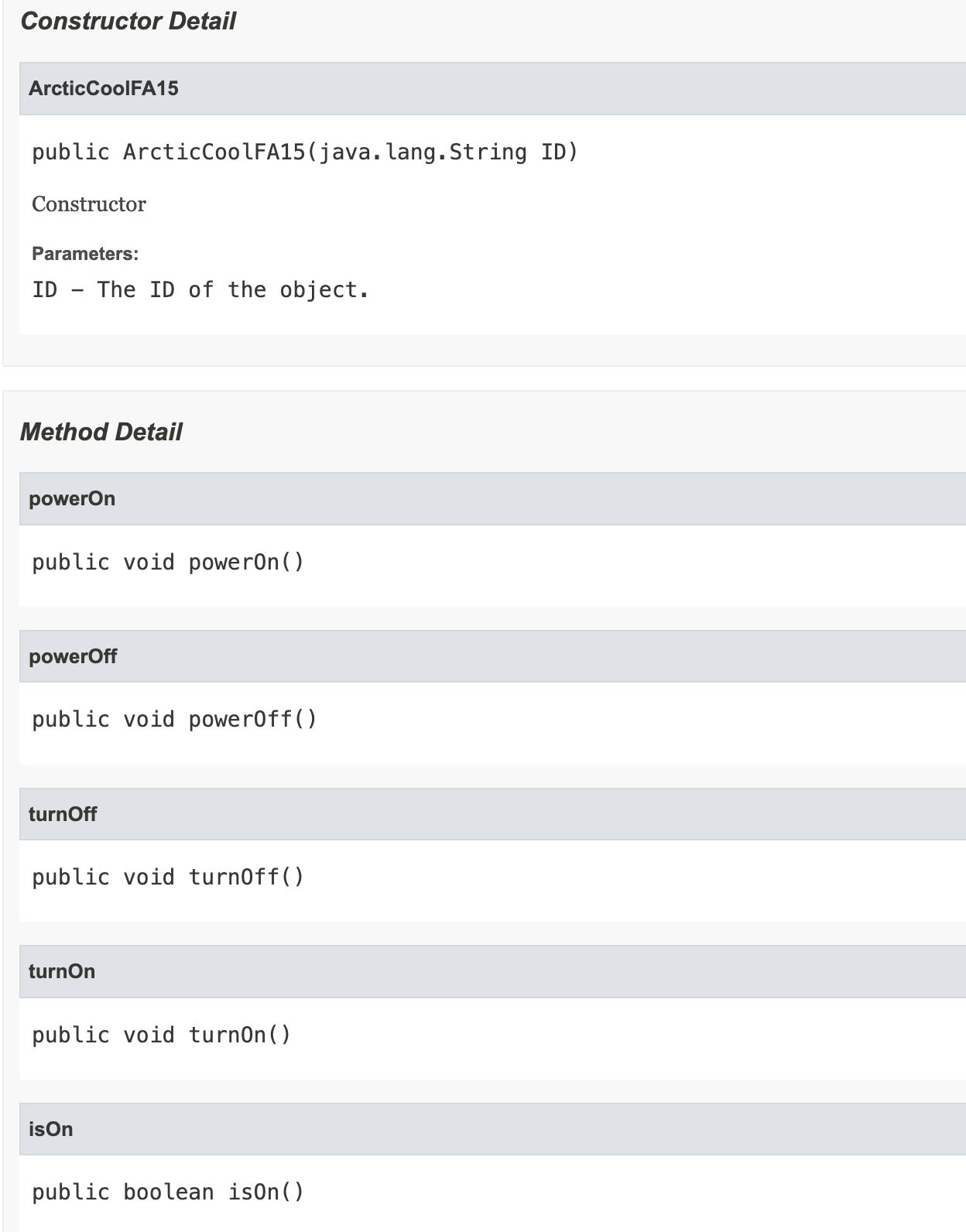
ASSIGNMENT OVERVIEW:
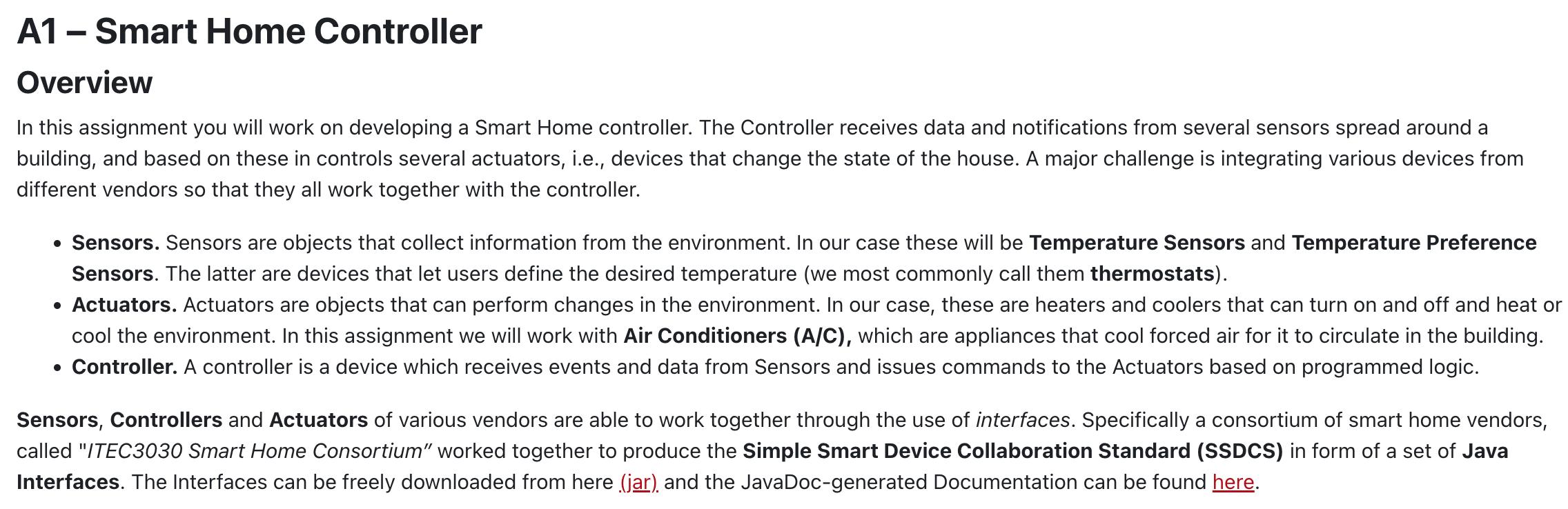
Develop a sequence diagram, describing how all pertinent classes (including those of the vendors) collaborate in a scenario in which the temperature has changed in a way that the a/c needs to be turned on. For simplicity, please consider only the most concrete classes in the collaboration (not abstract classes or interfaces).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
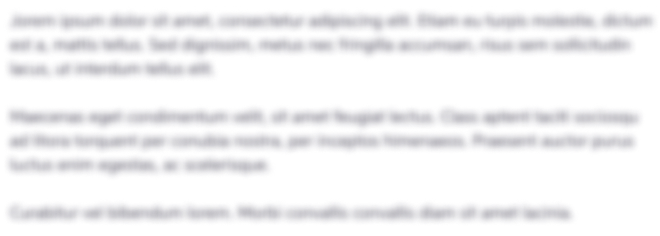
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started