Please use python language to do this thanks.
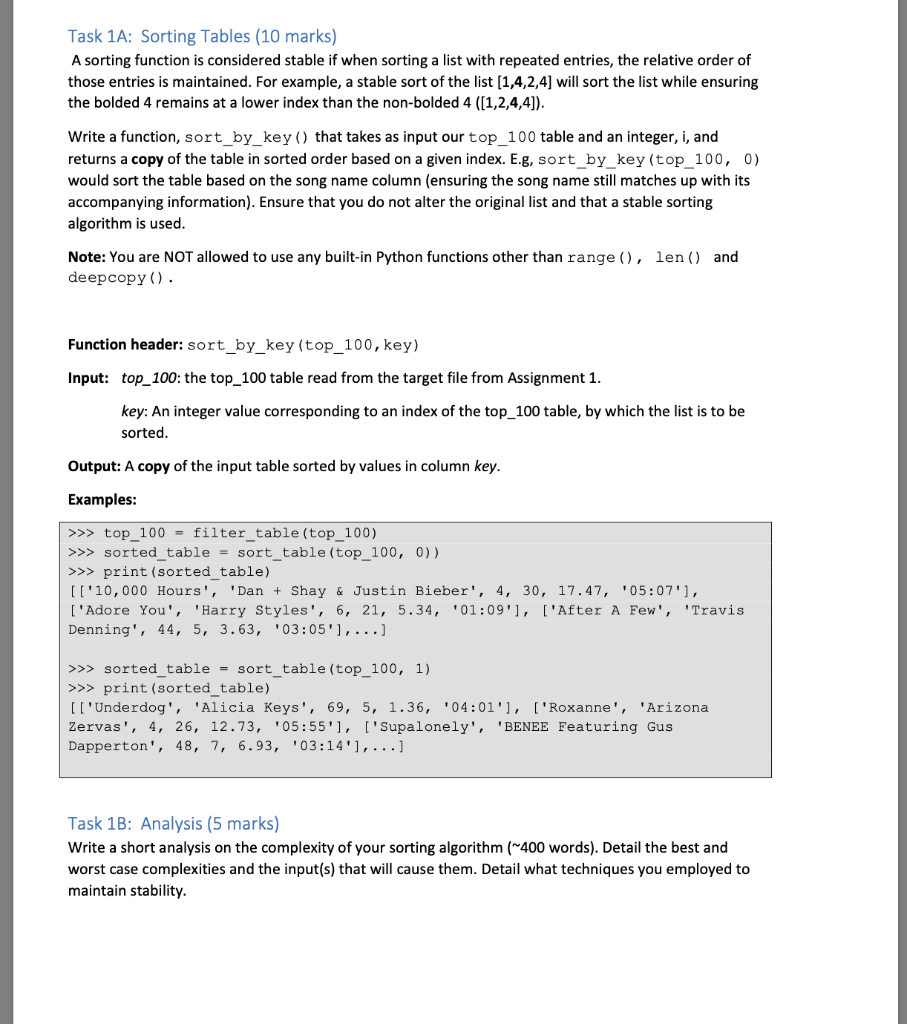
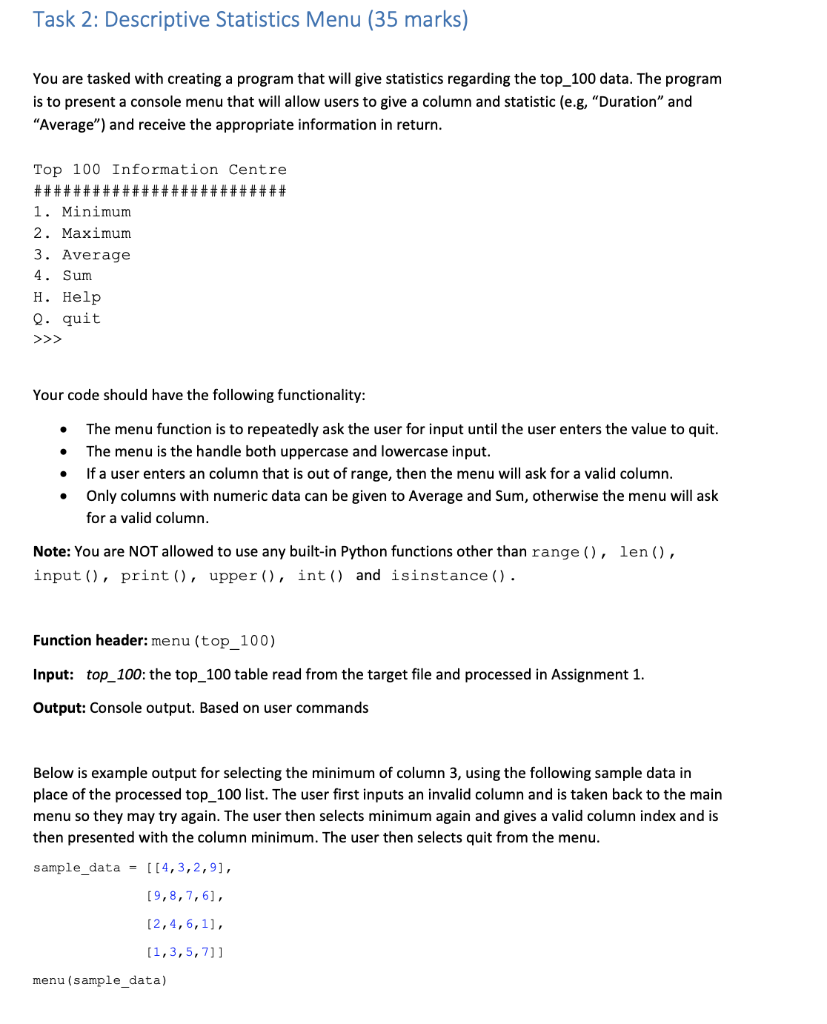
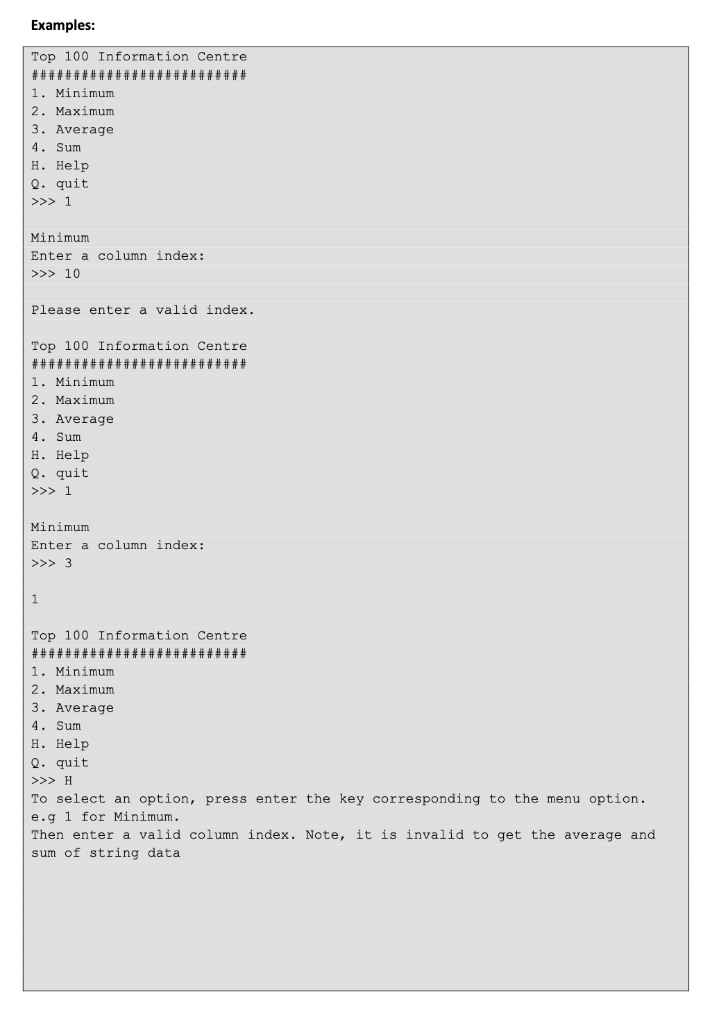
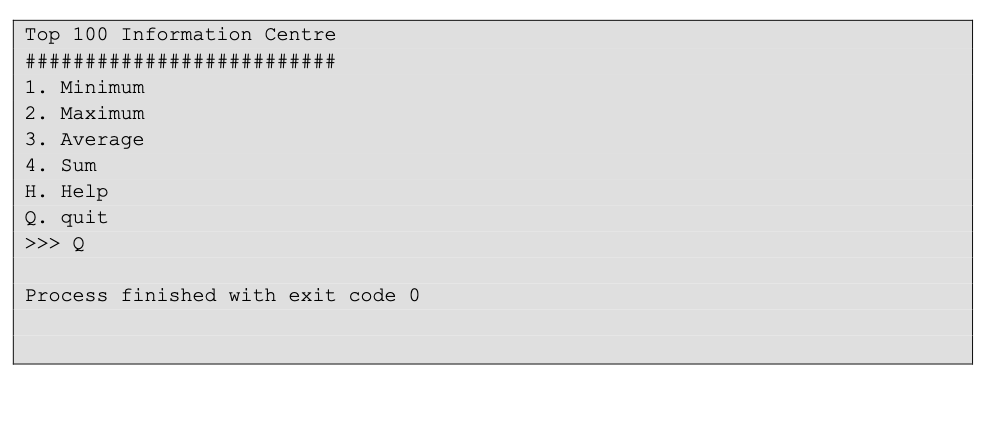
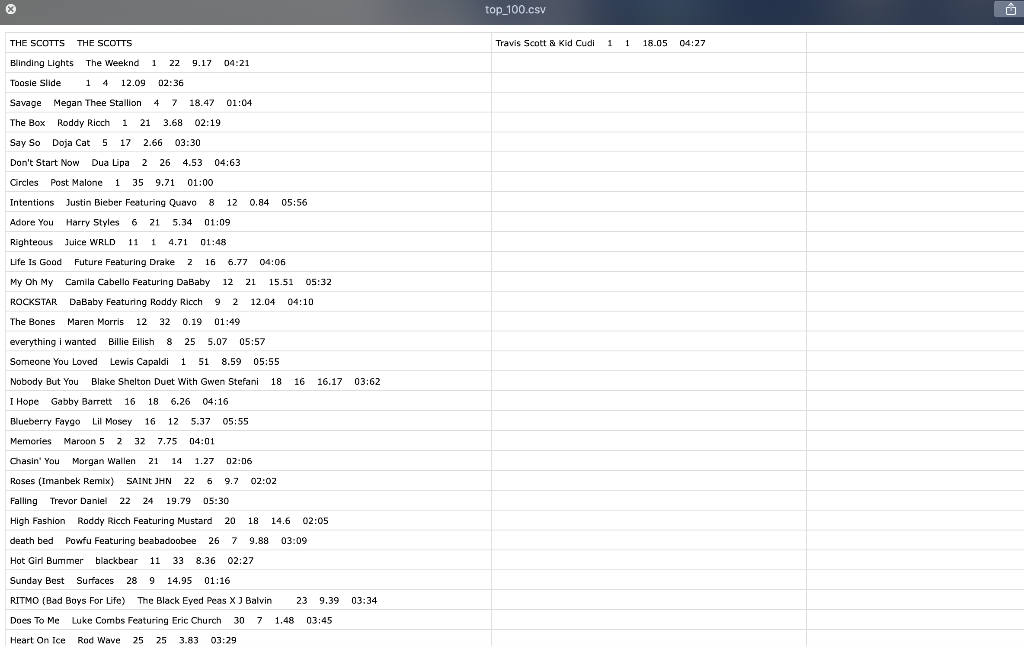
Task 1A: Sorting Tables (10 marks) A sorting function is considered stable if when sorting a list with repeated entries, the relative order of those entries is maintained. For example, a stable sort of the list (1,4,2,4] will sort the list while ensuring the bolded 4 remains at a lower index than the non-bolded 4 ([1,2,4,4]). Write a function, sort_by_key () that takes as input our top_100 table and an integer, i, and returns a copy of the table in sorted order based on a given index. E.g, sort_by_key (top_100, 0) would sort the table based on the song name column (ensuring the song name still matches up with its accompanying information). Ensure that you do not alter the original list and that a stable sorting algorithm is used. Note: You are NOT allowed to use any built-in Python functions other than range(), len() and deepcopy(). Function header: sort_by_key (top_100, key) Input: top_100: the top_100 table read from the target file from Assignment 1. key: An integer value corresponding to an index of the top_100 table, by which the list is to be sorted. Output: A copy of the input table sorted by values in column key. Examples: >>> top_100 = filter_table (top_100) >>> sorted_table = sort_table (top_100, 0)) >>> print (sorted_table) [['10,000 