Question
Problem 1: convert strings in snake_case to lowerCamelCase or UpperCamelCase. * * C programmers often use snake_case when naming functions or variables. In * JavaScript,
Problem 1: convert strings in snake_case to lowerCamelCase or UpperCamelCase.
*
* C programmers often use snake_case when naming functions or variables. In
* JavaScript, we use lowerCamelCase (first letter lower case) or UpperCamelCase
* (first letter Upper case) instead.
*
* You will write a function named toCamelCase() that accepts a string argument
* and converts it from snake_case to camelCase. The optional second argument
* determines whether or not to produce UpperCamelCase or not.
*
* The toCamelCase() function should work like this:
*
* toCamelCase('variable') returns 'variable'
* toCamelCase('variable_name') returns 'variableName'
* toCamelCase('variable_name', true) returns 'VariableName' ()
* toCamelCase('long_variable_name') returns 'longVariableName'
* toCamelCase('multiple___underscores') returns 'multipleUnderscores'
*
* @param {string} name - a string variable name to be converted
* @param {boolean} uppercase - (optional) whether to convert to UpperCamelCase
* defaults to `false`
* @return {string} - the converted camelCase version of the variable name
******************************************************************************/
function toCamelCase(name, uppercase) {
// Replace this comment with your code...
return name;
}
* Problem 2: create an HTML element with the given content.
*
* In HTML, a element is used to represent metadata about a web page. For
* example: who is tha page's author?
*
* If I wanted to indicate that a web page was written by Kim Lee, I would use
* the following tag:
*
* Write a function named createMetaTag() which accepts both name and content values.
* It should use these values to produce a new tag string. For example:
* * createMetaTag('description', 'Course notes for WEB222')
* * should return the following string of HTML:
* ''
* Make sure you remove any leading or trailing whitespace from the name and content
* values before you use them.
* createMetaTag('description', ' Course notes for WEB222 ')
should return the following string of HTML:
* ''
* Also, the double-quotes around name and content are optional if the value
* ''
* ''
*
* When creating your string, only include double-quotes when necessary.
** @param {string} name - the value for the name attribute
* @param {string} content - the value for the content attribute
* @returns {string} - a properly formatted tag
function createMetaTag(name, content) {
// Replace this comment with your code...
}
* Problem 3: extract Date from date string
* A date string is expected to be formatted in one of the following formats:
* 1. YYYY-MM-DD
* 2. DD-MM-YYYY
* Here, the Year (4 digits) may be listed first or last. The Month (2 digits)
* will always be in the middle position, and the Day (2 digits) will either
* be last (when Year is first) or first (when Year is last).
* January 15, 2023 could therefore be represented in either of the following
* formats:
* 2023-01-15
* 15-01-2023
*
* Write a function, parseDateString() that accepts a date string of the formats
* specified above, and returns a new JavaScript Date Object, set to the correct
* day. In your solution, you will need to use use the following Date methods:
* - new Date() - creates a new Date Object
* - setFullYear() - sets the Date Object's year value
* - setMonth() - sets the Date Object's month value
* - setDate() - sets the Date Object's day value
* * To help developers using your function, you are also asked to provide detailed
* error messages when the date string is formatted incorrectly. We will use the
* `throw` statement to throw a new Error object when a particular value is not
* For example: parseDateString('01-01-01') should fail, because the year is
* not 4 digits.
* Similarly, parseDateString('2021-1-01') should fail because
* the day is not 2 digits, and parseDateString('2021-01-1') should fail because
* the month is not 2 digits.
* Also, a totally invalid date string should also cause an exception to be thrown,
* for example parseDateString(null) or parseDateString("this is totally wrong").
*
* @param {string} value - a date string
* @returns {Date}
function parseDateString(value) {
// Replace this comment with your code...
}
* Problem 4: format a Date Object to use a given date string format.
* Building on your work in Problem 3 above, we want to be able to take a Date
* object, and format it into a string using one of the following 3 formats:
*
* 1. YYYY-MM-DD
* 2. DD-MM-YYYY
* 3. MM-DD-YYYY
* Meaning, Year (4 digits), Month (2 digits), Day (2 digits).
* Write a function, toDateString() that accepts a Date object and a date string
* format (e.g., "YYYY-MM-DD", "DD-MM-YYYY", or "MM-DD-YYYY"), and returns a date
* string formatted according to supplied format. Make sure your day and month
* values are padded with a leading '0' if necessary (e.g., 03 vs. 3).
* If something other than a valid Date Object is passed as the first argument,
* trying to call the Date methods will fail. You should use try/catch and
* throw a new Error object with an appropriate error message if this happens.
