Question
Please write in C++ using the code below. Thank you! -------------------------- pi.cpp -------------------------- #include pi.h #include #include Point::Point(double x, double y) : x(x), y(y) {}
Please write in C++ using the code below. Thank you!
--------------------------
pi.cpp
--------------------------
#include "pi.h"
#include
#include
Point::Point(double x, double y) : x(x), y(y) {}
double Point::distanceFromOrigin() { return sqrt(x * x + y * y); }
Point Point::uniformRandomPoint() {
double x = static_cast
double y = static_cast
return Point(x, y);
}
// F: implement this function
double approximatePi(int numDarts) {
int numInside = 0; // count how many darts landed inside the unit circle
// The ratio of the area of the unit circle to the area of the enclosing
// square is /4. That means numInside / numDarts is an
// approximation of /4. Use this fact to return an approximation of
// .
// F: this is just a stub return statement
return -42;
}
---------------------
pi.h
---------------------
#ifndef PI_H
#define PI_H
class Point {
public:
// gets a random point with x and y between 0 and 1
static Point uniformRandomPoint();
// Point constructor
Point(double x, double y);
// gets the distance that a current point object is from (0, 0)
double distanceFromOrigin();
private:
double x;
double y;
};
// Approximates by throwing numDarts darts
double approximatePi(int numDarts);
#endif /* PI_H */
-----------------------
testPi.cpp
-----------------------
#include
#include
#include
#include
#include
#include "pi.h"
#include "testing.h"
using namespace std;
void testPi();
int main() {
srand(time(0));
cout
testPi();
return 0;
}
void testPi() {
vector
for (int i = 0; i
approximations.push_back(approximatePi(1'000'000));
}
std::transform(approximations.begin(), approximations.end(),
approximations.begin(),
[](double d) { return fabs(M_PI - d); });
bool differencesAreSmall =
std::all_of(approximations.begin(), approximations.end(),
[](double d) { return d
bool answerIsntAlwaysTheSame =
std::min(approximations.begin(), approximations.end()) !=
std::max(approximations.begin(), approximations.end());
assertTrue(differencesAreSmall && answerIsntAlwaysTheSame, "pi tests");
}
Look at pi.h. You will be using the Point class (that I've already implemented for you) to implement the approximatePi function at the bottom. You are going to randomly throw numDarts onto a square with an inscribed circle centered at (0,0): Several of your darts will land inside the circle, but some will miss. Surprisingly, this game tells us something about it! (0,0) = The darts approximate area, and the ratio of the area of the circle to the area of the square is 1/4. So, if you divide the number of darts that landed inside the circle by the total number of darts thrown, you get an approximation of 11/4! All that's left is to turn that into an approximation of T. Area of circle: 11= Area of square: 22 = 4 We're going to cheat and work only in the first quadrant (where the x and y coordinates are always nonnegative), but the ratio remains the same. The Point class represents the coordinates where the dart landed. Do you see what method you might use to determine if the dart landed inside the circle? Implement the approximatePi function in pi.cpp. You can then run the tests in testPi.cpp using make testPi to compile and ./testPi to runStep by Step Solution
There are 3 Steps involved in it
Step: 1
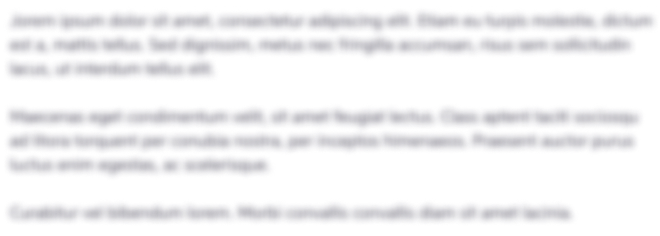
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started