Hours', 'Dan + Shay & Justin Bieber', 4, 30, 17.47, 05:07'], ['Adore You', 'Harry Styles', 6, 21, 5.34, '01:09'], ['After A Few', 'Travis Denning', 44, 5, 3.63, '03:05'],...] >>> sorted_table = sort_table (top_100, 1) >>> print (sorted_table) [['Underdog', 'Alicia Keys', 69, 5, 1.36, '04:01'], ['Roxanne', 'Arizona Zervas', 4, 26, 12.73, '05:55'], ['Supalonely', 'BENEE Featuring Gus Dapperton', 48, 7, 6.93, '03:14'],...] Task 1B: Analysis (5 marks) Write a short analysis on the complexity of your sorting algorithm (-400 words). Detail the best and worst case complexities and the input(s) that will cause them. Detail what techniques you employed to maintain stability. Task 2: Descriptive Statistics Menu (35 marks) You are tasked with creating a program that will give statistics regarding the top_100 data. The program is to present a console menu that will allow users to give a column and statistic (e.g, "Duration" and "Average") and receive the appropriate information in return. Top 100 Information Centre ######## # ## ## # # # # # # # 1. Minimum 2. Maximum 3. Average 4. Sum H. Help Q. quit >>> Your code should have the following functionality: . The menu function is to repeatedly ask the user for input until the user enters the value to quit. The menu is the handle both uppercase and lowercase input. If a user enters an column that is out of range, then the menu will ask for a valid column. Only columns with numeric data can be given to Average and Sum, otherwise the menu will