Question
Please, Please, I need help with why am I getting these errors Please, Please Next, you must develop a Python module in a PY file,
Please, Please, I need help with why am I getting these errors Please, Please
Next, you must develop a Python module in a PY file, using object-oriented programming methodology, to enable CRUD functionality for the database. To support code reusability, your Python code needs to be importable as a module by other Python scripts. Develop a CRUD class that, when instantiated, provides the following functionality:A Create method that inserts a document into a specified MongoDB database and collection
Input -> argument to function will be a set of key/value pairs in the data type acceptable to the MongoDB driver insert API call.
Return -> True if successful insert, else False.
A Read method that queries for document(s) from a specified MongoDB database and specified collection
Input -> arguments to function should be the key/value lookup pair to use with the MongoDB driver find API call.
Return -> result in cursor if successful, else MongoDB returned error message.
An Update method that queries for and changes document(s) from a specified MongoDB database and specified collection
Input -> arguments to function should be the key/value lookup pair to use with the MongoDB driver find API call. Last argument to function will be a set of key/value pairs in the data type acceptable to the MongoDB driver insert API call.
Return -> result in JSON format if successful, else MongoDB returned error message.
A Delete method that queries for and removes document(s) from a specified MongoDB database and specified collection
Input -> arguments to function should be the key/value lookup pair to use with the MongoDB driver find API call.
Return -> result in JSON format if successful, else MongoDB returned error message.
As you develop your code, be sure to use industry standard best practices such as proper naming conventions, exception handling, and in-line comments. This will ensure that your code is easy to read and reusable for future projects. Refer to the Python Style Guide, located in the Supporting Materials section, to help with these industry standard best practices. Note: If you completed the Module Four Milestone, you have already developed the Create and Read functionality.
Finally, you must test your Python module to make sure that it works. To do this, create a Python script that imports your CRUD Python module to call and test all instances of CRUD functionality. This script should be created in a separate Jupyter Notebook (IPYNB) file, and should import and instantiate an object from your CRUD library to effect changes in MongoDB. Be sure to use the username and password for the aacuser account for authentication when instantiating the class. After creating your script, execute it in Jupyter Notebook and take screenshots of the commands and their execution. These screenshots will later be included in your README file. Note: If you completed the Module Four Milestone, you have already begun this work. Expand your script to call and test the Update and Delete functionality.
README File
Grazioso Salvare has requested documentation to accompany the CRUD Python module. This will ensure that they are able to understand the work that was completed. It will also help them maintain the code for the database.
To document this project, you must create a README file that includes the following:
An explanation of the purpose of the CRUD Python module
An explanation of how the module should be used, including:
A description of the Python driver for Mongo that was used and why it was chosen
An explanation of the attributes and working functionality of the CRUD operations
A demonstration of the modules functional operations, including:
Screenshots of the MongoDB import execution. You took these screenshots in Step 1.
Screenshots of the user authentication execution. You took these screenshots in Step 2.
Screenshots of the CRUD functionality test execution. You took these screenshots in Step 4.
Note: If you completed the Module Four Milestone, you have already begun work on your README file. You will need to add more information to complete your README file and fully document your work in Project One.
What to Submit
To complete this project, you must submit the following:
Database Commands and CRUD Python Module
Submit the IPYNB and PY files containing your code for the project. This includes the Python module that you developed to enable all CRUD functionality and the Python script that calls that functionality. All code files should follow industry standard best practices, including well-commented code.
README File
Your submission should be a Word (DOC or DOCX) file containing an explanation of the Python module that you developed to enable CRUD functionality. In your README file, be sure to include all required screenshots.
Supporting Materials
The following resource(s) may help support your work on the project:
Data Set: Austin Animal Center Outcomes Spreadsheet Grazioso Salvare has provided you with this sample data set (CSV file) of animal center outcomes. This will become the basis of your database and can be used to test the functionality of your code. This data set has been modified for the purposes of this project. Specifically, the following columns have been added: location_lat (latitude), location_long (longitude), and age_upon_outcome_in_weeks (the age of the animal, given in weeks). Reference: Austin Animal Center. (2020). Austin animal center outcomes [Data set]. City of Austin, Texas Open Data Portal. https://doi.org/10.26000/025.000001
Reading: CS 340 Jupyter Notebook in Apporto (Virtual Lab) Tutorial PDF This tutorial will help you navigate Jupyter Notebook, your IDE for this course. Use this tutorial to learn how to access Jupyter Notebook via the Virtual Lab (Apporto), as well as how to create, save, and download your PY and IPYNB files.
Reading: CS 340 Mongo in Apporto (Virtual Lab) Tutorial PDF This tutorial will help you navigate the different Mongo tools needed for your development. Use this tutorial to help you import the data set and create the accounts needed for user authentication.
Textbook: Head First Python This Shapiro Library textbook was designed to teach the Python programming language. Refer to this resource if you need a refresher on any Python syntax as you develop your code.
Reading: Python Style Guide Refer to this style guide when developing your Python code for this project. It is important that your code follows industry standard best practices, such as including clear variable names, exception handling, and in-line comments throughout your code.
Reading: Make a README This reading describes the purpose behind README files, and will help you keep in mind the purpose and intended audience for your README file. You are not required to use the same sections as suggested in this reading. As a note, the examples in this article use the MD format, which is a common format for README files on GitHub. You have been asked to submit your README file as a Word document for this project.
