Question
PLEASE WRITE IN PYHTON I need help with 5 and 6 only, i will include my code for parts 1-4 at the end. PLease try
PLEASE WRITE IN PYHTON
I need help with 5 and 6 only, i will include my code for parts 1-4 at the end. PLease try to include a description of each part of code
Part 4
Write a function called "check_answer" which will determine if a given addition or subtraction problem was solved correctly. Here's the IPO notation for the function:
# function: check_answer # input: two numbers (number1 & number2, both integers); an answer (an integer) # and an operator (+ or -, expressed as a String) # processing: determines if the supplied expression is correct. for example, if the operator # is "+", number1 = 1, number2 = 2 and answer = 3 then the expression is correct # (1 + 2 = 3). # output: returns True if the expression is correct, False if it is not correct
Here's a sample program that you can use to test your function:
answer1 = check_answer(1, 2, 3, "+") print (answer1) answer2 = check_answer(1, 2, -1, "-") print (answer2) answer3 = check_answer(9, 5, 3, "+") print (answer3) answer4 = check_answer(8, 2, 4, "-") print (answer4)
And here's the expected output:
True True False False
Part 5
Move all of your functions into an external module. This can be done by creating a new .py file in the same folder as your source code file. You can then "import" the functions from this file by using the following syntax:
import myfunctions
... assuming that the file you created is named "myfunctions.py". You can then call your functions in your main source code file using "dot syntax", like this:
myfunctions.check_answer(1,1,2,"+")
Part 6
Finally, put everything together and write a program that lets the user practice a series of random addition and subtraction problems. Begin by asking the user for a number of problems (only accept positive values) and a size for their numbers (only accept numbers between 5 and 20). The generate a series of random addition and subtraction problems - display the numbers to the user with your digital display functions. Then prompt the user for an answer and check the answer using your check_answer function. Your program should also keep track of how many correct questions the user answered during their game. Here's a sample running of the program:
How many problems would you like to attempt? -5 Invalid number, try again How many problems would you like to attempt? 5 How wide do you want your digits to be? 5-20: 3 Invalid width, try again How wide do you want your digits to be? 5-20: 5 Here we go! What is ..... ***** * ***** * ***** * * ***** * * * * * * * = 4 Correct! What is ..... ***** * ***** * ***** ***** ***** * * * * = -5 Correct! What is ..... * * * * * ***** ***** * ***** * ***** = 0 Sorry, that's not correct. What is ..... ***** * ***** * ***** * * ***** * * * * * * * = 3 Correct! What is ..... ***** * ***** * ***** * * ***** * * ***** * ***** * ***** = 4 Correct! You got 4 out of 5 correct!
Added features
Add multiplication problems to the game. You will have to update your check_answer function as well as add a new operator function to display the multiplication sign.
Add division problems to the game. You will have to update your check_answer function as well as add a new operator function to display the division sign. For division problems you need to ensure that the result of the problem you present is a whole number. For example, the following would be valid division problems for this game:
2 / 2 = 0 4 / 2 = 1 9 / 3 = 3
However, the following would NOT be valid since the answers are not whole numbers:
5 / 2 = 2.5 9 / 8 = 1.125 8 / 3 = 2.6666666 (repeating)
Ensure that the division problems you supply to your players always yield a whole number result. You may need to generate a few different numbers in order to do this (hint: while loop!).Add in a "drill" mode to your game. If this mode is activated by the user they will be re-prompted to solve a problem that they got incorrect. Points are turned off in "drill" mode since the user can attempt a problem multiple times. Here's an example:
How many problems would you like to attempt? 2 How wide do you want your digits to be? 5-10: 5 Would you like to activate 'drill' mode? yes or no: yes What is ..... ***** * * * * * * ***** * * ***** * * ***** * ***** * ***** = 1 Sorry, that's not correct. = 2 Sorry, that's not correct. = 3 Correct! What is ..... ***** * * ***** * * ***** * * ***** * * ***** * ***** * ***** = 13 Correct! Thanks for playing!
