Question
Please write a code in Java where it says your // your code here to run the code smoothly. Import statements are not allowed to
Please write a code in Java where it says your // your code here to run the code smoothly. Import statements are not allowed to be used.
_ A Java method is a collection of statements that are grouped together to perform an operation. Some languages also call this operation a Function. When you call the System.out.println() method, for example, the system actually executes several statements in order to display a message on the console. Please write the code according to functions assigned for the assignment.
You can use these functions in the assignment.
Modifier and Type | Method | |
---|---|---|
static int | bestGuess(int firstGuess, int secondGuess, int answer) | |
static double | bigProduct(double firstNumber, double secondNumber) | |
static int | bigSum(int firstNumber, int secondNumber) | |
static int | countWord(java.lang.String word, java.lang.String text) | |
static boolean | hasBlank(java.lang.String original) | |
static boolean | hasWord(java.lang.String word, java.lang.String text) | |
static boolean | isCapital(java.lang.String original) | |
static boolean | isFloat(java.lang.String firstString) | |
static boolean | isInt(java.lang.String firstString) | |
static int | topSum(int firstNumber, int secondNumber, int thirdNumber, int fourthNumber) |
/** This exercise involves implementing several methods. Stubs for each method with documentation are given here. It is your task to fill out the code in each method body so that it runs correctly according to the documentation.
You can run this file by compiling TestMethods1.java It will call this program and run it, validating the test you choose.
Example inputs with output are provided in the comments before each method. At a minimum, your solutions must pass these tests. My version of TestMethods1 contains more than the examples given or those cases in your version of TestMethods1. Therefore, hardcoding the answers will not pass. **/
//imports are not allowed in this exercise.
public class ExerciseMethods1{
/** Boolean function returns true if the given string begins with a capital letter.
isCapital("Robert") returns true isCapital("CSCE111") returns true isCapital("bicycle") returns false isCapital("42") returns false isCapital("") returns false isCapital(" home") returns false isCapital("TAMU")) returns true @param original String, @return true if the first letter is a capital letter. False otherwise. */ public static boolean isCapital(String original) { //your code here return false; }//end isCapital
/** Int function that returns true if the string has a blank.
hasBlank("Robert Lightfoot") returns true hasBlank("Today is the best ever.") returns true hasBlank("abcdefghijklmnopqrstuvwxyz") returns false hasBlank("2590") returns false hasBlank("") returns false hasBlank(" home") returns true hasBlank("TAMU")) returns false @param original String, @return true if a blank is contained. False otherwise. */ public static boolean hasBlank(String original) { //your code here return false; }// end hasBlank
/** isInt function returns true if the string is an integer. false otherwise
isInt("12564") returns true isInt("-567") returns true isInt("23.9") returns false isInt("twenty two") returns false @param firstString String, @return true only if the input string only contains digits 0-9 and/or a leading "-". */ public static boolean isInt(String firstString) { //your code here return false; }// end isInt
/** isFloat function returns true if the string is a floating point number. false otherwise Note, like doubles, integers are acceptable.
isFloat("12564") returns true isFloat("-567") returns true isFloat("23.9") returns true isFloat("-56.7") returns true isFloat("-567h") returns false isFloat("twenty two") returns false @param firstString String, @return true only if the input string only contains an optional leading "-"digits 0-9 and/or a "." followed by optional trailing digits. */ public static boolean isFloat(String firstString) { //your code here return false; }// end isFloat
/** If the word is contained in the text, return true. Otherwise return false. Capitalization is not cosidered. Consider the whole word, "The" is not contained in "There are no more bananas."
hasWord("Nice", "Today is a nice day.") returns true hasWord("The", "There are no more bananas!") returns false // hasWord("bananas", "There are no more bananas?") returns true hasWord("any-string", "I can find any-string any-where.") returns true hasWord("I", "I can find any-string any-where.") returns true hasWord("Army", "I have decided to join the Navy.") returns false myFunctions.hasWord(" ", "") returns false @param word String, @param text String, @return true if the word in contained in the text. */ public static boolean hasWord(String word, String text) { //your code here return false; }//end hasWord
/** If the word is contained in the text, return the number of times it is contained. Otherwise return 0. If there is no word, or no text, return -1. Look at whole words, "the" is not contained in "these". Capitalization is not cosidered.
hasWord("the", "The weather outside is frightful.") returns 1 hasWord("any-where", "I can find any-string any-where.") returns 1 hasWord("farm","Old McDonald had a farm. EI EI O. And on that farm he had a cow.") returns 2 hasWord("do","I love dogs, do you?") returns 1 hasWord("Army", "I have decided to join the Navy.") returns 0 hasWord("","This is the fist line of over 1000 lines.") returns -1 @param word String, @param text String, @return returns the count of word in text, or -1 if either is null. */ public static int countWord(String word, String text) { //your code here return -1; }//end countWord
/** Given three integers, return whichever is closer to the answer. In the event of a tie return 0. Note that Math.abs(n) returns the absolute value of a number.
bestGuess(8, 13, 10) returns 8 bestGuess(13, 8, 10) returns 8 bestGuess(13, 7, 10) returns 0 @param firstGuess int number, @param secondGuess int number, @param answer int number, @return of two int guess values, whichever is closer to the answer. */ public static int bestGuess(int firstGuess, int secondGuess, int answer) { //your code here return 0; }//end bestGuess
/** Method topSum returns the sum of the largest two numbers sent.
topSum(3, 3, 3, 3) returns 6 topSum(1, 10, 45, 2) returns 55 topSum(-6, 2, -45, -8) returns -4 topSum(3, 113, 2, 200) returns 313 @param firstNumber int number, @param secondNumber int number, @param thirdNumber int number, @param fourthNumber int number, @return the sum of the largest two numbers sent. */ public static int topSum(int firstNumber, int secondNumber, int thirdNumber, int fourthNumber ) { //your code here return 0; }// end topSum
/** Method bigSum returns the sum of all numbers between the first number and the second number inclusive. Works in either direction.
bigSum(3, 3) returns 6 bigSum(1, 10) returns 55 bigSum(-6, 1) returns -20 bigSum(5, 3) returns 12 @param firstNumber int number, @param secondNumber int number, @return the sum of the numbers from the first to the second. */ public static int bigSum(int firstNumber, int secondNumber) { //Your code here return 0; }//end bigSum
/** Method bigProduct returns the product of all numbers between the first number and the second number inclusive. Works in either direction.
bigProduct(3, 3) returns 9 bigProduct(1, 10) returns 362880 bigProduct(-6, 1) returns 0 bigProduct(5, 3) returns 60 @param firstNumber double number, @param secondNumber double number, @return the product of the numbers from the first to the second. */ public static double bigProduct(double firstNumber, double secondNumber) { //Your code here return 0.0; }//end bigProduct
}//end ExerciseMethods1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
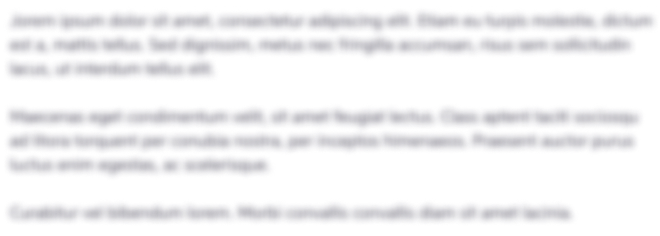
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started