Question
#pragma once #include #include using std::cout; using std::endl; using std::string; class Book { private: string title; string author; int ISBN; bool isCheckedOut; string checkedOutBy; public:
#pragma once #include #include using std::cout; using std::endl; using std::string; class Book { private: string title; string author; int ISBN; bool isCheckedOut; string checkedOutBy; public: //Constructor Book(string t = "N/A", string a = "N/A", int i = -1) { title = t; author = a; ISBN = i; isCheckedOut = false; checkedOutBy = ""; } //Getters string getTitle() { return title; } string getAuthor() { return author; } int getISBN() { return ISBN; } bool getStatus() { return isCheckedOut; } string getCheckedOutBy() { return checkedOutBy; } //Setters void setTitle(string new_t) { title = new_t; } void setAuthor(string new_a) { author = new_a; } void setISBN(int new_i) { ISBN = new_i; } // If the book is checked out, return false, //otherwise, set the checked out status to //true and update the name of the user that //checked out the book. bool checkOutBook(string n) { if (isCheckedOut == true) return false; else { isCheckedOut = true; checkedOutBy = n; return true; } } void returnBook() { isCheckedOut = false; checkedOutBy = ""; } void outputBook() { cout author = obj.author; this->title = obj.title; this->ISBN = obj.ISBN; this->checkedOutBy = obj.checkedOutBy; this->isCheckedOut = obj.isCheckedOut; return *this; } };
#include "CatalogClass.h" #include using std::cin; using std::getline; void populate_catalog(Catalog& my_catalog); int display_menu(); int main() { Catalog my_catalog; populate_catalog(my_catalog); cout = 1 && choice > choice; if (choice 8) { cout 8); return choice; } void populate_catalog(Catalog& my_catalog) { Book temp_obj; temp_obj.setAuthor("J.K. Rowling"); temp_obj.setTitle("Harry Potter and the Sorcerers Stone"); temp_obj.setISBN(98346); my_catalog.addBook(temp_obj); temp_obj.setAuthor("J.K. Rowling"); temp_obj.setTitle("Harry Potter and the Chamber of Secrets"); temp_obj.setISBN(19285); my_catalog.addBook(temp_obj); temp_obj.setAuthor("J.K. Rowling"); temp_obj.setTitle("Harry Potter and the Prisioner of Azkaban"); temp_obj.setISBN(88224); my_catalog.addBook(temp_obj); temp_obj.setAuthor("J.K. Rowling"); temp_obj.setTitle("Harry Potter and the Goblet of Fire"); temp_obj.setISBN(21001); my_catalog.addBook(temp_obj); temp_obj.setAuthor("J.K. Rowling"); temp_obj.setTitle("Harry Potter and the Order of Phoenix"); temp_obj.setISBN(66754); my_catalog.addBook(temp_obj); temp_obj.setAuthor("J.K. Rowling"); temp_obj.setTitle("Harry Potter and the Half-Blood Prince"); temp_obj.setISBN(50125); my_catalog.addBook(temp_obj); temp_obj.setAuthor("J.K. Rowling"); temp_obj.setTitle("Harry Potter and the Deathly Hallows"); temp_obj.setISBN(68304); my_catalog.addBook(temp_obj); temp_obj.setAuthor("C.S. Lewis"); temp_obj.setTitle("The Lion, the Witch, and the Wardrobe"); temp_obj.setISBN(45336); my_catalog.addBook(temp_obj); temp_obj.setAuthor("C.S. Lewis"); temp_obj.setTitle("Prince Caspian"); temp_obj.setISBN(76689); my_catalog.addBook(temp_obj); temp_obj.setAuthor("C.S. Lewis"); temp_obj.setTitle("The Voyage of the Dawn Treader"); temp_obj.setISBN(34982); my_catalog.addBook(temp_obj); temp_obj.setAuthor("C.S. Lewis"); temp_obj.setTitle("The Silver Chair"); temp_obj.setISBN(45993); my_catalog.addBook(temp_obj); temp_obj.setAuthor("C.S. Lewis"); temp_obj.setTitle("A Horse and His Boy"); temp_obj.setISBN(42398); my_catalog.addBook(temp_obj); temp_obj.setAuthor("C.S. Lewis"); temp_obj.setTitle("The Magician's Nephew"); temp_obj.setISBN(39203); my_catalog.addBook(temp_obj); temp_obj.setAuthor("C.S. Lewis"); temp_obj.setTitle("TheLast Battle"); temp_obj.setISBN(56342); my_catalog.addBook(temp_obj); temp_obj.setAuthor("E. B. White"); temp_obj.setTitle("Charlotte's Web"); temp_obj.setISBN(47851); my_catalog.addBook(temp_obj); temp_obj.setAuthor("F. Scott Fitzgerald"); temp_obj.setTitle("The Great Gasby"); temp_obj.setISBN(11934); my_catalog.addBook(temp_obj); temp_obj.setAuthor("S. E. Hinton"); temp_obj.setTitle("The Outsiders"); temp_obj.setISBN(72331); my_catalog.addBook(temp_obj); /* Feel free to add more books below to experiment with the catalog class! */ }
Have to make it where
Part 1 Instructions In the previous assignment, we created a class called "Book, which stored basic information about a book at a library. The program used this class to create sample book objects to store information about popular novels. In this program, we are going to build a new class called "Catalog, which will be used to store and manage books in the library. The program should support the following menu commands: 1. Search Catalog a. By Title b. By Author c. By ISBN 2. Add New Book 3. Get Book Status 4. Check in a Book 5. Check Out a Book 6. Exit Catalog -books: Book pointer -next_slot: int -capacity: intStep by Step Solution
There are 3 Steps involved in it
Step: 1
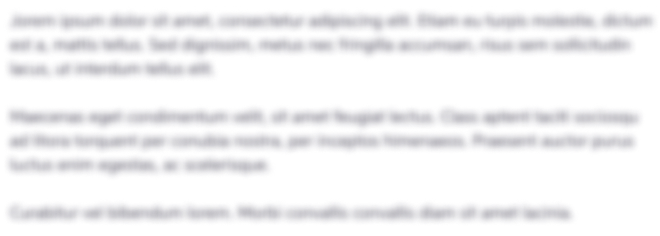
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started