Keep track of statistics by problem type. For example, at the end of your program you could display a display like the following that summarizes the performance of the player:
Total addition problems presented: 5 Correct addition problems: 4 (80.0%) Total subtraction problems presented: 4 Correct subtraction problems: 3 (75.0%) No multiplication problems presented Total division problems presented: 1 Correct division problems: 0 (0.0%)
If you implemented "drill" mode you should modify your statistics display to include information about how many "extra" attempts it took so solve those problems. For example:
Total addition problems presented: 5 # of extra attempts needed: 0 (perfect!) Total subtraction problems presented: 4 # of extra attempts needed: 1 No multiplication problems presented Total division problems presented: 1 # of extra attempts needed: 5
My code for parts 1-4
#Assignment 6 - Part 2
#write 10 new functions that generate the digits 0-9
#using your three line functions
def horizontal_line(n):
for i in range(n):
print("*",end ='')
print()
def line(s,h):
for i in range(h):
for i in range(s):
print(" ", end='')
print("*")
def vertical_lines(w,h):
for i in range(h):
print("*",end= '')
#the spaces when the width is 2 is 0 so subtract by 2
#for each star you want to remove a part of the width
for i in range(w-2):
print(" ", end= '')
print("*")
def number_0(w):
#top part of the 0
horizontal_line(w)
#middle 2 lines of 0. will always have height of 3 since the 0 will always
#be height 5
vertical_lines(w,3)
#bottom line of the 0
horizontal_line(w)
#print blank to separate it from following numbers
print()
def number_1(w):
#prints a line shifted over width - 1 to account for the star, since the
#star counts as a width slot
line(w-1,5)
#separates the one from the following numbers
print()
def number_2(w):
#creates the top of 2
horizontal_line(w)
#since height is always 5, we only need one star
#so the param for the function 'line' call will have a height of 1
#it will be shifted width - 1 to account for the star
line(w-1,1)
#intermediate line of the 2
horizontal_line(w)
#prints a line of height 1 shifted 0
line(0,1)
#closes the 2 with a bottom horizontal line
horizontal_line(w)
print()
def number_3(w):
#creates the top of 3
horizontal_line(w)
#the param for the function 'line' call will have a height of 1
#it will be shifted width - 1 to account for the star
line(w-1,1)
#middle line for the 3
horizontal_line(w)
#prints a line of height 1 shifted width - 1 like the previous line
line(w-1,1)
#closes the 3 with a horizontal line
horizontal_line(w)
print()
def number_4(w):
vertical_lines(w,2)
horizontal_line(w)
line(w-1,2)
def number_5(w):
horizontal_line(w)
line(0,1)
horizontal_line(w)
line(w-1,1)
horizontal_line(w)
def number_6(w):
horizontal_line(w)
line(0,1)
horizontal_line(w)
vertical_lines(w,1)
horizontal_line(w)
def number_7(w):
horizontal_line(w)
line(w-1,4)
def number_8(w):
horizontal_line(w)
vertical_lines(w,1)
horizontal_line(w)
vertical_lines(w,1)
horizontal_line(w)
def number_9(w):
horizontal_line(w)
vertical_lines(w,1)
horizontal_line(w)
line(w-1,2)
#testing program
print ("Number 1, width=5: ")
number_1(5)
print()
print ("Number 1, width=10: ")
number_1(10)
print()
print ("Number 1, width=2: ")
number_1(2)
#print numbers 0-9
number_0(5)
print()
number_1(5)
print()
number_2(5)
print()
number_3(5)
print()
number_4(5)
print()
number_5(5)
print()
number_6(5)
print()
number_7(5)
print()
number_8(5)
print()
number_9(5)
print()
#part 3
def minus(n):
for i in range(n):
print("*",end='')
print()
def plus(n):
for i in range(n):
if(i == int(n/2)):
minus(n)
else:
for j in range(int(n/2)):
print(" ",end='')
print("*")
n = int(input("Enter the number: "))
plus(n)
minus(n)
#part4
def check_answer(n1,n2,answer,operator):
if operator == "+":
return answer == n1 + n2
else if opertor == "-":
return answer == n1 - n2
return false
I just need help completing parts 5, 6 and the addtional part at the end
def minus(n):
for i in range(n):
print("*",end='')
print()
def plus(n):
for i in range(n):
if(i == int(n/2)):
minus(n)
else:
for j in range(int(n/2)):
print(" ",end='')
print("*")
n = int(input("Enter the number: "))
plus(n)
minus(n)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
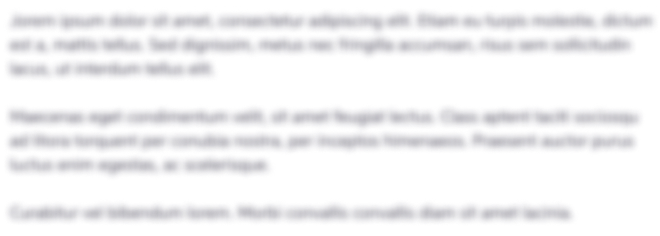
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started