Question
Problem 4.1 (Please Provide Full Code Like I Did with 3.3 Below) Thank you for your help. In this problem, we will read cars from
Problem 4.1
(Please Provide Full Code Like I Did with 3.3 Below)
Thank you for your help.
In this problem, we will read cars from a file, rather than typing them
in from the keyboard. Do the steps in order to build the solution to
this problem.
1. Copy and clean up code from problem 3.3
* Copy problem 3.3
* Change the name to Assignment 4, Problem 4.1
* Remove everything from the main function.
* Remove the execution results.
2. Modify the input function.
* Remove the & from the parameters in the function header, so they
are all values rather than references.
* Move the parameters from the function header and put them within
the input function, so they are now all local variables.
* Remove the parameters from the prototype for the input function,
so it matches the function header.
* At the bottom of the input function, declare a Car object named
temp using the constructor that takes the five parameters.
* Use the Car output function to print the Car.
* Call the input function from the main function with no arguments.
3. Create a file and use it for input. This is good because we will be
using the input many times.
* Create a file named cardata.txt (or use the one provided),
containing the following three lines of data (omit the heading
Line). If you create your own, make sure to follow the format.
Type ARR number kind loaded destination
Car CN 819481 maintenance false NONE
Car SLSF 46871 tank true Memphis
Car AOK 156
tender true McAlester
* In the input function, declare an object of type ifstream named
inputFile, which we will use to read from the file.
* At the beginning of the code for the input function, open the
file. If the open fails, send a message to stderr and exit the
program.
* In all the reads within the input function, remove the user
prompt and read from the inputFile object, rather than reading
from the stdin object.
* In the input function declare a string named type. Read the Type
field before reading the ARR field. We do not need the Type
field yet, but we need to read it to get it out of the way.
* Hint: We need to use getline when reading the destination.
using >> skips leading white space before reading the data.
getline does not skip this leading whitespace. So, before using
getline use the following code:
while(inputFile.peek() == ' ')
inputFile.get();
peek looks at the next character you are about to read. If it is
a space, get is used to read the space character, to get it out
of the way.
* Use a loop to read each line from the file. To do this use a
while loop including all the reading in the input function, as
well building and output of the Car.
Hint: you can do this with the following while statement:
while(inputFile.peek() != EOF)
The peek function will return EOF is there is no next character.
* At the bottom of the input function, close the file.
Order of functions in the code:
1. main
2. Car member functions
1. constructors in the order
1. default constructor
2. copy constructor
3. other constructors
2. output
3. setUp
3. operator== with Car parameters
4. input
Put an eye catcher before the beginning of each function, class, and the
global area:
// class name function name comment(if any)
*************************************************
3.3 CODE:
#include
using namespace std;
void input(string &reportingMark, int &carNumber, string &kind, bool &loaded, string &destination);
class Car { private: static const int FIELD_WIDTH = 22; string reportingMark; int carNumber; string kind; bool loaded; string destination;
public: Car() { setUpCar(reportingMark = "", carNumber = 0, kind = "other", loaded = false, destination ="NONE"); } Car(const Car &userCar) { setUpCar(userCar.reportingMark, userCar.carNumber, userCar.kind, userCar.loaded, userCar.destination); } Car(string reportingMark, int carNumber, string kind, bool loaded, string destination) { setUpCar(reportingMark, carNumber, kind, loaded, destination); } ~Car(){};
friend bool operator==(const Car &car1,const Car &car2); void setUpCar(string reportingMark, int carNumber, string kind, bool loaded, string destination); void output();
};
int main() { string reportingMark; int carNumber; string kind; bool loaded; string destination;
input(reportingMark, carNumber, kind, loaded, destination);
Car car1(reportingMark, carNumber, kind, loaded, destination); Car car2(car1); Car car3;
cout << endl << "Contents of car1:" << endl; car1.output();
cout << endl << "Contents of car2:" << endl; car2.output();
cout << endl << "Contents of car3:" << endl; car3.output(); if (car1 == car2) cout << "car1 is the same car as car2 "; else cout << "car1 is not the same car as car2 "; if (car2 == car3) cout << "car2 is the same car as car3 "; else cout << "car2 is not the same car as car3 "; //added code car2 = Car("UP",81002,"box",true,"Spokane");
cout << endl << "Contents of car1:" << endl; car1.output();
cout << endl << "Contents of car2:" << endl; car2.output();
cout << endl << "Contents of car3:" << endl; car3.output(); }
void Car::setUpCar(string reportingMark, int carNumber, string kind, bool loaded, string destination) { this->reportingMark = reportingMark; this->carNumber = carNumber; this->kind = kind; this->loaded = loaded; this->destination = destination; }
void Car::output() { cout << setw(FIELD_WIDTH) << "Reporting Mark: " << setw(FIELD_WIDTH) << reportingMark << endl; cout << setw(FIELD_WIDTH) << "Car Number: " << setw(FIELD_WIDTH) << carNumber << endl; cout << setw(FIELD_WIDTH) << "Kind: " << setw(FIELD_WIDTH) << kind << endl; cout << setw(FIELD_WIDTH) << "Loaded : " << setw(FIELD_WIDTH); if(loaded) cout << "Loaded" << endl; else cout << "Not Loaded" << endl; cout << setw(FIELD_WIDTH) << "Destination: " << setw(FIELD_WIDTH) << destination << endl; }
bool operator==(const Car &car1,const Car &car2) { if((car2.reportingMark.compare(car1.reportingMark) == 0) && car2.carNumber == car1.carNumber) return true; else return false; }
void input(string &reportingMark, int &carNumber, string &kind, bool &loaded, string &destination) { string isLoaded = ""; cout << "Enter Reporting Mark: "; cin >> reportingMark; cout << "Enter Car Number: "; cin >> carNumber; cout << "Enter kind: "; cin >> kind; cout << "Enter loaded : "; cin >> isLoaded;
if(isLoaded == "true") loaded = true; else loaded = false; cout << "Enter destination : "; cin.ignore(); getline(cin, destination);
while(loaded && destination == "NONE") { cout << "Since the car is loaded it must have a destination, please enter destination: "; getline(cin, destination); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
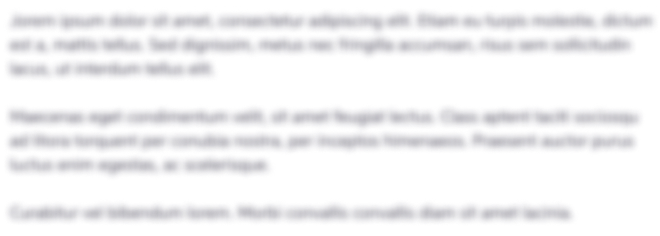
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started