Answered step by step
Verified Expert Solution
Question
1 Approved Answer
## Problem 1: Insect/Bee/Butterfly Abstract Classes * Note that the Autograder is looking for exact variable/method names. * The ** Insect ** class represents a
## Problem 1: Insect/Bee/Butterfly Abstract Classes *Note that the Autograder is looking for exact variable/method names.* The **Insect** class represents a generic insect species. You will specialize it with subclasses. For the Insect class, add 3 variables: * A String called `name` to store the insects name. This variable should be protected. * An int called `wingCount` to store the number of wings. This variable should be protected. * A constant (final) static int called `LEG_COUNT` to store the number of legs. Set it to 6. This constant should be public. Add get and set methods for name and wingCount. Think about: why are name and wingCount protected? Why is `LEG_COUNT` public, and why doesn't it need get and set methods? Insect objects need to create a String containing all data about that Insect. But, we don't know exactly what data will be stored for each specific Insect (a Bee's data will be different to a Butterfly's) so we can't write the code in this method yet. In Insect.java, add an **abstract** method called `speciesDataReport` like so, ``` abstract String speciesDataReport(); ``` The subclasses you are about to create will have have the same `speciesDataReport` method, and these methods will have code in. The **Butterfly** class represents a butterfly species. It will be a subclass of Insect. Configure Butterfly to extend Insect. Butterfly needs two new variables: * A String called `wingColor` to store the butterflys wing color * A String called `favoriteFlower` to store the butterflys favorite flower Add get and set methods for the `wingColor` and `favoriteFlower` variables. Think about: should you make these variables public, private, package-protected, or protected? Do you need get and set methods for name and wingCount in Butterfly? Why or why not? Also, add a constructor that takes 4 arguments in this order: the butterflys name, number of wings, wing color, and favorite flower. Also, add a method to the Butterfly class called `speciesDataReport()`. This method should **override** the method with the same name in Insect. In this method, create and return a String containing all the info for a Butterfly object its name, wing color, number of wings, number of legs, and favorite flower. The **Bee** class represents a bee species. It will be a subclass of Insect. Bee needs to have two variables: * A String called `bodyColor` for the bees body color * A boolean called `makesHoney` for whether this species of bee makes honey (not all bees do) Add get and set methods for `bodyColor` and `makesHoney`. Call the methods for `makesHoney` getMakesHoney and setMakesHoney. If you create the methods with IntelliJ the default names might be different. Think about: should you make these variables public, private, package-protected, or protected? Do you need get and set methods for name and wingCount in Bee? Why or why not? Add a constructor to set all the variables a Bee object, has in this order; name, number of wings, body color, makes honey. And, Bee needs a method called `speciesDataReport()` that creates and returns a String containing all of the data for a Bee species. This method should **override** the method with the same name in Insect. This method has the same name as the Butterfly method, but prints out the Bee information. As it prints out the information, it should print This bee does make honey or this bee does not make honey instead of true or false. **Use the *Question_1_Insect_Manager* class to create some Insect, Bee and Butterfly objects.** Test your Butterfly class by writing code in the Question_1_InsectManager class to create some test Butterfly object. Create two Butterfly objects, and then call the `speciesDataReport()` method on each to display all the data for each of the Butterfly objects. Suggested butterflies: * A Monarch butterfly has 4 wings, its wings are orange and black, and likes a plant called milkweed. * A Common Yellow Swallowtail butterfly has 4 wings, its wings are yellow and black, and likes a plant called milk parsley. Test your Bee class by writing code in the InsectManager class to create some test Bee object. Create two Bee objects, and then call the `speciesDataReport()` method on each to display all the data for each of the Bee objects. Suggested bees: * name = Honey bee, color = yellow and black, makes honey = true, number of wings = 4 * name= Bumble bee, color = yellow, black and white, makes honey = false, number of wings = 4 Last task: Create an ArrayList or LinkedList of Insects. Add all of your Bee and Butterfly objects to this list. Use a for each loop to iterate over your list and call `speciesDataReport()` for each Insect, like this, ``` LinkedListinsects = new LinkedList(); //Create and add some Butterfly and Bee objects to the list for (Insect i : insects) { String data = i.speciesDataReport(); System.out.println(data); } ``` For which object is the speciesDataReport being called for? Notice that the calls to `speciesDataReport` run the method of that name for the correct type of Insect. It will call the speciesDataReport from the Butterfly class if the Insect object in the list is a Butterfly, and it will call the speciesDataReport from the Bee class if the Insect object in the list is a Bee. So what's happening with the *abstract* Insect class? Can you make an Insect object? The first line of the class is `public