Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Problem 1 : Hash table data structure with your own program created similar to the ones provided to answer all the questions insert ( key
Problem : Hash table data structure with your own program created similar to the ones provided to answer all the questions insertkey value getkey deletekey containskey keys and values Implement a hash table data structure using Java. Your implementation should include the following operations: insertkey value: Inserts a keyvalue pair into the hash table. getkey: Retrieves the value associated with a given key. If the key is not found, return None. deletekey: Deletes a keyvalue pair from the hash table based on the provided key. containskey: Checks if the hash table contains a specific key and returns True if found, False otherwise. keys: Returns a list of all keys in the hash table. values: Returns a list of all values in the hash table. You can choose to implement collision handling using techniques like chaining linked lists or open addressing linear probing, etc. And ensure that your hash table can handle resizing when it reaches a certain load factor. Provide the code for your hash table implementation, including any helper functions or classes, and demonstrate how you would use it to perform the above operations. Test your implementation with various scenarios to ensure its correctness and efficiency. Please refer the following code snippet in Java below that implements a simple hash table: import java.util.HashMap; public class HashTableExample public static void mainString args Create a hash table HashMap hashTable new HashMap; Insert keyvalue pairs into the hash table hashTable.putapple; hashTable.putbanana; hashTable.putorange; hashTable.putgrape; Retrieve the value associated with a specific key int bananaQuantity hashTable.getbanana; System.out.printlnQuantity of bananas: bananaQuantity; Check if a key exists in the hash table boolean containsPear hashTable.containsKeypear; System.out.printlnDoes the hash table contain 'pear'? containsPear; Remove a keyvalue pair from the hash table hashTable.removeorange; Print all keys and their corresponding values in the hash table for String key : hashTable.keySet int value hashTable.getkey; System.out.printlnKey: key Value: value; a Explain the purpose of the HashTableExample class and its methods. b Describe the process of inserting keyvalue pairs into this hash table. c Demonstrate how to retrieve the value associated with the key "banana" from the hash table. d Explain the role of the containsKey and how it can be useful. What is the role of containsKey here? e How would you remove the keyvalue pair associated with the key "orange" from the hash table? f Provide a code snippet to print all keys and their corresponding values in the hash table Collision handling using techniques like chaining linked lists or open addressing linear probing, etc. as well resizing when it reaches a certain load factor
Problem : Hash table data structure with your own program created similar to the ones provided to answer all the questions
insertkey value getkey deletekey containskey keys and values
Implement a hash table data structure using Java.
Your implementation should include the following operations:
insertkey value: Inserts a keyvalue pair into the hash table.
getkey: Retrieves the value associated with a given key. If the key is not found, return None.
deletekey: Deletes a keyvalue pair from the hash table based on the provided key.
containskey: Checks if the hash table contains a specific key and returns True if found, False otherwise.
keys: Returns a list of all keys in the hash table.
values: Returns a list of all values in the hash table.
You can choose to implement collision handling using techniques like chaining linked lists or open addressing linear probing, etc.
And ensure that your hash table can handle resizing when it reaches a certain load factor.
Provide the code for your hash table implementation, including any helper functions or classes, and demonstrate how you would use it to perform the above operations. Test your implementation with various scenarios to ensure its correctness and efficiency.
Please refer the following code snippet in Java below that implements a simple hash table:
import java.util.HashMap;
public class HashTableExample
public static void mainString args
Create a hash table
HashMap hashTable new HashMap;
Insert keyvalue pairs into the hash table
hashTable.putapple;
hashTable.putbanana;
hashTable.putorange;
hashTable.putgrape;
Retrieve the value associated with a specific key
int bananaQuantity hashTable.getbanana;
System.out.printlnQuantity of bananas: bananaQuantity;
Check if a key exists in the hash table
boolean containsPear hashTable.containsKeypear;
System.out.printlnDoes the hash table contain 'pear'? containsPear;
Remove a keyvalue pair from the hash table
hashTable.removeorange;
Print all keys and their corresponding values in the hash table
for String key : hashTable.keySet
int value hashTable.getkey;
System.out.printlnKey: key Value: value;
a Explain the purpose of the HashTableExample class and its methods.
b Describe the process of inserting keyvalue pairs into this hash table.
c Demonstrate how to retrieve the value associated with the key "banana" from the hash table.
d Explain the role of the containsKey and how it can be useful. What is the role of containsKey here?
e How would you remove the keyvalue pair associated with the key "orange" from the hash table?
f Provide a code snippet to print all keys and their corresponding values in the hash table
Collision handling using techniques like chaining linked lists or open addressing linear probing, etc. as well resizing when it reaches a certain load factor
Step by Step Solution
There are 3 Steps involved in it
Step: 1
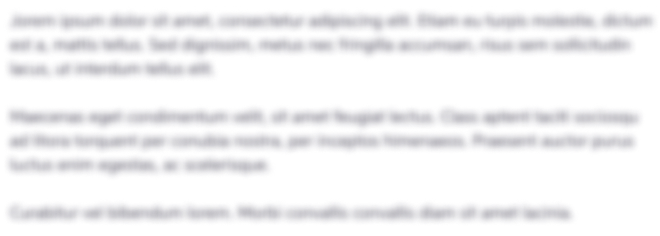
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started