Question
Problem 4: You are now going to create a class on your own that calculates the sales tax on a purchase and return the total
Problem 4: You are now going to create a class on your own that calculates the sales tax on a purchase and return the total price of the purchase. (This should be similar to the BankAccount class).
-
Create a class named SalesTax.
-
You will need two instance variables totalCost and taxRate. Each should be a double.
-
Create a constructor that initializes the instance variable, totalCost and tax. The constructor should be patterned after BankAccount with a default constructor and the totalCost set to 0 and the taxRate to a default of .05.
-
Create another constructor with one argument in the parameter. The argument will hold the tax. Set the taxRate to tax and the totalCost to 0.
-
Create a method called calculateTotal. (This method should be similar to Deposit) with no return value.
-
This method should accept as input the price of the purchase (a parameter variable called purchase)
-
Calculate the totalCost. (Think about the formula you will need to do this.) You will need to set totalCost = the formula you determine. You will return this totalCost in another method. Remember you should not return a value in a mutator method.
-
-
There should be a method getTotalCost.
-
This method has only one purpose and that is to return the totalCost. It returns a double. (See getBalance from BankAccount)
-
Write a SalesTaxTester class. This will be very similar to the BankAccountTester on page 101 of your text. It will have a main.
-
-
Create an object of type SalesTax using the default constructor.
-
Call the calculateTotal method. Use a purchase price of $50.
-
Print the results.
-
Create an object of type SalesTax and provide an argument of .08.
-
Call the calculateTotal method with a purchase price of $100.
-
Print the results.
-
Step by Step Solution
There are 3 Steps involved in it
Step: 1
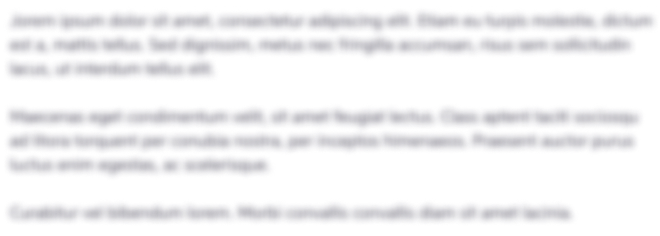
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started