Question
Problem 1. (1) Implement a class Clock whose getHours and getMinutes methods return the current time at your location. (Call java.time.LocalTime.now().toString() or, if you are
Problem 1.(1) Implement a class Clock whose getHours and getMinutes methods return the current time at your location. (Call java.time.LocalTime.now().toString() or, if you are not using Java 8, new java.util.Date().toString() and extract the time from that string.) Also provide a getTime method that returns a string with the hours and minutes by calling the getHours and getMinutesmethods.
(2) Provide a subclass WorldClock whose constructor accepts a time offset. For example, if you live in California, a new WorldClock(3) should show the time in New York, three time zones ahead. Which methods did you override? (You should not override getTime.)
Skeleton Code Below
World Clock-
/** * PART II. * Provide a subclass of Clock namded WorldClock whose constructor * accepts a time offset. For example, if you live in California, * a new WorldClock(3) should show the time in New York, three * time zones ahead. You should not override getTime. */ public class WorldClock extends Clock { // Your work goes here . . .
}
Clock-
import java.time.Instant; import java.time.LocalDateTime; import java.time.ZoneId; /** Part I: Implement a class Clock whose getHours, getMinutes and getTime methods return the current time at your location. Return the time as a string.
There are two ways to retrieve the current time as a String:
1) Before Java 8 use new Date().toString() 2) With Java 8, you can use 3 classes in the java.time package: Instant, LocalDateTime and ZoneId. Here's how you do it: String timeString = LocalDateTime.ofInstant(Instant.now(), ZoneId.systemDefault()).toString()
With either method, you'll need to extract the hours and minutes as a substring. */ public class Clock { /** * gets hours * @return hours of current time in string */ public String getHours() { final int HOUR_START = 11; return currentTime().substring(HOUR_START, HOUR_START + 2); }
/** * gets minutes * @return hours of current time in string */ public String getMinutes() { final int MINUTE_START = 14; return currentTime().substring(MINUTE_START, MINUTE_START + 2); }
/** * gets current time composed of hours and minutes * @return time in string; */ public String getTime() { return getHours() + ":" + getMinutes(); }
/** Returns the current Date and Time as a String. */ private String currentTime() { return LocalDateTime.ofInstant(Instant.now(), ZoneId.systemDefault()).toString(); } }
ClockDemo
/** * Tests the Clock and WorldClock Classes. */ public class ClockDemo { public static void main(String[] args) { //test WorldClock System.out.println(""); System.out.println("Testing WorldClock class"); int offset = 3; System.out.println("Offset: " + offset); WorldClock wclock = new WorldClock(offset); System.out.println("Hours: " + wclock.getHours()); System.out.println("Minutes: " + wclock.getMinutes()); System.out.println("Time: " + wclock.getTime()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
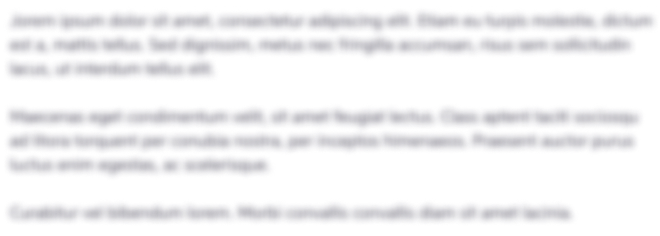
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started