Question
Previous Class public class Card { //Define the enumerated class Color, Shape, and Fill //to get the card's fear=tures. public enum Color { RED ,
Previous Class
public class Card
{
//Define the enumerated class Color, Shape, and Fill
//to get the card's fear=tures.
public enum Color {RED, PURPLE, GREEN};
public enum Shape {OVAL, SQUARE, DIAMOND};
public enum Fill {SOLID, HATCHED, OUTLINE};
//Declare the required member variables.
private int rank_Of_Card;
private Color card_color;
private Shape card_shape;
private Fill fill_card;
//Define the constructor of the class.
public Card(int cardRank, Color col, Shape sh, Fill
fil)
{
this.rank_Of_Card = cardRank;
this.card_color = col;
this.card_shape = sh;
this.fill_card = fil;
}
//Define the method toString() to reperesent a card
//on the board.
public String toString()
{
return rank_Of_Card + "_" + card_color + "_" +
card_shape + "_" + fill_card;
}
//Define the method checkSet().
public static boolean checkSet(Card card_one, Card
card_two, Card card_three)
{
//Initialize the required variables.
boolean rank_status = false;
boolean color_status = false;
boolean shape_status = false;
boolean fill_status = false;
//If rank of each card is same, then set the
//rank status true.
if(card_one.rank_Of_Card == card_two.
rank_Of_Card && card_one.rank_Of_Card ==
card_three.rank_Of_Card)
rank_status = true;
//If rank of each card is different, then set
//the rank status true.
else if(card_one.rank_Of_Card != card_two.
rank_Of_Card && card_two.rank_Of_Card !=
card_three.rank_Of_Card && card_one.
rank_Of_Card != card_three.rank_Of_Card)
rank_status = true;
//If fill of each card is same, then set
//the fill status true.
if(card_one.fill_card == card_two.fill_card
&& card_one.fill_card == card_three.
fill_card)
fill_status = true;
//If fill of each card is different, then set
//the fill status true.
else if(card_one.fill_card != card_two.
fill_card && card_two.fill_card !=
card_three.fill_card && card_one.fill_card
!= card_three.fill_card)
fill_status = true;
//If shape of each card is same, then set
//the shape status true.
if(card_one.card_shape == card_two.
card_shape && card_one.card_shape ==
card_three.card_shape)
shape_status = true;
//If shape of each card is different, then set
//the shape status true.
else if(card_one.card_shape != card_two.
card_shape && card_two.card_shape !=
card_three.card_shape && card_one.card_shape
!= card_three.card_shape)
shape_status = true;
//If color of each card is same, then set
//the color status true.
if(card_one.card_color == card_two.
card_color && card_one.card_color ==
card_three.card_color)
color_status = true;
//If color of each card is different, then set
//the color status true.
else if(card_one.card_color != card_two.
card_color && card_two.card_color !=
card_three.card_color && card_one.card_color
!= card_three.card_color)
color_status = true;
//Get the result of AND of each status.
boolean isCardSet = rank_status && color_status
&& fill_status && shape_status;
//Return the result.
return isCardSet;
}
}
//Deck.java:
//Import the required packages.
import java.util.ArrayList;
//Define the class Deck.
public class Deck
{
//Declare the object of the array list of Card
//objects.
private ArrayList
public Deck()
{
//Initialize the color, fill and shape of the
//cards.
Card.Color[] card_colors = Card.Color.values();
Card.Fill[] fill_cards = Card.Fill.values();
Card.Shape[] card_shapes = Card.Shape.values();
//Create an object of array list of card objects.
card_obj = new ArrayList
//Start the four for loops till the length of
//color, shape, fill arrays and 3 ranks.
for(int rank_num = 1; rank_num
{
for(int col = 0; col
col++)
for(int sh = 0; sh
card_shapes.length; sh++)
for(int fil = 0; fil
length; fil++)
//Initialize the object of
//the card with required
//values and add it into the
//array list.
card_obj.add(new
Card(rank_num,
card_colors[col],
card_shapes[sh],
fill_cards[fil]));
}
}
//Define the method shuffle().
public void shuffle()
{
//Start the for loop till the size of the array
//list of card objects.
for(int i = 0; i
{
//Swap the current card with a random card
//in the deck of cards.
int random_index =(int) (Math.random() *
card_obj.size());
Card temp_card = card_obj.get(i);
card_obj.set(i, card_obj.get(random_index));
card_obj.set(random_index, temp_card);
}
}
//Define the method isEmpty().
public boolean isEmpty()
{
//Return the result of the condition if the
//size of the deck of the cards is 0.
return card_obj.size() == 0;
}
//Define the method getTopCard().
public Card getTopCard()
{
//If the deck of cards is not empty.
if(!isEmpty())
//Remove the first card from the array
//list and return that card.
return card_obj.remove(0);
//Otherwise, return nothing.
else
return null;
}
//Define the method toString().
public String toString()
{
//Declare a string variable to store the
//resulted string.
