Question
In this lab, you will be creating a class that implements the Rule of Three (A Destructor, A Copy Constructor, and a Copy Assignment Operator).
In this lab, you will be creating a class that implements the Rule of Three (A Destructor, A Copy Constructor, and a Copy Assignment Operator). You are to create a program that prompts users to enter in contact information, dynamically create each object, then print the information of each contact to the screen. Some code has already been provided for you. To receive full credit make sure to implement the following:
- Default Constructor - set Contact id to -1
- Overloaded Constructor - used to set the Contact name, phoneNumber and id (Should take in 3 parameters)
- Destructor
- Copy Constructor
- Copy Assignment Operator
- Any other useful functions (getters/setters)
Main.cpp
#include
#include
#include "Contact.h"
using namespace std;
int main() {
const int NUM_OF_CONTACTS = 3;
vector contacts;
for (int i = 0; i < NUM_OF_CONTACTS; i++) {
string name, phoneNumber;
cout << "Enter a name: ";
cin >> name;
cout << "Enter a phoneNumber; ";
cin >> phoneNumber;
// TODO: Use i, name, and phone number to dynamically create a Contact object on the heap
// HINT: Use the Overloaded Constructor here!
// TODO: Add the Contact * to the vector...
}
cout << "----- Contacts ----- ";
// TODO: Loop through the vector of contacts and print out each contact info
// TODO: Make sure to call the destructor of each Contact object by looping through the vector and using the delete keyword
return 0;
}
Contact.h
#ifndef CONTACT_H
#define CONTACT_H
#include
#include
using std::string;
using std::cout;
class Contact {
public:
Contact();
Contact(int id, string name, string phoneNumber);
~Contact();
Contact(const Contact& copy);
Contact& operator=(const Contact& copy);
private:
int *id = nullptr;
string *name = nullptr;
string *phoneNumber = nullptr;
};
#endif
Contact.cpp
#include "Contact.h"
Contact::Contact() {
this->id = new int(-1);
this->name = new string("No Name");
this->phoneNumber = new string("No Phone Number");
}
Contact::Contact(int id, string name, string phoneNumber) {
// TODO: Implement Overloaded Constructor
// Remember to initialize pointers on the heap!
}
Contact::~Contact() {
// TODO: Implement Destructor
}
Contact::Contact(const Contact ©) {
// TODO: Implement Copy Constructor
}
Contact &Contact::operator=(const Contact ©) {
// TODO: Implement Copy Assignment Operator
return *this;
}
Step by Step Solution
3.41 Rating (151 Votes )
There are 3 Steps involved in it
Step: 1
SOLUTION Contacth ifndef CONTACTH define CONTACTH include include using stdstring using stdcout clas...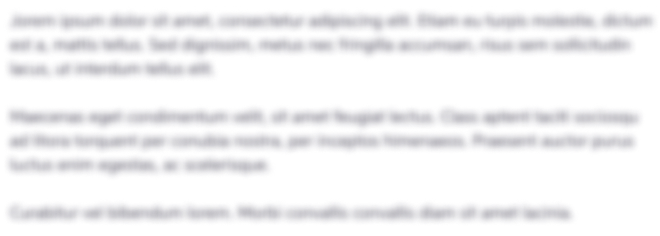
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started