ask for a valid column. . Note: You are NOT allowed to use any built-in Python functions other than range(), len(), input(), print (), upper(), int() and isinstance(). Function header:menu (top_100) Input: top_100: the top_100 table read from the target file and processed in Assignment 1. Output: Console output. Based on user commands Below is example output for selecting the minimum of column 3, using the following sample data in place of the processed top_100 list. The user first inputs an invalid column and is taken back to the main menu so they may try again. The user then selects minimum again and gives a valid column index and is then presented with the column minimum. The user then selects quit from the menu. sample_data - [(4,3,2,9), [9,8,7,6], [2,4,6,1], [1,3,5,7]] menu (sample_data) Examples: Top 100 Information Centre ######### ######### 1. Minimum 2. Maximum 3. Average 4. Sum H. Help Q. quit >>> 1 Minimum Enter a column index: >>> 10 Please enter a valid index. Top 100 Information Centre ########################## 1. Minimum 2. Maximum 3. Average 4. Sum H. Help Q. quit >>> 1 Minimum Enter a column index: >>> 3 1 Top 100 Information Centre ######## ############ 1. Minimum 2. Maximum 3. Average 4. Sum H. Help Q. quit >>> H To select an option, press enter the key corresponding to the menu option. e.g 1 for Minimum. Then enter a valid column index. Note, it is invalid to get the average and sum of string data Top 100 Information Centre ## ## 1. Minimum 2. Maximum 3. Average 