Question
Please who can help me with this question in Java Use the LinkedBinaryTree below as a super class to implement class BinarySearchTree ONLY for sorting
Please who can help me with this question in Java
Use the LinkedBinaryTree below as a super class to implement class BinarySearchTree ONLY for sorting purposes, such as sorting integers, double numbers and arrays of strings.
Input: A set of random integers generated by a random generator. Display all unsorted numbers
Output: All the sorted integers.
Thank you
************************************************
import java.util.ArrayList; import java.util.Random; public class LinkedBinaryTreeimplements BinaryTree {
public interface BTPosition
/** Returns the element stored at this position */ // public E element(); /** Sets the element stored at this position */ public void setElement(E o); /** Returns the left child of this position */ public BTPosition
protected static class BTNode implements BTPosition {
private E element;// element stored at this node private BTPosition left, right, parent; // adjacent nodes /** Main constructor */ public BTNode(){} public BTNode(E element, BTPosition parent, BTPosition left, BTPosition right) { setElement(element); setParent(parent); setLeft(left); setRight(right); } /** Returns the element stored at this position */ public E element() { return element; }
/** Sets the element stored at this position */ public void setElement(E o) { element=o; }
/** Returns the left child of this position */ public BTPosition getLeft() { return left; }
/** Sets the left child of this position */ public void setLeft(BTPosition v) { left=v; }
/** Returns the right child of this position */ public BTPosition getRight() { return right; }
/** Sets the right child of this position */ public void setRight(BTPosition v) { right=v; }
/** Returns the parent of this position */ public BTPosition getParent() { return parent; }
/** Sets the parent of this position */ public void setParent(BTPosition v) { parent=v; }
}
protected BTPosition root; protected int size;
public LinkedBinaryTree() { root=null; size=0;
} public int size(){return size;} public boolean isEmpty(){return size()==0;} public boolean isInternal(Positionv) { checkPosition(v); return (hasLeft(v)|| hasRight(v));
} public boolean isExternal(Positionv) { checkPosition(v); return(!isInternal(v)); } public boolean isRoot(Positionv) { checkPosition(v); return(v==root()); } public boolean hasLeft(Positionv) { BTPositionvv=checkPosition(v); return(vv.getLeft()!=null); } public boolean hasRight(Positionv) { BTPosition vv= checkPosition(v); return (vv.getRight()!=null); } public Position root() { if(root==null)throw new IllegalArgumentException("The tree is empty"); return root; } public Position left(Positionv) { BTPositionvv= checkPosition(v); PositionleftPos=vv.getLeft(); if(leftPos==null)throw new IllegalArgumentException("Has no Left child"); return leftPos; } public Position right(Positionv) { BTPositionvv= checkPosition(v); PositionrightPos=vv.getRight(); if(rightPos==null)throw new IllegalArgumentException("Has no right child"); return rightPos; } public Position parent(Positionv) { BTPositionvv= checkPosition(v); PositionparentPos= vv.getParent(); if (parentPos==null) return null; return parentPos; } public Iterable> children(Positionv) { ArrayList> children= new ArrayList>(); if(hasLeft(v)) children.add(left(v)); if(hasRight(v)) children.add(right(v)); return children;
} public Iterable>positions() { ArrayList>positions= new ArrayList>(); if(size!=0) inorderPositions(root(),positions); return positions; } public Iterable iterator() { Iterable>positions=positions(); ArrayListelements= new ArrayList(); for(Positionpos:positions) elements.add(pos.element()); return elements;