abstract class Insect { //class definition follows` And it contains an abstract method, `public abstract void speciesDataReport();` So if you wrote code like this, `Insect i = new Insect();` The compiler will report an error. An abstract class is one that you can't make objects from. You can't make an Insect object from the Insect class. If you wrote `Insect i = new Insect();` in your program, verify that you see a compiler error, and then remove it. You can subclass an abstract class, (create classes that declare that they are a subclass of Insect) and the subclasses *can* be used to make objects. You can make Bee and Butterfly objects. Note that a Bee object is also an Insect object. A Butterfly object is also an Insect object. So why is this useful? It's helpful in a scenario where you have a general description class, but you know that you will be specializing it with more than one subclass; and only the subclasses will be doing tasks in your program. You can now specify required behavior in the (abstract) superclass and enforce that the subclasses will be able to provide this behavior too. Insect doesn't want to implement speciesDataReport. It only represents general Insect objects, not a particular species - so it doesn't make sense to implement a real speciesDataReport. It doesn't make much sense to make an Insect object. It's too vague to be useful in your program. But, it does make sense to create Bee or Butterfly objects. So, that's why we create an abstract speciesDataReport in Insect. Abstract, for the method, means that all Insect objects will have a method called speciesDataReport. But Insect doesn't want to define exactly what speciesDataReport does, and Insect doesn't need to implement it. So declare it as abstract in Insect, and the compiler will make sure all subclasses of Insect will create a speciesDataReport method. So, the compiler knows that every actual Insect object (in our case, Bee and Butterfly objects) will have to have an speciesDataReport. Abstract classes can define abstract methods. These are methods that you are requiring subclasses to have, but Insect doesn't need to decide how exactly it will be done. So anything that subclasses Insect will be required to have a `speciesDataReport` method, and each subclass can decide how it will be implemented - so each subclass can generate it's own specific speciesDataReport. This is useful if you need to guarantee that all subclasses need to have a particular behavior. We'll see some more examples of this when we look at GUIs. For example, we'll use the Java library class AbstractTableModel to create out own TableModel classes, that will be used to supply tabular data in a GUI. Finally, imagine you don't have the `abstract void speciesDataReport();` line in Insect. Comment this line out, and then look at your loop in the InsectManager class. The compiler will be reporting an error. The loop is printing Bee and Butterfly data, and these classes both have speciesDataReport. So why does the compiler complain? The Insect class doesn't have a method called speciesDataReport. While Bee and Butterfly both do, there is nothing to guarantee you wont add an Insect object to this LinkedList, and Insect does not have this method. So the compiler reports an error. Now uncomment this line `abstract void speciesDataReport();` and verify your code compiles and runs as expected.
NB: PLEASE REVIEW THE ABOVE INSTRUCTIONS AND ANSWER THE FOLLOWING " TODO QUESTIONS":
/** * Represents a Bee species. */ public class Bee { // TODO make this a subclass of Insect // TODO add a boolean called makesHoney // TODO add a String called bodyColor // TODO add a constructor to set all necessary values // TODO override the speciesDataReport to return a String with Bee information
/** * Represents a butterfly species */ public class Butterfly { // TODO make this a subclass of Insect // TODO add a String called wingColor. Add get and set methods // TODO add a String called favoriteFlower. Add get and set methods // TODO add a constructor to set all necessary values // TODO override the speciesDataReport method to return a String with Butterfly information
/** * Represents a general insect species */ public abstract class Insect { // TODO add a String called name // TODO add an int called wingCount // TODO add a static constant int called LEG_COUNT, set it to 6 // TODO add an abstract method called speciesDataReport that subclasses will be forced to override // speciesDataReport should take no arguments, and return a String. // You don't need to write the method body. Insect's subclasses will do that. }
/** * Represents a Bee species. */ public class Bee { // TODO make this a subclass of Insect // TODO add a boolean called makesHoney // TODO add a String called bodyColor // TODO add a constructor to set all necessary values // TODO override the speciesDataReport to return a String with Bee information
public class Question_1_InsectManager { public static void main(String[] args) { Question_1_InsectManager insectManager = new Question_1_InsectManager(); insectManager.testInsects(); } public void testInsects() { // TODO Create an ArrayList of Insect objects // TODO Create two example Butterfly objects and add them to the ArrayList // TODO Create two example Bee objects and add them to the ArrayList // TODO loop over the ArrayList and call speciesDataReport() for each Insect (Butterfly, Bee) object in the ArrayList } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
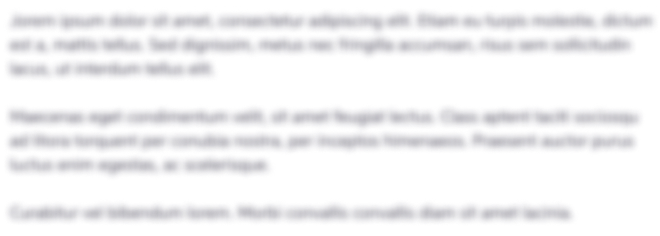
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started