4. Sum H. Help Q. quit >>> Q Process finished with exit code 0 top_100.csv THE SCOTTS THE SCOTTS Travis Scott & Kid Cudi 1 1 1 18.05 04:27 Blinding Lights The Weeknd 1 22 9.17 04:21 Toosie Slide 1 4 12.09 02:36 Savage Megan Thee Stallion 4 7 18.47 01:04 The Box Roddy Ricch 1 21 3.68 02:19 Say So Doja Cat 5 17 2.66 03:30 26 4.53 04:63 Don't Start Now Dua Lipa 2 Circles Post Malone 1 35 9.71 01:00 Intentions Justin Bieber Featuring Quavo 8 12 0.84 05:56 01:09 Adore You Harry Styles 6 21 5.34 Righteous Juice WRLD 11 1 4.71 01:48 Life Is Good Future Featuring Drake 2 16 6.77 04:06 My Oh My Camila Cabello Featuring DaBaby 12 21 15.51 05:32 ROCKSTAR DaBaby Featuring Roddy Ricch 92 12.04 04:10 16 16.17 03:62 The Bones Maren Morris 12 32 0.19 01:49 everything i wanted Billie Eilish 8 25 5.07 05:57 Someone You Loved Lewis Capaldi 1 51 8.59 05:55 Nobody But You Blake Shelton Duet With Gwen Stefani 18 I Hope Gabby Barrett 16 18 6.26 04:16 Blueberry Faygo Lil Mosey 16 12 5.37 05:55 Memories Maroon 5 2 32 7.75 04:01 Chasin' you Morgan Wallen 21 14 1.27 02:06 Roses (Imanbek Remix) SAINT JHN 22 6 9.7 02:02 Falling Trevor Daniel 22 24 19.79 05:30 High Fashion Roddy Ricch Featuring Mustard 20 18 14.6 02:05 death bed Powfu Featuring beabadoobee 26 7 9.88 03:09 Hot Girl Bummer blackbear 11 33 8.36 02:27 Sunday Best Surfaces 28 9 14.95 01:16 RITMO (Bad Boys For Life) The Black Eyed Peas X J Balvin 23 9.39 03:34 Does To Me Luke Combs Featuring Eric Church 307 1.48 03:45 Heart On Ice Rod Wave 25 25 3.83 03:29