Question
Please Solve using Java For the next lab, you will apply the knowledge that you have gained in class and through the readings to createa
Please Solve using Java
For the next lab, you will apply the knowledge that you have gained in class and through the readings to createa program using Object Oriented classes with inheritance.
In this lab you will continue to update the program that allows the user to create an estimate of the cost to build multiple tables.This will be a menu driven program that will continue until the user specifically states that they do not want any additional estimates or until they reach 10 estimates (whichever comes first).
Requirements:
This program will need to do the following:
The program needs to create a new class called Table -
oThe class has three instance variables
A string to hold the table shape
A string to hold the table material
A double to hold the area of the table
oThe class has two constructors
A default constructor that sets the strings to "NONE" and the numbers to 0.
A constructor that can accept values for all three instance variables
oThe class has a get and set method for all three instance variables
oThe class has a method that returns a double and calculates the cost of the table
The cost of the table is the area of the table * cost of the material selected
oThe class has a method that returns an integer and calculates the number of chairs the table can accommodate
If the area of the table is less than 3000 sq. inches, the table can accommodate 4 chairs
If the area is greater than 3000 sq. inches and less than or equal to 5000 sq. inches, the table can accommodate 6 chairs
If the area is greater than 5000 sq. inches, the table can accommodate 8 chairs
The program needs to create a new superclass called Customer and two subclasses called Commercial and Individual
- The superclass has three protected instance variables
- String to hold name
- String to hold email address
- String to hold phone number
- The superclass has one private variable
- String to hold account number
- In this class, the account number is ALWAYS set to "NONE"
The superclass has two constructors
oA default constructor that sets the strings to "NONE" and the numbers to 0.
oA constructor that can accept values for all three instance variables
- The superclass has a get and set method for all three instance variables
- The superclass has a toString() method
- The subclass Commercial has two private instance variables
- String to hold account number
- String to hold account type
The subclass Commercial has two constructors
oA default constructor that sets the strings to "NONE" and the numbers to 0.
oA constructor that can accept values for all three protected instance variables for the superclass and the two instance variables for the subclass.
- The subclass Commercial has a get and set method for both instance variables.NOTE: The getAccNum method must be an Overriden method
- The subclass Commercial has a toString() method that calls the super class toString and adds the account number and the account type to the output
- The subclass Individual has five private instance variables
- String to hold account number
- String to hold credit card type
- String to hold credit card number
- Integer to hold credit card expiration month
- Integer to hold credit card expiration year
The subclass Individual has two constructors
oA default constructor that sets the strings to "NONE" and the numbers to 0.
oA constructor that can accept values for all three instance protected variables of the superclass and the five instance variables for the subclass.
- The subclass Individual has a get and set method for all five instance variables.NOTE: The getAccNum method must be an Overriden method
- The subclass Individual must have a method that returns a String that has only the last four digits of the credit card number (see ADDITIONAL NOTES below for details on how to do this).
- The subclass Individual must have a method that returns a Boolean value that determines if the credit card enter is expired based on today's date (see ADDITIONAL NOTES below for details on how to do this).
- The subclass Individual has a toString() method that calls the super class toString and adds the account number, the credit card type, the last four digits of the cred card number, and a line that says if the credit card is expired or not.
The driver program needs to create an array of objects of type Table
The driver program needs to create an empty object of type Customer, type Commercial and type Individual.
The driver program should call a void method to display a welcome message. Include your name as the table builder.
The driver program must ask the user for the following information:
- Their name
- Their email address
- Their phone number
- Their customer type
- This is a menu driven request which must be checked for validity
- If the customer is a guest, ask for no more information
- If the customer is commercial
- Ask for account number and account type
- If the customer is an individual
- Ask for the account number, the credit card type, the credit card number, the expiration month and the expiration year; the month and year must be checked for validity
The driver program instantiates an object based on the customer type; this instantiation should use the constructor that take values for the full set of instance variables.
The driver program should call an integer method to display a menu of the shapes of the table.The menu should display Rectangular, Circular, Trapezoid and End Program
- The method must assure that the menu item entered is valid.If an invalid entry is made, the method should display an error message, redisplay the menu and allow for reentry
- Once the user enters a valid entry for a table, the method returns the entry to main
Inside main, the driver program must use a switch statement for the next steps
- If the user selects rectangular:
- The program must allow entry of the length and the width.The program must validate the entry (both sides must be > 0).
- The program calls an OVERLOADED method to calculate the total area (area = length * width). Once the calculation is done, the method returns the area to main.
