Question
Please write the ArrayList code so that the driver will generate the given output. Thanks. __________________________________ public class MyArrayList { // Data fields private E[]
Please write the ArrayList code so that the driver will generate the given output. Thanks.
__________________________________
public class MyArrayList {
// Data fields private E[] theData; private int size = 0; private int capacity = 0;
// Constants private static final int INIT_CAPACITY = 10;
// Constructors /* public MyArrayList() { this(INIT_CAPACITY); //theData = (E[]) new Object[INIT_CAPACITY]; capacity=INIT_CAPACITY;
} */ @SuppressWarnings("unchecked") public MyArrayList(int initCapacity) { theData = (E[]) new Object[initCapacity]; capacity=initCapacity; }
// Methods public boolean add(E e) { if(e == null) { throw new NullPointerException(); }
//*** Put Code here if size reaches capacity theData[size] = e; size++;
return true; } // End add(E e) method
public void add(int index, E e) {
} // End add(int index, E e) method
@SuppressWarnings("unchecked") public void clear() {
} // End clear() method
public void print() {
} public boolean equals(Object o) { if(o == null) { return false; }
if(getClass() != o.getClass()) { return false; }
MyArrayList otherO = (MyArrayList) o;
if(size != otherO.size) { return false; }
for(int i = 0; i
return true; } // End equals(Object o) method
public E get(int index) {
} // End get(int index) method
public int indexOf(Object o) {
} // End indexOf(Object o) method
public boolean isEmpty() { return size == 0; } // End isEmpty() method
public E remove(int index) {
} // End remove(int index) method
public boolean remove(Object o) { //*** using indexOf remove Object must code remove(index) first
return true; } // End remove(Object o) method
public E set(int index, E e) { if(index = size) { throw new ArrayIndexOutOfBoundsException(index); }
if(e == null) { throw new NullPointerException(); } E temp = theData[index]; theData[index] = e; return temp; } // End set(int index, E e) method
public int size() { return size; } // End size() method
private void reallocate() { System.out.println("CALLING reallocate"); //*** Code for reallocate @SuppressWarnings("unchecked")
} // End reallocate() method
}//End Class
______________________________
import java.util.*;
public class MyArrayListDriver {
public static void main(String[] args) { // TODO Auto-generated method stub System.out.println(" *********** Testing **************"); MyArrayListlist=new MyArrayList(10); String s2="I have not yet begun to fight"; StringTokenizer tokenizer=new StringTokenizer(s2); while(tokenizer.hasMoreTokens()) { String token=tokenizer.nextToken(); System.out.println(list.add(token)); //This will print true all the time -- why ???? }//end for for(int i=0;i { System.out.println(list.get(i)); }//end for System.out.println(" ********** Testing ************ "); System.out.println(" ********** Testing the methods add, get, size , reallocate************ "); MyArrayListlist2=new MyArrayList(); list2.add("Namath"); list2.add("Snell"); list2.add("Boozer"); list2.add("Biggs"); list2.add("Sauer"); list2.add("Maynard"); list2.add("Atkinson"); list2.add("Sample"); list2.add("Dockery"); list2.add("Philbin"); list2.add("Baker"); list2.add("Parelli"); for(int i=0;i { System.out.println(list2.get(i)); }//end for System.out.println(" ********** Testing the methods add(int, obj) ************ "); list2.add(0,"Bradshaw"); list2.add(2,"Swann"); list2.add(4,"Harris"); list2.add(6,"Mantle"); list2.add(8,"Pasterini"); list2.add(10,"Block"); list2.add(12,"Unitas"); list2.add(14,"Jones"); list2.add(16,"Riggins"); list2.add(18,"Strawberry"); list2.add(20,"Johnson"); list2.add(22,"Sayers"); list2.add(24,"Dykstra"); for(int i=0;i { System.out.println(list2.get(i)); } System.out.println(" ********** Testing the methods set(i,obj) ************ "); list2.set(0, "Long Branch"); list2.set(2, "Belmar"); list2.set(4, "Asbury Park"); list2.set(list2.size()-1, "Red Bank"); for(int i=0;i { System.out.println(list2.get(i)); } System.out.println(" ********** Testing the methods indexOf(obj) ************ "); System.out.println(list2.indexOf("Long Branch")); System.out.println(list2.indexOf("Riggins")); System.out.println(list2.indexOf("Baker")); System.out.println(list2.indexOf("Red Bank")); System.out.println(list2.indexOf("Montreal")); System.out.println(" ********** Testing the methods isEmpty() and remove(Obj) ************ "); while(!list2.isEmpty()) { System.out.println(list2.remove(0)); } System.out.println("The size is now " + list2.size()); System.out.println(" ********** Testing the try catch for get(i) ************ "); //Re-populate the list list2.add("Namath"); list2.add("Snell"); list2.add("Boozer"); list2.add("Biggs"); list2.add("Sauer"); list2.add("Maynard"); list2.add("Atkinson"); list2.add("Sample"); list2.add("Dockery"); list2.add("Philbin"); list2.add("Baker"); list2.add("Parelli"); try { list2.get(-2); } catch(ArrayIndexOutOfBoundsException e) { System.out.println(e); }//end try catch try { list2.get(1000); } catch(ArrayIndexOutOfBoundsException e) { //e.printStackTrace(); System.out.println(e); }//end try catch try { list2.get(list2.size()); } catch(ArrayIndexOutOfBoundsException e) { System.out.println(e); }//end try catch System.out.println(" ***** Testing Remove(Object obj) ******"); System.out.println(list2.remove("Namath")); System.out.println(list2.remove("Biggs")); System.out.println(list2.remove("Boozer")); for(int i=0;i { System.out.println(list2.get(i)); } System.out.println(" ***** Testing remove(int index) ******"); System.out.println(list2.remove(0)); System.out.println(list2.remove(2)); System.out.println(" ******* Printing Result from remove(int index)******"); for(int i=0;i { System.out.println("index: "+ i+"\tObject: "+list2.get(i)); } System.out.println("Finally lets test our throwing and catching errors"); try { list2.remove(list2.size()); } catch(ArrayIndexOutOfBoundsException e) { System.out.println(e); }//end try catch try { //list2.add(-4,"Namath");//Only one will be caught Make sure that we know that it will drop out of the try catch block once an error is caught list2.add(2, null); } catch(ArrayIndexOutOfBoundsException e) { System.out.println(e); }//end try catch catch(NullPointerException e) { System.out.println(e); }//end try catch try { list2.add(-4,"Namath");//Only one will be caught Make sure that we know that it will drop out of the try catch block once an error is caught //list2.add(2, null); } catch(ArrayIndexOutOfBoundsException e) { System.out.println(e); }//end try catch catch(NullPointerException e) { System.out.println(e); }//end try catch System.out.println(" *** Finaly lets make sure that our ArrayList is intact"); System.out.println("In other words, our throwing errors did not stop our program because we CAUGHT Them"); for(int i=0;i { System.out.println(list2.get(i)); } }//end main
}//end class!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
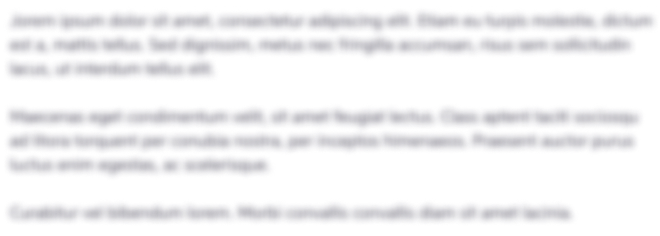
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started