Question
Primitive Types, Searching, Recursion a) Create a class Homework (in a file homework.h and homework.cpp). Review the lectures and textbook to learn about the content
Primitive Types, Searching, Recursion
a) Create a class Homework (in a file homework.h and homework.cpp). Review the lectures and textbook to learn about the content of each file.
b) Create a function initializeArray that receives two parameters: an array of integers and the array size. Use a for loop and an if statement to put 1s in the odd positions of the array and 0s in the even positions. Hint: review pointers as parameters.
c) Create a function printArray that receives as parameters an array of integers and the array size. Use a for statements to print all the elements in the array. Hint: review pointers as parameters.
d) Create a function arraySelectionSort that receives as parameters an array of integers and the array size, and order the array element in ascending order. Implement Selection Sort algorithm. It should be Selection Sort, not Bubble Sort, not Quick Sort, etc. If you do not remember selection sort, this link could be useful: https://goo.gl/hrAdMo
e) Create a recursive function that calculate and returns the factorial of a number. The function receives the number (integer number) as parameter
f) Create a file main_part1.cpp. Copy the following main function in your class,
int main() {
int a [10] = {3, 5, 6, 8, 12, 13, 16, 17, 18, 20};
int b [6] = {18, 16, 19, 3 ,14, 6};
int c [5] = {5, 2, 4, 3, 1};
Homework h;
// testing initializeArray
h.printArray(a, 10); // print: 3, 5, 6, 8, 12, 13, 16, 17, 18, 20
h.initializeArray(a, 10); h.printArray(a, 10); // print: 0, 1, 0, 1, 0, 1, 0, 1, 0, 1
// testing initializeArray
h.printArray(b, 6); // print: 18, 16, 19, 3 ,14, 6
h.arraySelectionSort (b, 6);
h.printArray(b, 6); // print: 3, 6, 14, 16, 18, 19
// testing factorial
cout <
Step by Step Solution
There are 3 Steps involved in it
Step: 1
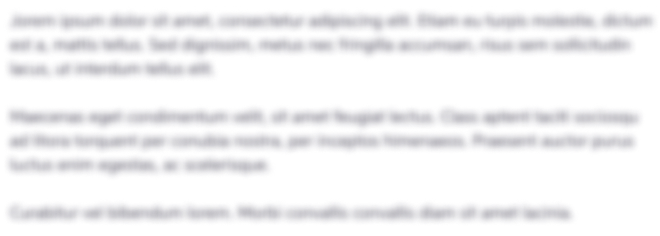
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started