Question
Please provide the solution for this assignment in Lisp code. Do NOT use any AI tools like Chat-GPT to generate codes/answers as that'd a violation.
Please provide the solution for this assignment in Lisp code.
Do NOT use any AI tools like Chat-GPT to generate codes/answers as that'd a violation.
-------------------------------------------------------------------------------------------------------
_____ _________________ | | | (CUBE A) (CUBE B) | D | H | (CUBE C) (CUBE D) |_____|_________________| (BAR G) (BAR H) | | | | (VERTICAL G) | | | C | (HORIZONTAL H) | | |_____| (ON G *TABLE*) | | | | (ON A *TABLE*) | G | | B | (NEAR A G); "A is one unit to the right | | |_____| of G; valid only for blocks on *TABLE* | | | | (ON B A) | | | A | (ON C B), *TABLE*____|_____|_____|_____|_______(ON H C); This is "symmetrical"-ON
Again be sure to use LOCAL variables within functions (e.g., introduced by 'let') except for truly global parameters (such as a hash table) that multiple functions need to access. Use names of form *....* for global parameters. Unless stated otherwise, include input checking in your functions; i.e., if the user gives an argument which is not of the specied form, your function should return **ERROR**, and briefly indicate the kind of error.
Informal description ```````````````````` As a final Blocks World task, you are asked to implement "spatial question answering" (QA) for Blocks World structures, as an add-on to the previously programmed ability to build specified goal structures. This task is somewhat simpler than Lisp 1 and Lisp 2 (hopefully reducing end-of-semester stress).
The idea is to code an interactive "Blocks World agent" (details below) that first asks the user "What would you like me to build?". Then in response to the user's specification, the agent tries to produce a construction satisfying the specification, and then prompts the user repeatedly with "Do you have a spatial question?"; then, unless the user says NO to halt the process, it tries to answer the user's question. Questions are posed declaratively, e.g., (SUPPORTS C H) means "Does block C directly support bar H?". Besides SUPPORTS questions, the user can also ask ON, HORIZONTAL, VERTICAL, and HELPS-SUPPORT questions. ON in questions will be interpreted as allowing for any of ON-2, ON-1, ON, ON+1, ON+2 in a specific structural description. (HELPS-SUPPORT X1 X2) will be considered to hold just in case there's a SUPPORT-chain from X1 to X2. (This is the least trivial part of the assignment.)
NOTE: If there were more time for Lisp3, it would have been interesting to infer relations like RIGHT-OF, or ENTIRELY-ABOVE as well. This could be done by first inferring left-corner position indices (0, 1, ...) for the bottom blocks, where 0 is the left corner position of the block that was first put on the table. The other left-corner positions follow from the NEXT-TO and NEAR relations among the bottom blocks, and from the unit size of cubes and the length (viz. 3) of horizontal bars. Once we know left corners of bottom blocks, we can infer bottom left corners of all blocks from what they're on and the levels (as per Lisp2) of the blocks they're on. While you probably don't regret not needing to write such code, it's worth pondering -- simply from an AI perspective -- how challenging the traditional Blocks World reasoning problems can be; and here we're just considering 2-D configurations of 8 blocks, coming in two simple shapes. LLMs are very poor at these sorts of problems!
Tasks ````` 0. Note that when the user calls the Blocks World agent, the *BLOCKS* global parameter should have been initialized to ((CUBE A) (CUBE B) (CUBE C) (CUBE D) (CUBE E) (CUBE F) (BAR G) (BAR H) (HORIZONTAL G) (HORIZONTAL H)), as in Lisp1 and Lisp2.
1. Write a function (DEFUN BW-AGENT ( ) ...); note: empty argument list.
First this should set *FACTS-HT* and *SUPPORTS* to new hash tables, as they may be filled from a previous function call. Likewise it should set *BLOCKS-AT-LEVEL-I* to a new length-12 array.