- If the user selects circular:
- The program must allow entry of the diameter.The program must validate the entry (must be > 0).NOTE:The program must allow entry of the diameter.It is NOT correct to enter in the radius.In the real world, the radius cannot be accurately measured.
- The program calls an OVERLOADED method to calculate the total area (area = PI * radius * radius).Once the calculation is done, the method returns the area to main. NOTE: use the construct Math.PI to get the Java library version of PI
- If the user selects trapezoid (see area calculation below):
- The program must allow entry of the short base, the long base and the height.The program must validate all of the entries (must be > 0).
- The program will calculate the total area (area = (short+long)/2 * height). Once the calculation is done, the method returns the area to main.
Once the area is calculated, the code should call a method to ask the user what type of material they want to use:
- The method displays a menu with two options
- Option 1 is laminate - the laminate costs $0.125 per square inch
- Option 2 is oak - oak costs $0.25 per square inch
- The method must validate the entry of the choice.
- If the menu entry is invalid, the method must display an error message, redisplay the menu and allow reentry of the option
- Once a valid option is selected, the method returns the value to main.
Once the material option is returned, the driver program will instantiate the object of the array element.The program will then use the set methods to store the area, the shape, and the material for each table in the appropriate array object.
The program needs to keep track of the total number of estimates that have been requested.
The menu redisplays and allows a new estimate to be requested.
Once the user selects End Program or has reached the 10th entry, the program will display the customer information (based on the customer type) and then call a method to display an output report, a method to calculate the total cost of all of the tables and a third method to display the final estimation report.
- The method for the output report has the array of Table objects and the total tables estimated as the parameters.The method displays the following:
- A title
- The table number
- The shape and the calculated area of the table via get methods
- The type of the material for the table via a get method
- The cost of the table via the object method to calculate cost
- The number of chairs that the table can accommodate via the object method to calculate the number of chairs
- The method to determine the total cost of the tables will be a double method that accepts the object array and the total number of tables as the parameters
- The total cost method must use a regular for loop to calculate the total cost via calls to an object method for the table costs.
- The method for the final output report will display:
oIf the user only asked for one table, the output will display the final total estimate with table as singular
oIf the user asked for multiple tables, the output will display the number of tables (with tables plural) and the total cost
oIf the user did not request any estimates at all, the program display a message stating that.
oIf the user purchased more than $1000, the estimate has a discount of 5%.The method should call another method to determine the discount.If the discount is greater than 0, the method should display the discount amount and the final total.
oIf the user purchased more than $3000, the estimate has a discount of 10%.The method should call another method to determine the discount.If the discount is greater than 0, the method should display the discount amount and the final total.
oDisplay a final goodbye message and end the program.Remember to close out the Scanner object.
All numbers should be declared as public static final values; make sure that there are no hard-coded numbers anywhere in the code
Additional Requirements
The final product should have the main method and the following additional methods (10 total in addition to main):
- Void method to display the welcome message
- Integer method to display the menu and input the shape of the table
- Three, overloaded double methods to calculate the area
- Integer method to display the menu and input the material
- Void method to display the output report for the all of the tables in a single report
- A double method to calculate the total cost of all of the tables
- Void method to display the final estimate total with discount (if applicable) and a goodbye message
- Double method to calculate the discount
- An end-of-line comment must appear after each closing brace describing the block being closed.
Proper indentation must be used.
EXTRA CREDIT
For 5 points of extra credit, if the program encounters a credit card that is expired, display a warning message and require the user to update the expiration month and year to a valid month and year (based on the today's date).
