Question
Please show the output which run in Esciplse with the full typed current code for the project blew,thank you so much. PROJECT DESCRIPTION The bank
Please show the output which run in Esciplse with the full typed current code for the project blew,thank you so much.
PROJECT DESCRIPTION
The bank is in desperate need of analytics from its clients for its loan application process. Currently records show 600 clients exist and the bank is hoping to expand its clientele and its potential by offering premium loans to deserved grantees.
Perform the data analysis as follows for this lab.
Project Details
-Add to your existing project files from lab 2, a new class called Records. Have the class extend the BankRecords class to grab hold its instance methods plus the BankRecord object array.
-Provide at least 2 comparator classes implementing the java.util.Comparator interface for comparing various fields for the following data analysis requirements.
-Perform the following analysis requirements and output detail for the Records class
Display the following data analytics in a coherent manner to the console:
average income for males vs. females
number of females with a mortgage and savings account
number of males with both a car and 1 child per location
Write all displayed data to a text file called bankrecords.txt relative to your project path as well. Append your name to the end of the file plus the date.
BANKRECORDs.java
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
public class BankRecords extends Client {
/**
* Arrays of objects to hold different attributes
*/
private String[] id;
private int[] age;
private Sex[] sex;
private Region[] region;
private double[] income;
private String[] isMarried;
private int[] children;
private String[] hasCar;
private String[] saveAccount;
private String[] currentAccount;
private String[] mortgage;
private String[] pep;
/**
* Arraylist to store the data input
*/
private ArrayList
@Override
public void readData() {
File file = new File("bank-Detail.csv");
input = new ArrayList
try {
Scanner scanner = new Scanner(file);
while (scanner.hasNext()) {
String row = scanner.nextLine();
input.add(row);
}
} catch (FileNotFoundException e) {
System.out.println("Input file not found");
}
}
@Override
public void processData() {
if (input != null) {
if (input.size() > 0) {
/**
* initializing all instance field arrays
*/
int n = input.size();
id = new String[n];
age = new int[n];
sex = new Sex[n];
region = new Region[n];
income = new double[n];
children = new int[n];
hasCar = new String[n];
isMarried=new String[n];
saveAccount = new String[n];
currentAccount = new String[n];
mortgage = new String[n];
pep = new String[n];
for (int i = 0; i < n; i++) {
/**
* getting the current row
*/
String row = input.get(i);
/**
* Splitting the row by comma into a string arrau
*/
String[] fields = row.split(",");
/**
* Extracting each fields and adding to corresponding arrays
*/
id[i] = fields[0].trim();
age[i] = Integer.parseInt(fields[1].trim());
if (fields[2].trim().equalsIgnoreCase("FEMALE")) {
sex[i] = Sex.FEMALE;
} else {
sex[i] = Sex.MALE;
}
String reg = fields[3].trim();
if (reg.equalsIgnoreCase("INNER_CITY")) {
region[i] = Region.INNER_CITY;
} else if (reg.equalsIgnoreCase("TOWN")) {
region[i] = Region.TOWN;
} else if (reg.equalsIgnoreCase("RURAL")) {
region[i] = Region.RURAL;
} else {
region[i] = Region.SUBURBAN;
}
income[i] = Double.parseDouble(fields[4].trim());
if (fields[5].trim().equalsIgnoreCase("YES")) {
isMarried[i]="YES";
} else {
isMarried[i] = "NO";
}
children[i] = Integer.parseInt(fields[6]);
if (fields[7].trim().equalsIgnoreCase("YES")) {
hasCar[i] = "YES";
} else {
hasCar[i] = "NO";
}
if (fields[8].trim().equalsIgnoreCase("YES")) {
saveAccount[i] = "YES";
} else {
saveAccount[i] = "NO";
}
if (fields[9].trim().equalsIgnoreCase("YES")) {
currentAccount[i] = "YES";
} else {
currentAccount[i] = "NO";
}
if (fields[10].trim().equalsIgnoreCase("YES")) {
mortgage[i] = "YES";
} else {
mortgage[i] = "NO";
}
if (fields[11].trim().equalsIgnoreCase("YES")) {
pep[i] = "YES";
} else {
pep[i] = "NO";
}
}
}
}
}
private Object toString(boolean bool, String string, String string2) {
// TODO Auto-generated method stub
return null;
}
@Override
public void printData() {
/** %10s %10d %10s %15s %10.2f %10s */
System.out.printf("%10s %10s %10s %15s %10s %10s ", "ID", "AGE", "SEX",
"REGION", "INCOME", "MORTGAGE");
int n = id.length;
if (n > 600) {
/**
* Regulating to display first 25 records only, if more exist
*/
n = 600;
}
for(int i=0;i System.out.printf("%10s %10d %10s %15s %10.2f %10s ", getId()[i], getAge()[i], getSex()[i], getRegion()[i], getIncome()[i], getMortgage()[i]); } } /** * Required getters and setters */ public String[] getId() { return id; } public void setId(String[] id) { this.id = id; } public int[] getAge() { return age; } public void setAge(int[] age) { this.age = age; } public Sex[] getSex() { return sex; } public void setSex(Sex[] sex) { this.sex = sex; } public Region[] getRegion() { return region; } public void setRegion(Region[] region) { this.region = region; } public double[] getIncome() { return income; } public void setIncome(double[] income) { this.income = income; } public String[] getIsMarried() { return isMarried; } public void setIsMarried(String[] isMarried) { this.isMarried = isMarried; } public int[] getChildren() { return children; } public void setChildren(int[] children) { this.children = children; } public String[] getHasCar() { return hasCar; } public void setHasCar(String[] hasCar) { this.hasCar = hasCar; } public String[] getSaveAccount() { return saveAccount; } public void setSaveAccount(String[] saveAccount) { this.saveAccount = saveAccount; } public String[] getCurrentAccount() { return currentAccount; } public void setCurrentAccount(String[] currentAccount) { this.currentAccount = currentAccount; } public String[] getMortgage() { return mortgage; } public void setMortgage(String[] mortgage) { this.mortgage = mortgage; } public String[] getPep() { return pep; } public void setPep(String[] pep) { this.pep = pep; } public ArrayList return input; } public void setInput(ArrayList this.input = input; } public static void main(String[] args) { /** * Defining a BankRecords object and reading,processing & printing data */ BankRecords records = new BankRecords(); records.readData(); records.processData(); records.printData(); } } /** * enumeration to represent the gender of the client */ enum Sex { MALE, FEMALE } /** * enumeration to represent the region of the client */ enum Region { INNER_CITY, TOWN, RURAL, SUBURBAN } Client.java public abstract class Client { /** * method prototype to read data */ public abstract void readData(); /** * method prototype to process data */ public abstract void processData(); /** * method prototype to print data */ public abstract void printData(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
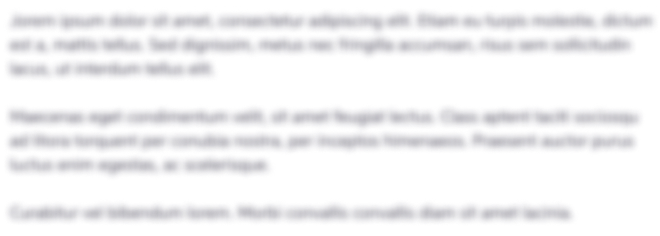
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started