Question
Please refer to program 4 code below and use same variables! -- There needs to be at least TWO non trival functions! PROGRAMMING ASSIGNMENT #5
Please refer to program 4 code below and use same variables! -- There needs to be at least TWO non trival functions!
PROGRAMMING ASSIGNMENT #5 This program is similar to programming assignment #4. That is, you will have all of the requirements as in Programming Assignment #4, including data error checking on all inputs. Your program is to behave in the exact same manner as assignment #4, however how you structure your code is a different story. Now we will use functions. Recall Programming Assignment #4: Write a C program that allows the user to make some simple banking transactions. The program should first prompt the user to enter the current balance of his/her bank account (in dollars and cents, not less than zero). The program should then prompt the user to enter the number of withdrawals to make, and then the number of deposits to make. For this assignment, let's set a maximum of 5 deposits and 5 withdrawals (etc.) Here is the change for program #5: There should be at least two non-trivial functions in the program. (Non-trivial means that the function does more than simply print a line of text.) Here are some examples of what you might want to use for your functions: Input functions:
1. Obtain opening (current) balance.
2. Obtain number the number of deposits.
3. Obtain number of withdrawals.
4. Obtain deposit amounts.
5. Obtain withdrawal amounts.
Output functions:
1. Display closing balance and associated message.
2. Display message based on closing balance.
3. Display bank record.
Hints: Your code from assignment #4 does not need to be modified too much to solve this problem. The algorithm is the same. Outputting the contents of the arrays in "record form" is pretty straightforward, and should be done in a loop. (This could be a good place for a function.) The most complicated of the above functions is the one which would display the bank record. If you decide that you want to attempt this one, I will give you a head start by providing the function header as shown here: void (display_bank_record (float start_balance, float deposits [ ], int num_deposits, float withdrawals [ ], int num_withdrawals, float end_balance) Good luck!
>>> Here is Assignment Four
#include
/* Variable Declarations ----------------------------------------- */
/* I have made these variables global so that every function can access*/
int i;
int number_of_deposits;
int number_of_withdrawals;
float beginning_balance, closing_balance;
float deposit_total = 0;
float withdrawal_total = 0;
float deposits[50], withdrawals[50];
/* Functions Declarations ----------------------------------------- */ void inputBalance(); void inputWithdrawls_Deposit(); void display_bank_record (float start_balance, float deposits [ ], int number_of_deposits, float withdrawals [ ], int number_of_withdrawals, float end_balance) ;
int main(int argc, char *argv[]) {
/* Greeting ----------------------------------------------------- */
/* -------------------------------------------------------------- */
printf ("Welcome to the Computer Banking System ");
printf (" "); // spaciNG
//*******Function Calling inputBalance(); inputWithdrawls_Deposit();
for (i = 1; i <= number_of_deposits; ++i)
{
do
{
printf ("Enter the amount of deposit #%i: ", i);
scanf ("%f", &deposits[i]);
if (deposits[i] <= 0)
{
printf ("***Deposit amount must be at least zero, please re-enter. ");
}
} while (deposits[i] <= 0);
deposit_total = deposit_total + deposits[i];
}
printf (" "); // spacing
for (i = 1; i <= number_of_withdrawals; ++i)
{
do {
printf ("Enter the amount of withdrawal #%i: ", i);
scanf ("%f", &withdrawals[i]);
if (withdrawals[i] < 0)
{
printf ("Withdrawal amount must be at least zero, please re-enter. ");
}
else if (withdrawals[i] > beginning_balance)
{ printf ("***Withdrawal amount eiceeds current balance, please re-enter. ");
} /* do - if - else loop ends when amount is greater than 0 */
} while (withdrawals[i] > beginning_balance || withdrawals[i] <=0);
withdrawal_total = withdrawal_total + withdrawals[i];
closing_balance = beginning_balance - withdrawal_total + deposit_total;
if (closing_balance == 0)
{
printf (" ***No more withdrawals allowed; current balance is 0.*** ");
number_of_withdrawals = i;
}
/* Closing Balance ------------------------------------------ */
/* ---------------------------------------------------------- */
printf (" "); // spacing
printf ("***The closing balance is %.2f*** ", closing_balance);
/* Message displayed will depend on closing balance.--------- */
/* ---------------------------------------------------------- */
if (closing_balance >= 50000.00)
printf ("***It is time to invest some money!*** ");
else if (closing_balance >= 15000.00)
printf ("***Maybe you should consider a CD.*** ");
else if (closing_balance >= 1000.00)
printf ("***Keep up the good work!*** ");
else if (closing_balance >= 0)
printf ("Your balance is getting low!*** ");
} /* end if-else loop */
/* Bank Record --------------------------------------------- */
/* --------------------------------------------------------- */
printf (" "); // spacing
printf ("***Bank Record*** ");
display_bank_record (beginning_balance, deposits, number_of_deposits, withdrawals, number_of_withdrawals, closing_balance) ; //function call
return 0; }
/* ***Function Definations *****/
void inputBalance() {
/* Prompt user to enter current balance. ----------------------- */
/* ------------------------------------------------------------- */
printf ("Enter your current balance in dollars and cents: ");
scanf ("%f", &beginning_balance);
while (beginning_balance < 0) { printf ("***Beginning balance must be at least zero, please re-enter. "); scanf ("%f", &beginning_balance);
}
}
void inputWithdrawls_Deposit() {
/* Prompt user to enter number of withdrawals. ------------------- */
/* --------------------------------------------------------------- */
printf (" "); // spacing
printf ("Enter the number of withdrawals (0 - 5): ");
scanf ("%i", &number_of_withdrawals);
/* Limits user to number of withdrawals between 0 and 5 */
while (number_of_withdrawals < 0 || number_of_withdrawals > 5)
{ printf ("***Invalid number of withdrawals, please re-enter. "); scanf ("%i", &number_of_withdrawals);
}
do
{
/* Prompt user to enter number of deposits. ------------------------ */
/* ----------------------------------------------------------------- */
printf (" "); // spacing
printf ("Enter the number of deposits (0 - 5): ");
scanf ("%i", &number_of_deposits);
/* Limits user to number of deposits between 0 and 5 */
if (number_of_deposits < 0 || number_of_deposits > 5)
printf ("***Invalid number of deposits, please re-enter."); }
while (number_of_deposits < 0 || number_of_deposits > 5);
}
void display_bank_record (float start_balance, float deposits[ ], int number_of_deposits, float withdrawals[ ], int number_of_withdrawals, float end_balance) { /****Starting Balance****/
printf ("Starting Balance: $%.2f ", beginning_balance);
/****total deposits****/
for (i = 1; i <= number_of_deposits; ++i)
{
printf ("Deposit #%i: %.2f ", i, deposits[i]);
}
printf (" ");
/****total Wihdrawls****/
for (i = 1; i <=number_of_withdrawals; ++i)
{
printf ("Withdrawal #%i: %.2f ", i, withdrawals[i]);
} /* end for loop */
printf (" ");
/****Endng Balance****/
printf ("Ending Balance: $%.2f ", end_balance);
printf (" ");
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
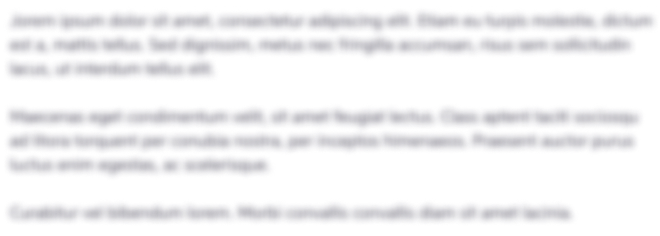
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started