ADDITIONAL NOTES
To get a substring of a String, Java uses the .substring() and .length() methods.For example, if the String alpha is "The alphabet".The code to extract the last three characters would be:
sTemp = alpha.substring(alpha.length() - 3);
This code gets the string starting from 3 characters from the end and assigns it to sTemp
This is used if the developer does not know the length of the string upfront.So, this would work on any string that is at least 3 characters long
To get the current date;
import java.time.LocalDate //import the local date library before the class
the following is all done inside the method to do the comparison:
int iMon, iYear//create variables for the month and year
LocalDate today = LocalDate.now();//fills today with the current date
iMon = today.getMonthValue();//call method to get integer Month
iYear = today.getYear();//call method to get integer Year
now the code can test to see if the date is in the past
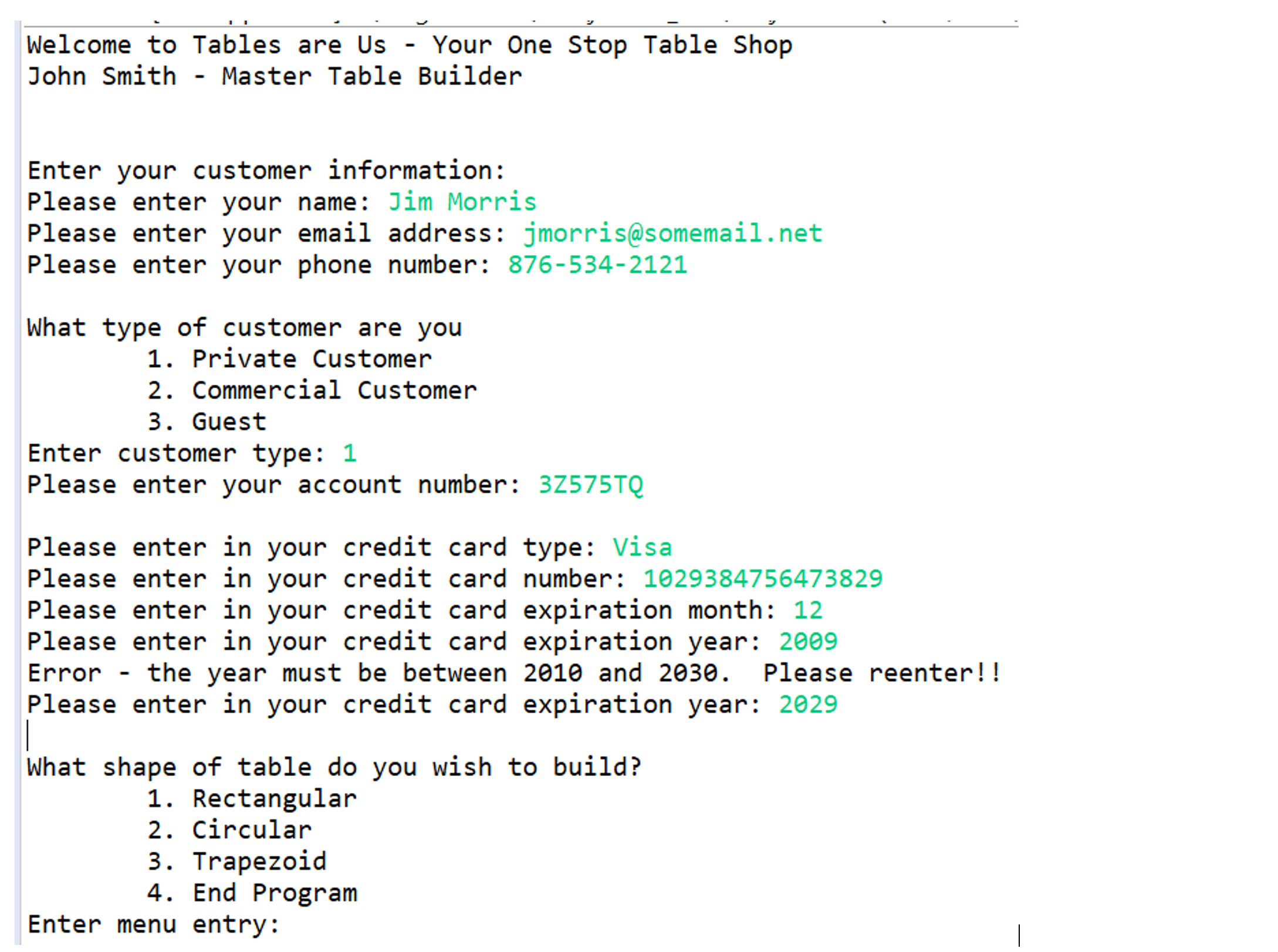
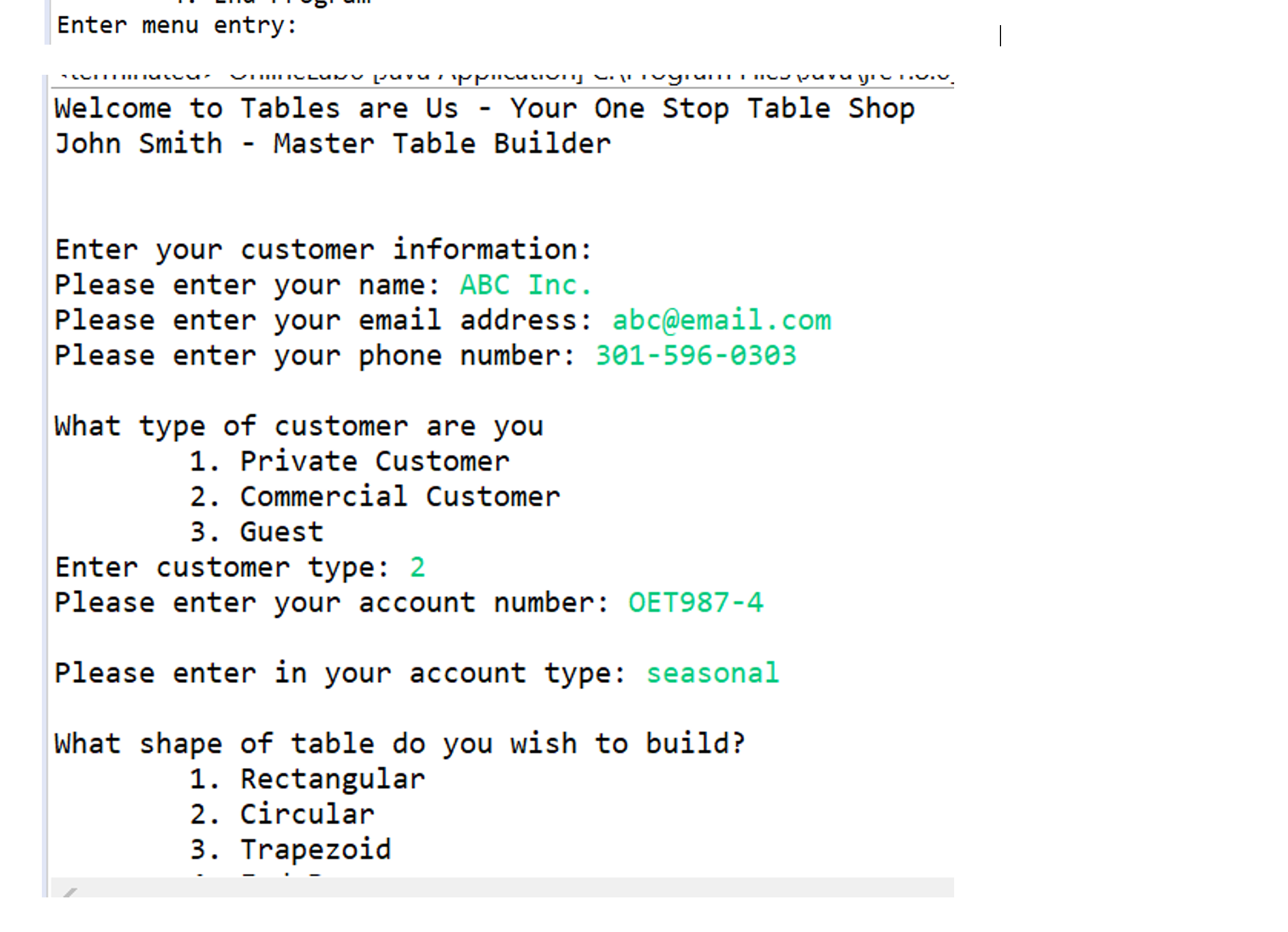
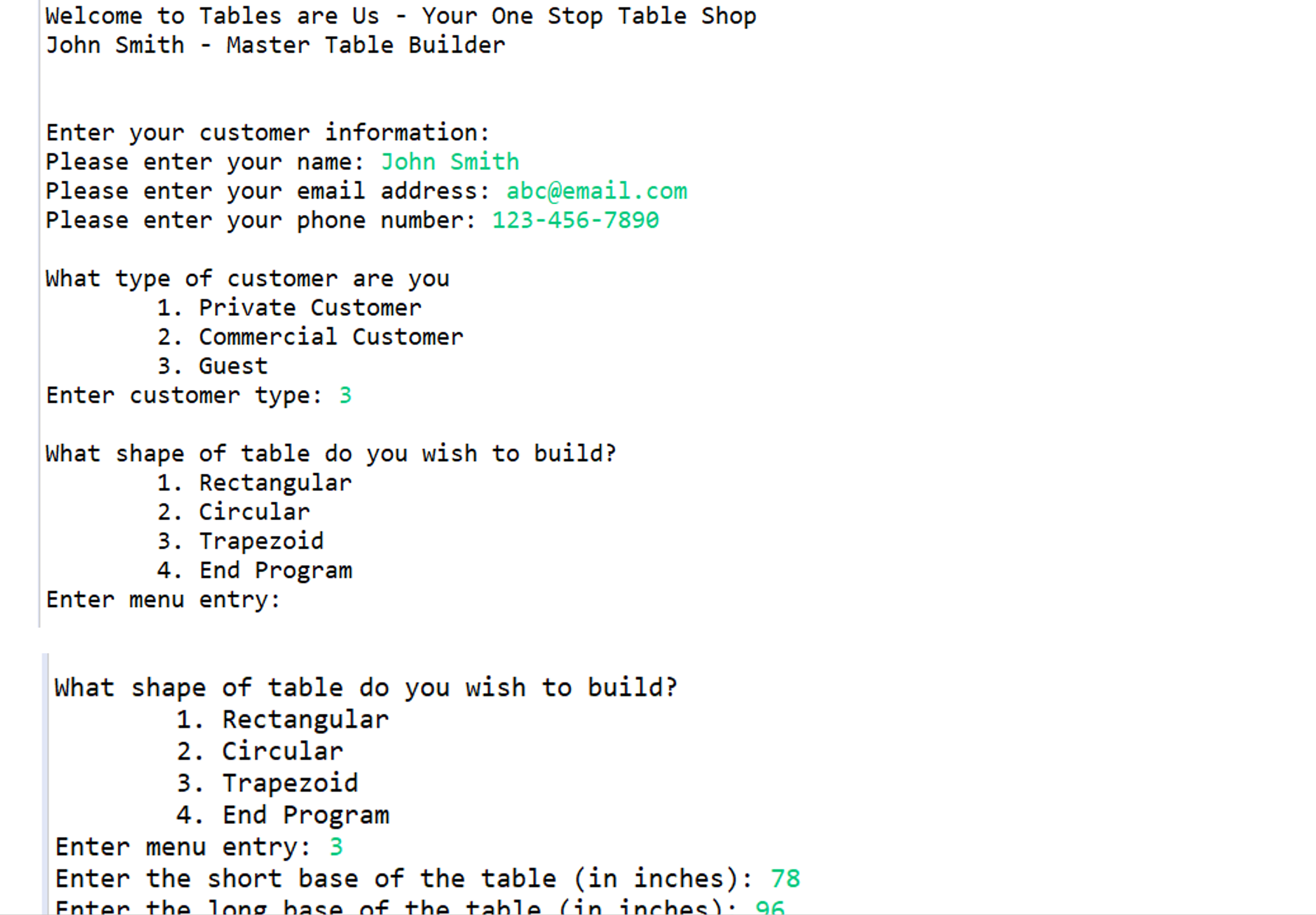
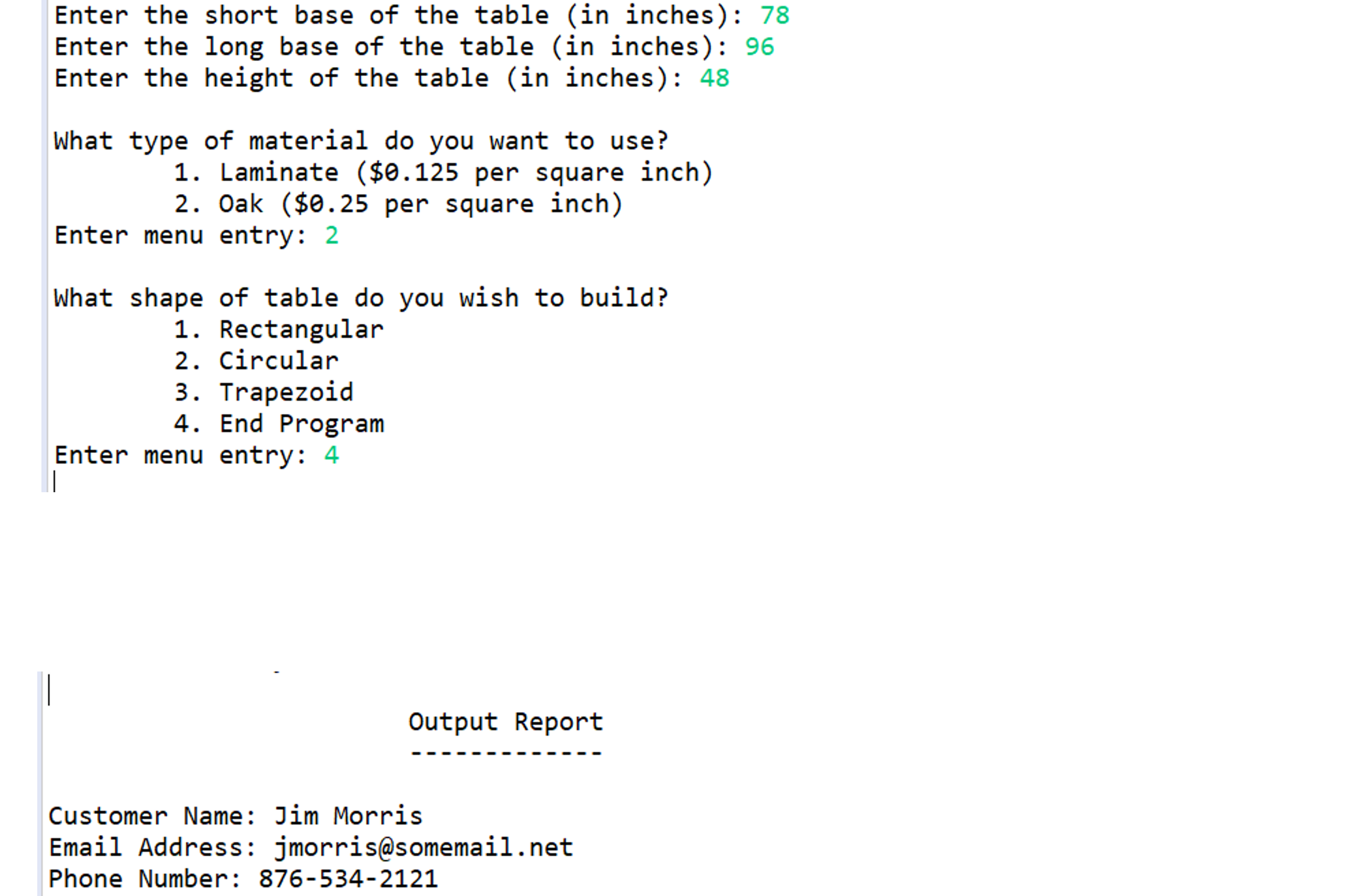
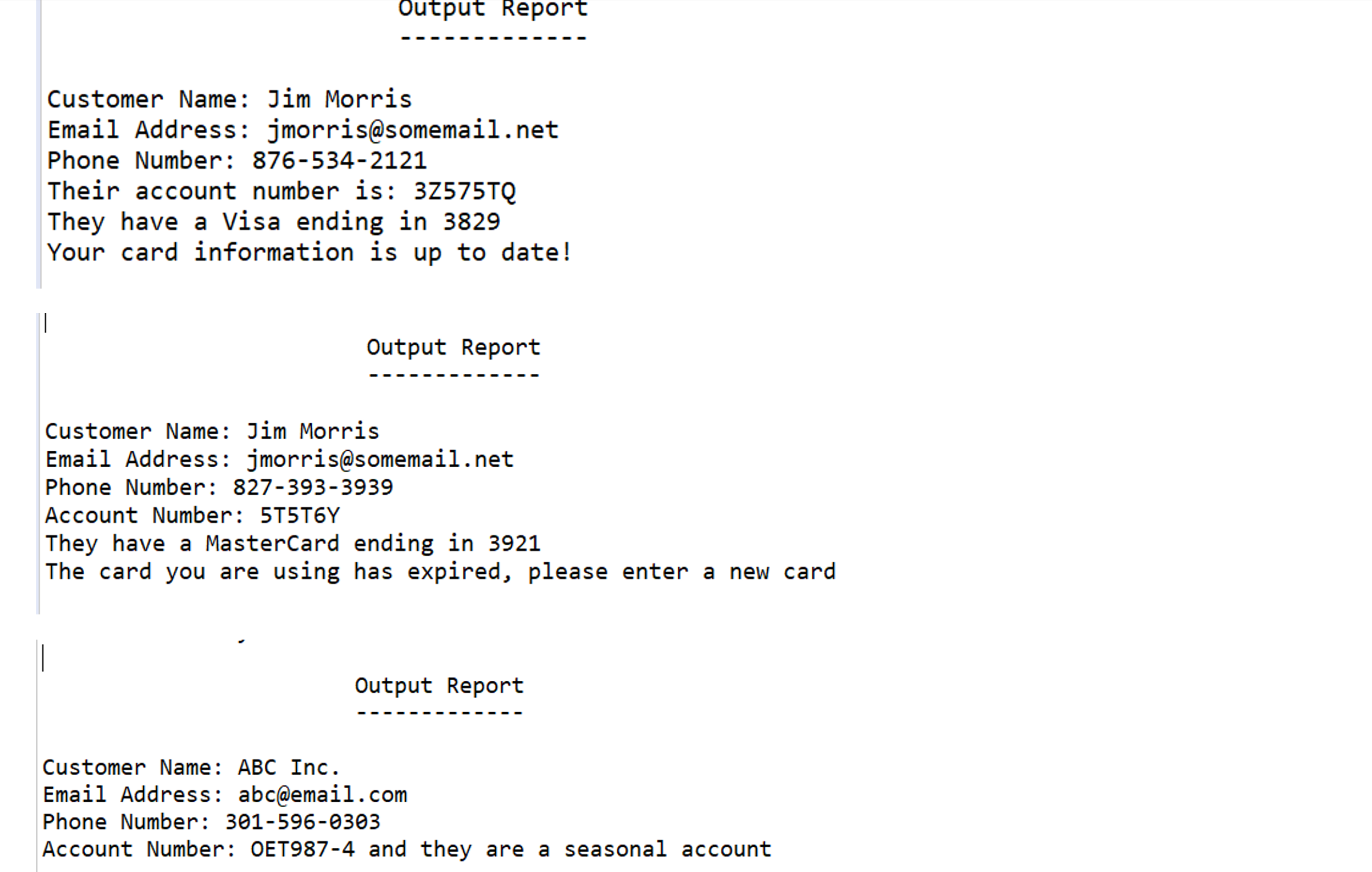
