Question
Please rewrite following pseudocode to match EXAMPLE given below pseudocode: Pseudocode // Initialize the Hash Table class class HashTable // Data Members vector nodes unsigned
Please rewrite following pseudocode to match EXAMPLE given below pseudocode:
Pseudocode
// Initialize the Hash Table class
class HashTable
// Data Members
vector
unsigned int tableSize
// Member functions
hash(key)
Insert(bid)
PrintAll()
Remove(bidId)
Search(bidId)
END
-------------------
// Initialize the Node class
class Node
// Data Members
Bid bid
unsigned int key
Node *next
// Member functions
default constructor
initialize with a bid
initialize with a bid and a key
END
-------------------
// Initialize the Hash Table constructor
HashTable()
// Initialize tableSize
tableSize = DEFAULT_SIZE
// Resize nodes size
nodes.resize(tableSize)
END
-------------------
// Initialize the Hash Table constructor with size
HashTable(size)
// Invoke local tableSize to size with this->
this->tableSize = size
// Resize nodes size
nodes.resize(tableSize)
END
-------------------
// Implement logic to free storage when class is destroyed
HashTable::~HashTable()
// Erase nodes beginning
nodes.erase(nodes.begin())
END
-------------------
// Implement logic to calculate a hash value
unsigned int HashTable::hash(key)
// Return key tableSize
RETURN key % tableSize
END
-------------------
// Implement logic to insert a bid
void HashTable::Insert(bid)
// Create the key for the given bid
unsigned int key = hash(atoi(bid.bidId.c_str()))
// Retrieve node using key
Node* node = &nodes[key]
// If no entry found for the key
IF node->key == UINT_MAX
// Assign this node to the key position
node->bid = bid
node->key = key
// Else if node is not used
ELSE IF node->key == UINT_MAX
// Assign old node key to UNIT_MAX, set to key, set old node to bid and old node next to null pointer
node->key = UINT_MAX
node->next = new Node(bid, key)
// Else find the next open node
ELSE
Node* curr = node
WHILE curr->next != nullptr
curr = curr->next
// Add new newNode to end
curr->next = new Node(bid, key)
END
-------------------
// Implement logic to print all bids
void HashTable::PrintAll()
// For node begin to end iterate
FOR i = 0 TO nodes.size()
Node* node = &nodes[i]
// If key not equal to UINT_MAX
IF node->key != UINT_MAX
// Output key, bidID, title, amount and fund
cout << node->key << ": " << node->bid.bidId << " | " << node->bid.title << " | "
<< node->bid.amount << " | " << node->bid.fund << endl
// Node is equal to next iter
node = node->next
// While node not equal to nullptr
WHILE node != nullptr
// Output key, bidID, title, amount and fund
cout << node->key << ": " << node->bid.bidId << " | " << node->bid.title << " | "
<< node->bid.amount << " | " << node->bid.fund << endl
// Node is equal to next node
node = node->next
END IF
END FOR
END
-------------------
// Implement logic to remove a bid
void HashTable::Remove(bidId)
// Set key equal to hash atoi bidID cstring
unsigned int key = hash(atoi(bidId.c_str()))
// Erase node begin and key
nodes.erase(nodes.begin() + key)
END
-------------------
// Implement logic to search for and return a bid
Bid HashTable::Search(bidId)
// Create the key for the given bid
unsigned int key = hash(atoi(bidId.c_str()))
// If entry found for the key
IF nodes[key].key != UINT_MAX
// Return node bid
RETURN nodes[key].bid
// If no entry found for the key
// Return bid
Bid bid
// While node not equal to nullptr
Node* node = nodes[key].next
WHILE node != nullptr
// If the current node matches, return it
IF node->bid.bidId == bidId
RETURN node->bid
// Node is equal to next node
node = node->next
END WHILE
// Return bid
RETURN bid
END
EXAMPLE that above pseudocode should be written as:
SelectionSort(numbers, numbersSize) { i = 0 j = 0 indexSmallest = 0 temp = 0 // Temporary variable for swap for (i = 0; i < numbersSize - 1; ++i) { // Find index of smallest remaining element indexSmallest = i for (j = i + 1; j < numbersSize; ++j) { if ( numbers[j] < numbers[indexSmallest] ) { indexSmallest = j } } // Swap numbers[i] and numbers[indexSmallest] temp = numbers[i] numbers[i] = numbers[indexSmallest] numbers[indexSmallest] = temp } } Thus, your pseudocode can be:
SelectionSort (vector list, int start, int end):
Definitions:
min as int (index of the current minimum bid), initialize as 0
size_t as int equal to list.size()
pos as int is the position within bids that divides sorted/unsorted, initialized to 0
iterate over list from index=pos to index = size_t -1
set min = pos
iterate over list from index = pos + 1 to index = t_size -1 remaining elements to the right of position
if this element's title is less than minimum title
min = index;
if (min != pos) {
swap the current minimum with bid at index pos
Step by Step Solution
There are 3 Steps involved in it
Step: 1
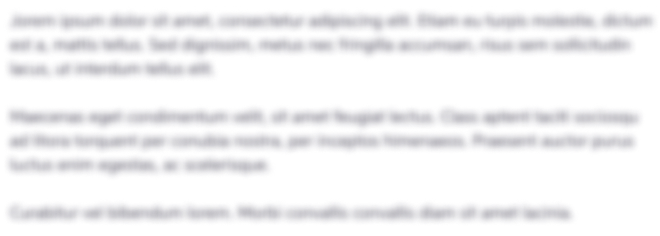
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started