Question
Please note , you are required to include the following when you use g++ to compile: -pedantic For example: g++ -pedantic filename.cpp This will give
Please note, you are required to include the following when you use g++ to compile: -pedantic
For example:
g++ -pedantic filename.cpp
This will give a warning if you attempt to use any non-standard feature in g++. You must correct your code so that you do not receive any warnings of this type.
- Log on to the Linux server.
- Create four files:
- Rational.h
- Rational.cpp
- RationalMain.cpp
- Makefile
- In Rational.h:
#ifndef _RATIONAL_H_
#define _RATIONAL_H_
#include
using namespace std;
class Rational
{
int _p;
int _q;
public:
Rational();
Rational(int P, int Q = 1);
void display() const; // _p:_q
void add(const Rational&);
void sub(const Rational&);
void mult(const Rational&);
void div(const Rational&);
};
#endif
In Rational.cpp, implement these member functions. You must make sure that you never have
_q zero[1]. Your Rational number must be stored in reduced form, that is, _p and _q must be relatively prime.
You must not use any built-in functions
In RationalMain.cpp, write a test main to test your code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
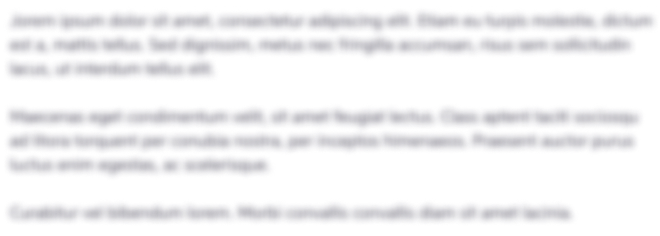
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started