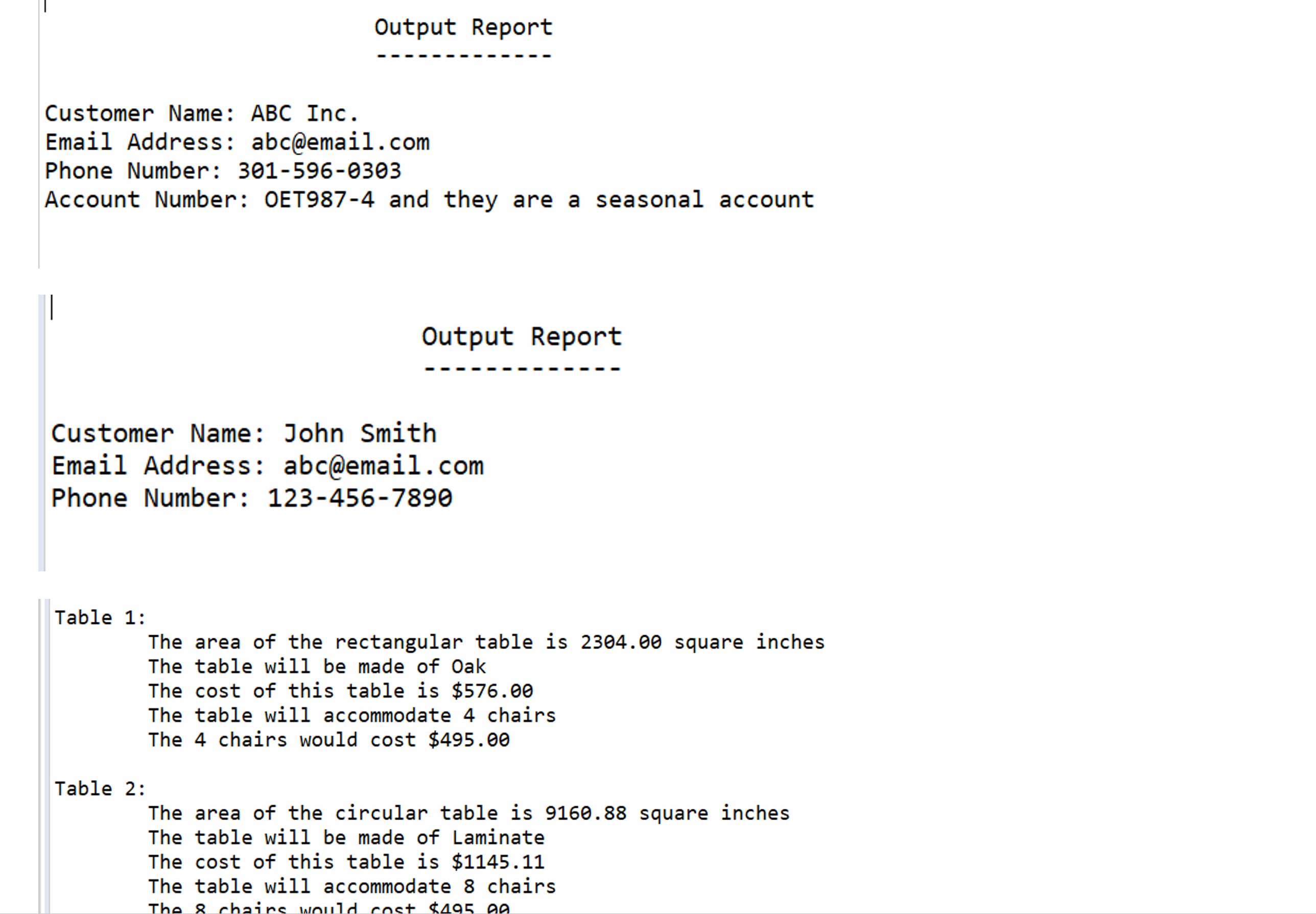
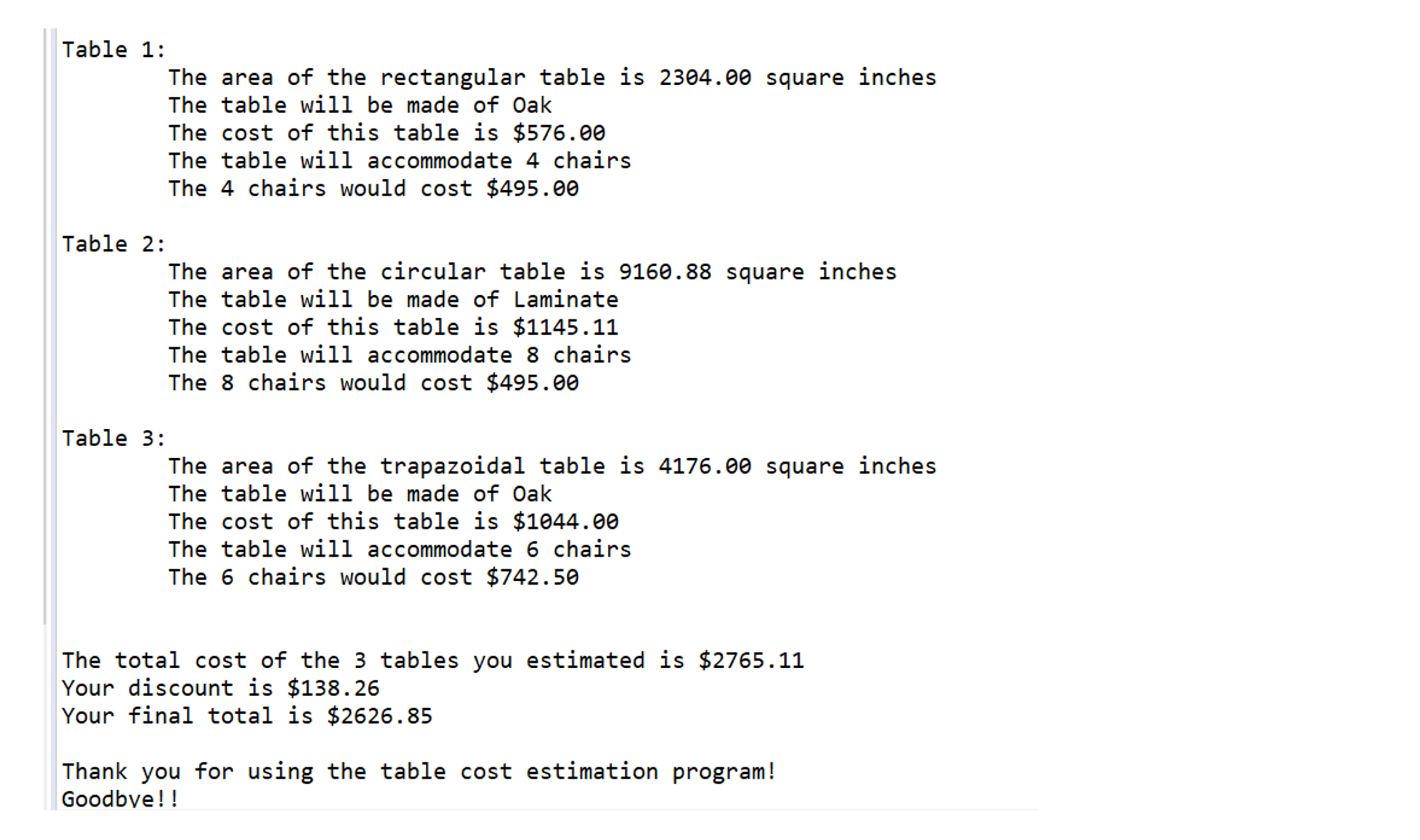
Step by Step Solution
There are 3 Steps involved in it
Step: 1
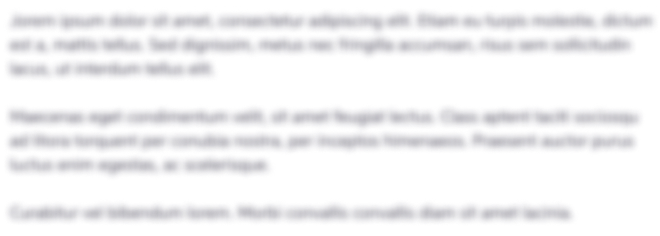
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started