* See https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/try...catch
* If the date string format is not one of the 3 listed above, throw a new Error
* with an appropriate error message explaining the problem.
* NOTE: it should be possible to use parseDateString() from the previous question
* and toDateString() to reverse each other. For example:
* toDateString(parseDateString('2021-01-29), "YYYY-MM-DD") should return '2021-01-29'
* toDateString(parseDateString('2021-01-29), "DD-MM-YYYY") should return '29-01-2021'
* toDateString(parseDateString('29-01-2021), "MM-DD-YYYY") should return '01-29-2021'
*
* @param {Date} value - a Date Object to be formatted
* @param {string} format - a format string, one of "YYYY-MM-DD", "DD-MM-YYYY", or "MM-DD-YYYY"
* @returns {string} - the formatted date string
function toDateString(value, format) {
// Replace this comment with your code...
}
* Problem 5: parse a time duration
* Time duration is defined as a length of time expressed in hours, minutes, and
* seconds. An example, the duration from 9:15 AM to 10:45 AM is:
* Hours: 1
* Minutes: 30
* Seconds: 0
* A dataset includes thousands of time duration, stored as strings. However, over
* the years, different authors have used slightly different formats.
* All of the following are valid and need to be parsed:
* 1. "1:30:0"
* 2. "1h30m0s"
* In the first case, the values are separated by `:`. In the second, the values
* are followed by the unit (h, m, s).
* Valid Hour values are positive integers between 0 and 24.
* Valid Minute values are positive integers between 0 and 59.
* Valid Second values are positive integers between 0 and 59.
* If the input duration is invalid, return the value `null`.
* Parse the value and return a new string in the following form:
* "(hours, minutes, seconds)"
* @param {string} value - a time duration string in one of the given forms
* @returns {string|null} - a time duration formatted as "(hours, minutes, seconds)"
* or `null` if the duration isn't valid/recognized
function normalizeDuration(value) {
// Replace this comment with your code...
}
* Problem 6: format any number of durations as a list in a string
* You are asked to format time durations (hour, min, sec) in a list using your
* normalizeDuration() function from problem 5.
* Where normalizeDuration() takes a single duration string, the formatDurations()
* function takes a list of any number of duration strings, parses them,
* filters out any invalid ones, and creates a list.
* For example: given the following durations, "1h13m2s" and "4:16:24",
* a new list would be created of the following form "((1, 13, 2), (4, 16, 24))".
* Notice how the list has been enclosed in an extra set of (...) braces, and each
* duration is separated by a comma and space.
* The formatDurations() function can take any number of arguments, but they must all
* be strings. If any of the values can't be parsed by normalizeDuration() (i.e., if
* it returns null), skip the value. For example:
* formatDurations("1h13m2s", "300:600:900", "4:16:24") should return
* "((1, 13, 2), (4, 16, 24))" and skip the invalid duration.
* @param {number} arguments - any number of string duration arguments can be passed
* @returns {string}
function formatDurations(...values) {
// Replace this comment with your code...
}
* Problem 7: determine file type from a filename
* To identify the type of a file, the Operating System looks at the filename and
* extension. The OS needs to know the file type to open it with the correct
* program.
* Write a function, typeFromFilename(), which should take a filename and return the
* type of file it is (e.g., 'text', 'image', 'video', etc.), based on the
* following extensions:
* - .txt, .rtf, .doc, .docx --> 'text'
* - .jpg, .jpeg, .gif, .bmp, .ico, .cur, .png, .svg, .webp --> 'image'
* - .mp3, .wav --> 'audio'
* - .mp4, .webm, .mpeg, .avi --> 'video'
* - .json --> 'data'
* - .csv, .xls --> 'spreadsheet'
* - .ttf, .woff --> 'font'
* - .exe, .dll --> 'binary'
* - .zip --> 'archive'
* NOTE: any other extension should return 'unknown', to indicate that it is an
* unknown file type. You should also use 'unknown' if the file has no extension.
* @param {string} filename - a filename
* @returns {string}
******************************************************************************/
function typeFromFilename(filename) {
// Replace this comment with your code...
// NOTE: Use a switch statement in your solution.
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
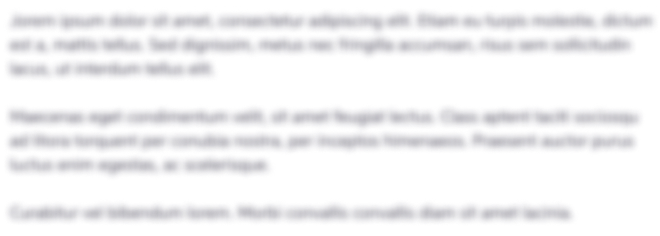
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started