Then the program should begin by prompting the user with the question "What would you like me to build?" (use Lisp's (format t "..." ...) for this). The user then supplies a goal structure specification, i.e., a list in which all the properties that should be true in the instantiated specification are listed, including the CUBE, BAR, and various types of ON-properties, as well as NEXT-TO and NEAR relations and any VERTICAL properties (for vertical bars in the goal structure). Be sure to include (ON ?X *TABLE*) for blocks ?X even if (NEXT-TO ?X ..) or (NEAR ?X ..) are specified in the goal specification (implying that ?X is on *TABLE*). The goal specification will have variables (names beginning with a question mark) for all blocks, and the only individual constant in the goal description will be *TABLE*. Assuming that the construction (using code from Lisp1 and Lisp2) succeeds without an error message (in that process also filling in the *FACTS-HT* and *SUPPORTS* hash tables, and the *BLOCKS-AT-LEVEL-I* array), BW-AGENT should then print out, on separate lines, - the list of block bindings used, - the construction steps, and - the instantiated specification (i.e., the user's goal specification with variables replaced by constants).
BW-AGENT then asks "Do you have a spatial question?" The user then either prints NO, terminating the interaction (BW-AGENT prints "Good bye" as its final output), or supplies a question in one of the forms (where X, Y are constants): (SUPPORTS X Y), (ON X Y), (HORIZONTAL X), (VERTICAL X), or (HELPS-SUPPORT X Y). SUPPORTS, HORIZONTAL, and VERTICAL should be interpreted in exactly the way they were defined in Lisp1 and Lisp2. But (ON X Y) as a query is treated as true if (as already mentioned) any one of the ON-relations ON-2, ON-1, ON, ON+1, ON+2 hold for X, Y. (HELPS-SUPPORT X Y) will require a subroutine SUPPORT-CHAIN (see below). If the queried property is found to be true, BW-AGENT should respond with , e.g., "Yes, block C SUPPORTS block H", "Yes, block H is HORIZONTAL", "Yes, block A HELPS-SUPPORT block H", etc. (The required answer patterns should be clear from this -- and are easily constructed using (format t "..." ...).) If the queried property is not found to be true, the output can simply be "No". Note that by the Closed World Assumption, we'll be answering "No" to questions such as (HORIZONTAL A), even though A isn't a bar.
After a query has been answered, BW-AGENT goes back to asking "Do you have a spatial question?", and so on, until the USER answers NO.
2. Write the function (DEFUN SUPPORT-CHAIN (X Y) ...),
as required by BW-AGENT. X, Y are expected to be distinct names of blocks, or X could by *TABLE*. It's assumed that the global *SUPPORTS* hash table has been filled in when this is called.
The output should be a support chain -- a sequence of block names (or possibly an initial *TABLE*) starting with X and ending with Y, where each block (partially or fully) supports the next one in the chain. Note that support chains for X and Y are not necessarily unique. Here are two example structures and some support chains:
HHHHHH SOME SUPPORT-CHAINS: GG CC (SUPPORT-CHAIN *TABLE* H) = (*TABLE* G H), (*TABLE* A B C H) GG BB (SUPPORT-CHAIN A C) = (A B C) GG AA (SUPPORT-CHAIN G H) = (G H) TTTTTTTTTT
Example 2 (shoe) ````````` HHHHHH SOME SUPPORT-CHAINS: DD EEFF (SUPPORT-CHAIN *TABLE* H) = (*TABLE* A C D H), (*TABLE* B G E H) CCGGGGGG (SUPPORT-CHAIN B F) = (B G F) AA BB (SUPPORT-CHAIN B D) = NIL [this leads to a "No" for HELPS-SUPPORT] TTTTTTTTTTTT
You are urged to think recursively in writing this function!
3. Demonstrate your code for (1) and (2) for enough examples to convincingly show your code works for diverse examples. Discuss any points of interest. (If you did more than what is asked for, make clear what it is!) -----------------------------------------------------------------------------------------------
- Please include Lisp codes for all parts as I cannot ask this question in parts and under separate questions without previous parts.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
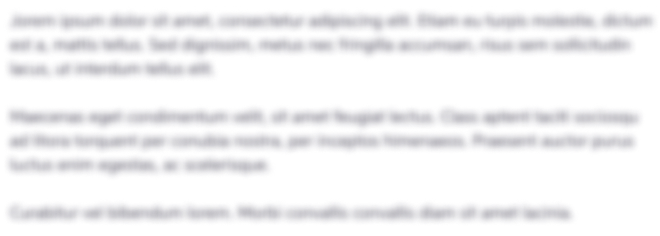
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started