} public Position sibling(Positionv) { Positionparent=validate(v); parent=parent(v); if(parent==null) return null; if(v==left(parent)) return right(parent); else return left(parent); } /* BTPositionvv = checkPosition(v); PositionparentPos=vv.getParent(); if(parentPos!=null) { BTPositionsiblingPos; BTPositionleftPos= parentPos.getLeft(); //BTPositionrightPos=parentPos.getRight(); if(leftPos==vv) siblingPos=parentPos.getRight(); else siblingPos=parentPos.getLeft(); if(siblingPos!=null) return siblingPos; } throw new IllegalArgumentException("No sibling");
}*/ public E replace(Positionv, E o) { BTPositionvv= validate(v); E temp= v.element(); vv.setElement(o); return temp;
} protected BTPosition checkPosition(Positionv) { if(v==null||!(v instanceof BTPosition)) throw new IllegalArgumentException("invalid position"); return (BTPosition)v; } protected BTPosition createNode(E element, BTPositionparent,BTPosition left,BTPosition right) { return new BTNode(element, parent, left, right); } protected void inorderPositions(Positionv, ArrayList>pos) { if(hasLeft(v)) inorderPositions(left(v),pos); pos.add(v); if(hasRight(v)) inorderPositions(right(v),pos); } public int depth(TreeT, Positionv ) { if(T.isRoot(v)) return 0; else return 1+ depth(T,T.parent(v)); } public int height1(Tree T) { int h=0; for(Positionv: T.positions()) { if(T.isExternal(v)) h=Math.max(h,depth(T,v)); } return h; } public PositionaddRoot(E e)throws IllegalStateException { if(!isEmpty()) throw new IllegalStateException("Tree has got a root already"); size=1; root=createNode(e,null,null,null); return root;
} public Position insertLeft(Positionv, E e)throws IllegalArgumentException { BTPosition vv= validate(v); if(vv.getLeft()!=null) throw new IllegalArgumentException(" V has already a left child ");
BTPositionchild=createNode(e ,vv,null,null); vv.setLeft(child); size++; return child; } public Position insertRight(Positionv,E e)throws IllegalArgumentException { BTPositionvv= validate(v); if(vv.getRight()!=null) throw new IllegalArgumentException("V has got a child already "); BTPosition child=createNode(e,vv, null,null); vv.setRight(child); size++; return child; } public E remove(Positionv)throws IllegalStateException { BTPositionvv=validate(v); BTPosition leftPos=vv.getLeft(); BTPosition rightPos=vv.getRight(); if(leftPos!=null&&rightPos!=null) throw new IllegalStateException("Cannot remove a node that has 2 children"); BTPositionc; if(leftPos!=null) c=leftPos; if(rightPos!=null) c=rightPos; else c=null; if(vv==root) { if(c!=null) c.setParent(null); root=c; } else { BTPositionp=vv.getParent(); if(vv==p.getLeft()) p.setLeft(c); else p.setRight(c); if(c!=null) c.setParent(p); } size--; return v.element(); } public void attach(Positionv, BinaryTreeT1,BinaryTreeT2) throws IllegalArgumentException { BTPositionvv=validate(v); if(isInternal(v)) throw new IllegalArgumentException("cannot attach from an internal node"); if(T1.isEmpty()) { BTPosition t1=validate(T1.root()); vv.setLeft(t1); t1.setParent(vv); } if(T2.isEmpty()) { BTPositiont2=checkPosition(T2.root()); vv.setRight(t2); t2.setParent(vv); }
} public BTPosition validate(Positionp)throws IllegalArgumentException { if(!(p instanceof BTPosition)) throw new IllegalArgumentException("Not valid Position Type."); BTPosition v=(BTPosition)p; if(v.getParent()==v) throw new IllegalArgumentException("p is no longer in the tree"); return v;
} /*public static void main(String [] args) { LinkedBinaryTree T= new LinkedBinaryTree(); Random rand= new Random(); int n=10; int j; if(T.isEmpty()) T.addRoot(rand.nextInt(1000)); System.out.println("the root of the tree is:"+T.root().element()); Positionp=T.root(); for(int i=0;i<=n;i++) { j=rand.nextInt(100); if(j%2==0) { T.insertLeft(p,j); p=T.left(p); } else { T.insertRight(p,j); p=T.right(p);
} } System.out.println("the size of the tree is "+ T.size()); System.out.println("height1 of the tree is "+T.height1(T)); System.out.println("depth of the tree is "+T.depth(T,T.parent(p))); System.out.println("Print all the elements in the tree:"); int count=0; for(Integer i:T.iterator()) { count++; System.out.println("["+i+","+count+"]");
}*/
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
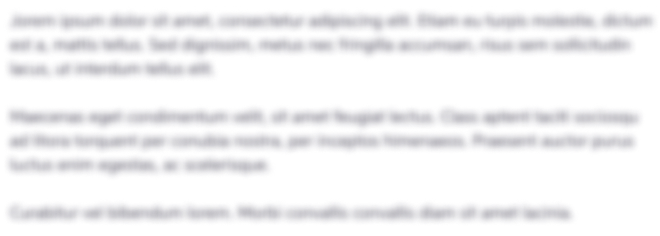
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started