String res = "";
//Start a for each loopto access the
//items of the array list.
for(Card card: card_obj)
res += card + " ";
//Return the resultant string.
return res;
}
}
//BoardSquare.java:
//Define the class BoardSquare,
public class BoardSquare
{
//Declare the required member variales.
private Card card;
private int row_pos, col_pos;
boolean isSetSelect;
//Define the constructor of the class.
public BoardSquare(Card card, int rpos, int cpos)
{
//Initialize the required variables.
this.card = card;
this.row_pos = rpos;
this.col_pos = cpos;
this.isSetSelect = false;
}
//Define the method getCard() to return the card.
public Card getCard()
{
return card;
}
//Define the method setCard() to set the card value.
public void setCard(Card card)
{
this.card = card;
}
//Define the method getRowValue() to return the row
//position.
public int getRowValue()
{
return row_pos;
}
//Define the method setRowValue() to set the row
//position.
public void setRowValue(int row)
{
this.row_pos = row;
}
//Define the method getColValue() to return the
//coloumn position.
public int getColValue()
{
return col_pos;
}
//Define the method setColValue() to set the
//coloumn position.
public void setColValue(int col)
{
this.col_pos = col;
}
//Define the method getSelect() to return the
//result of whether the set is selected or not.
public boolean getSelect()
{
return isSetSelect;
}
//Define the method setSelect() to set the
//result of whether the set is selected or not.
public void setSelected(boolean select)
{
this.isSetSelect = select;
}
}
//Board.java:
//Import the required package.
import java.util.ArrayList;
//Define the class Board.
public class Board
{
//Declare the array list of array list of board
//square.
private ArrayList
board;
//Define the constructor of the class.
public Board(Deck deck)
{
//Create an object of the array list of array
//list of board square.
board = new ArrayList //Start the for loop till the number of rows //in the board. for(int row_index = 0; row_index row_index++) { //Add the array list of the board square //objects. board.add(new ArrayList //Start the for loop till the number of //columns of the board. for(int col_index = 0; col_index col_index++) { //Add the array list of the board //square objects with required top card //at required row and column position. board.get(row_index).add(new BoardSquare(deck.getTopCard(), row_index, col_index)); } } } //Define the method replaceCard(). public void replaceCard(Card rep_card, int row, int col) { //Call the method getBoardSquare(). getSquareBoard(row, col).setCard(rep_card); } //Define the method getSquareBoard(). public BoardSquare getSquareBoard(int row, int col) { //Return the card at the required row and //column position. return board.get(row).get(col); } //Define the method add3(). public void add3(Deck deck) { //Get the required column position. int col_index = board.get(0).size() - 1; //Start the for loop till the number //of rows. for(int row_index = 0; row_index row_index++) { //Add the required card at required //position. board.get(row_index).add(new BoardSquare(deck.getTopCard(), row_index, col_index)); } } //Define the method getCard(). public Card getCard(int row, int col) { //Return the cards at required position. return getSquareBoard(row, col).getCard(); } //Define the method numRows(). public int numRows() { //Retirn the size of the board. return board.size(); } //Define the numCols(). public int numCols() { //Return the size of the first item of //the arraylist board. return board.get(0).size(); } //Define the method toString(). public String toString() { //Get the required number of rows and //columns. int num_rows = numRows(); int num_cols = numCols(); //Declare a resultant string. String res = ""; //Start the for loops till number of //rows and columns. for(int rpos = 0; rpos rpos++) { for(int cpos = 0; cpos cpos++) { //Append the required card to //the resultant string. res += getCard(rpos, cpos). toString() + "\t"; } //Append " " to the resultant string. res += " "; } //Return the resultant string. return res; } } //Test.java: //Define the class Test to test the board. public class test { //Start the main() method. public static void main(String[] args) { //Create an object of the class Deck. Deck d = new Deck(); //Call shuffle() method. d.shuffle(); //Create an object of the Board class and pass //the deck class object. Board b = new Board(d); //Display the board. System.out.println(b); //Display the card of the board at 0,0. System.out.println(" " + b.getCard(0, 0) + " "); System.out.println(b); //If the set of the card is set,then display the //message set. if(Card.checkSet(b.getCard(0, 0), b.getCard(0, 1), b.getCard(0, 2))) System.out.println("set"); //Otherwise, display the message not set. else System.out.println("not a set"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
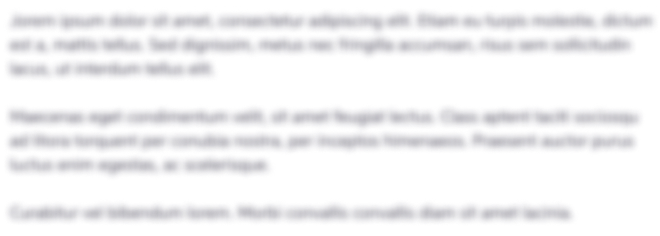
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started