All the code
Animalshelter.py
from jupyter_plotly_dash import JupyterDash import dash_core_components as dcc import dash_html_components as html import dash from dash.dependencies import Input, Output import json import uuid import urllib.parse
from pymongo import MongoClient from bson.json_util import dumps class AnimalShelter(object): """ CRUD operations for Animal collection in MongoDB """
def __init__(self, username, password): # Initializing the MongoClient using the specified path to my port # access the MongoDB databases and collections. self.client= MongoClient('mongodb://localhost:29840') #init connect to mongodb with authentication #self.client = MongoClient('mongodb://%s:%s@localhost:29840'%(username, password)) #Setting the AAC database to be worked from self.database = self.client['AAC'] # The method to implement the C in CRUD. def create(self, data): #Checks to see if the data is null or empty and returns false in either case if data is not None: if data: self.database.animals.insert_one(data) return True else: return False
#Read method that queries for document(s) from a specified MongoDB database and specified collection def read(self, query): try: result = self.collection.find(query) return result except Exception as e: return str(e) #An Update method that queries for and changes document(s) from a specified MongoDB database and specified collection def update(self, query, data): try: self.collection.update_many(query, {'$set': data}) return True except Exception as e: return str(e)
#A Delete method that queries for and removes document(s) from a specified MongoDB database and specified collection def delete(self, query): try: self.collection.delete_many(query) return True except Exception as e: return str(e)
TestinApp.PY
from AnimalShelter import AnimalShelter # Test cases for animalshelter input and output # Create a class object with database name and collection name AnimalShelter = AnimalShelter
# Insert a animal records using create method animal_data = [ { "name":"bruno", "species":"cat", "age": 4 }, { "name":"missy", "species":"dog", "age": 2 }, { "name":"sticky", "species":"dog", "age": 7 } { "name": "Tom", "species": "Cat", "age": 5 } ]
# Print the returned output for your reference print(animal.create(AnimalShelter))
# Now read using read command read_test = animal.read({"species":"Dog"}) # print the cursor object returned by read command print(read_test) print(" ") print("Data from cursor") # Print the data from the cursor for data in read_test: print(data)
# Update elements using update updateDogName = animal.update({ "species":"Dog" },{ "$set":{"name":"sticky"} }) print("Objects that got updated ") print(updateDogName)
Testing.ipnb
from AnimalShelter import AnimalShelter
# Instantiate an object from AnimalShelter AnimalShelter = AnimalShelter("aacuser", "*************")
# Test create method data = {"name": "Tom", "species": "Cat", "age": 5} animal_shelter.create(data)
# Test read method data = animal_shelter.read({"name": "Tom"}) for d in data: print(d) Error message
PLEASE HELP;******************************** IT IS OVERDUE*************************************************** PLEASE HELP.
Testing - Jupyter Notebook - Mozilla Firefox Examples/Project_One/ testinApp.py - Jupyter Te *AnimalShelter.py - Jupy Testing - Jupyter Notebo (i) (i) localhost:8888otebooks/Examples/Project_One/Testing.ipynb III (9) jupyter Testing Last Checkpoint: Yesterday at 12:57 AM (autosaved) Logout \begin{tabular}{l} Insert \\ \\ \hline \end{tabular} \begin{tabular}{l|l|l|l|l|l} Cell & Kernel & Widgets Help \\ \hline M Run & & C & B & Code \\ \hline \end{tabular} In [4]: from Animalshelter import Animalshelter \# Instantiate an object from Animalshelter AnimalShelter = Animalshelter("aacuser", ") \# Test create method data = { "name": "Tom", "species": "Cat", "age": 5 animal_shelter.create (data) \# Test read method data = animal_shelter. read({ "name": "Tom" }) for d in data: print(d) ModuleNotFounderror Traceback (most recent call last) in 1 from Animalshelter import Animalshelter 2 3 \# Instantiate an object from Animalshelter 4 AnimalShelter = AnimalShelter("aacuser", "Software2019") 5 ModuleNotFoundError: No module named 'Animalshelter' In [ ]: class Animalshelter(AAC): "" CRUD operations for Animal collection in MongoDB """ def _init__(self, username, password): \# Initializing the Mongoclient using the specified path to my port \# access the MongoDB databases and collections. self.client= MongoClient ('mongodb:// localhost:29840') \#init connect to mongodb with authentication \#self.client = Mongoclient('mongodb://\%S:\%s@localhost:29840\%(username, password)) \#Setting the AAC database to be worked from self. database = self. client ['AAC'] \# The method to implement the C in CRUD. def create(self, data): \#Checks to see if the data is null or empty and returns false in either case if data is not None: if data: self.database.animals.insert_one (data) return True else: return False \#Read method that queries for document(s) from a specified MongoDB database and specified collection def read(self, query): try: result = self. collection.find(query) return result except Exception as e: return str(e) \#An Update method that queries for and changes document(s) from a specified MongoDB database an specified collection def update(self, query, data): try: ModuleNotFoundError: No module named 'Animalshelter
Step by Step Solution
There are 3 Steps involved in it
Step: 1
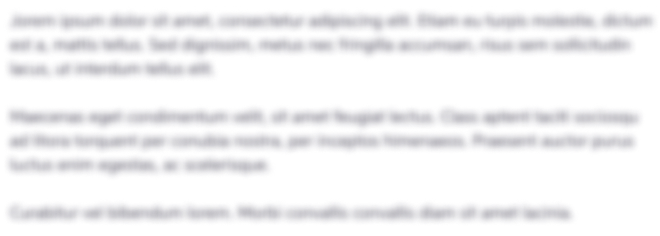
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started