Question
PoliceReport.java import java.util.ArrayList; /* * A police report is a report that keeps track of the criminal's name, and the list of crimes as well
PoliceReport.java
import java.util.ArrayList;
/*
* A police report is a report that keeps track of the criminal's name, and the list of crimes as well as the
* date of each crime
*/
//-----------Start below here. To do: approximate lines of code = 1
// Make class PoliceReport a subclass of Report
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
{
private String criminalName;
private ArrayList crimes;
private ArrayList dates;
public PoliceReport(String title, String author, String date, String name)
{
super(title,author,date);
this.criminalName = name;
crimes = new ArrayList();
dates = new ArrayList();
}
//Overrides the print() method in class Document
public void print()
{
//-----------Start below here. To do: approximate lines of code = 4
// Set the first section string in the inherited array list sections to the string:
// "Criminal Name: " followed by the criminal's name followed by ""
// Then set each subsequent string in the sections arraylist to the crime description (from crimes array list) followed by
// " " followed by the date of the crime (from the dates array list)
// Then finally print the police report by properly calling the print() method in the superclass Report
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
//-----------Start below here. To do: approximate lines of code = 3
// Create a method void addCrime(String crime, String date) that adds the crime to the array list of crimes
// and adds the date to the array list of dates
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
Report.java
import java.util.ArrayList;
/*
* A simple general class that describes a report. It consists of a title, author, date and a list of sections
*/
public class Report
{
private String title;
private String author;//school name for report card
private String date;
private ArrayList sections;
public Report(String title, String author, String date)
{
this.title = title;
this.author = author;
this.date = date;
sections = new ArrayList();
}
public String getTitle()
{
return title;
}
public void setTitle(String title)
{
this.title = title;
}
public String getAuthor()
{
return author;
}
public void setAuthor(String author)
{
this.author = author;
}
public String getDate()
{
return date;
}
public void setDate(String date)
{
this.date = date;
}
public void setSection(String section)
{
sections.add(section);
}
public void print()
{
System.out.println(title + "" + author + " " + date);
for (int i = 0; i < sections.size(); i++)
{
System.out.println(sections.get(i));
}
}
}
SalesReport.java
import java.util.ArrayList;
/*
* A sales report is a report that keeps track of the sales person's name, and the items they sold as well as the
* price of each item
*/
//-----------Start below here. To do: approximate lines of code = 1
// Make class SalesReport a subclass of Report
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
{
private String salesPerson;
private ArrayList itemsSold;
private ArrayList itemPrices;
public SalesReport(String title, String author, String date, String name)
{
super(title,author,date);
this.salesPerson = name;
itemsSold = new ArrayList();
itemPrices = new ArrayList();
}
//Overrides the print() method in class Report
public void print()
{
//-----------Start below here. To do: approximate lines of code = 4
// Set the first section string in the inherited array list sections to the string:
// "Sales Person: " followed by the name of the sales person followed by ""
// Then set each subsequent string in the sections arraylist to the item description (from itemsSold array list) followed by
// " " followed by the item price (from itemPrices array list)
// Then finally print the sales report by properly calling the print() method in the superclass Report
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
//-----------Start below here. To do: approximate lines of code = 3
// Create a method void addItem(String itemName, double price) that adds the sales item to the array list of itemsSold
// and adds the price to the array list of itemPrices
//-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions.
}
QH4.java
/*
* A program to test two subclasses of superclass Report: PoliceReport and SalesReport
*/
public class QH4
{
public static void main(String[] args)
{
PoliceReport policeReport = new PoliceReport("Police Report", "Officer S. DoGood", "April 2021", "T. BadGuy");
policeReport.addCrime("Theft", "March 16, 2021");
policeReport.addCrime("Jaywalking", "February 8, 2021");
policeReport.addCrime("Fraud", "December 19, 2020");
policeReport.addCrime("Trespassing", "March 8, 2021");
System.out.println("Testing Police Report");
Report report = policeReport;
report.print();
System.out.println("Expected:Police ReportOfficer S. DoGood April 2021Criminal Name: T. BadGuy");
System.out.println("Theft March 16, 2021Jaywalking February 8, 2021Fraud December 19, 2020Trespassing March 8, 2021");
SalesReport salesReport = new SalesReport("Sales Report", "Best Buy", "Nov. 2020", "T. McInerney");
salesReport.addItem("Razer Laptop", 4000.0);
salesReport.addItem("Microsoft Surface", 2200.0);
salesReport.addItem("iPhone 10", 1100.0);
salesReport.addItem("Dell Laptop", 550.0);
System.out.println("Testing Sales Report");
report = salesReport;
report.print();
System.out.println("Expected:Sales ReportBest Buy Nov. 2020Sales Person: T. McInerney");
System.out.println("Razer Laptop 4000.0Microsoft Surface 2200.0iPhone 10 1100.0Dell Laptop 550.0");
}
}
Step by Step Solution
3.50 Rating (150 Votes )
There are 3 Steps involved in it
Step: 1
OUTPUT PoliceReportjava import javautilArrayList A police report is a report that keeps track of the criminals name and the list of crimes as well as the date of each crime Start below here To do appr...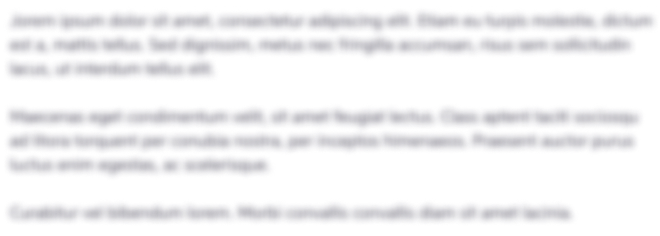
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started