Question
Please read the instructions and finish the code. Please read the comments as well as you code :) All the info is pasted here for
Please read the instructions and finish the code. Please read the comments as well as you code :) All the info is pasted here for you.
Thank you for your help!
This class is complete. It does not need anything.
/**
* Salary for baseball players is stored.
*
*/public class PlayerSalary
{
//Constructor for objects of class PlayerSalary
int yearID;
String teamID;
String leagueID;
String playerID;
int salary;
public PlayerSalary(int yearID, String teamID, String leagueID, String playerID, int salary)
{
// initialize instance variables
this.yearID = yearID;
this.teamID = teamID;
this.leagueID = leagueID;
this.playerID = playerID;
this.salary = salary;
}
/**
* returns a String representation of a PlayerSalary object
*/
public String toString(){
return "Players information " +
" Year : " + yearID +
" Team : " + teamID +
" League : " + leagueID +
" Player : " + playerID +
" Salary : " + salary ; // this is the format with info
}
// Add getter methods below
public int getYearID() { return yearID; }
public String getTeamID() { return teamID; }
public String getLeagueID() { return leagueID; }
public String getPlayerID() { return playerID; }
public int getSalary() { return salary; }
// Add a main method here to test this class
public static void main(String [] args){
PlayerSalary info$ = new PlayerSalary(2015,"Lakers","NBA","Some",60000) ;
System.out.println(info$.getPlayerID());
System.out.println(info$.getYearID());
System.out.println(info$.getLeagueID());
}
}
// edn of class
~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
This is where the playerSalary class is going to be used.
import java.io.File;
import java.io.FileNotFoundException;
import java.util.*;
/*
* PlayerSalaries database
*/
public class PlayerSalaries
{
ArrayList
public PlayerSalaries(String filename) throws FileNotFoundException
{
//Get scanner instance - this will read lines from file
Scanner scanner = new Scanner(new File(filename));
// Process CSV file -
// reading line of text, then scanning the text for tokens
// delimited by comma or end of line
// Read the first int in file - it indicates how many
// records follow
int howMany = scanner.nextInt();
// Instantiate/allocate salaries list - use
// first number in file to set list capacity
// YOUR CODE to instantiate/allocate salaries list GOES HERE
salaries = new ArrayList
// Consume rest of line 1
scanner.nextLine();
scanner.nextLine();// And consume line 2
// Read each line/record in file
// currenlty only reads 2 lines so you can see what is happening.
// When you are comfortable processing all data in file,
// change 3 to howMany
for(int lineNum = 1; lineNum
{
// Read entire line into a String
String line = scanner.nextLine();
// Associate a Scanner with the String
Scanner lineScanner = new Scanner(line);
// State that commas will be the delimiter/separator -
// since the data in a CSV file
lineScanner.useDelimiter(",");
// The following code domonstrates how to pull words out of file
System.out.println(" This is what is on line " + lineNum);
while(lineScanner.hasNext()){ // parse line
// Read a word and print it
System.out.println(lineScanner.next().get(4));
//System.out.println(lineScanner.nextLine());
}
// ADD CODE to create a PlayerSalary object and add it to the database
}
}
public int averageSalaryInYear(int year)
{
int salaries, count, i ;
double avg;
// for ( i = ; i
//placeholder return, this should return average salary int
return -1;
}
public PlayerSalary findPlayerInYear(String player, int year)
{
//placeholder return, this should return PlayerSalary Object
return null ;
}
public PlayerSalary highestSalaryInYear(int year)
{
//placeholder return, this should return PlayerSalary Object
return null;
}
public PlayerSalary lowestSalaryInYear(int year)
{
//placeholder return, this should return PlayerSalary Object
return null;
}
public PlayerSalary highestSalaryInTeamInYear(String team, int year)
{
//placeholder return, this should return PlayerSalary Object
return null;
}
public PlayerSalary lowestSalaryInTeamInYear(String team, int year)
{
//placeholder return, this should return PlayerSalary Object
return null;
}
public PlayerSalary highestSalaryInLeagueInYear(String team, int year)
{
//placeholder return, this should return PlayerSalary Object
return null;
}
public PlayerSalary lowestSalaryInLeagueInYear(String team, int year)
{
//placeholder return, this should return PlayerSalary Object
return null;
}
public ArrayList
{
//placeholder return, this should return PlayerSalary ArrayList
return null;
}
public void displayAllSalaries(){
for (int i = 0; i
// Note, this depends on the PlayerSalary class
// having a toString method
System.out.println(salaries.get(i));
}
}
// end of class
*****************************************************************************************************************
This is the main where everything is going to done.
import java.io.*;
import java.util.Scanner;
public class SalaryLookUp
{
public static void main(String[] args) throws FileNotFoundException
{
//PlayerSalaries ps1 = new PlayerSalaries("Salaries.csv");
displayMenu();
}
public static void displayMenu(){
System.out.println("1: See average salary in a specific year");
System.out.println("2: See a specific players salary in a specific year");
System.out.println("3: See the highest salary in a specific year");
System.out.println("4: See the lowest salary in a specific year");
System.out.println("5: See the highest salary on a specific team in a specific year");
System.out.println("6: See the lowest salary on a specific team in a specific year");
System.out.println("8: See the lowest salary in league in a specific year");
System.out.println("9: Compare salaries of two specific players in a specific year");
System.out.println();
System.out.println("10: Quit");
}
}
Programming Assignment Overview: Let's Play Ball! Collaboration policy : same as HW 4 There is a HW5 download folder for you on OAKS. In this assignment, you will write a program to reads in the salary data from baseball players, store the data in a list (ArrayList) of objects, and provides several methods to query the data. The data is provided to you in a comma- delimited text file which is part of a bigger database. We will only use the Salaries.csv file provided. You need to implement the following UML: The Constructor provided reads in the comma-delimited data file. Player Salaries You must add the code to create a list of Player Salary objects, - Salaries: ArrayListStep by Step Solution
There are 3 Steps involved in it
Step: 1
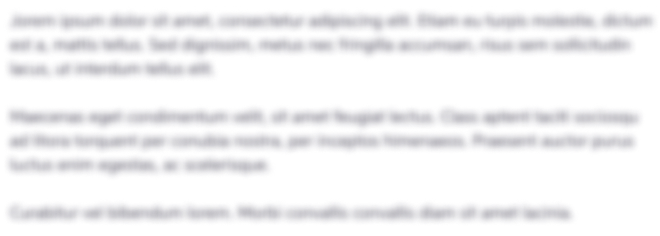
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started