Question
Please read all instructions and highlight or BOLD any changes made. Thanks! Pseudocode thus far: // Vector pseudocode Open file containing course information Create empty
Please read all instructions and highlight or BOLD any changes made. Thanks!
Pseudocode thus far:
// Vector pseudocode
Open file containing course information
Create empty vector to store course objects
Read each line
WHILE file has a next line
Parse each line
Verify at least two parameters on each line
IF (parameters.size()
Display ERROR message
END
Check if prerequisite exists as a course in the file
for p in prerequisites:
found = false
for c in courses:
if (c.courseNumber == p):
found = true
break
IF not found
Display ERROR
END
Create course object
Store course object in vector
CLOSE FILE
Function for searching for a specific course and Display information
Search for the course in the vector
for c in courses:
IF (c.courseNumber == courseNumber)
Print course information
Print prerequisite course information
Verify at least two parameters on each line
IF (parameters.size()
Display ERROR
END
Check if prerequisite exists as a course in the file
for p in prerequisites:
found = false
for c in courses:
IF (c.courseNumber == p):
found = true
BREAK
IF not found
Display ERROR
END
Create a course object
Store course object in vector
CLOSE FILE
Function for searching for a specific course and printing its information and prerequisites
void printCourseInformation(vector
Search for the course in the vector
for c in courses:
if (c.courseNumber == courseNumber)
Display course information
Display prerequisite course information
// Hashtable pseudocode
open file "courseInformation.csv" for reading
IF file does not exist
Display ERROR and exit
ELSE
create empty hash table "courses"
WHILE a line exists in file
read line
split line into tokens
IF number of tokens is
display ERROR
continue to next line
END IF
IF first token not a number
display ERROR
continue to next line
END IF
IF a third token exists
IF third token not a number
Display ERROR
continue to next line
END IF
check if third token is a key in "courses"
IF not
Display ERROR
continue to next line
END IF
END IF
create new course object with first token as course number, second token as course title, and third as prerequisite
insert course object into "courses" with course number as key
END WHILE
END IF
CLOSE FILE
Create course objects and store in Hash Table
class Course
courseNumber
courseTitle
prerequisite
constructor(courseNumber, courseTitle, prerequisite)
this.courseNumber = courseNumber
this.courseTitle = courseTitle
this.prerequisite = prerequisite
END constructor
END class
Create empty hash table named "courses"
FOR each line
create new course object with line tokens as arguments
insert course object into "courses" with course number as key
END FOR
FOR each key in "courses" hash table
retrieve course object from "courses" hash table
Display courseNumber, courseTitle, and prerequisite
END FOR
Define function to display course info and prerequisites
loop through hash table
FOR (int i = 0; i
IF current index not empty
Create pointer to head of linked list at current index
loop through linked list at current index
Display the course number, title, and prerequisites
loop through prerequisites of the current course
Display prerequisite course number
END WHILE
move to next course in linked list
END IF
END FOR
END
// Tree pseudocode
open file "courseInformation.csv" for reading
IF file does not exist
Display ERROR and exit
ELSE
create empty tree data structure "courses"
WHILE a line exists in file
read line
split line into tokens
IF number of tokens is
display ERROR
continue to next line
END IF
IF first token not a number
display ERROR
continue to next line
END IF
IF a third token exists
IF third token not a number
Display ERROR
continue to next line
END IF
Check if third token exists as a node in "courses"
IF not
Display ERROR
continue to next line
END IF
END IF
create new course object with first token as course number, second token as course title, and third as prerequisite
insert course object as a node in "courses" with the prerequisite as its parent node
END WHILE
END IF
CLOSE FILE
Create course objects and store in Tree Data Structure
class Course
courseNumber
courseTitle
prerequisite
constructor(courseNumber, courseTitle, prerequisite)
this.courseNumber = courseNumber
this.courseTitle = courseTitle
this.prerequisite = prerequisite
END constructor
END class
Define function to traverse and display course info and prerequisites
function displayCourses(node)
display courseNumber, courseTitle, and prerequisite of current node
IF node has children
FOR each child of node
call displayCourses function with child as argument
END FOR
END IF
END
call displayCourses function with root node of "courses" tree data structure as argument
Example Runtime Analysis
When you are ready to begin analyzing the runtime for the data structures that you have created pseudocode for, use the chart below to support your work. This example is for printing course information when using the vector data structure. As a reminder, this is the same pairing that was bolded in the pseudocode from the first part of this document.
Code | Line Cost | # Times Executes | Total Cost |
for all courses | 1 | n | n |
if the course is the same as courseNumber | 1 | n | n |
print out the course information | 1 | 1 | 1 |
for each prerequisite of the course | 1 | n | n |
print the prerequisite course information | 1 | n | n |
Total Cost | 4n + 1 | ||
Runtime | O(n) |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
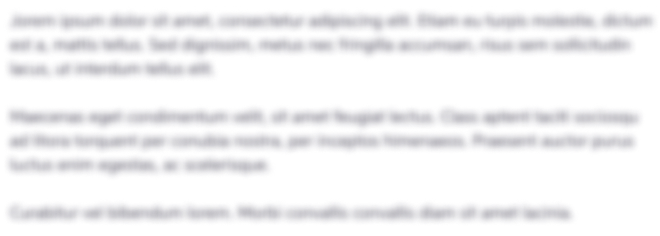
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started